TECHNICAL SHARING
REACT
WORKSHOP

OUTLINE
ES6+
React
Redux
Unit Testing
webpack
ECMAScript
-
Created to standardize JavaScript, so as to foster multiple independent implementations.
-
Edition 5th - 2009
-
Edition 6th (ES6, ECMAScript 2015) - 2015
-
Edition 7th - 2016
-
Edition 8th - 2017
-
ECMAScript 2018 was finalized in January 2018
Brief History of
ES6+
-
let, const
-
Arrow Functions
-
Classes
-
Modules
-
Syntactic Sugar
ES6+
-
Block-scoped binding constructs
let, const
var x = 1
if (true) {
var x = 2
console.log(x)
// 2
}
console.log(x) // 2
let x = 1
if (true) {
let x = 2
console.log(x)
// 2
}
console.log(x) // 1
ES6+
-
Single-assignment
let, const
const foo = 'bar'
foo = 'baz' // throws an TypeError
const animal = { name: 'dog' }
animal.name = 'cat' // 'cat'
ES6+
-
Shorter functions
Arrow Functions
const arr = [ 1, 2, 3 ]
arr.map(function (e) {
return e + 1
})
arr.map((e) => {
return e + 1
})
arr.map(e => e + 1)
ES6+
-
No binding of this
Arrow Functions
this.name = 'ben'
const somebody = {
name: 'jas',
arrowFnGetName: () => this.name,
regularFnGetName: function() { return this.name }
}
console.log(somebody.arrowFnGetName()) // 'ben'
console.log(somebody.regularFnGetName()) // 'jas'
ES6+
- Syntactic sugar on top of prototype-based inheritance
Classes
function Animal (name) {
this.name = name
}
Animal.prototype.speak = function () {
console.log(this.name + ' makes some noise')
}
Animal.prototype.jump = function () {
console.log(this.name + ' jumps')
}
function Cat (name) {
Animal.call(this, name)
}
Cat.prototype = Object.create(Animal.prototype)
Cat.prototype.speak = function () {
console.log(this.name + ' meows')
}
var cat = new Cat('Tigger')
cat.speak() // 'Tigger meows'
cat.jump() // 'Tigger jumps'
class Animal {
constructor(name) {
this.name = name
}
speak () {
console.log(this.name + ' makes some noise')
}
jump () {
console.log(this.name + ' jumps')
}
}
class Cat extends Animal {
speak () {
console.log(this.name + ' meows')
}
}
const cat = new Cat('Tigger')
cat.speak() // 'Tigger meows'
cat.jump() // 'Tigger jumps'
- simpler & clearer syntax
ES6+
Modules
// foobar.js
const foo = 'foo'
export default foo
export const bar = 'bar'
// main.js
import foo, { bar } from 'foobar.js'
- Native support for modules
-
A Library for UI building
-
Thinking in Component (State Machine)
-
Props & States
-
Virtual DOM Tree
-
JSX (Declarative UI)
REACT
LIFECYCLE
REACT
- HTML written in JavaScript
- using {} to inject js
JSX
React.createElement(
'a',
{
href: 'https://google.com/'
},
'GOOGLE'
)
<a href='https://google.com/'>GOOGLE</a>
Common Mistakes
CASE 1
class MyComponent extends React.Component {
render() {
return (
<h1>Please click the button</h1>
// render button
<button>Click Me</button>
);
}
}
Common Mistakes
CASE 2
constructor(props) {
super(props);
this.state.name = '123'
}
render() {
return (
<div>
<h1>{`Hello! ${this.state.name}`}</h1>
</div>
);
}
Common Mistakes
CASE 3
constructor(props) {
super(props);
this.setState({
value: 'Foo'
})
}
componentDidMount() {
this.setState({
value: 'Foo'
})
}
Common Mistakes
CASE 4
class Demo extends React.Component {
constructor(props) {
super(props);
this.state = { greeting: 'Hello World!' }
}
render() {
<MyCustomComponent
id='custom_component'
class='components'
>
<h1>greeting</h1>
</MyCustomComponent>
}
}
Common Mistakes
CASE 5
class Demo extends React.Component {
constructor(props) {
super(props);
this.state = { greeting: 'Hello <br/> World!' }
}
render() {
return (
<MyCustomComponent>
<h1>{ this.state.greeting }</h1>
</MyCustomComponent>
)
}
}
Common Mistakes
CASE 6
class Demo extends React.Component {
handleChange(event) {
this.setState({ foo: 'foo' })
}
render() {
return (
<input onChange={this.handleChange} />
);
}
}
Common Mistakes
CASE 7
class Demo extends React.Component {
render() {
this.setState({ name: 'Kobe' });
return (
<div>`Miss ${name} so much...`</div>
);
}
}
Common Mistakes
CASE 8
componentDidMount() {
console.log(this.state.count) // count == 0
this.setState({ count: ++count });
console.log(this.state.count)
}
REACT
- Container Component (Smart Component)
- Presentational Component (Dumb Component)
- Function Components
- Props Validation
REACT
- propTypes
- defaultProps
- Dev environment works only
PROPS VALIDATION
REACT
Diff
-
A predictable state container
-
A data flow architecture
-
Inspired by Flux
REDUX
Core Concepts
STORE
ACTION
REDUCER
App state, a plain JavaScript object
A plain JavaScript object to change state
Takes state and action, returns next state
REDUX
Three Principles
-
Single source of truth
-
State is read-only
-
Changes are made with pure function
REDUX
Basic Data Flow

REDUX
Data Flow with Side Effects
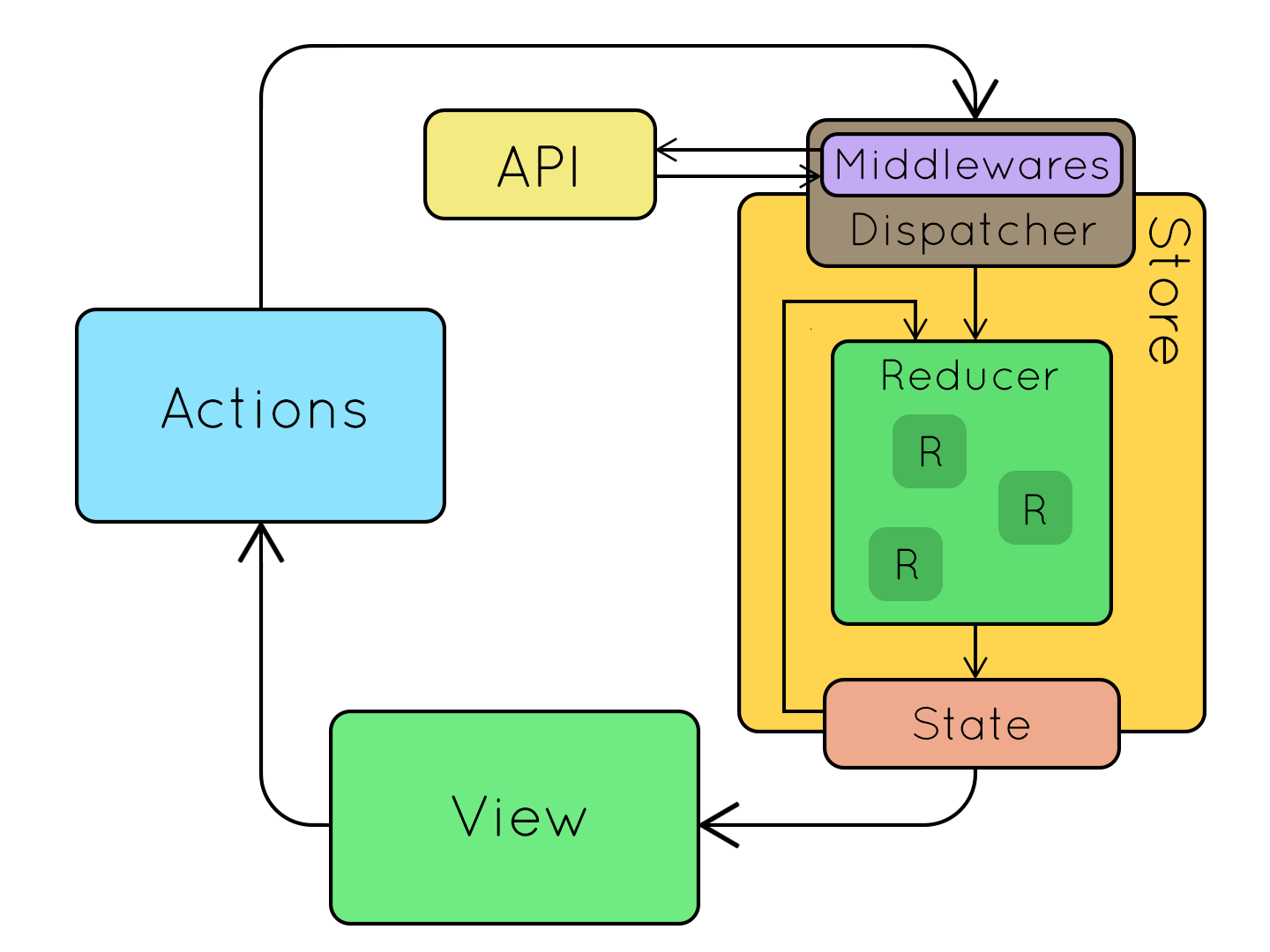
UNIT TESTING
UNIT TESTING
-
React Component Testing
-
Redux-related Testing
-
Action Creator Testing
-
Reducer Testing
-
-
Helpers/Libraries Testing
Snapshot Testing
Snapshot Testing
- Snapshot tests are a very useful tool whenever you want to make sure your UI does not change unexpectedly.
- Renders a UI component, takes a screenshot, then compares it to a reference image stored alongside the test.
- Snapshot Testing
webpack
-
A module bundler for modern JavaScript applications
-
Builds a dependency graph of modules, then packages them into a small number of bundles
WEBPACK
-
Entry
-
Output
-
Loaders
-
Plugins
React 16.3 - 16.5
-
Fragment, Context API, Refs, Strict Mode, New Lifecycles, Profiler
React 16.6
-
lazy, memo, contextType
Roadmap
One More Thing...
React 16.7 (alpha)
-
Hooks
REACT WORKSHOP @ SHOPLINE
By hinx
REACT WORKSHOP @ SHOPLINE
- 919