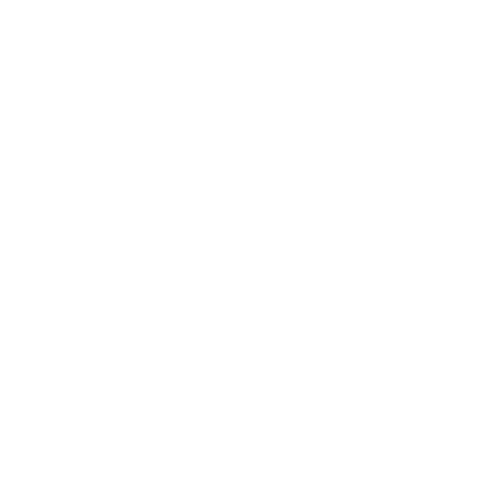
Http, Angular 2
in 10 minutes
Marcell Kiss


marcellkiss@budacode.com
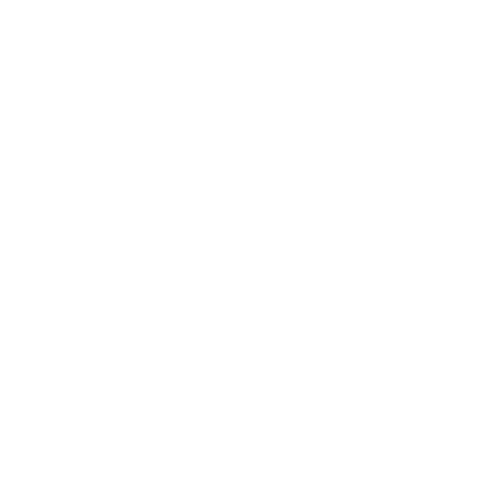


Developer
Co-Organizer
Example
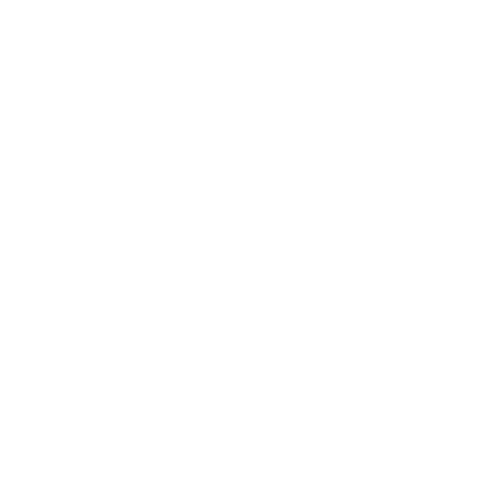
Best place to start:
import {Http, HTTP_PROVIDERS} from 'angular2/http';
@Component({
selector: 'http-app',
viewProviders: [HTTP_PROVIDERS],
templateUrl: 'people.html'
})
class PeopleComponent {
constructor(http: Http) {
http.get('people.json')
// Call map on the response observable
.map(res => res.json())
// Subscribe to the observable
.subscribe(people => this.people = people);
}
}
Text
Http API
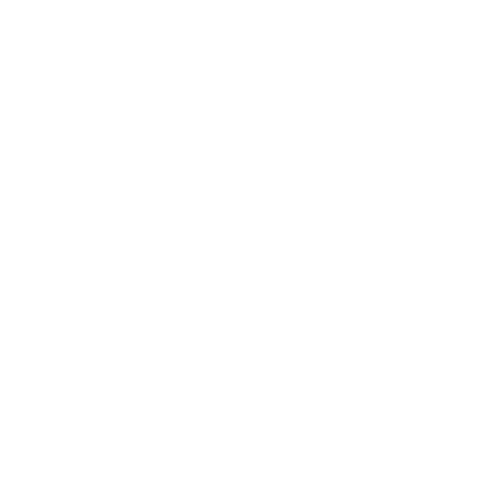
https://angular.io/docs/ts/latest/api/http/Http-class.html
constructor(
_backend: ConnectionBackend, _defaultOptions: RequestOptions
)
request(
url: string | Request,
options?: RequestOptionsArgs
) : Observable<Response>
get(
url: string,
options?: RequestOptionsArgs
) : Observable<Response>
Classes
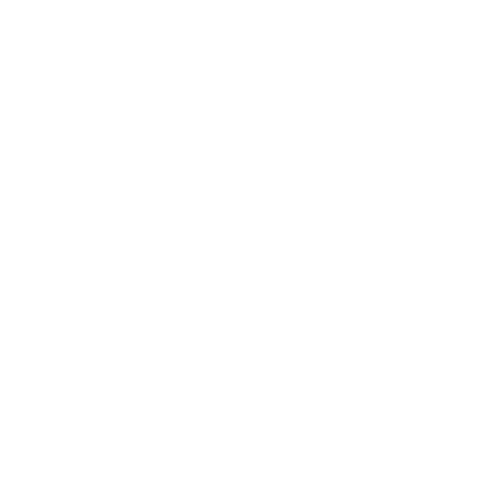
Change headers
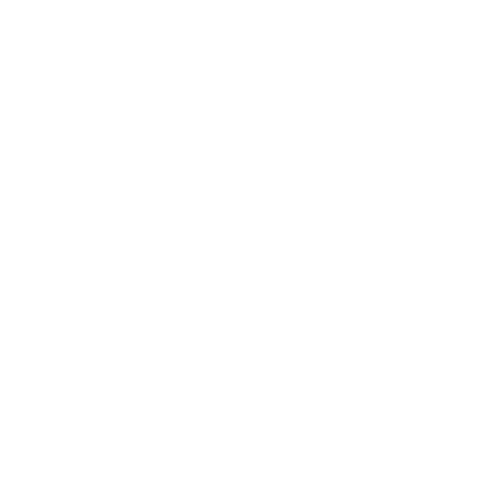
let headers = new Headers();
headers.append('Content-Type', 'application/json');
let authToken = localStorage.getItem('auth_token');
headers.append('Authorization', `Bearer ${authToken}`);
return this.http
.get('/profile', { headers: headers })
Observable benefits
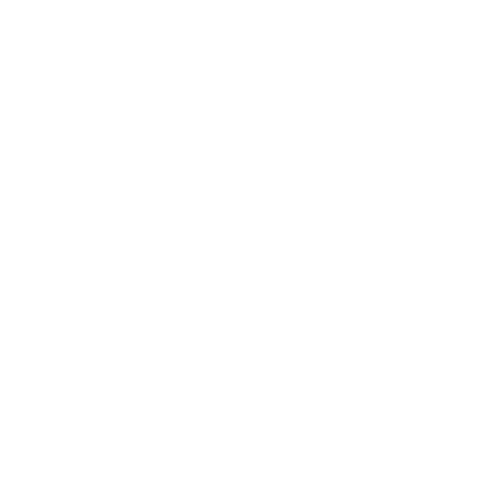
RxJS 5 is everywhere
keywords: observer, observable, operator
Easy to cancel (dispose)
Complex compositions
Data streams
Observable operators
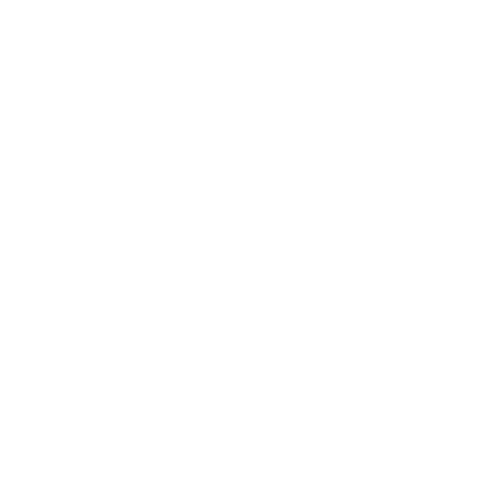
.map
// Using a function
var source = Rx.Observable.range(1, 3)
.map(function (x, idx, obs) {
return x * x;
});
var subscription = source.subscribe(
function (x) {
console.log('Next: ' + x);
},
function (err) {
console.log('Error: ' + err);
},
function () {
console.log('Completed');
});
// => Next: 1
// => Next: 4
// => Next: 9
// => Completed
Observables, more...
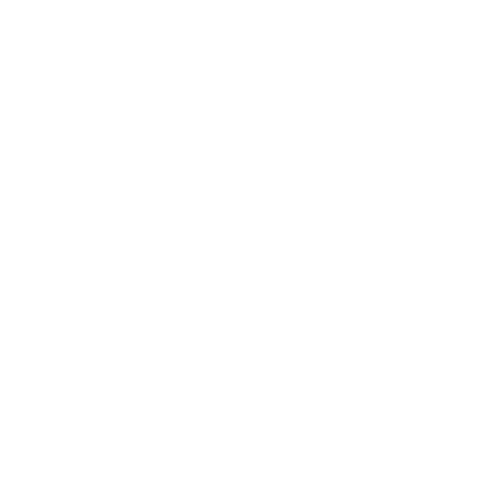
map
filter
delay
retry
forkJoin
flatMap
Good old modules
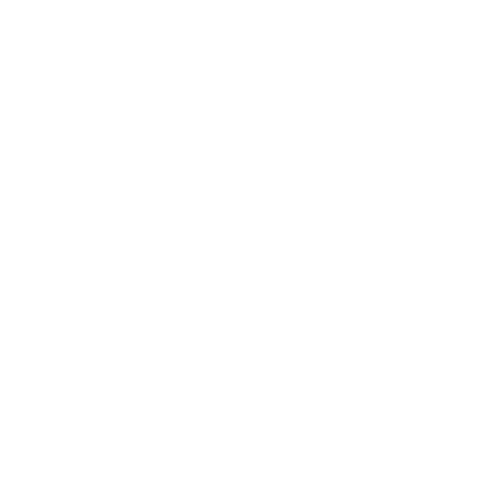
$resource -> no info
restangular 2 -> WIP
Conclusion
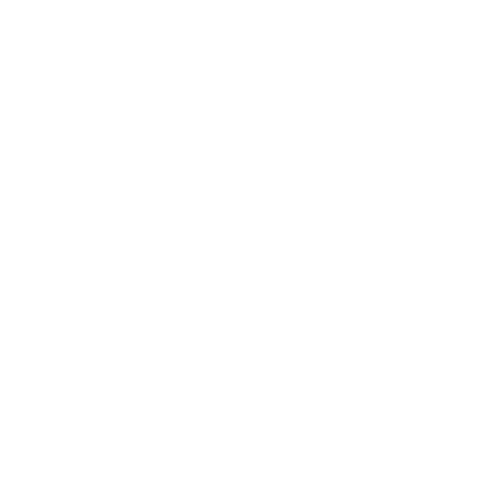
Observables
Documentation
Learning curve
Thanks
for your attention!
Q & A
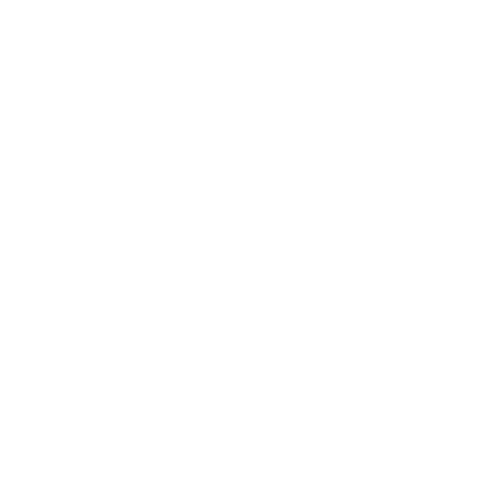
@martzellk
Sources
https://auth0.com/blog/2015/10/15/angular-2-series-part-3-using-http/
http://www.metaltoad.com/blog/angular-2-http-observables
http://blog.thoughtram.io/angular/2016/01/06/taking-advantage-of-observables-in-angular2.html
http://chariotsolutions.com/blog/post/angular2-observables-http-separating-services-components/
http://www.syntaxsuccess.com/viewarticle/angular-2.0-and-http
https://github.com/Reactive-Extensions/RxJS
https://github.com/Reactive-Extensions/RxJS/blob/master/doc/api/core/observable.md
https://egghead.io/lessons/rxjs-rxjs-observables-vs-promises
https://github.com/Reactive-Extensions/RxJS/blob/master/doc/gettingstarted/promises.md
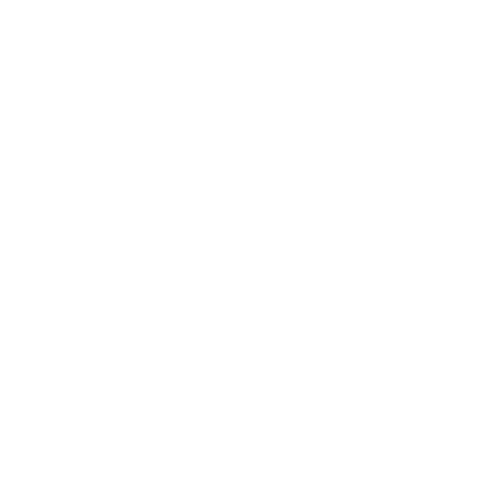
Http in Angular 2
By Marcell Kiss
Http in Angular 2
- 1,435