Ajax and the XMLHttpRequest
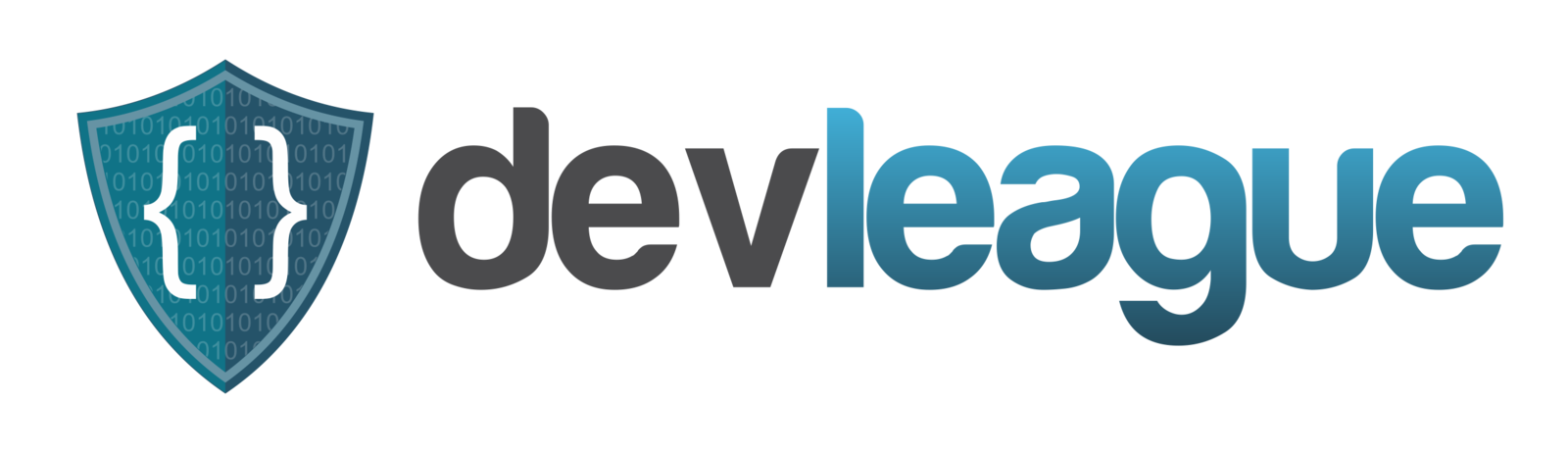
What is Ajax?
The term Ajax was coined as an acronym for Asynchronous JavaScript and XML
The XMLHttpRequest interface was created by Microsoft in the early 2000's and by ~2005 the major browsers had implemented the functionality.
Game changing technology for the way we are accustomed to using the internet today.
Sooo....what is Ajax?
The XMLHttpRequest interface essentially gives us a "backdoor" for making HTTP requests from a web server.
Around the time of inception, XML Web Services were the most common form of M2M communication on the internet.
However, since it is JUST an HTTP request any MIME type supported by a web server can be requested
Today, JSON(JavaScript Object Notation) is the primary standard for transferring data via REST API's.
Sounds cool. How do I use it?
There are 2 primary ways that AJAX is currently used today on the web:
- Native browser XmlHttpRequest API
- jQuery $.ajax() method
Because of cross browser differences and the handling of multiple states many people often default to the ease of the jQuery $.ajax() methods.
This really dates back to Microsoft deciding to use a different naming and instantiation method in IE6 and earlier.
XMLHttpRequest
All modern browsers support the XMLHttpRequest interface consistently.
var httpRequest;
if (window.XMLHttpRequest) { // Mozilla, Safari, IE7+ ...
httpRequest = new XMLHttpRequest();
} else if (window.ActiveXObject) { // IE 6 and older
httpRequest = new ActiveXObject("Microsoft.XMLHTTP");
}
//All browsers
httpRequest.onreadystatechange = function(){
// process the server response
};
Cross-browser method of creating an XMLHttpRequest object.
After object instantiation of the object, all methods of the object are consistent.
Making the Request
var httpRequest;
if (window.XMLHttpRequest) { // Mozilla, Safari, IE7+ ...
httpRequest = new XMLHttpRequest();
} else if (window.ActiveXObject) { // IE 6 and older
httpRequest = new ActiveXObject("Microsoft.XMLHTTP");
}
httpRequest.open('GET', 'http://www.reddit.com/r/webdev.json', true);
httpRequest.send(null);
The open() method takes 3 arguments:
- method - The HTTP request method(GET, POST, PUT, DELETE)
- url - The URL to send the request to
- async - Default true. Perform the send() method asynchronously.
Handling the Response
httpRequest.onreadystatechange = function(){
if (httpRequest.readyState === 4) {
if (httpRequest.status === 200) {
updateUI(httpRequest.responseText);
} else {
throw new Error('There was a problem with the request.');
}
}
}
Since the HTTP request goes through multiple states we must check for proper state of a successful completed request.
Our handler will be called multiple times on every state change.
Handling the Response
httpRequest.onreadystatechange = function(){
if (httpRequest.readyState === 4) {
if (httpRequest.status === 200) {
updateUI(httpRequest.responseText);
} else {
throw new Error('There was a problem with the request.');
}
}
}
Possible States:
0: Unsent - The open() method has not been called.
1: Opened - The send() method has been called. Connection is open.
2: Headers_Received - send() has been called and headers are available.
3: Loading - Downloading response. Response not completed.
4: Done - The response is complete and data is available.
Handling the Response
httpRequest.onreadystatechange = function(){
if (httpRequest.readyState === 4) {
if (httpRequest.status === 200) {
updateUI(httpRequest.responseText);
} else {
throw new Error('There was a problem with the request.');
}
}
}
Status:
All HTTP requests receive an HTTP status response code signifying if the request was successful or not.
A status of 200 means the request was successful and the response is accessible via the responseText property.
With the proper combination of readyState and status we can process the response.
jQuery $.ajax()
jQuery gives us a nice abstraction layer and interface for sending/receiving cross-browser XMLHttpRequest(s)
jQuery gives us a nice abstraction layer for sending/receiving XMLHttpRequest(s)
$.ajax({
method: 'GET',
url: 'http://reddit.com/r/webdev.json'),
dataType: 'json'
})
.done(function(data) {
//handle successful response
processResponse(data);
})
.fail(function() {
//Handle errors
handleError();
})
.always(function() {
//Always update the UI with status
});
REMEMBER: It's just an XMLHttpRequest
jQuery $.ajax()
NOTE: Always check what version of jQuery you are using and consult the documentation for supported methods and syntax.
The jQuery API has gone through multiple revisions and many examples will be different depending on the version of jQuery you are using.
More jQuery $.ajax()
jQuery also supports other more direct methods for specific types of requests. Again they all use the same underlying XMLHttpRequest interface but just a more concise way to make your requests.
Resources
Ajax Schmajax
By Jason Sewell
Ajax Schmajax
- 2,177