EthereumQL
@krzkaczor
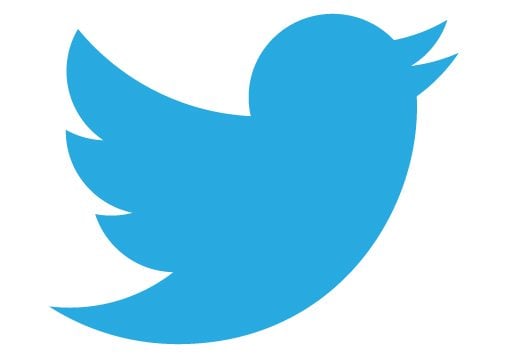

Use GraphQL to interact with Ethereum smart contracts
Ethereum 101


Blockchain
- distributed
- immutable
- slow to modify
- eventual consistent
- every node stores all data

CAP Theorem


How slow?
- new block each ~15 seconds
- 12 confirmations to be considered "sealed"
= 3 minutes

384,400,000 ÷ 299,792,458 = 1.28222038194 s.
1.28222038194 * 3 = 3.84s


- (almost) turing complete programs,
- running on EVM (solving halting problem, determinism),
- fast read operations
- slow state modification operations
- transparent
Ethereum Smartcontracts:
contract DumbContract {
uint public counter;
function DumbContract() public {
counter = 0;
}
function counterWithOffset(uint offset) public constant returns (uint sum) {
return counter + offset;
}
// a wild state transition appears!
function countup(uint offset) public {
counter += offset;
}
}
contract DumbToken {
mapping(address => uint256) balances;
function balanceOf(address tokenOwner) public constant returns (uint balance) {
return balances[tokenOwner];
}
function transfer(address to, uint tokens) public returns (bool success) {
balances[msg.sender] = balances[msg.sender].sub(tokens);
balances[to] = balances[to].add(tokens);
return true;
}
}

How do developers communicate with Ethereum blockchain?

Network of nodes
JSON-RPC API
{
"jsonrpc": "2.0",
"id": 3,
"method": "eth_call",
"params": [
{ "to": "0xf432cec23b2a0d6062b969467f65669de81f4653", "data": "0x87055008" },
"latest"
]
}
- no request batching
- can be time-consuming to execute
- hard to cache
- access to one value at the time

Web3.js - JSON-RPC client for browsers
import web3 from 'web3';
const myContract = web3.eth.contract(abi).at("0xf432cec23b2a0d6062b969467f65669de81f4653");
myContractInstance.myMethod((e, r) => console.log(r));
[
{
"constant": true,
"inputs": [],
"name": "SOME_BOOLEAN_VALUE",
"outputs": [{ "name": "", "type": "bool" }],
"payable": false,
"stateMutability": "view",
"type": "function"
},
{
"constant": true,
"inputs": [],
"name": "SOME_NUMERIC_VALUE",
"outputs": [{ "name": "", "type": "uint256" }],
"payable": false,
"stateMutability": "view",
"type": "function"
}
]
Common problems while interacting with smart contracts
- data over-fetching
- lack of type safety
- super slow


Meet EthereumQL

https://github.com/krzkaczor/EthereumQL

export const Wallet = graphql(gql`
query {
universe {
neumark {
balanceOf(address: "0x...") {
balance
}
}
euroToken {
balanceOf(address: "0x...") {
balance
}
}
}
}
`)(WalletComponent);
Client-side usage with React and Apollo
import { generateExecutableSchema } from "ethereumql";
const schema = generateExecutableSchema("DumbContract", contractAbi, web3);
Server-side usage
API files
- companion to ABI files
- provides missing type information
- caching policy
- derived from solidity code by comments

Client-centric architecture for dApps
What about mutations?
stick to web3 or even better, use

https://github.com/Neufund/TypeChain
THANKS!

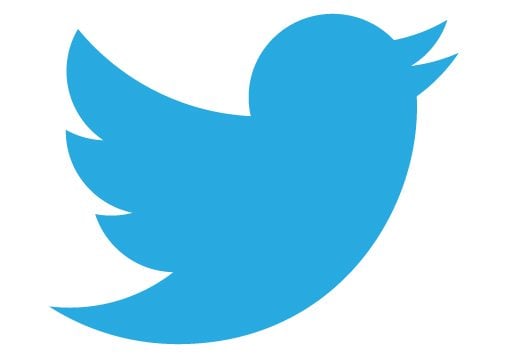
@krzkaczor

https://github.com/krzkaczor/EthereumQL
EthereumQL
By krzkaczor
EthereumQL
- 842