Introduction into Automation & Cypress.io
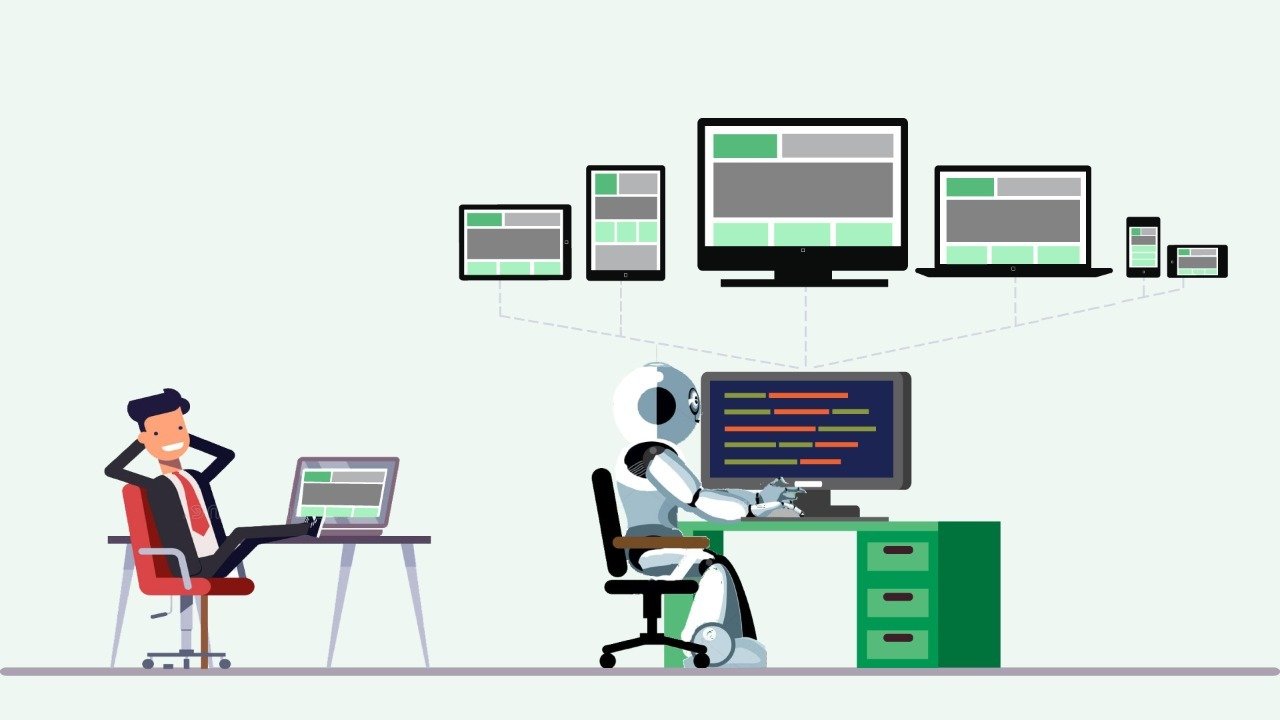


This is a generic view of a page
Looking closely we can distinguish it's sections

Basic concepts
- Page Object
- Driver Object
- Spec test file

Page Object
export class AdminLoginPage {
getSubmitButton(): Cypress.Chainable<JQuery<HTMLElement>> {
return cy.get('button[type="submit"]');
}
getEmail(): Cypress.Chainable<JQuery<HTMLElement>> {
return cy.get('#email');
}
getSignInLogo(): Cypress.Chainable<JQuery<HTMLElement>> {
return cy.get('.MuiSvgIcon-root');
}
getPassword(): Cypress.Chainable<JQuery<HTMLElement>> {
return cy.get('#password');
}
getEmailAddressLabel(): Cypress.Chainable<JQuery<HTMLElement>> {
return cy.get('label[for="email"]');
}
getPasswordLabel(): Cypress.Chainable<JQuery<HTMLElement>> {
return cy.get('label[for="password"]')
}
getFavicon(): Cypress.Chainable<JQuery<HTMLElement>> {
return cy.get('head link[rel="shortcut icon"]');
}
}
Driver Object
class LoginDriver {
constructor() {
this.page = new LoginPage();
}
clearLoginForm() {
this.page.getUserNameInput().type(EMPTY_STRING);
this.page.getPasswordInput().type(EMPTY_STRING);
}
isSubmitButtonDisabled() {
return this.page.getSubmitButton().disabled;
}
isSubmitButtonEnabled() {
return !this.isSubmitButtonDisabled()
}
}
Spec file
describe('Login page test suite', () => {
let driver;
beforeEach(() => {
driver = new LoginDriver();
});
it('should have the submit button disabled when credentials are missing', () => {
driver.clearLoginForm();
expect(driver.isSubmitButtonDisabled()).toBe(true);
});
});
Cypress
- Test runner review
- Demo

Cypress in a nutshell
- In Cypress - Page object and driver are combined
- Cypress main object is global (cy)
-
Supports a chainable fluent like API
-
cy.get('.product').should('not.exist');
-
cy.get('.product').its('length').should('be.eq',list.length);
-
-
Supports a promise like API - but it's NOT
-
cy.get('.nav').then(($nav) => {})
-
-
If an element is not found - an error is thrown and the test fails
- You can still explicitly use assertions
- It's better to use data-cy, data-test, data-testid for selectors
- For repeated actions - consider using commands.js
- Async tasks are defined as a plugin
- Fixtures
https://github.com/Cymbio/cymbio-automation-tests
The End
Introduction into Automation & Cypress.io
By Eyal Mrejen
Introduction into Automation & Cypress.io
- 95