Web Cartography 5
JavaScript
Why?
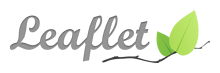



What?
user interaction
...
How?
interpreted
free
<head> // or anywhere
<script src=”utils.js”></script>
</head>
Data types and variables
weakly typed language
Numerical data
whole numbers
fractional numbers
Text data
"string" or
'string' or
"I\"m a string" or
'I\'m a string" or
'I"m a string' or
"I'm a string"
Boolean data
true/false
new Boolean(false) === false (wtf?)
Variables
in-memory data
temporal
case sensitive
reserved words
[a-zA-Z0-9_$]
var firstVariable; // declare
firstVariable = 1; // assign value
alert(firstVariable);
firstVariable = 'hello';
alert(firstVariable);
var secondVariable = 2;
firstVariable = secondVariable;
console.log(firstVariable);
secondVariable = 'world';
console.log(firstVariable); data are copied on assignment
Calculations
var sum = 1 + 2 + 4 + 5;
sum += 20;
sum *= 5;
sum /= 9;
sum -= 1;
String operations
var str = 'Hello ' + 'world';
var name = prompt("Your name is", "unknown");
alert('Hello, ' + name);
document.write('Hello, ' + name); // document?
alert ("I'm " + 26 + " years old");
Arrays
more than one item of data at the same time
zero-based indexing!
var myArray = [];
myArray[0] = 'Hello';
myArray[1] = 'World';
myArray[2] = 1500;
var myArray2 = ['how', 'are', 'you'];
Exercise
degrees to decimal converter

Decisions
Comparison operators
>, <, >=, <=, ===, ==, !=, !==
var bigEnough = 1000 > 1;
If
if (1000 > 1) {
alert('Big enough');
}
var x = 0;
var y = x > 0 ? 'positive' : 'negative';
console.log(y);
logical operators
- AND &&
- OR ||
- NOT !
t && t -> true
t && f -> false
f && t -> false
f && f -> false
t || t -> true
t || f -> true
f || t -> true
f || f -> false
!t -> f
!f -> t
!!t -> t
!!f -> f
if (condition1) {
statement1
} else if (condition2) {
statement2
}
else if (condition3) {
statement3
}
...
else {
statementN
}
Switch
switch (expression) {
case value1:
//Statements executed when the result of expression matches value1
[break;]
case value2:
//Statements executed when the result of expression matches value2
[break;]
...
case valueN:
//Statements executed when the result of expression matches valueN
[break;]
default:
//Statements executed when none of the values match the value of the expression
[break;]
}
Loops
For
for ([initialization]; [condition]; [final-expression]) {
statement
}
for (var i = 0; i <= 9; i++) {
console.log(i);
// more statements
}
var arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for (var len = arr.length - 1; len >= 0; len -= 1) {
console.log('index: ', len);
console.log('value: ',arr[len]);
}
While
unknown number of iterations
while (condition) {
statement
}
var n = 1000,
x = 0;
while (n > 0) {
x += n;
n -= 1;
}
Do...while
executes at least once
do {
statement
} while (condition);
var i = 0;
do {
i += 1;
console.log(i);
} while (i < 0);
Break and Continue
exit the loop prematurely
var arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
for (var i = 0, len = arr.length; i < len; i +=1) {
if (arr[i] % 2 > 0) {
break;
}
console.log(arr[i]);
console.log(arr[i]/2);
}
var arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
for (var i = 0, len = arr.length; i < len; i +=1) {
if (arr[i] % 2 > 0) {
continue;
}
console.log(arr[i]);
console.log(arr[i]/2);
}
Functions
parameters
return values
function scope
function name([param[, param[, ... param]]]) {
statements
}
name([param[, param[, ... param]]]) // function call
Exercise
degrees to decimal converter

Web Cartography 5: JavaScript
By Michal Zimmermann
Web Cartography 5: JavaScript
- 1,639