Docker: The basics
by Joe Meilinger
What/why docker?
- Lightweight "Virtual Machine" runner
- Enforces much of 12-factor application design
- Provides a simpler mechanism to move an application from development to other environments
- Reduces ops burden when utilizing a container orchestrator (eg. Kubernetes, Kubernetes derivates, docker swarm, etc) versus managing application servers
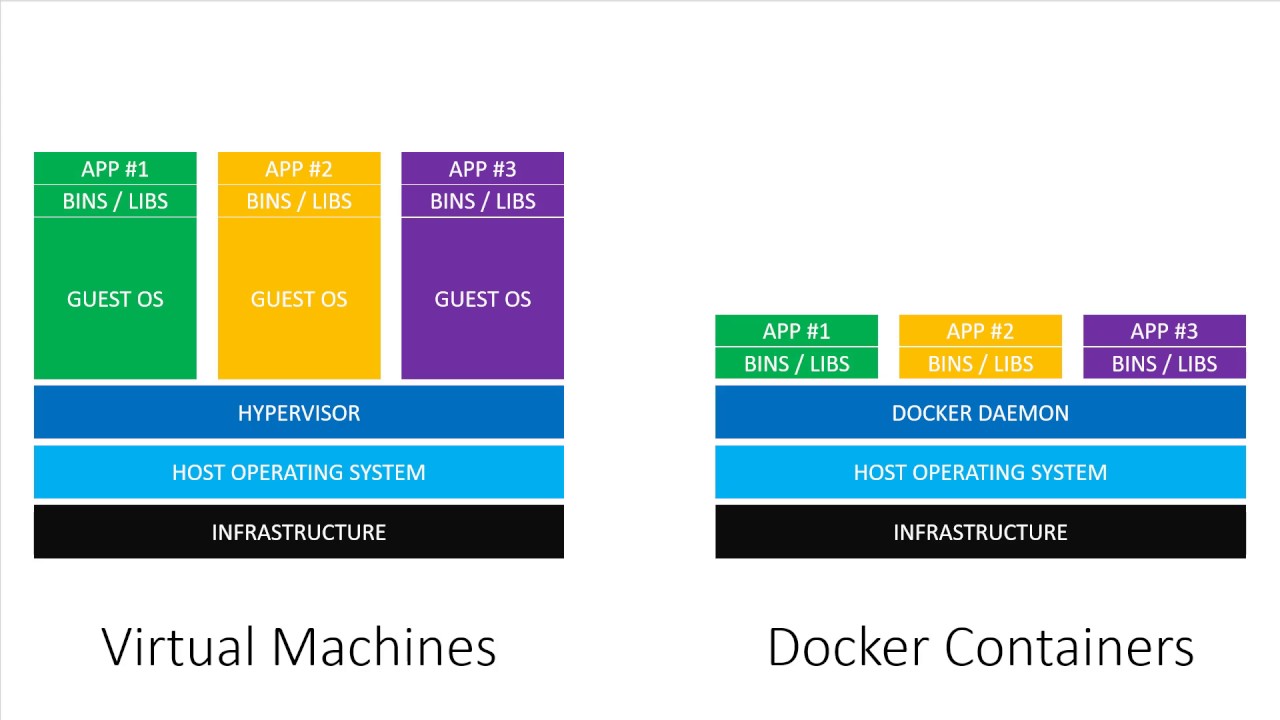
Text
Virtual Machines vs Docker Containers
Images vs containers
- Images are built by running commands contained in a Dockerfile
- Images are stored in a registry and contain a complete binary representation of the layers used to create the image
- Containers are running images
- In OOP: Image = Class
- In OOP: Container = Object
- Containers can have volumes mapped, run in a network, have environment variables injected, etc
- Containers are meant to be lightweight and disposable
- Runs a single process (which can spawn others as needed)
Getting setup
- Install Docker for Windows/Docker for Mac
- From bash/git-bash, verify docker is running properly via:
> docker ps # list running containers
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS
> docker images # list images in local registry
REPOSITORY TAG IMAGE ID CREATED SIZE
Basic Docker cli commands
- docker ps -- displays list of running containers
- docker images -- displays a list of local registry images
- docker build -- build a docker image
- docker run -- run a container for an image
- docker exec -- run a process on a running container
- docker logs -- display STDOUT/STDERR of container
- docker system prune -- clean up your local system
Building an image
- Uses a Dockerfile to build
- By default, publishes image to a local registry
- Never build actual configuration into images, defaults are fine
- Same image should be able to be used across environments as configuration would be the only thing changing
> cat Dockerfile
FROM python:3.8
WORKDIR /app
COPY . /app
RUN pip install -r requirements.txt
ENTRYPOINT ["python", "app.py"]
> docker build . # builds docker image using auto-generated name and ID
> docker build . -t my-image # builds docker image, tagging w/ "latest"
> docker build . -t my-image:1.0 # builds docker image, tagging w/ "1.0"
Running a container from an image
- Pulls image from a registry (local or remote)
- Runs on a docker host (local machine or remote host)
> docker run [IMAGE] # runs image by repo:tag OR image id using default entrypoint
> docker run -it [IMAGE] /bin/bash # runs image using your specified entrypoint (/bin/bash)
> docker run -p 3000:5000 [IMAGE] # runs image, mapping port 5000 on the container 3000 on host
Image Registry
img1
img2
img3
container1
from img1
Docker runtime
Mapping volumes/attaching to containers/injecting environment variables etc
> docker run -v "$(pwd)":/app [IMAGE] # run image, mount current host folder to /app on container
> docker exec -it [CONTAINER_ID] /bin/bash # execute command on running container (/bin/bash)
> cat vars
VAR1=VAL1
VAR2=VAL2
> docker run --env-file vars [IMAGE] # runs image, using env variables from "vars" file
- Able to overlay a host filesystem location to a container's filesystem via bind mount
- Can run an additional process (ex. interactive shell) on a running container via "docker exec" command
Networking containers together
- Docker utilizes internal/bridged networks
- If you're running more than one container, probably easiest to create a docker-compose.yml to orchestrate a stack
API
UI
Docker runtime
FGDRT + C3PO example
- Run local C3PO + API via docker-compose
- Run FGDRT API + FGDRT UI
Docker runtime
C3PO DB
C3PO API
FGDRT API
FGDRT UI
localhost:3001
> docker-compose -d --build
> docker-compose --build
Resources
Step-by-step tutorial/example
Docker: The basics
By Joe Meilinger
Docker: The basics
- 196