JavaScript One

Introduction to JavaScript
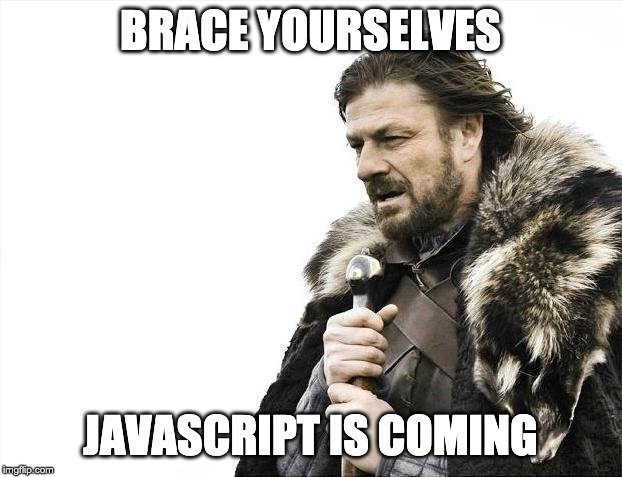
Student Learning Objectives
- Student can declare a variable
- Student can assign a variable a value
- Student can reassign a variable a value
- Student can recite the JavaScript Data Types
- Student can describe the difference between Null and Undefined
- Student can describe the difference between weak (dynamic) and strong (static) typing.
- Student can create an array literal
- Student can hard code values into an array literal
- Student can create an object literal
- Student can add multiple key/value pairs to object
- Student can declare if/elseif/else blocks
- Student can nest if statements
- Student can use logical operators
- Student can create a function expression
- Student can create a function declaration
- Student can invoke a function
- Student can return a value from a function
- Student can describe the difference between parameters and arguments
- Student can access variables from local function scope to global scope
- Student can declare a let or const variable
- Student can assign a let a value
- Student can use let or const to create block-scoped local variables
What is JavaScript?
JavaScript was initially created "to make web pages alive"
JavaScript is a programming language that contains logic.
JavaScript is often used alongside HTML and CSS, but can also be used for other purposes
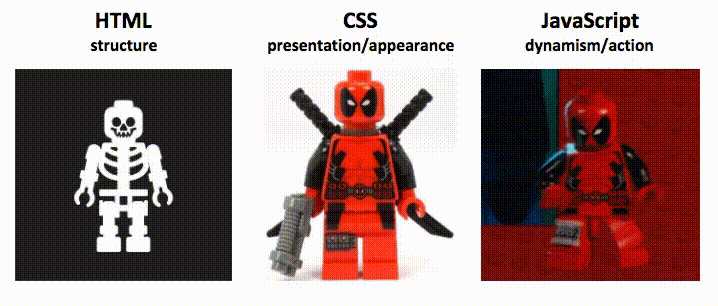
JavaScript provides the dynamism and the action of a website.
JavaScript Variables
Variables are used to store values in JavaScript. There are three ways to create, or declare, a variable:
var name = "Matias";
let name = "Matias";
const name = "Matias";
Declares a variable
The name of the variable
The value of the variable
JavaScript Data Types
JavaScript is built with several different data types.
Number
String
Boolean
Undefined
Null
Array
Object
let newString = "hello"
let newNumber = 7
let newBoolean = true
let newUndefined = undefined
let newNull = null
let newArray = []
let newObject = {}
Numbers
Numbers refer to any integer or "floating point "number.
NaN, which stands for Not a Number, is, ironically, a number datatype.
Math operations can be performed on Number data types.
var num = 2;
var numTwo = 2;
var sum = 2 + 2; //=> 4
var summedNums = num + numTwo //=> 4
Above, both the 'sum' and 'summedNums' variables have a value of 4.
Strings
Strings are characters wrapped in quotes.
A string can be created using double, single, or back-tick quotes.
let name = "Matias";
let name = 'Matias';
let name = `Matias`;
Strings can also be added together, an operation called concatenation.
Using back-ticks, variables can also be placed directly into a string. This is known as a template string, or template literal.
let name = "Matias";
let greeting = "Hello, "
let nameGreet = greeting + name;
let tempString = `Hello, ${name}`;
Booleans
The boolean data type has only two values: true and false.
let isAndrewAwesome = true;
let isItPartyTime = false;
Null and Undefined
Null means the absence of a value, or that the value represents is nothing.
Undefined is a data type itself, and occurs when a variable is declared but not given a value;
let nothing = null;
let notDefined;
Arrays
Arrays in JavaScript are a data type that is used to create lists, or indexed data.
They are created using [angle brackets], with each element separated by a comma.
These elements are organized by indexes, which start at 0.
Arrays can hold any data type in an index, including other arrays!
let myArray = [1, 'Hello', true, null];
0
1
2
3
Index:
Objects
Objects are a data type that is used to create representations of related data.
Objects are separated into key/value pairs, with the key being how we reference each value.
Object keys are also called properties interchangeably.
Objects are created using {curly brackets}.
let myObj = {
name: 'Matias',
age: 26
};
JavaScript Functions
Functions can be seen as 'actions' in our JavaScript, and they help bring our code to life.
Functions are what are used to "do stuff" or "make stuff happen" on a webpage
function sayHello(){
return 'Hello!'
};
sayHello();
Function Syntax
There are two ways we can create functions, known as function declarations and function expressions.
//Function Declaration
function sayHello(){
return 'Hello!'
};
//Function Expression
var sayHello = function(){
return 'Hello!'
};
Function keyword tells JS that this is a function
Name of the function
What the function returns
The above code is just the blueprint of the function.
To actual use our function, we have to invoke, or call, the function, as seen below.
sayHello();
Parameters and Arguments
When building, or defining our function, we can specify parameters for our function.
Parameters are placeholders for arguments that will be passed in when the function is called.
function toaster(slot1, slot2){
return `${slot1} was toasted, ${slot2} was burned`;
};
toaster('rye bread', 'wheat bread');
Truthy and Falsy Values
In JavaScript, all values are either considered truthy or falsy values.
There are only six values in JavaScript that are falsy.
False
Null
Undefined
Empty String
0 (as a number)
NaN
Operators
= Assignment Operator
== Equal to
=== Strictly Equal to
> Greater than
>= Greater than or equal to
< Less than
<= Less than or equal to
&& Logical 'and' Operator
|| Logical 'or' Operator
!== Not Equal to
If/Else Statements
If/Else Statements allow functionality dependent on conditions that are passed in
if(7 === 7){
return'This is true!'
} else if(7 === '7'){
return 'This is not true!'
} else {
return 'Default Action'
}
Condition to be evaluated
Action block
Multiple comparisons can be evaluated in an if/else statement
The else block runs if none of the conditions pass.
Introduction to Scope
In JavaScript, everything has a scope; this refers to the 'level' the code is running in.
The top most scope is known as the global scope.
The scope in functions is known as the local, or lexical scope.
let global = "Global Scope";
function lexFunc(){
return "Lexical Scope";
};
JavaScript 1
By matias_perez
JavaScript 1
Introduction to JavaScript
- 334