Android SL4A
Before called ASE (Android Scripting Environment)

Started in 2009 by Damon Kohler
20% time project at Google.
Python, Perl, Ruby, Lua, BeanShell, Javascript, Tcl, Shell and more.
20% time project at Google.
Python, Perl, Ruby, Lua, BeanShell, Javascript, Tcl, Shell and more.
IS SL4A Useful?
SIMPLE EXAMPLE
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.os.Bundle;
import android.widget.EditText;
import android.widget.Toast;
public class SimpleName extends Activity {
private EditText m_text = null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("Hello!");
builder.setMessage("What is your name?");
m_text = new EditText(this);
builder.setView(mEditText);
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(SimpleName.this, "Hello World!", Toast.LENGTH_SHORT).show();
} });
...
import android.app.Activity; import android.app.AlertDialog; import android.content.DialogInterface; import android.os.Bundle; import android.widget.EditText; import android.widget.Toast; public class SimpleName extends Activity { private EditText m_text = null; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Hello!"); builder.setMessage("What is your name?"); m_text = new EditText(this); builder.setView(mEditText);
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(SimpleName.this, "Hello World!", Toast.LENGTH_SHORT).show();
} });
...
SIMPLE EXAMPLE IN PYTHON
import android
droid = android.Android()
name = droid.getInput("Hello!", "What is your name?")
droid.makeToast("Hello, %s" % name.result)
Python program: 4 SLOC
HOW does it work?
-
Android() object
-
Talks to a service called SL4A
- (Scripting Layer for Android)
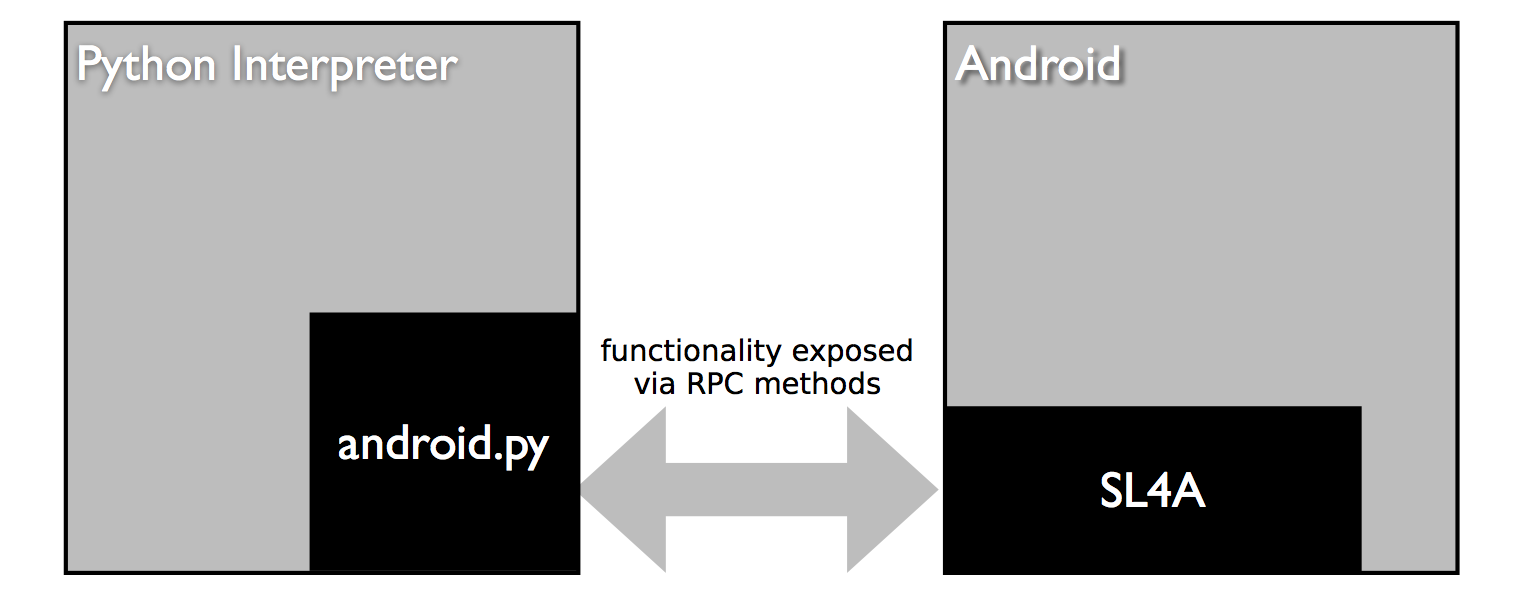
SL4a Architecture
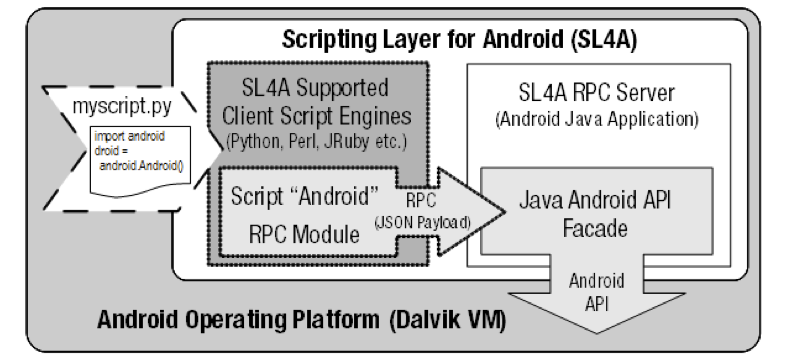
- Interoperation of scripting language engines, ported to the Android platform API via remote procedure calls (RPCs) to a server implemented as a standard Android Java application.
-
API facade exposing subset or Android API , accesible using JSON-RPC
-
RPC API compiled in dex bytecode, no additional interpreting in Java
JSON-based RPC
Any program that implements a compatible JSON–based RPC interfacing module or set of routines can potentially invoke the SL4A RPC Server.
https://github.com/damonkohler/sl4a/blob/master/python/ase/android.py
{ "Image": { "Width": 800, "Height": 600, "Title": "View from 15th Floor", "Thumbnail": { "Url": "http://www.example.com/image/481989943", "Height": 125, "Width": "100" }, "IDs": [116, 943, 234, 38793],
...
SL4a Functionality
-
ActivityResult
-
Android
-
ApplicationManager
• BatteryManager
-
Camera
-
CommonIntents
-
Contacts
-
Event
-
EyesFree
-
Location
-
MediaPlayer
- MediaRecorder
-
Phone
-
Preferences
- SensorManager
-
Settings
-
Sms
- SpeechRecognition
-
ToneGenerator
-
WakeLock
-
Wifi
-
UI
FACADES
-
A facade represents a collection of functionality
-
@Rpc annotation exposes methods
-
@RpcParameter, @RpcDefault, @RpcOptional describe method arguments.
UI using WEB View
Communicating through events
example.html
<script language= "javascript" type= "text/javascript">
var droid = new Android();
var date = new Date();
droid.eventPost("EVENT_A", "Page Loaded On " + date);
</script>
example.py
import android
droid = android.Android()
droid.webViewShow("/sdcard/sl4a/scripts/html/example.html")
result = droid.eventWaitFor("EVENT_A").result[“data”]
self.droid.log("Received data from EVENT_A: " + result)
Examples
Speech Recognition
import android
droid = android.Android()
message = droid.recognizeSpeech("Say something?")
droid.makeToast("You said: %s" % message.result)
text-to-speech
import android
droid = android.Android()
droid.vibrate()
droid.ttsSpeak("Hello, this is android speaking!")
Web view
import android
droid = android.Android()
message = droid.webViewShow("http://xkcd.com/353/")
SMS
import android
droid = android.Android()
droid.smsSend(someNumber,"SMS Sent!")
Dialogs
Python for android
https://code.google.com/p/python-for-android/
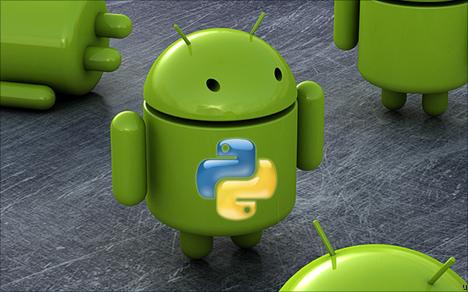
UI with FULLSCREENWRAPPER2
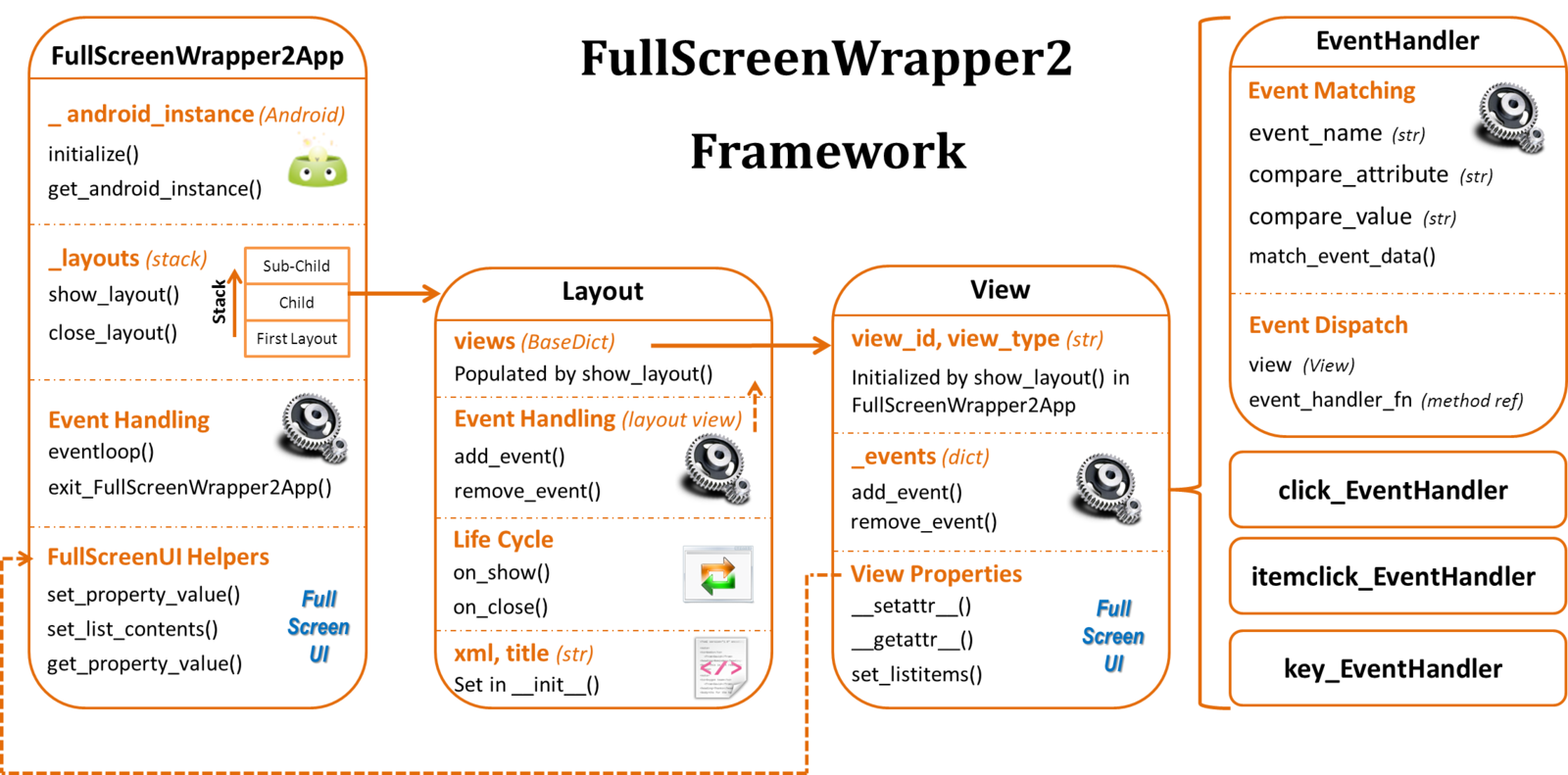
You can make Screens like this
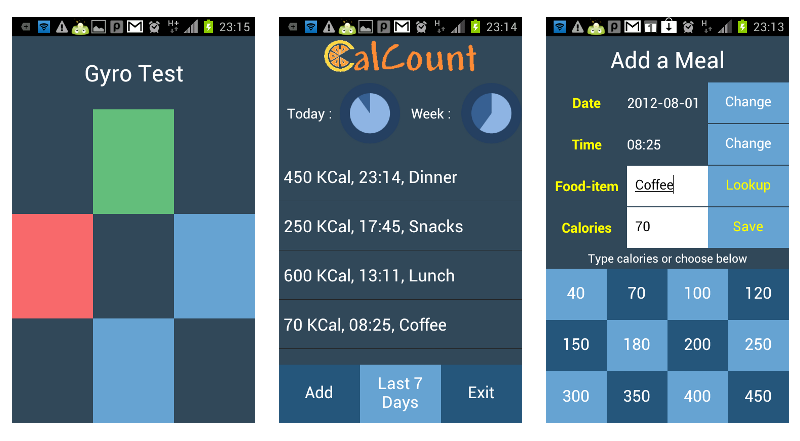
Demo code
import android, random
from fullscreenwrapper2 import *
class DemoLayout(Layout):
def __init__(self):
super(DemoLayout,self).__init__(xmldata,"FullScreenWrapper Demo")
def on_show(self):
self.add_event(key_EventHandler(handler_function=self.close_app))
self.views.but_change.add_event(click_EventHandler(self.views.but_change, self.change_color))
self.views.but_exit.add_event(click_EventHandler(self.views.but_exit, self.close_app))
self.views.txt_colorbox.background="#ffffffff"
def on_close(self):
pass
def close_app(self,view,event):
FullScreenWrapper2App.exit_FullScreenWrapper2App()
def change_color(self,view, event):
colorvalue = "#ff"+self.get_rand_hex_byte()+self.get_rand_hex_byte()+self.get_rand_hex_byte()
self.views.txt_colorbox.background=colorvalue
def get_rand_hex_byte(self):
j = random.randint(0,255)
hexrep = hex(j)[2:]
if(len(hexrep)==1):
hexrep = '0'+hexrep
return hexrep
if __name__ == '__main__':
droid = android.Android()
random.seed()
FullScreenWrapper2App.initialize(droid)
FullScreenWrapper2App.show_layout(DemoLayout())
FullScreenWrapper2App.eventloop()
PACKAGING IN APK
Python project template
that will embed a Python interpreter
and your Python scripts into an APK.
and
YOUR OWN PYTHON DISTRIBUTION
APK compiling:
- Python libraries
- All selected libraries (kivy, pygame, pil...)
- A java bootstrap
-
A build script
Cross-compiling python
for android
Where is SL4A USED?
Not much more, really, but some cool examples.
- SmallSat
- Cellbots – http://www.cellbots.com/
- Oplop – http://code.google.com/p/oplop/
- Broadcast – http://github.com/mleone/broadcast/
Android SL4A
By Jorge Vila Forcén
Android SL4A
- 3,536