Ibrahim AshShohail
- Computer Science Graduate
- Senior Developer at Tamkeen Technologies
- Certified Zend Developer
- +8 years of PHP experience
- Open Source advocate, contributer to Laravel
What is a data structure?
- Organization of data
Big O notation O( )
- Describe the complexity of an algorithm
- Time and space
- Relative to the input size
- For O(1), size does not effect the algorithm
-
For O(log n), size effects the algorithm logarithmically
-
For O(n), size effects the algorithm proportionally
- For, O(n^2), size effects the algorithm exponentionally
Examples
$array = [1132, 2512, 4734, 5845, 7453];
// How long will this take?
$array[2];
function getIndex($array, $val) {
foreach ($array as $i => $value) {
if ($value == $val) {
return $i;
}
}
}
// What about this?
getIndex($array, rand(1, 100000);
$array = range(1, 1000);
What if:
PHP Arrays!!!
An array in PHP is actually an ordered map. A map is a type that associates values to keys. This type is optimized for several different uses; it can be treated as an array, list (vector), hash table (an implementation of a map), dictionary, collection, stack, queue, and probably more. As array values can be other arrays, trees and multidimensional arrays are also possible.

Trying to be a lot of things in the same time can be UGLY

Or COOL
PHP Arrays
- Large waste of memory
- 14,649,072 bytes
= 13.97 MBs
SplFixedArray
- Better for real arrays
-
5,600,648 bytes
= 5.34 MBs
$array = range(1, 1000000);
$array = new SplFixedArray(100000);
for ($i = 0; $i < 100000; ++$i) {
$array[$i] = $i;
}
Using an Array
- Memory: 81.87 MBs
- Time: 0.093 ms
Using a Class
- Memory: 49.37 MBs
- Time: 0.238 ms
$array[] = [
'username' => 'username',
'password' => 'password',
'email' => 'e@mail.com',
'age' => 25,
]
$array[] = new User(
'username',
'password',
'e@mail.com',
25
);
Creating 100,000 Users
Classes are 2.5 times slower than arrays
What are true arrays?
- Fixed-size integer-indexed collections
0
1
3
2
When to use arrays?
- Fixed size collection
- Maintain order
- Not linked
- Memory efficient
SplFixedArray
class SplFixedArray implements Iterator , ArrayAccess , Countable {
public __construct ([ int $size = 0 ] )
public count() : int
public current() : mixed
public fromArray(array $array [, bool $save_indexes = true ] ) : static
public getSize() : int
public key() : int
public next() : void
public rewind() : void
public setSize(int $size ) : int
public toArray() : array
public valid() : bool
}
Lists
Types of Lists
- Singly-linked lists
- Doubly-linked lists



SplDoublyLinkedList
class SplDoublyLinkedList implements Iterator , ArrayAccess , Countable {
public __construct()
public add(mixed $index , mixed $newval) : void
public top() : mixed
public bottom() : mixed
public count() : int
public current() : mixed
public isEmpty() : bool
public key() : mixed
public next() : void
public pop() : mixed
public prev() : void
public push(mixed $value) : void
public rewind() : void
public shift() : mixed
public unshift(mixed $value) : void
public valid() : bool
public serialize() : string
public unserialize(string $serialized) : void
}

Queues
Queue Representation
HEAD
TAIL
Dequeue
Enqueue
HEAD
TAIL
When to use a Queue?
- FIFO
- Sequential Processing
- Size doesn't matter
- Messaging
- Priority Queues?
<?php
interface Queue
{
// Enqueue an item
public enqueue($item);
// Dequeue the first item
public dequeue();
}
// (Optional) What is the first item
public first();
// (Optional) What is the last item
public last();
// (Optional) How can we know the number of elements?
public count();
// (Optional) What's the O() of this method?
public contains();
SplQueue
SplQueue extends SplDoublyLinkedList implements Iterator , ArrayAccess , Countable {
public __construct()
public dequeue() : mixed
public enqueue(mixed $value) : void
public SplDoublyLinkedList::isEmpty() : bool
public SplDoublyLinkedList::count() : int
public SplDoublyLinkedList::add(mixed $index , mixed $newval) : void
public SplDoublyLinkedList::push(mixed $value) : void
public SplDoublyLinkedList::pop() : mixed
public SplDoublyLinkedList::shift() : mixed
public SplDoublyLinkedList::unshift(mixed $value) : void
public SplDoublyLinkedList::top() : mixed
public SplDoublyLinkedList::bottom() : mixed
public SplDoublyLinkedList::current() : mixed
public SplDoublyLinkedList::key() : mixed
public SplDoublyLinkedList::next() : void
public SplDoublyLinkedList::prev() : void
public SplDoublyLinkedList::rewind() : void
public SplDoublyLinkedList::valid() : bool
}
Stack

Stack Representation
TOP
POP
PUSH
TOP
TOP
Stack Overflow ?
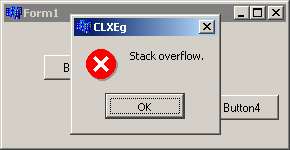
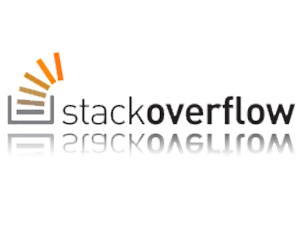
When to use a Stack?
- LIFO
- Keeping record of progress
- Syntax parsing (parenthesis)
<?php
interface Stack
{
// Pop an item
public pop();
// Push an item to the stack
public push($item);
}
// (Optional) What is the first item
public top();
// (Optional) What is the last item
public bottom();
// (Optional) How can we know the if the stack is empty?
public isEmpty();
// (Optional) What's the O() of this method?
public contains();
SplStack
SplQueue extends SplDoublyLinkedList implements Iterator , ArrayAccess , Countable {
public __construct()
public SplDoublyLinkedList::isEmpty() : bool
public SplDoublyLinkedList::count() : int
public SplDoublyLinkedList::add(mixed $index , mixed $newval) : void
public SplDoublyLinkedList::push(mixed $value) : void
public SplDoublyLinkedList::pop() : mixed
public SplDoublyLinkedList::shift() : mixed
public SplDoublyLinkedList::unshift(mixed $value) : void
public SplDoublyLinkedList::top() : mixed
public SplDoublyLinkedList::bottom() : mixed
public SplDoublyLinkedList::current() : mixed
public SplDoublyLinkedList::key() : mixed
public SplDoublyLinkedList::next() : void
public SplDoublyLinkedList::prev() : void
public SplDoublyLinkedList::rewind() : void
public SplDoublyLinkedList::valid() : bool
}
Trees
Larry D. Moore CC BY-SA 3.0 http://en.wikipedia.org/wiki/The_Big_Tree,_Rockport#mediaviewer/File:Big_tree.jpg


Tree Representation

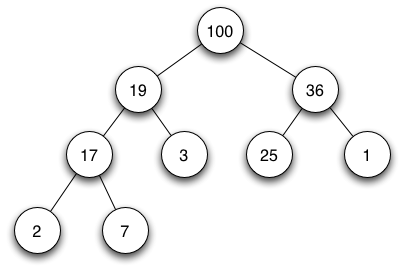
Types of trees
- Binary trees
- Heaps
- AVL Trees
- ...
- http://en.wikipedia.org/wiki/List_of_data_structures#Trees
SplHeap
abstract class SplHeap implements Iterator , Countable {
public __construct()
abstract protected int compare(mixed $value1 , mixed $value2)
public count() : int
public current() : mixed
public extract() : mixed
public insert(mixed $value) : void
public isEmpty() : bool
public key() : mixed
public next() : void
public recoverFromCorruption() : void
public rewind() : void
public top() : mixed
public valid() : bool
}
Traversal strategies
- Pre-order
- In-order
- Post-order
- Level-order

SPL alternatives?
- Your own implementation?
- Please no...
- https://github.com/morrisonlevi/Ardent
- https://github.com/morrisonlevi/SPL-Collections
Thank you
@ibrasho
ibra.sho@gmail.com
Watering the Trees: An introduction to data structures
By Ibrahim AshShohail
Watering the Trees: An introduction to data structures
- 212