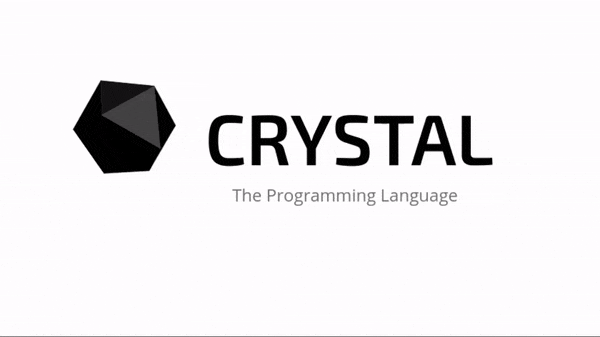
A language for humans and computers.
nil
x = nil
x = nil
x = "Hello BorderlessEngConf!"
x = nil
# Here 'x' is a Nil
x = "Hello BorderlessEngConf!"
# 'x' is now a String
if rand > 0.5
x = nil
else
x = "Hello BorderlessEngConf!"
end
x
# ?
if rand > 0.5
x = nil
else
x = "Hello BorderlessEngConf!"
end
x
# Here 'x' is a `String?`. This is
# shorthand for `String | Nil`.
"foo".upcase # => "FOO"
"foo".does_no_exist
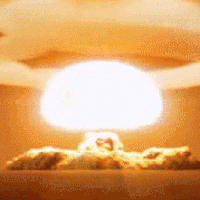
(Saturday, 2am)
"foo".does_not_exist
error in line 1
Error: undefined method 'does_not_exist' for String
if rand > 0.5
x = "foo"
else
x = 42
end
x.upcase
if rand > 0.5
x = "foo"
else
x = 42
end
x.upcase
Error: undefined method 'upcase' for Int32 (compile-time type is (Int32 | String))
if rand > 0.5
x = "foo"
# All good!
x.upcase
else
x = 42
# Also fine
x * 1729
end
class Foo
end
class Foo < Bar
end
class Foo
include Bar
end
struct Foo
end
class Foo
# Accessible to the local instance only
@instance_var : String
# Shared between all `Foo`s
@@class_var : Bool
end
class Foo
def hello
#...
end
end
class Foo
def hello : String
return "Hello world!"
end
end
class Foo
def hello(name : String) : String
return "Hello #{name}!"
end
end
class Foo
def hello(name : String = "world") : String
return "Hello #{name}!"
end
end
class Foo
def hello(name : String = "world", shout : Bool = false) : String
if shout
name = name.upcase
end
return "Hello #{name}!"
end
end
class Foo
def hello(name : String = "world", shout : Bool = false)
if shout
name = name.upcase
end
return "Hello #{name}!"
end
end
class Foo
def hello(name : String = "world", shout : Bool = false)
if shout
name = name.upcase
end
"Hello #{name}!"
end
end
class Foo
def hello(name : String = "world", shout : Bool = false)
name = name.upcase if shout
"Hello #{name}!"
end
end
class Foo
def hello(name = "world", shout = false)
name = name.upcase if shout
"Hello #{name}!"
end
end
class Foo
def hello(name, shout = false)
name = name.upcase if shout
hello name
end
def hello(name = "world")
"Hello #{name}!"
end
end
def twice
yield
yield
end
twice do
puts "Hello!"
end
3.times do |i|
puts i
end
3.times do |i|
puts i
end
struct Int
def times
i = 0
while i < self
yield i
i += 1
end
end
end
3.times do |i|
puts i
end
struct Int
def times
i = 0
while i < self
yield i
i += 1
end
end
end
i = 0
while i < 3
puts i
i += 1
end
3.times do |i|
puts i
end
i = 0
while i < 3
puts i
i += 1
end
Crystal’s job is to generate LLVM code
—Ary
- Macros for meta-programming
- Human friendly concurrency via CSP
- An extensive, modern standard library
- Doc generation
- Test framework
- Common code formatting
- Dependency management
All in the compiler and standard tools
A welcoming, and friendly community.
WTF is this thing?
Why?
WTF is this thing?
Why Crystal?
crystal-lang.org
PlaceOS.com
Copy of Crystal: a language for humans and computers
By Kim Burgess
Copy of Crystal: a language for humans and computers
A brief tour of one of the most enjoyable and performant ways you can express ideas today.
- 79