echo "Bash" > search & find
What You will Learn
- How to find a folder or a file
- Search for a pattern in a string or text
- manipulate a text using the command line
Find A File or A Folder
find MyFolder
find . -name "myFile.txt"
-
find
- a file path
- an expression
Find A File or A Folder
* - any number of characters
? - one character
[] - any of the characters inside the brackets
find . -name "*.html" # anything that ends with .html
find . -name "*.???" # anything that ends with a three letter file extension like .txt or .css
find . -name "[fts]*" # anything that starts with the letter f t or s
find . -name "*main*" # anything that has the text main somewhere in the filename
Find A File or A Folder
sudo apt install mlocate
Search inside a text
echo "Lisa
Mark
Elie
Beth
Tim
Elizabeth
Tom
Matt
Liza
Janey
Jane
Shana" >> people.txt
cat people.txt | grep Tim
Search inside a text
grep -i "elie" people.txt # case insensitive
grep -iw "beth" people.txt # case insensitive + full world
grep -v "Beth" people.txt # everything but
grep -c "Jane" people.txt # count
grep -ni "Jane" people.txt # print with line numbers
Search inside a text
grep -wc "...." people.txt
grep -wc "T.*" people.txt
grep -wc "[LME].*" people.txt
grep -wc "[^T].*" people.txt
The real power of grep shines when using regular expressions
PRACTICE
echo "Lisa
Mark
Elie
Beth
Tim
Elizabeth
Tom
Matt
Liza
Janey
Jane
Shana" >> people.txt
- I want all people with names starting by T
- All people with names of 5 or more characters
- All people not having a e in their name
PRACTICE - solution
grep "T" people.txt
grep "....." people.txt
grep -v "e" people.txt
# Thanks @delhaj ❤️
Regular Expression
cut
echo "Issam,Pokemon, 5
Marta,Extreme sports, 2
Dhirar,SublimeText, 1
JC,Stargate, 7
Wenting,Disney world, 6
Shuting,Bag Raiders, 3
Stephanie,Wolves, 4
Sebastien,Naruto, 8
Trung,CSGO, 10
Rahul,GOT, 9" > best_people_ever.txt
cat best_people_ever.txt | cut -d ',' -f 1 # Issam, Marta...
cat best_people_ever.txt | cut -d ',' -f 2 # Pokemon, Extreme sports...
Used to cut lines based on a delimiter or a number of characters
sort
echo "Issam,Pokemon, 5
Marta,Extreme sports, 2
Dhirar,SublimeText, 1
JC,Stargate, 7
Wenting,Disney world, 6
Shuting,Bag Raiders, 3
Stephanie,Wolves, 4
Sebastien,Naruto, 8
Trung,CSGO, 10
Rahul,GOT, 9" > best_people_ever.txt
cat best_people_ever.txt | sort -t ',' -k 1 # sort names
cat best_people_ever.txt | sort -t ',' -k 3 # sort by number ⚠️
Used to sort lines based
head & tail
echo "Issam,Pokemon, 5
Marta,Extreme sports, 2
Dhirar,SublimeText, 1
JC,Stargate, 7
Wenting,Disney world, 6
Shuting,Bag Raiders, 3
Stephanie,Wolves, 4
Sebastien,Naruto, 8
Trung,CSGO, 10
Rahul,GOT, 9" > best_people_ever.txt
# are these two lines below equivalent ?
cat best_people_ever.txt | sort -t ',' -k 3 -g | head -n 2
cat best_people_ever.txt | sort -t ',' -k 3 -g -r | tail -n 2
head -> output first lines of a file
tail -> output last lines of a file
Practice
echo "Issam,Pokemon, 5
Marta,Extreme sports, 2
Dhirar,SublimeText, 1
JC,Stargate, 7
Wenting,Disney world, 6
Shuting,Bag Raiders, 3
Stephanie,Wolves, 4
Sebastien,Naruto, 8
Trung,CSGO, 10
Rahul,GOT, 9" > best_people_ever.txt
- What music group Shuting listens to every single day ?
- I want all people's activity whose name length is higher than 6 sorted by their index (reversed)
SED
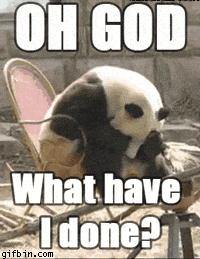
SED
Stream EDitor or Sed works as follows:

SED
Often used to search and replace or substitute, the basic form is the following
sed -e [line number or regular expression]s/regular-expression/replacement/[flags] text
echo "I have three dogs and two cats" > animals.txt
sed -e 's/dog/cat/g' -e 's/cat/elephant/g' animals.txt --debug
sed -e 's@dog@cat@g' -e 's@cat@elephant@g' animals.txt # equivalent
n replace nth instance of pattern with replacement
g replace all instances of pattern with replacement
SED
echo "line 1 (one)
line 2 (two)
line 3 (three)" > file.txt
sed -e '1,2d' file.txt
sed -e '3d' file.txt
sed -e '1,2s/line/LINE/' -e '/^line/'d file.txt
sed -e '1,2s/line/LINE/' -e '/^line/!'d file.txt
sed -e [line number or regular expression]d text
SED
echo "hello
this text is wiped out
Wiped out
hello (also wiped out)
WiPed out TOO!
goodbye
(1) This text is not deleted
(2) neither is this ... ( goodbye )
(3) neither is this
hello
but this is
and so is this
and unless we find another g**dbye
every line to the end of the file gets deleted" > text.txt
sed -e '/hello/,/goodbye/d' text.txt
sed -e [address1, address2]d text
SED
#!/bin/sh
X='word1\|word2\|word3\|word4\|word5'
sed -e "
/$X/!d
/$X/{
s/\($X\).*/&/
s/.*\($X\)/&/
q
}" $1
Sed can do much more with the use of subroutines
& refers to the first match (sometimes people use \1)
q forces the end of the evaluation
PRACTICE
echo "Pour jouer au tennis de tableen 2020, il faut:
1 table de tennis de table
2 raquettes
3 balles de ping pong
et de la bonne motivation !" > ping_pong.txt
-> 1 table de tennis de table !!!
-> 2 raquettes !!!
-> 3 balles de ping pong !!!
I want this !
sed -e '/^[0-9].*/!d' -e 's/^[0-9].*/-> & !!!/g' ping_pong.txt
awk
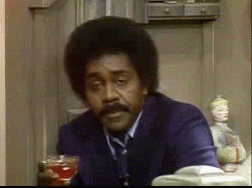
And you were thinking sed was powerful ?
awk
Awk is almost a programming language in itself. It can do any kind of text manipulation you can think of.

awk
echo "hey, how are you ?" | awk 'BEGIN{printf"---|Start|--\n"} {print} END{printf"---|End|---\n"}'
echo "hey, how are you ?
good and you" | awk 'BEGIN{printf"---|Start|--\n"} {sub(/you/ ,"we"); print} END{printf"---|End|---\n"}'
xargs
xargs command is used to build an execution pipeline from standard input.
echo 'one two three' | xargs mkdir
ls # one two three
find *.txt | xargs grep '1'
Practice
echo "Issam-Hammi-pizza
Rahul-Gakhar-vegetables
Wenting-Bao-steaks
Sebastien-Dubuc-budda bowl
Dhirar-Elhaj-tacos" > food.txt
- replace all - by : using sed
- return all first names and their favorite dishes separated by a space
Practice
echo "1>>>>2
2>>>>3
3>>>>4
4>>>>5" > numbers.txt
- Using cut print out just the numbers 2 3 4 5. Then use xargs to print them all on 1 line
- find all text files in your current directory and print all numbers
Bash - session 3
By Issam Hammi
Bash - session 3
Third session focused on text manipulation
- 540