Things you May not know about javascript
GitHub: @loveky
Agenda
- Primitive types vs Objects
- Variable hoisting
- Constructor function
- Apply & Call in Function.prototype
- Closure
- Tips
Primitive types vs Objects
- number
- string
- boolean
- null
- undefined
-
object
-
array
-
regexp
-
function
PRIMITIVE TYPES VS OBJECTS(cont.)
js> typeof 1
"number"
js> typeof "1"
"string"
js> typeof true
"boolean"
js> typeof null
"object"
js> typeof undefined
"undefined"
js> typeof {}
"object"
js> typeof []
"object"
js> typeof /a/
"object"
js> typeof function() {}
"function"
Wrapper object for primitive type
js> "string".toUpperCase()
"STRING"
js> 1.1234.toFixed(2)
"1.12"
comparison
js> 1 === 1
true
js> "string" === "string"
true
js> new String("abc") === new String("abc")
false
js> new Number(1) === new Number(1)
false
Array is object
js> a = ["a", "b", "c"]
["a", "b", "c"]
js> a[0]
"a"
js> a["0"]
"a"
js> a["0"] = 100
100
js> a
[100, "b", "c"]
variable hoisting
var a = 1;
function test() {
console.log(a);
var a = 100;
console.log(a);
}
test()
var a = 1; function test() {
var a;
console.log(a); a = 100; console.log(a); } test()
VARIABLE HOISTING(cont.)
var a = function() {console.log(1)}; function test() { a(); var a = function() {console.log(100)}; a(); } test()
var a = function() {console.log(1)};
function test() {
a();
function a() {console.log(100)}
a();
}
test()
Constructor function
function Foo() {
this.welcome = "What's up, dude"
}
Foo.prototype.hi = function() {
console.log(this.welcome);
};
a = new Foo();
constructor function(cont.)
function Foo() {
this.welcome = "What's up, dude";
return "abc";
}
a = new Foo();
function Foo() {
this.welcome = "What's up, dude";
return {a:1};
}
a = new Foo();
Apply & Call in function.prototype
function total_price() {
return this.count * this.price;
}
total_price.call({count:4, price: 5})
APPLY & CALL IN FUNCTION.PROTOTYPE(cont.)
[].slice.call(arguments)
Array.prototype.slice.call(arguments)
js> var numbers = [5, 6, 2, 3, 7];
js> Math.max.apply(null, numbers);
7
APPLY & CALL IN FUNCTION.PROTOTYPE(cont.)
Apply in real world
jQuery.proxy()
Takes a function and returns a new one that will always have a particular context.
proxy = function() {
return fn.apply( context || this, args.concat( slice.call( arguments ) ) );
};
https://github.com/jquery/jquery/blob/2.1.1/src/core.js#L442-L467
APPLY & CALL IN FUNCTION.PROTOTYPE(cont.)
Call in real world
angular.isRegExp
toString = Object.prototype.toString;
function isRegExp(value) {
return toString.call(value) === '[object RegExp]';
}
https://github.com/angular/angular.js/blob/v1.2.20/src/Angular.js#L549-L551
closure
What is closure?
JavaScript allows inner functions
Inner functions are allowed to access all of the local variables, parameters and declared functions within their outer function
A closure is formed when one of those inner functions is made accessible outside of the function in which it was contained
closure(cont.)
Closure in Action
function makeFunc() { var name = "Mozilla"; function displayName() { alert(name); } return displayName; } var myFunc = makeFunc(); myFunc();
closure(cont.)
When to use closure?
var arr = []
for(var i=0; i < count; i++) {
arr[i] = function(){
console.log(i)
};
}
> arr[0]()
5
> arr[1]()
5
closure(cont.)
When to use closure?
var arr = []
function buildCallback(data) {
return function() {
console.log(data)
}
}
for(var i=0; i < count; i++) {
arr[i] = buildCallback(i);
}
closure(cont.)
When to use closure?
setTimeout(hideMenu, 500);
Tips
Native JavaScript Environment
brew install spidermonkey
brew install v8
alias jsc="/System/Library/Frameworks/JavaScriptCore.framework/Versions/Current/Resources/jsc"
ECMA5 compatibility
Use '===' instead of '=='
js> true == 1
true
js> 1 == "1"
true
js> [] == false
true
js> [0] == false
true
js> obj = {toString: function() {return "a"}}
({toString:(function () {return "a";})})
js> obj == "a"
true
js> obj = {valueOf: function() {return 1}}
({valueOf:(function () {return 1;})})
js> obj == 1
true
Useful methods in Array.prototype
Array.prototype.every
Array.prototype.some
Array.prototype.forEach
Array.prototype.map
Array.prototype.filter
Array.prototype.reduce
Array.prototype.reduceRight
Books
Object-Oriented JavaScript, 2nd Edition
Effective JavaScript : 编写高质量JavaScript代码的68个有效方法
JavaScript语言精粹
Secrets of the JavaScript Ninja
Thank you!
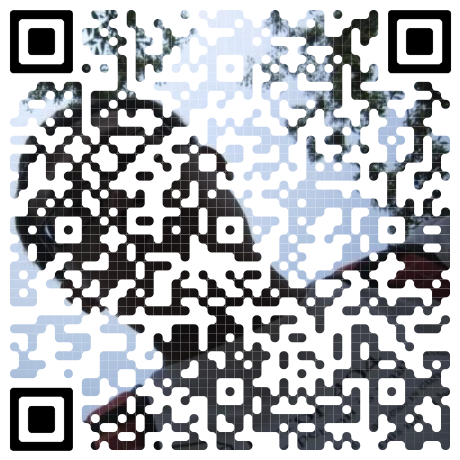
Things you May not know about javascript
By loveky
Things you May not know about javascript
- 1,599