Recursion(Recursion(Recursion()))


Recursion is often described as...
or...

or even...
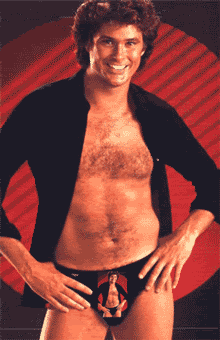
WTF?!
Recursion is simply:
Allowing a function to call itself from within it's function scope.
var recursify = function(num){
//base case
if(num === 0){
return;
}
console.log(num);
recursify(--num);
};
recursify(10); // 10 9 8 7 6 5 4 3 2 1
That's it.
When to use it:
We use recursion when we have an input structure to our function that can be of infinite dimensions.
Some mathematical things that I don't know about.
Could you imagine having to traverse all the elements in an HTML document with for loops?!
When our input to our function is going to be similar in type but differening values repeatedly.
What to remember:
- ALWAYS define your base case first
- Make sure you're input is changing every time you recall your function
- Forgetting either of these scenarios will help you write the infinite loop algorithm.
- Once a function returns, the call stack will unwind and finally exit from it's initial call.
Base Case: The point at which your input value stops the function from calling itself in order to "unwind" it's returns
var recursify = function(num){
//base case
if(num === 0){
return;
}
console.log(num);
recursify(--num);
console.log(num); // <- new console.log AFTER we call ourself
};
recursify(10);
// 10 9 8 7 6 5 4 3 2 1 0 1 2 3 4 5 6 7 8 9
Let's add another line to visualize this:
The second console.log never get's called until a return statement is called at our base case.
Every function will get "returned to" and finish executing lines of code after the function was called and naturally return on their own.
Recursion
By Jason Sewell
Recursion
- 2,519