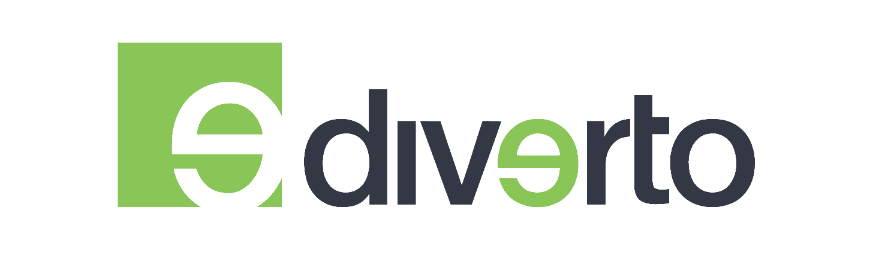
Are we safe yet?
So much security controls out there
Vlatko Kosturjak (@k0st), DORS/CLUC 2018, Zagreb, 20th of April, 2018
Agenda
- Introduction
- Past security protections
- New security protections
- Recommendations
- Summary
- Questions and answers
15 minutes
About me
- CTO at Diverto
- Open source (security) developer
- Authored own tools/projects
- Contributed to many existing projects
- https://github.com/kost
- DORS/CLUC and HULK
- was president of CLUG/HULK
- was part of organizing comittee of DORS/CLUC
Anything problematic?
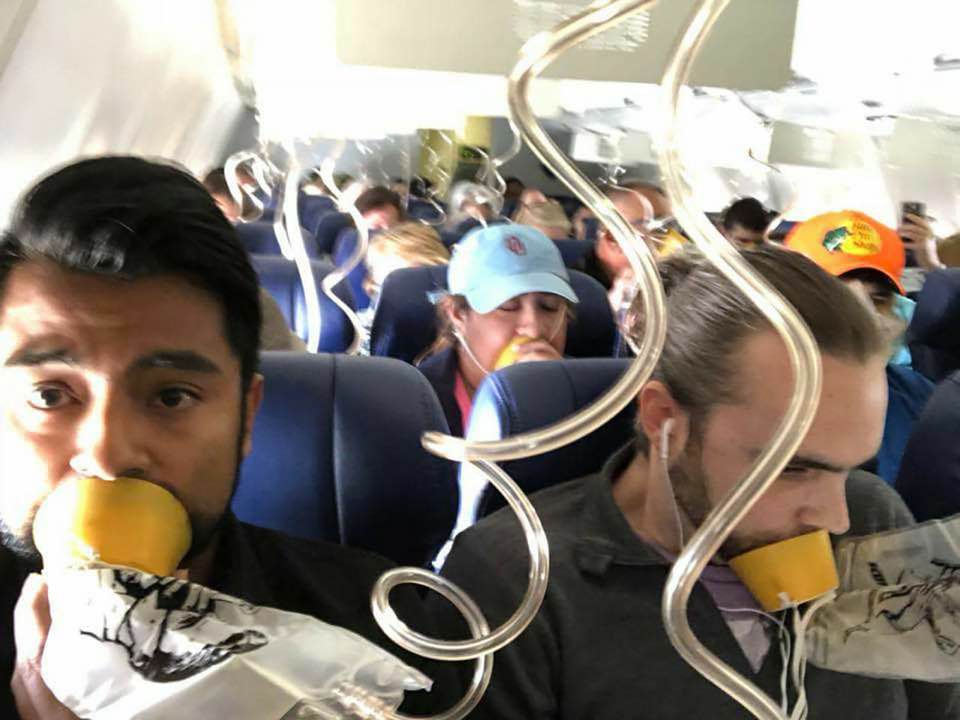
Introduction
#include <stdio.h>
int main (int argc, char *argv[]) {
char buffer[1024];
strcpy (buffer,argv[1]);
printf (buffer);
}
Security issues here
- not checking size of buffer
strcpy (buffer,argv[1]);
printf (buffer);
// TODO: Will add check later
Why problematic?
$ gdb -q --args ./ex2 AAAAAAAAAAAAAAAAAAAAAAAAAAAAAA
Reading symbols from ./ex2...(no debugging symbols found)...done.
(gdb) r
Starting program: /dc2018/ex2 AAAAAAAAAAAAAAAAAAAAAAAAAAAAAA
Program received signal SIGSEGV, Segmentation fault.
0x0000414141414141 in ?? ()
(gdb) i r
rax 0x1e 30
rdi 0x60202e 6299694
rbp 0x4141414141414141 0x4141414141414141
rsp 0x7fffffffdd70 0x7fffffffdd70
[...]
rip 0x414141414141 0x414141414141
gs 0x0 0
Security issues here
- Most architectures affected
- Intel, ARM, MIPS, ...
- Scripting language is not affected?
- How do you parse XML, talk TLS, etc?
- Bindings can be affected
- libxml, OpenSSL, yourCustomLib...
- Exploitation
- Remote Code Execution (RCE)
- Privilege Escalation
- Data Modification
- Information disclosure
Kernel protections
Feature | Controllable | Description |
---|---|---|
ASLR | randomize_va_space | Address space Randomization |
NX | noexec=on noexec=off |
Not executable memory pages |
Compiler flags
Flag | Description | Prevention |
---|---|---|
-z noexecstack | Marks stack as non executable | executing shellcode on stack |
-fstack-protector-all -fstack-protector-strong |
Adds stack canaries | prevents function return overwritting |
-D_FORTIFY_SOURCE=2 | Add extra check to dang f() | prevents buffer overfows |
Compiler flags 2
Flag | Description | Prevention |
---|---|---|
-Wl,-z,relro | linker marks sections read only | prevents GOT overwrite attacks |
-Wl,-z,now | all symbols are resolved at load time | prevents GOT overwrite attacks |
fPIE -pie -fPIC |
generate position independent code/executable | randomize address space (ASLR) |
Control flow integrity
- Security control
- Exploitation mitigation technique
- Preventing attacker to take control of flow
- Control flow Guard (CFG)
- Microsoft Implementation, 2014,Windows 8.1 update 3
- Microsoft 10 Creator update, kernel compiled with CFG
- Have its vulns as well
- Overwritting CFI check itself, for example
grsec lovers?
- Reuse Attack Protector (RAP)
- Hardened OS Linux Kernel, 2017
- Control flow mitigation implementation
- 5.4% performance hit
- Reference
Clang CFI
- Control flow integrity
- Available in clang 3.9+
-fsanitize=cfi
GCC?
- GCC 8.x
- Initial support for CFI
- Available in gcc 8.1
-fcf-protection=full -mcet
- You will need Intel processor which supports CFI
Intel?
- Control-flow Enforcement(CFE)
- Technology Preview, June 2017, rev 2.0
- Hardware Assisted Control-flow technology
-mcet
Few things BTW
- GCC 8.1
- Intel landed patches
- Not released yet
- Intel CFE
- Did not find any processor which supports CFE
- AFAIK Intel did not release any CPU with CFE
- *Commercially available
- TL;DR
- No production releases
- Limited to Intel x86_64(AMD? ARM? MIPS?)
So, no more buffer overflows?
typedef struct {
char name[16];
char password[18];
char privilege;
char description[20];
} person;
person tmp;
strcpy (tmp.name,username);
Only harder to exploit
- It prevents most dangerous exploitation
- Problem is still there
- Only harder to exploit
So, how I should compile?
- Something like this:
clang-3.9 ex7.c -Wl,-z,now -Wl,-z,relro -fpie -pie -fstack-protector-strong -D_FORTIFY_SOURCE=2 -O2 -fvisibility=hidden -flto -fsanitize=cfi -o ex7
clang-3.9 ex7.c -Wl,-z,now -Wl,-z,relro -fpie -pie -fstack-protector-all -D_FORTIFY_SOURCE=2 -O2 -fvisibility=hidden -flto -fsanitize=cfi -o ex7
How I can detect protections on binaries?
- checksec
- No support for
- CFI
- nm [binary] | grep [cfi symbol]
$ nm ex7-prot | grep handle_cfi_check_fail
0000000000104a40 T __ubsan_handle_cfi_check_fail
0000000000104a90 T __ubsan_handle_cfi_check_fail_abort
$ nm ex7 | grep handle_cfi_check_fail
exit 1
Fixing at the source
#include <stdio.h>
int main (int argc, char *argv[]) {
char buffer[10];
strncpy (buffer,argv[1], 10);
printf ("%s",buffer);
}
Asan?
- Address Sanitizer detects
- use-after-free
- buffer overflows
- memory leaks
-fsanitize=address
- x2 performance penalty
- not production? testing/fuzzing
Summary
- Take advantage of x86_64
- pass by register
- NX
- better ASLR
- Make sure it is x86_64
- distro/kernel/libs/bins
- executable itself
- Use clang
- state of the art protections
- use the security features/flags
- gcc is lagging behind
Are we safe yet?
By k0st
Are we safe yet?
Presented at DORS/CLUC 2018, Zagreb, 20th of April, 2018
- 1,250