Redux powered Android Dev
Me
Mobile Enthusiast (Android / iOS)
Love Kotlin / Swift + Opensource projects
Join mercari (merpay) in 2018 :)

github.com/kittinunf

@kittinunf
Yet another architecture?

Programming is abstraction
data class Car(
val make: String,
val wheel: Int,
val color: Color
)
val car1 = Car("Honda", 4, Color.WHITE)
val car2 = Car("Toyota", 8, Color.RED)
State?
class View {
// 3 VISIBLE, INVISIBLE, GONE
var visibility: Int,
// 2 focused, not
var focused: Boolean,
// 2 activated, not
var activated: Boolean,
// 2 enabled, not
var enabled: Boolean
}
// possibility 3 * 2 * 2 * 2
MV(P)(VM)
view.chatListSignals
.map { itemCount -> itemCount == 0 }
.subscribe { emptyList ->
view.setChatListViewVisibility(if (emptyList) GONE else VISIBLE)
view.showEmptyState(emptyList)
}.addTo(disposables)
paginator.results.subscribe { response ->
showConversationResults(response)
}.addTo(disposables)
paginator.errors
.subscribe(view::showError)
.addTo(disposables)
view.onLoadMoreSignals.subscribe {
paginator.loadNext()
}.addTo(disposables)
view.onDeleteSignal
.flatMap { chatMembership -> repository.leaveChat(chatMembership.chat.id) }
.filter { response -> !response.isSuccessful }
.subscribe(view::showError)
.addTo(disposables)
view.onLeaveSignal
.flatMap { chatMembership -> repository.leaveChat(chatMembership.chat.id) }
.filter { response -> !response.isSuccessful }
.subscribe(view::showError)
.addTo(disposables)
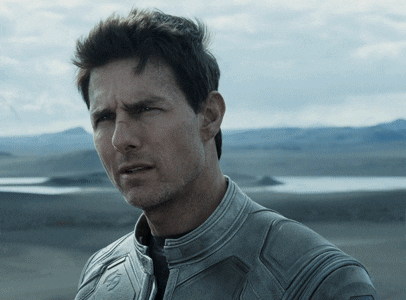
State is easy
But maintaining state is quite hard
Then, why not use abstraction that is serious about maintaining the state?
Idea of Redux
3 Principles of Redux
Redux attempts to make the state mutation predictable
Single source of truth
State is read only
Changes made with pure function
Idea of Redux




Action
State
Store
Reducer
Action
Payload of information from User / Screen to Store
sealed class Action
class Load : Action
class ShowResult(list: List<Data>) : Action
class ShowError(error: String) : Action
State
A single source of truth to represent the screen
data class State(
// represent list of data on the screen
data: List<Data>,
loading: Boolean,
error: String?
)
// or you can do even better with sealed class
sealed class State
object Loading : State
class Success(list: List<Data>)
class Failure(reason: String)
Reducer
Pure function where we change our State
val reducer = object : Reducer<State> {
override fun reduce(currentState: State, action: Action):
State {
return when (action) {
Loading -> currentState.copy(loading = true)
is ShowResult -> currentState.copy(
list = action.list,
loading = false
)
is ShowError -> currentState.copy(
error = action.reason,
loading = false
)
}
}
}
Store
Store is where the things get connecting, glues everything together
Store
Reducer
View (consumer)
Update your view in predictable way
store.states.subscribe { state ->
// state is a snapshot of thing on the screen
// at any point in time
if (loading == true) {
recyclerView.setVisibility = View.GONE
loadingView.setVisibility = View.VISIBLE
} else {
recyclerView.setVisibility = View.VISIBLE
loadingView.setVisibility = View.GONE
adapter.update(state.list)
adapter.notifyDataSetChanged()
}
}
Fits everything together
Store
Reducer
State (new)
State

Action
Wait you don't tell me what the Library you use?
To be honest, you could just write your own
I wrote my own for today's demo
DEMO

Devtools?
What if I am telling you that we can do that™ with AS
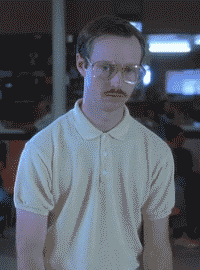
Demo again?

Q & A
Redux Powered Android Dev
By Kittinun Vantasin
Redux Powered Android Dev
- 1,724