JavaScript Crash Course
Масиви
4
Зміст:
- Масиви в JavaScript
- Створення масивів
- Модифікація масивів
- Використання
- Бонус*
Масиви в JavaScript
Що таке масив (array)?
Клас Array в JavaScript — це глобальний об’єкт, який використовується при побудові масивів даних, схожих на список.

Що може міcтити масив?
Масив може містити, як дані одного типу, так і дані різних типів.

1
"Text"
{ n: 1}
[]
Щоб дізнатись, яка кількість елементів в масиві:
myArray.length
Як отримати елемент із масива?
Кожен елемент масива отримує свій індекс - це цілі числа, починаючи із 0.

1
"Text"
{ n: 1}
[]
Щоб отримати елементи із масива:
1
0
2
3
myArray[0];
myArray[2];
myArray[1];
Створення масивів
Створення масивів
// empty array to modify later
let emptyArray = [];
// array with nums
const numsArray = [1, 2, 3, 4];
// array with strings
const strArray = ['there', 'can', 'be', 'strings'];
// mix of different data types
const mixArray = [1, 'a', { n: 1 }, [1,2,3]];
Створення масивів з використанням класу Array
// create an empty array
const nums = new Array();
// add numbers
nums.push(1);
nums.push(2);
nums.push(3);
// print array
console.log(nums);
Доступ до елементів масива
// array with strings
const strArray = ['there', 'can', 'be', 'strings'];
console.log(strArray[1]); // output 'can'
console.log(strArray[0]); // output 'there'
// mix of different data types
const mixArray = [1, 'a', { n: 1 }, [1,2,3]];
console.log(mixArray[0]); // output 1
console.log(mixArray[2]); // output { n: 1 }
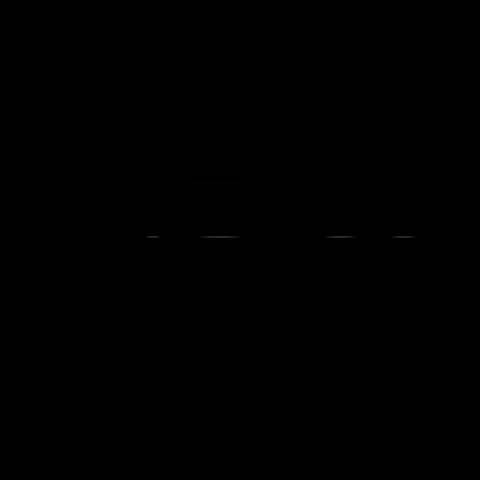
Модифікації масивів
Модифікація масива
// array with mixed types
const mixArray = [1, 'str', 'there', { a: 1 }];
// to add a new element
mixArray[4] = 100;
// to change value
mixArray[0] = 0;
// delete element
delete mixArray[4];
Методи для модифікації масивів
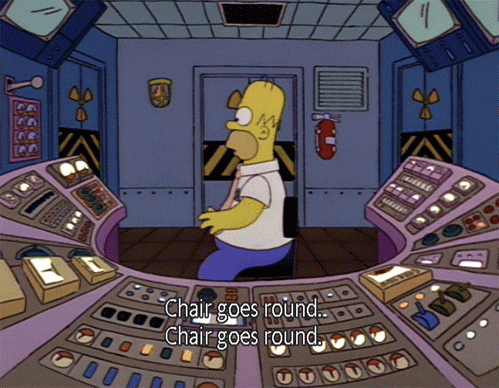
Метод push(): додавання нового елемента
// array with mixed types
const mixArray = [1, 'str', 'there', { a: 1 }];
// to add a new element
mixArray.push(100);
mixArray.push({ b: 10 });
mixArray.push('test');
Метод pop(): видалення останнього елемента
// array with mixed types
const mixArray = [1, 'str', 'there', { a: 1 }];
// remove last 2 elements
mixArray.pop();
mixArray.pop();
console.log(mixArray); // [1, 'str']
Метод unshift(): додавання на початок елементів
// array with mixed types
const mixArray = [1, 'str', 'there', { a: 1 }];
// to add new elements
mixArray.unshift(100);
mixArray.unshift(33, 101);
console.log(mixArray); // output [33, 101, 100, 1, 'str', 'there', {…}]
Метод shift(): видалення першого елемента
// array with nums
const nums = [1, 2, 3, 4];
// remove 2 first elements
nums.shift(); // return 1
nums.shift(); // return 2
console.log(nums); // [3, 4]
Метод concat(): об'єднання масивів
const ar1 = [1, 2];
const ar2 = [3, 4];
// concat arrays
const result = ar1.concat(ar2);
console.log(result); // [1,2,3,4]
Використання масивів
Отримання даних і їх використання
const data = [
{ user: 'John' },
{ user: 'Tom' },
{ user: 'Petr' },
];
// use data
function greeting(userName) {
return "Hello, " + userName + "!";
}
greeting(data[0].user);
greeting(data[1].user);
greeting(data[2].user);
// later we will use it together with loops :)
Сторення нових даних
const data = [];
// add data
function add(obj, arr) {
arr.push(obj);
}
add({ n: 1 }, data);
add({ n: 3 }, data);
add({ n: 5 }, data);
// later we will use it together with loops :)
Бонус
Стартове значення параметру функції
function extendConfig(objExt, config = {}) {
const newObj = Object.assign({}, config, objExt);
return newObj;
}
const result = extendConfig(
{ test: true, v: '10.0.3'},
{ module: 'Car'}
);
console.log(result);
Дефолтне значення параметру функції + spread operator
function extendConfig(objExt, config = {}) {
return {
...objExt,
...config,
};
}
const result = extendConfig(
{ test: true, v: '10.0.3'},
{ module: 'Car'}
);
console.log(result);
Деструктуризація об'єкта
const config = {
name: 'MyTests',
tests: 'unit',
version: '10.0.3',
extra: {
tests: 'e2e',
}
}
const { tests, extra } = config;
console.log(tests, extra);
Деструктуризація масива
const data = [
'Games',
{
tests: 'e2e',
}
]
const [ moduleName, extra ] = data;
console.log(moduleName, extra);
Деструктуризація масива для перенесення елементів
function swap(arr, i, j) {
// change places of array elements
[arr[i], arr[j]] = [arr[j], arr[i]];
}
const unsortedNums = [1, 3, 2];
swap(unsortedNums, 1, 2);
console.log(unsortedNums);
Q & A
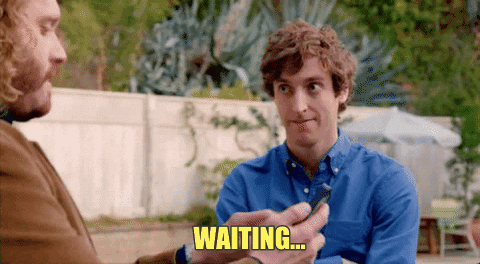
6 JavaScript Crash Course
By Inna Ivashchuk
6 JavaScript Crash Course
- 542