Creating Type Safe DApps with Typescript and TypeChain
@krzkaczor
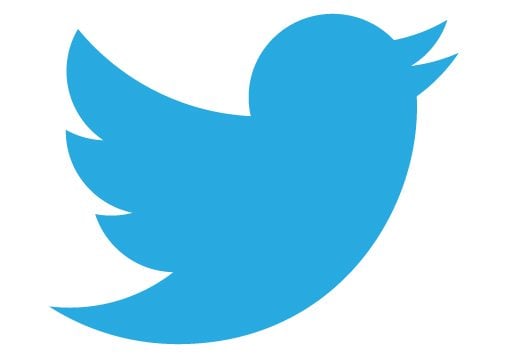
@ethereum_ts
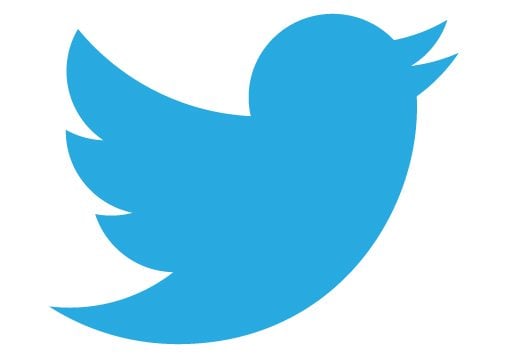
"Javascript is pretty good language"
~ some JS devs
class Cat extends Animal {
meow = async () => {
console.log("returned promise of moewing");
}
}
function main() {
const cat = new Cat();
cat.meow();
}
Modern Javascript
"Typescript is even a better language"
~ me
class Cat extends Animal {
meow = async () => {
console.log("returned promise of moewing");
}
}
function main() {
const cat = new Cat();
cat.meow(); // floating promise detected!
}
Typescript = Javascript + types
function sum(a, b) {
return a + b;
}
const four = sum(2,2);
function sum(a: number, b: number) {
return a + b;
}
const four = sum(2,2);
vs
TS's type system:
- structural typing
- advanced type level features (conditional types, mapped types)
- entirely stripped out from the output
- allows advanced refactoring
const MyContract = web3.eth.contract(abiArray);
// instantiate by address
const contractInstance = MyContract.at(address);
// call
const result = await myContractInstance.myConstantMethod('myParam');
// tx
const resultTx = await myContractInstance.myStateChangingMethod('someParam1', 23,
{value: 200, gas: 2000});
Interacting with smart contracts using JS and Web3js
TypeChain

Typescript bindings for Ethereum smart contracts
ABI
=>
TS wrapper
< demo >
Features
- static typing
- perfect IDE support for dapp development
- multiple targets
- web3.js 0.20/1.0/2.0
- ethers
- truffle
- custom targets
Why would you want to use it?
- better DX
- saves time
- helps during migrations
Future
- bypass ABIs limitation
ethereum-ts/TypeChain

THANKS!
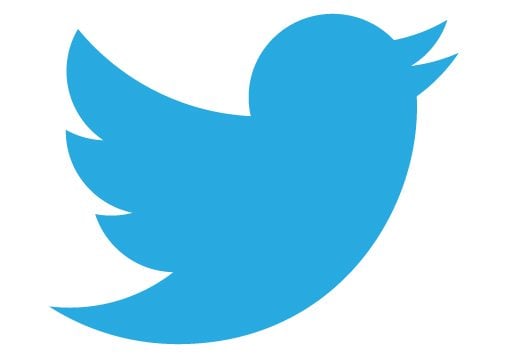
@krzkaczor
https://github.com/ethereum-ts/TypeChain
Creating Type Safe DApps with Typescript and TypeChain
By krzkaczor
Creating Type Safe DApps with Typescript and TypeChain
- 513