actors in the jvm
Irving Cordova
Threads considered harmful

Threads considered harmful
-
Synchronization
-
Deadlock
All memory is shared, so access must be synchronized
Use of shared resources that are locked opens the possibility of deadlocks
Threads considered harmful
-
Hard to debug
-
Hard to optimize
Bugs hard to reproduce, harder to fix
Locks create contention, which can slow performance.
Threads considered harmful

In short you need to be a wizard
alternatives?

Alternatives?

Alternatives?


The Actor ModeL
The Actor Model

The Actor Model
The Actor Model
The Actor Model

Surely not in my jvm
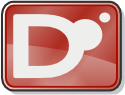

Surely not in my JVM

Surely in my JVM!

The project

AkkA
- public class Greeting implements Serializable {
- public final String who;
- public Greeting(String who) { this.who = who; }
- }
- public class GreetingActor extends UntypedActor {
- LoggingAdapter log = Logging.getLogger(getContext().system(), this);
- public void onReceive(Object message) throws Exception {
- if (message instanceof Greeting)
- log.info("Hello " + ((Greeting) message).who);
- }
- }
- ActorSystem system = ActorSystem.create("MySystem");
- ActorRef greeter = system.actorOf(new Props(GreetingActor.class), "greeter");
- greeter.tell(new Greeting("Charlie Parker"));
Akka

akka

AKKA
Pros
- Conceptually simple
- Can be used as a library
- Not intrusive
- Can use remote actors
AKKA
Cons
- Cannot call new for actors
GPARS
GPARS
GPars
public class StatelessActorDemo {public static void main(String[] args) throws InterruptedException { final MyStatelessActor actor = new MyStatelessActor(); actor.start(); actor.send("Hello"); actor.sendAndWait(10); actor.sendAndContinue(10.0, new MessagingRunnable<String>() { @Override protected void doRun(final String s) { System.out.println("Received a reply " + s); } }); } }
class MyStatelessActor extends DynamicDispatchActor { public void onMessage(final String msg) { System.out.println("Received " + msg); replyIfExists("Thank you"); }
public void onMessage(final Integer msg) { System.out.println("Received a number " + msg); replyIfExists("Thank you"); }
public void onMessage(final Object msg) { System.out.println("Received an object " + msg); replyIfExists("Thank you"); } }
GPars

Gpars
Pros
- Very Simple to use
- Integrates cleanly
- Not intrusive
- Clean code for receiving messages
- Easy to reply to messages
Gpars
Cons
- No support for remote actors
- No built-in routing
- There is not as much support
- Groovy is not very performant
Jetlang

Jetlang
JETLANG
Fiber fiber = new ThreadFiber();
fiber.start();
final CountDownLatch latch = new CountDownLatch(2);
Runnable toRun = new Runnable(){
public void run(){
latch.countDown();
}
};
//enqueue runnable for execution
fiber.execute(toRun);
//repeat to trigger latch a 2nd time
fiber.execute(toRun);
latch.await(10, TimeUnit.SECONDS);
//shutdown thread
fiber.dispose();
Jetlang

Jetlang
Pros
- Fast
- Open Source
- No external dependencies
- Explicit control over channels and threads
Jetlang
Cons
- Syntax to use it is very obtrussive
- Programmer must become well acquainted with library
- Sometimes awkward to program
Questions?

actors in the jvm
By Irving Cordova
actors in the jvm
- 2,034