What is JS?????
-
Javascript is a high level, interpreted programming language. It is dynamic, weakly typed, prototype based, single threaded language.
-
Javascript is initially created as a client side language for browsers.
-
But, now Javascript is used in other places like Node JS in server and React Native in Mobile platform.
JS an Intro
Dynamic Language
Dynamic programming language is a class of high level programming language which, at runtime, execute many common programming behaviors that static programming languages perform during compilation.
In Javascript, you can change a variable which was defined as string during initialization to number on runtime. But, this is not possible on static programming language. For eg,
var str = "name";
str = 1000;
In the above example, str is initialized as "name" which is of data type string. But, later it is changed to a value called 1000 which is of data type number. We can do these things only in dynamic languages.
Weakly Typed Language
In Javascript, when we assign a value to a variable, there is no need to specify the data type of that variable. The interpreter will understand the typeof the variable implicitly on runtime.
We can understand the weakly typed nature of JS, using the following example,
var a = 7;
var b = "7";
var c = a + b; // 77
In the above example, a holds an integer value 7 and b hold a string value 7. When declaring the values for these variables we are not explicitly mentioning any data type. Also, when these two variables are added then then output will be a string "77".
In strongly typed language to achieve this both the variables should be of type string. So, we have to convert a to type of string. Since Javascript is weakly typed language it implicitly converts a to string and concat both the values.
Prototype Based Language
Javascript's inheritance works differently when compared to other classical languages like Java. Parent to child relationship in JS is based on the proto reference.
function Vehicle(type) {
this.type = type;
}
function Car(color, company, model) {
this.color = color;
this.company = company;
this.model = model;
}
var vehicle = new Vehicle("4 Wheeler");
Car.prototype = vehicle;
var car = new Car("red", "TATA", "TIGOR");
console.log(car.type); // "4 Wheeler"
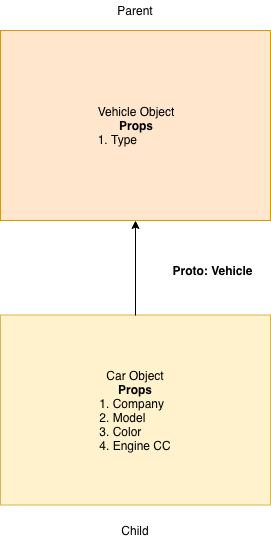
Single Threaded
Javascript is a single threaded language, which means at any given point of time only one instruction will be executed.
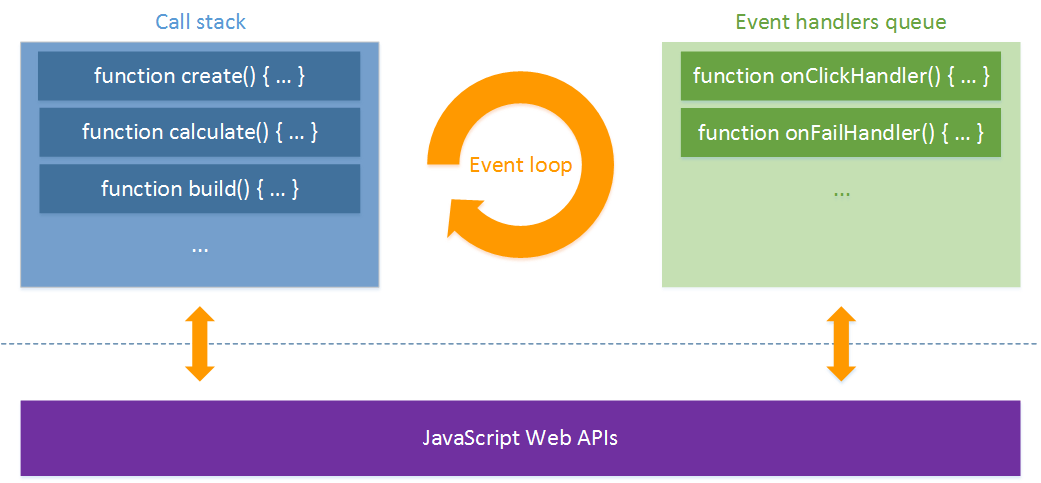
-
Since, each browser uses different Javascript Engine to interpret JavaScript.
-
So, It was necessary to maintain a standard across the browsers.
-
That's why, We have a standard created by ECMA International called "ECMAScript".
-
We have versions like ES5, ES6, ES7.
-
Browsers understandable version is ES5. But we code in ES6 which is then transpiled to ES5.
ECMA Standard
Some of the cool features of ES6 are,
Cool Features of ES6
- Class Keyword
- Arrow Functions
- const, let
- Spread Operator
- Default Parameters
- Module Exporting
- Promises
One Important Thing about JS
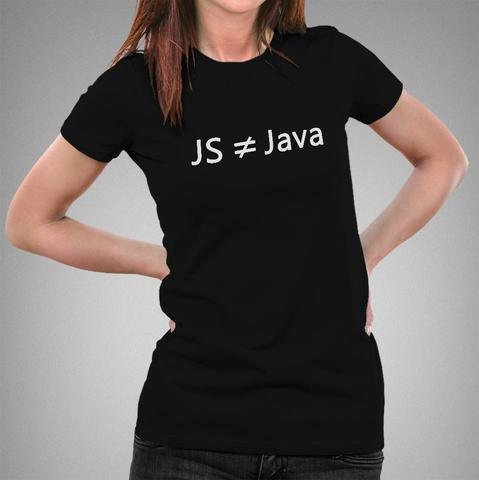
Popularity of Javascript
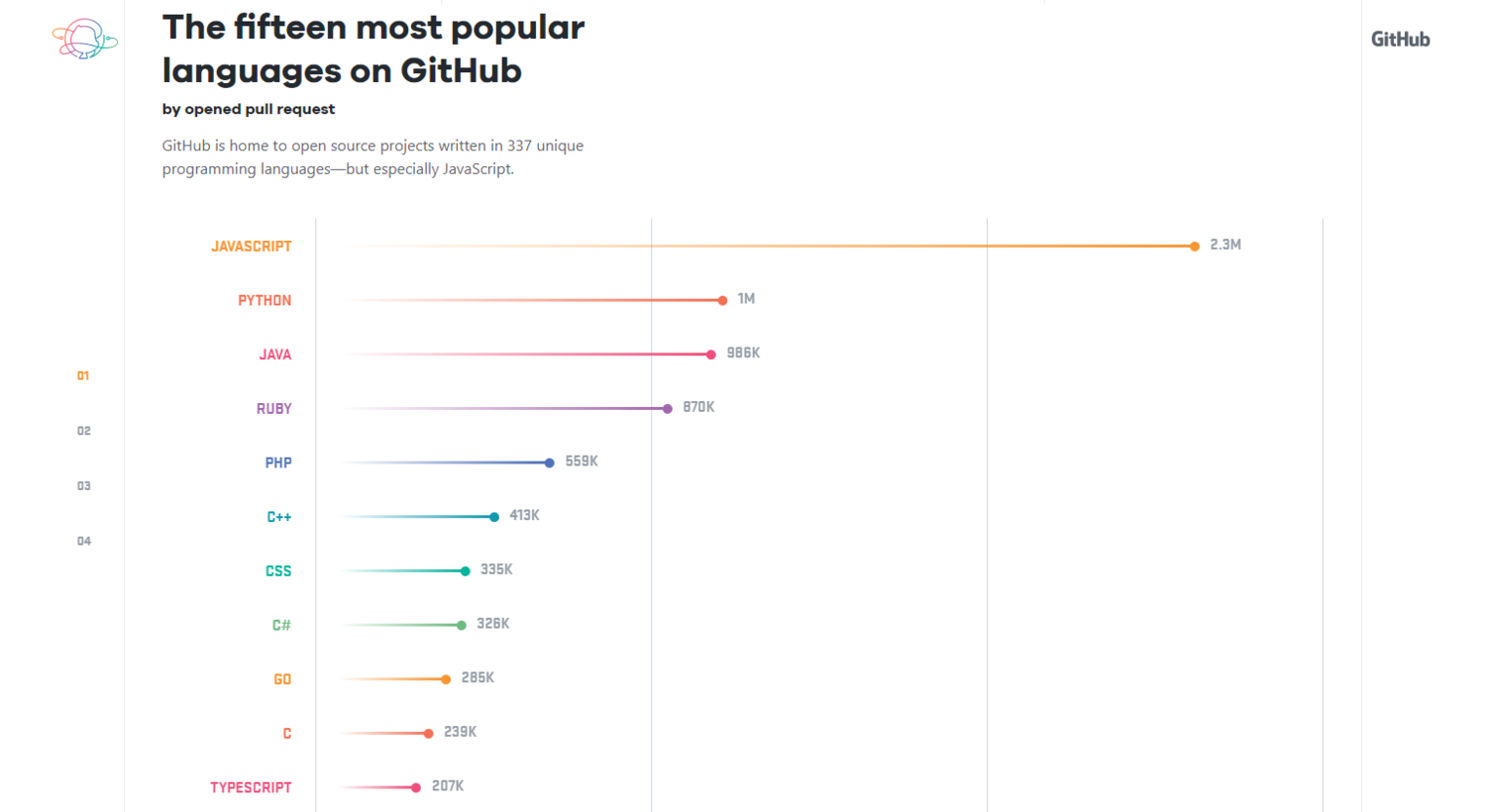
King of Web
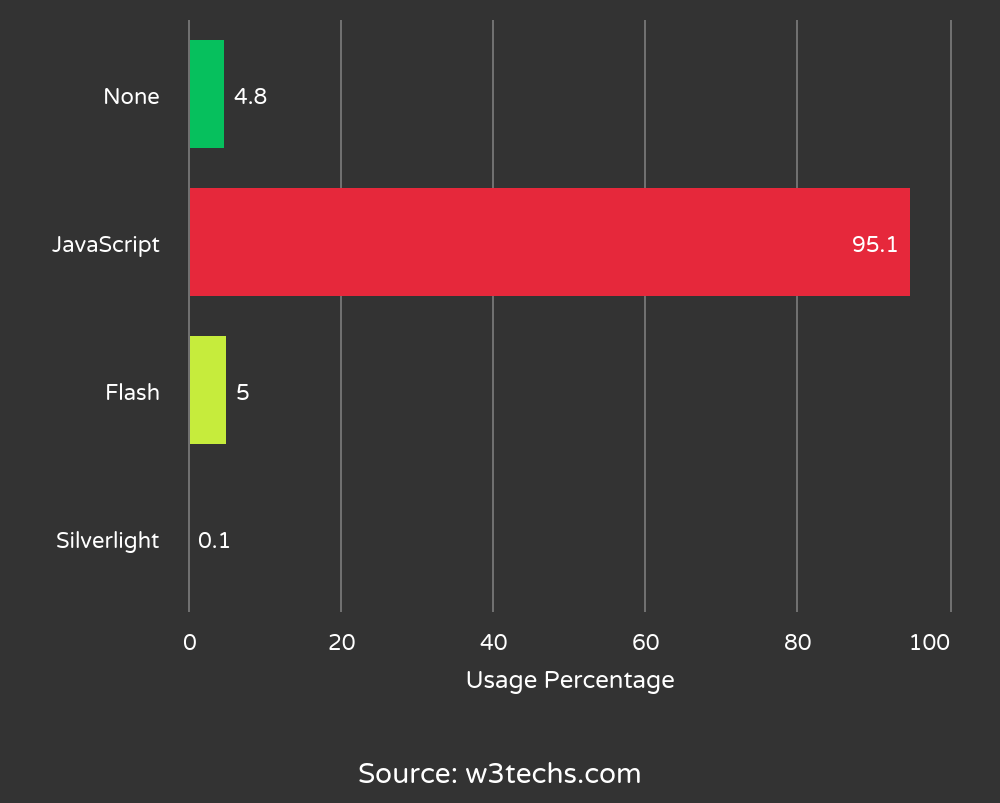
Where it is used?
Web Development
Javascript libraries like React, Vue, AngularJS are used to write client side part of an application
BackEnd Development
Javascript can be used in backend as well. Using NodeJS we can write backend rest API's.
Mobile App Development
Thanks to React Native. Using this we can write mobile native apps.
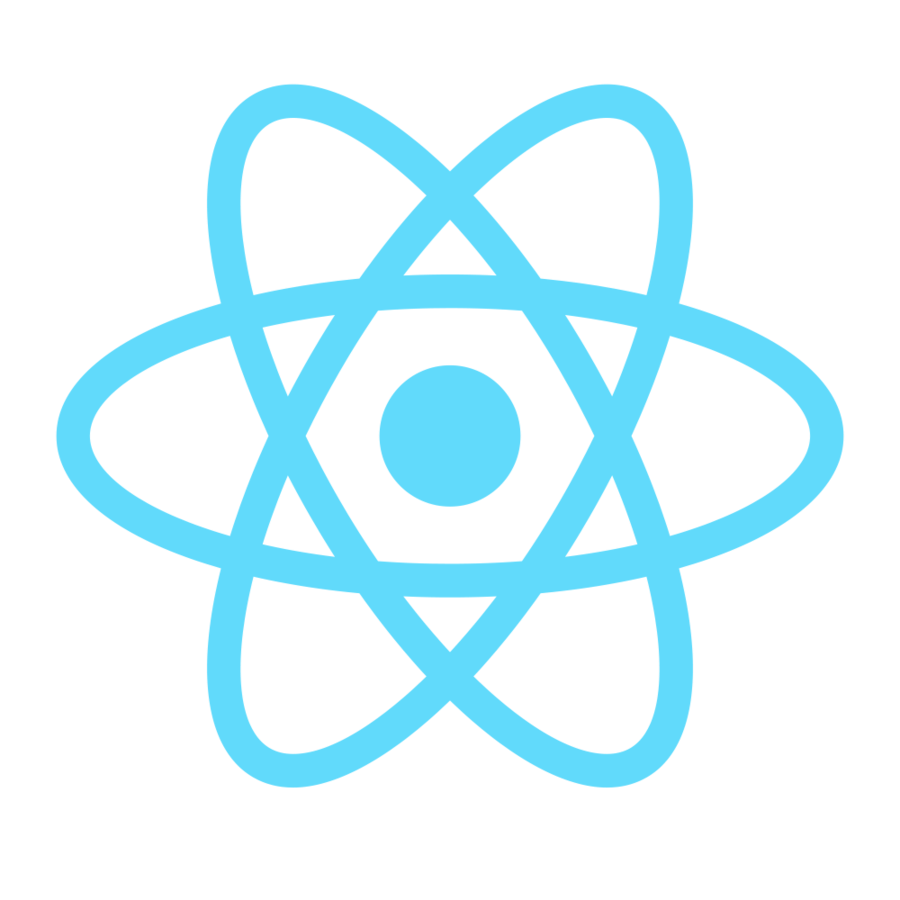
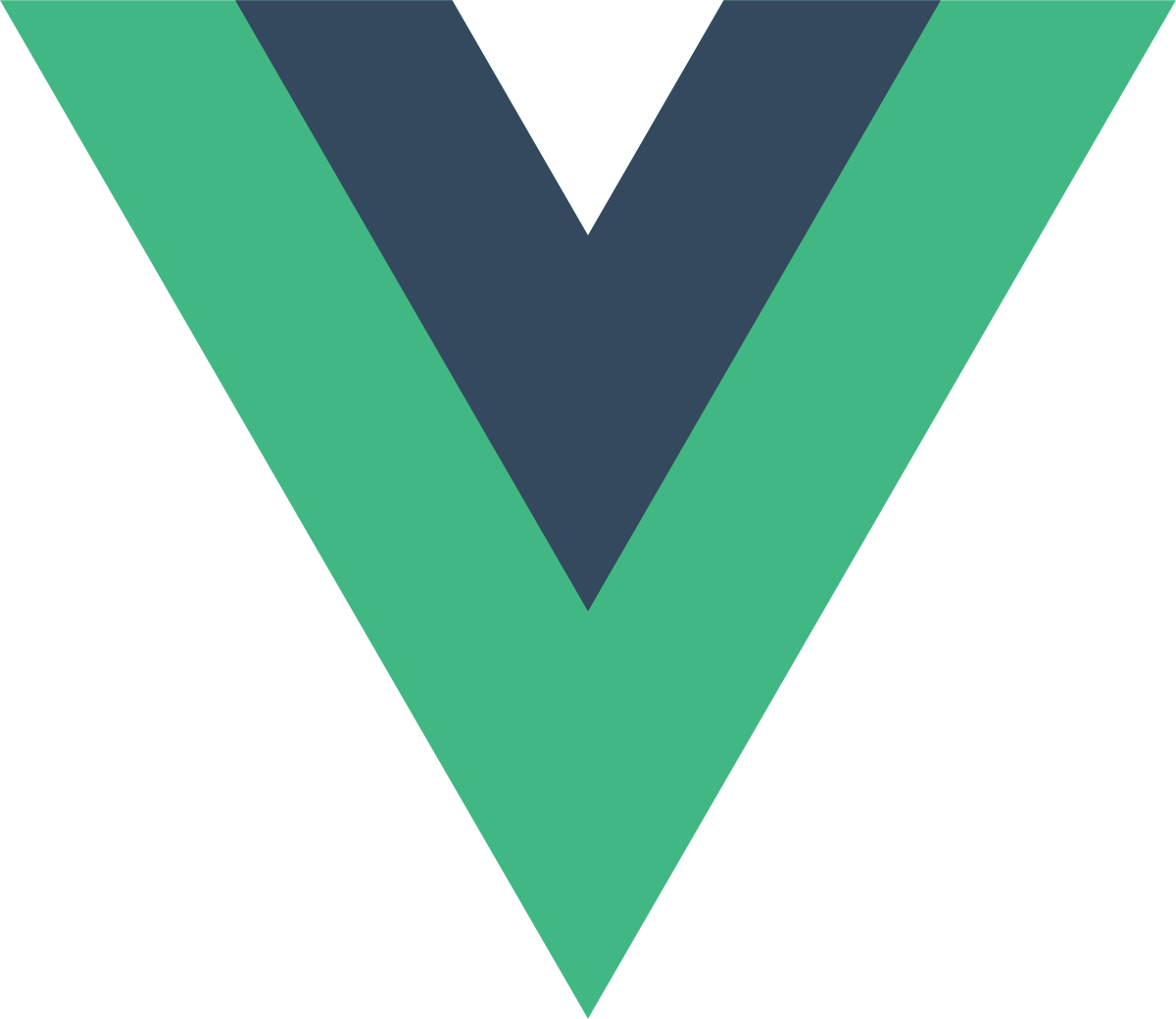
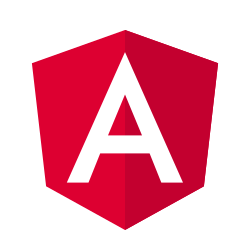
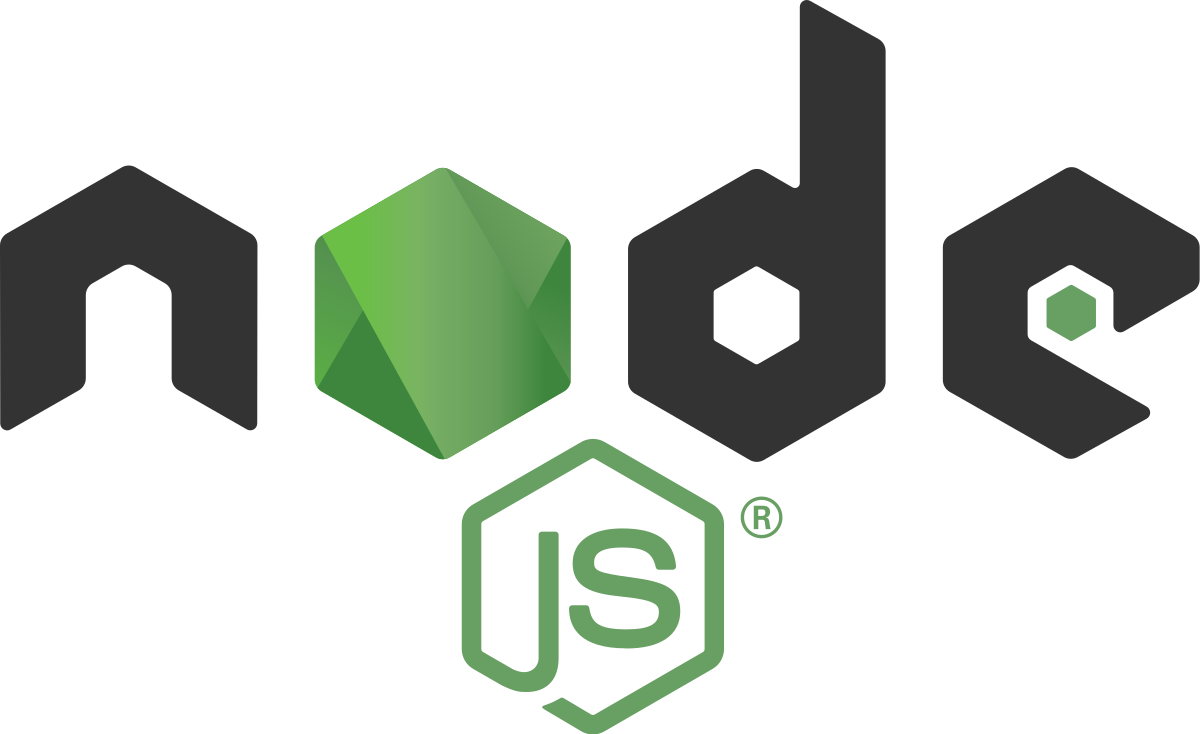

QUICK RECAP
What is JS?????
By hentry martin
What is JS?????
- 267