JavaScript
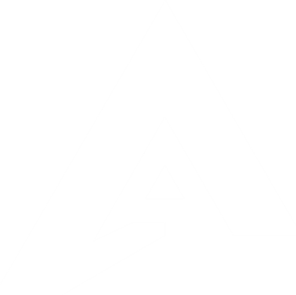
What is JavaScript?
- JavaScript is a cross-platform, object-oriented scripting language.
- It is used to make webpages interactive (e.g., complex animations, clickable buttons, popup menus).
- JavaScript can be used for both client-side and server-side applications.
JavaScript in Web Browsers
Client-Side JavaScript:
- Extends core JavaScript by adding objects to control the browser and the Document Object Model (DOM).
- Allows developers to create dynamic and interactive elements on webpages, such as:
- Manipulating HTML elements.
- Responding to user events (e.g., mouse clicks, form inputs, page navigation).
JavaScript on the Server
Server-Side JavaScript:
- Extends core JavaScript for server environments using frameworks like Node.js.
- Enables the creation of more advanced web functionalities, such as:
- Real-time collaboration between multiple computers.
- Handling server-side operations like database communication and file manipulation.
Key Features of JavaScript
- JavaScript provides a standard library of objects, such as:
- Array, Date, and Math.
- It includes a core set of language elements:
- Operators, control structures, and statements.
- Can be extended with additional objects for various purposes (client-side or server-side).
Interactivity and Connectivity
- In the browser, JavaScript can modify the webpage's DOM dynamically.
- Server-side JavaScript (e.g., Node.js) can handle custom requests from client-side scripts, enabling a seamless interaction between client and server.
JavaScript | Java |
---|---|
Object-oriented. No distinction between types of objects. Inheritance is through the prototype mechanism, and properties and methods can be added to any object dynamically. | Class-based. Objects are divided into classes and instances with all inheritance through the class hierarchy. Classes and instances cannot have properties or methods added dynamically. |
Variable data types are not declared (dynamic typing, loosely typed). | Variable data types must be declared (static typing, strongly typed). |
Cannot automatically write to hard disk. | Can automatically write to hard disk. |
JavaScript and the ECMAScript Specification
- JavaScript is standardized by Ecma International, an association for standardizing information and communication systems.
- The standardized version of JavaScript is called ECMAScript.
- ECMAScript ensures that JavaScript behaves consistently across all environments that support the standard.
The ECMA-262 and ISO Standards
- The ECMAScript standard is documented in the ECMA-262 specification.
- ECMA-262 is also approved as an ISO standard (ISO-16262).
- The specification is available on the Ecma International website.
What ECMAScript Covers
- The ECMAScript specification defines core language features:
- Syntax, types, operators, objects, and control structures.
- Does not include the Document Object Model (DOM), which is standardized by the W3C and WHATWG.
- The DOM is how HTML documents are exposed to scripts, allowing JavaScript to manipulate web pages.
ECMAScript Specification
vs.
JavaScript Documentation
ECMAScript Specification:
- Provides a detailed set of requirements for language implementation (e.g., SpiderMonkey in Firefox, V8 in Chrome).
- Intended for developers who implement JavaScript engines, not for script programmers.
JavaScript Documentation
- Designed for script programmers.
- Focuses on practical aspects of JavaScript relevant to developers.
- Covers language features, the DOM, and other web-specific APIs.
Running JavaScript
Recent versions of Firefox, Chrome, Microsoft Edge, and Safari all support the features discussed in this guide.
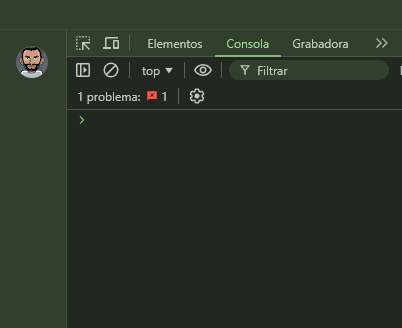
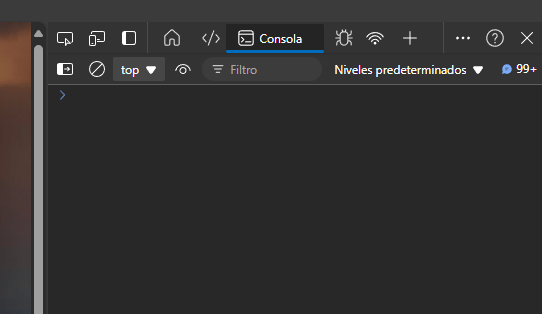
Introduction to JavaScript Syntax
-
JavaScript Syntax Influences
- Borrows from Java, C, C++
- Influenced by Awk, Perl, Python
- JavaScript is case-sensitive
- Uses Unicode character set
Statements and Semicolons
-
Statements:
- Instructions in JavaScript
- Separated by semicolons (
;
)
-
Semicolon Usage:
- Not necessary if statements are on separate lines
- Required when multiple statements are on the same line
-
Best Practice:
- Always use semicolons to avoid errors
- Automatic Semicolon Insertion (ASI) rules exist
JavaScript Input Elements
-
Source Text:
- Scanned from left to right
- Converted into input elements: tokens, control characters, etc.
-
Whitespace:
- Includes spaces, tabs, and newlines
- Considered as whitespace and ignored
Comments in JavaScript
// a one line comment
/* this is a longer,
* multi-line comment
*/
-
Comment Behavior:
- Cannot nest block comments
- Comments behave like whitespace and are discarded
-
Hashbang Comments:
-
#!/usr/bin/env node
(for specifying a JS engine)
-
Variable Declarations
// Bindings
var mainAxis = "row";
let i = 10;
const dot = ".";
-
var
: Declares a variable (function/global scope) -
let
: Declares a block-scoped variable -
const
: Declares a block-scoped, read-only constant
let mood = "light";
console.log(mood);
mood = "dark";
console.log(mood);
Binding Names
Can be any word, digits, $ and _
but the name must not start with a digit
Variable Scope
- Global Scope: Default scope for all script-mode code
- Module Scope: For module-mode code
- Function Scope: Created with functions
-
Block Scope: Created with curly braces
{}
(forlet
andconst
)
if (Math.random() > 0.5) {
const y = 5;
}
console.log(y); // ReferenceError: y is not defined
Variable Hoisting
-
var
declarations are hoisted -
let
andconst
declarations are not hoisted to the top of their block - Variables declared with
let
orconst
cannot be accessed before declaration
console.log(x); // undefined
var x = 3;
function test() {
console.log(y); // undefined
var y = "local value";
}
Global Variables
-
Global Object:
- In web pages, the global object is
window
- Use
window.variable
orglobalThis.variable
to access globals
- In web pages, the global object is
-
Cross-window Access:
- Access global variables from different windows using
parent.variableName
- Access global variables from different windows using
Constants in JavaScript
- Cannot change value after assignment
- Must be initialized during declaration
const PI = 3.14;
const MY_OBJECT = { key: "value" };
MY_OBJECT.key = "otherValue"; // Allowed
const MY_ARRAY = ["HTML", "CSS"];
MY_ARRAY.push("JAVASCRIPT");
console.log(MY_ARRAY); // ['HTML', 'CSS', 'JAVASCRIPT'];
Environment
The collection of bindings and their values that exist at a given time is called the environment.
var nombre = prompt("Ingrese su Nombre");
var mensaje = `Hola ${nombre}, que hermoso eres`;
console.log(mensaje);
alert(mensaje);
Data Types
Seven data types that are primitives:
- Boolean: true and false.
- null: A special keyword denoting a null value.
- undefined: A top-level property whose value is not defined.
- Number: An integer or floating point number.
- BigInt: An integer with arbitrary precision.
- String: A sequence of characters that represent a text value.
- Symbol: A data type whose instances are unique and immutable.
and
- Object
Numbers
fixed with 64 bits
// All the following values are Numbers
10
-10
10.2
-10.2
console.log(typeof 10);
// 10 x 10 ^ 2
10e2;
// -10 x 10 ^ 3
-10e3;
// Operators
10 + 11;
10 - 11;
10 * 20;
13 / 40;
40 % 2;
Operators
Arithmetic Operators: These are used to perform basic mathematical operations.
// Hierarchy of operators
3 + 5 / 2(3 + 5) / 2;
Operators
Operator Hierarchy (Order of Operations): JavaScript follows the standard mathematical order of operations, also known as "PEMDAS" (Parentheses, Exponents, Multiplication and Division, Addition and Subtraction). Operations within parentheses are executed first, followed by division, and then addition.
// Infinity AND -Infinity
1 / 0; -1 / 0;
// NaN
0 / 0;
Operators
Infinity and -Infinity: In JavaScript, dividing a number by zero results in Infinity
or -Infinity
, depending on the sign of the number.
NaN (Not-a-Number): This special value represents an undefined or unrepresentable value, particularly in arithmetic operations where the result is not a real number, such as dividing zero by zero.
String
16 bits per string element
// String Values
'I am a String'
"I am a String"
'Scape character \' '
"Scape character \" "
' Multiline
line 2 '
// Template String
`half of 100 is ${100 / 2}` // half of 100 is 50
// String Values
"a" + "b";
"a".length;
"abcdefg"[0];
"abcdefg"[3];
"a,b,c,d".split(",");
" ok ".trim(); // "OK"
String
// Boolean Values
true
false
5 > 2
5 >= 2
3 < 4
4 <= 4
5 != 5
5 == 5
"5" == 5
5 === 5
"5" !== 5
Boolean
// Boolean Values
true
false
null || 2
null && 2
true || false
true && false
!true
!false
NaN == NaN // → false
Boolean
Short-circuiting of logical operators
console.log(null || "user")
// → user
console.log("Agnes" || "user")
// → Agnes
JavaScript Data Type Conversion
- Dynamically Typed Language: JavaScript does not require explicit data type declaration.
- Automatic Type Conversion: Data types are converted as needed during execution.
let answer = 42;
// No error, type is dynamically changed
answer = "Thanks for all the fish!";
Numbers and the '+' Operator in JavaScript
- The
+
operator can lead to type conversion when used with strings and numbers. - Numeric values are converted to strings in expressions involving
+
.
x = "The answer is " + 42; // Result: "The answer is 42"
y = 42 + " is the answer"; // Result: "42 is the answer"
z = "37" + 7; // Result: "377"
Type Conversion with Other Operators
- Operators other than
+
do not convert numbers to strings. - JavaScript treats operands as numbers where possible.
"37" - 7; // Result: 30
"37" * 7; // Result: 259
Converting Strings to Numbers
- parseInt(): Converts a string to an integer (whole number).
- parseFloat(): Converts a string to a floating-point number (decimal).
-
Unary Plus (
+
): Converts a string directly to a number.
parseInt("101", 2); // Result: 5 (binary to decimal conversion)
"1.1" + "1.1"; // Result: "1.11.1" (string concatenation)
(+"1.1") + (+"1.1"); // Result: 2.2 (number addition)
Array Literals
- An array literal is a list of expressions enclosed in square brackets
[]
. - Initializes an array with specified values.
- The array's length is set to the number of elements.
- Note: An array literal creates a new array object each time it is evaluated.
const coffees = ["French Roast", "Colombian", "Kona"];
Arrays in Different Scopes
- Global Scope: Array literal created once when the script loads.
- Function Scope: A new array is instantiated every time the function is called.
- Note: Array literals create Array objects.
function createArray() {
const array = ["Item1", "Item2"];
// New array created on each function call
}
Extra Commas in Array Literals
- Extra Commas: Leave empty slots in the array.
- Trailing Commas: Ignored in array literals.
const fish = ["Lion", , "Angel"];
// Result: [ 'Lion', <1 empty item>, 'Angel' ]
const myList = ["home", , "school"];
// Length: 3, myList[1] is empty
const myList2 = [, "home", , "school"];
// Length: 4, myList[0] and myList[2] are missing
const myList3 = ["home", , "school", ,];
// Length: 4, myList[1] and myList[3] are missing
Handling Extra Commas
Trailing Commas: Help keep git diffs clean by adding one line for new elements.
const myList = [
"home",
"school",
+ "hospital",
];
Expression
// Expressions
10 + 5 <= 10 + 6
Mental Exercise
let x = 10,
y = "a";
console.log( y === b || x >= 10 );
Which is the result?
Block statement
{
statement1;
statement2;
// …
statementN;
}
The most basic statement is a block statement, which is used to group statements. The block is delimited by a pair of curly braces
Control Flow
- if else
- while
- for
- switch
// IF
if (2 >= 3) {
console.log(0);
} else {
console.log(1);
}
if (2 >= 3) {
console.log(0);
} else if( 4 < 2) {
console.log(1);
} else {
console.log(2);
}
switch (prompt("What is the weather like?")) {
case "rainy":
console.log("Remember to bring an umbrella.");
break;
case "sunny":
console.log("Dress lightly.");
case "cloudy":
console.log("Go outside.");
break;
default:
console.log("Unknown weather type!");
break;
}
// Loop While
let a = 10;
while (a >= 0) {
console.log(a);
a--;
}
// Loop Do While
do {
console.log(a);
a++
} while(a <= 10);
for(let i = 0 ; i <= 10 ; i++) {
console.log(i);
}
// The are other syntax, see the next slides
The following values evaluate to false (also known as Falsy values):
- false
-
undefined
-
null
-
0
-
NaN
-
the empty string ("")
Exception Handling Statements
- Throw Statement: Used to throw an exception.
- Try...Catch Statement: Used to handle exceptions thrown by the throw statement.
-
Exception Types: Various types of objects can be thrown, including:
- ECMAScript exceptions
- DOMException
The throw
Statement
Purpose: To throw an exception with a specified value.
throw expression;
throw "Error2"; // String type
throw 42; // Number type
throw true; // Boolean type
throw {
toString() {
return "I'm an object!";
},
};
The try...catch
Statement
Purpose: To catch and handle exceptions.
try {
// Statements to try
} catch (exception) {
// Statements to handle the exception
}
function getMonthName(mo) {
mo--; // Adjust month number for array index
const months = ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"];
if (months[mo]) {
return months[mo];
} else {
throw new Error("InvalidMonthNo");
}
}
try {
monthName = getMonthName(myMonth);
} catch (e) {
monthName = "unknown";
logMyErrors(e); // Handle the error
}
The finally
Block
Purpose: To execute statements after try and catch blocks, regardless of whether an exception was thrown
- Executes even if an exception is thrown or not.
- Overrides return values and re-thrown exceptions from the catch block.
openMyFile();
try {
writeMyFile(theData);
} catch (e) {
handleError(e);
} finally {
closeMyFile(); // Always executed
}
Nesting try...catch
Statements
- Purpose: To handle exceptions at different levels of the code.
-
Rules:
- Inner
try
blocks must have afinally
block if they don't have their owncatch
block. - The enclosing
try...catch
statement'scatch
block handles exceptions if no innercatch
block is present.
- Inner
Utilizing Error Objects
- Purpose: To provide detailed information about exceptions.
-
Properties:
-
name
: General class of the error (e.g.,DOMException
). -
message
: Detailed error message.
-
function doSomethingErrorProne() {
if (ourCodeMakesAMistake()) {
throw new Error("The message");
} else {
doSomethingToGetAJavaScriptError();
}
}
try {
doSomethingErrorProne();
} catch (e) {
console.error(e.name); // 'Error'
console.error(e.message); // 'The message'
}
Functions
- Functions are fundamental building blocks in JavaScript.
- They perform tasks or calculate values.
- A function takes input and returns an output.
- Functions must be defined in the scope from which they are called.
Defining Functions
Function Declarations: Consist of:
-
function
keyword - Function name
- List of parameters in parentheses
- Function body in curly braces
Parameters: Passed by value. Changes to objects or arrays inside the function affect the original object or array.
function square(number) {
return number * number;
}
Function Parameters
Arguments are optional if you pass too many, the extra ones are ignored. If you pass too few, the missing parameters get assigned the value undefined.
function minus(a, b) {
if (b === undefined) return -a;
else return a - b;
}
console.log(minus(10));
console.log(minus(10, 5));
function power(base, exponent = 2) {
let result = 1;
for (let count = 0; count < exponent; count++) {
result *= base;
}
return result;
}
Function Parameters
Objects: Changes to object properties are visible outside the function.
function myFunc(theObject) {
theObject.make = "Toyota";
}
const mycar = {
make: "Honda",
model: "Accord",
year: 1998,
};
console.log(mycar.make); // "Honda"
myFunc(mycar);
console.log(mycar.make); // "Toyota"
Function Parameters
Arrays: Changes to array elements are visible outside the function.
function myFunc(theArr) {
theArr[0] = 30;
}
const arr = [45];
console.log(arr[0]); // 45
myFunc(arr);
console.log(arr[0]); // 30
Function Expressions
- Functions can be created using function expressions.
- Can be anonymous or named.
const square = function (number) {
return number * number;
};
console.log(square(4)); // 16
const factorial = function fac(n) {
return n < 2 ? 1 : n * fac(n - 1);
};
console.log(factorial(3)); // 6
Functions as Arguments
- Functions can be passed as arguments to other functions.
function map(f, a) {
const result = new Array(a.length);
for (let i = 0; i < a.length; i++) {
result[i] = f(a[i]);
}
return result;
}
const cube = function (x) {
return x * x * x;
};
const numbers = [0, 1, 2, 5, 10];
console.log(map(cube, numbers)); // [0, 1, 8, 125, 1000]
Conditional Function Definition
Functions can be defined conditionally.
let myFunc;
if (num === 0) {
myFunc = function (theObject) {
theObject.make = "Toyota";
};
}
Function Scope
- Scope: Variables defined inside a function are only accessible within that function.
- Access: A function can access all variables and functions in its defining scope.
const num1 = 20;
const num2 = 3;
const name = "Chamakh";
function multiply() {
return num1 * num2;
}
console.log(multiply()); // 60
function getScore() {
const num1 = 2;
const num2 = 3;
function add() {
return `${name} scored ${num1 + num2}`;
}
return add();
}
console.log(getScore()); // "Chamakh scored 5"
Recursion and the Function Stack
- Recursion: A function that calls itself. Requires a base condition to stop.
- Function Stack: Recursion uses a stack to manage function calls.
Closure
is the combination of a function and the lexical environment within which that function was declared.
A closure is a function that remembers its lexical scope even when it's executed outside of that scope. In simpler terms, it's a function that "remembers" the variables and the environment in which it was created.
function multiplier(factor) {
return number => number * factor;
}
let twice = multiplier(2);
console.log(twice(5));
Multiply-Nested Functions and Scope Chaining
- Scope Chaining: Nested functions can access variables from all containing scopes.
- Example: A function inside another can access all variables from its parent functions.
function A(x) {
function B(y) {
function C(z) {
console.log(x + y + z);
}
C(3);
}
B(2);
}
A(1); // Logs 6
Name Conflicts
When variables or parameters with the same name exist in different scopes, the innermost scope takes precedence.
function outside() {
const x = 5;
function inside(x) {
return x * 2;
}
return inside;
}
console.log(outside()(10)); // 20
-
Private variables: Closures allow you to create variables that are only accessible from within the inner function, simulating the behavior of private variables in object-oriented languages.
-
Callbacks and events: They are essential for handling callbacks and events in JavaScript, allowing a function to access variables from an outer scope.
-
Modules: Closures are used to create modules, encapsulating related code and data.
-
Currying: Currying, a technique for transforming functions with multiple arguments into a sequence of functions with a single argument, relies on closures
Rest Parameters
function min(...numbers) {
let result = Infinity;
for (let number of numbers) {
if (number < result) result = number;
}
return result;
}
let words = ["never", "fully"];
console.log(["will", ...words, "understand"]);
Arrow functions
- Definition: Arrow function expressions offer a shorter syntax and differ from traditional function expressions.
-
Characteristics:
- Shorter syntax.
- No own
this
,arguments
,super
, ornew.target
. - Always anonymous.
Will work more in the next slides
// binding function
function play() {
console.log("Ta Ta Taaaaaaaaaaaaa");
}
var scream = function () {
console.log("Aaaaahhhh!!")
};
var motivate = () => {
console.log("Just do it!")
}
Arrays
Arrays are a fundamental data structure in JavaScript. They allow you to store multiple items under a single variable name. This makes it easier to manage and work with collections of data.
Creating Arrays
-
Array literal: This is the most common way to create an array. You simply enclose a comma-separated list of items within square brackets (
[]
). -
Array constructor: The
Array()
constructor can also be used to create arrays. However, it is generally less common than the array literal syntax.
const fruits = ['apple', 'banana', 'orange'];
const numbers = new Array(1, 2, 3, 4, 5);
Accessing Array Elements
You can access individual elements of an array using their index. The index starts from 0, so the first element has an index of 0, the second element has an index of 1, and so on.
const firstFruit = fruits[0];
Array Properties and Methods
Arrays have a number of built-in properties and methods that allow you to perform various operations on them. Here are some commonly used ones:
- length: This property returns the number of elements in the array.
- push(): This method adds one or more elements to the end of an array and returns the new length of the array.
- pop(): This method removes the last element from an array and returns the removed element.
- shift(): This method removes the first element from an array and returns the removed element.
Array Properties and Methods
- unshift(): This method adds one or more elements to the beginning of an array and returns the new length of the array.
- slice(): This method extracts a section of an array and returns a new array containing the extracted elements.
- concat(): This method merges two or more arrays and returns a new array containing the elements of all the input arrays.
let list = [2, 3, 5, 7, 11];
list.push(12); // Add an element in the end of array
console.log(list);
console.log(list.pop()); // Extract a last element of array
console.log(list);
list.unshift(1); // Add an element beginning of the array
list.shift(1); // Extract an element beginning of the array
console.log(list.indexOf(2))
console.log(list.slice(2,5));
console.log(list.slice(5));
console.log(list.concat([1,3,4,5]));
const array1 = ['a', 'b', 'c'];
for (const element of array1) {
console.log(element);
}
For of statement
Date Object in JavaScript
- JavaScript doesn't have a native date data type.
- The
Date
object provides methods to work with dates and times.
Creating Date Objects
const now = new Date();
console.log(now); // Outputs the current date and time
const specificDate = new Date('2024-09-01T10:00:00');
console.log(specificDate); // Outputs: Sat Sep 01 2024 10:00:00 GMT...
const dateFromComponents = new Date(2024, 8, 1, 10, 0, 0); // Note: Month is zero-indexed
console.log(dateFromComponents); // Outputs: Sun Sep 01 2024 10:00:00 GMT...
Getting Date Components
const date = new Date();
console.log(date.getFullYear()); // Outputs the current year
console.log(date.getMonth()); // Outputs the current month (0-11)
console.log(date.getDate()); // Outputs the current day of the month
console.log(date.getHours()); // Outputs the current hour
console.log(date.getMinutes()); // Outputs the current minute
console.log(date.getSeconds()); // Outputs the current second
Setting Date Components
const date = new Date();
date.setFullYear(2025);
date.setMonth(11); // December
date.setDate(25);
console.log(date); // Outputs: Thu Dec 25 2025 ...
date.setHours(15);
date.setMinutes(30);
date.setSeconds(45);
console.log(date); // Outputs: Thu Dec 25 2025 15:30:45 GMT...
Formatting Dates
const date = new Date();
console.log(date.toDateString()); // Outputs: Fri Sep 01 2024
console.log(date.toTimeString()); // Outputs: 10:00:00 GMT+0000 (Coordinated Universal Time)
console.log(date.toLocaleDateString()); // Outputs: 9/1/2024 (depends on locale)
console.log(date.toLocaleTimeString()); // Outputs: 10:00:00 AM (depends on locale)
Date Arithmetic
const date = new Date();
const futureDate = new Date(date);
futureDate.setDate(date.getDate() + 10); // Add 10 days
console.log(futureDate); // Outputs: (Date 10 days from now)
const date1 = new Date();
const date2 = new Date('2024-09-01');
const diffInMilliseconds = date2 - date1;
const diffInDays = diffInMilliseconds / (1000 * 60 * 60 * 24);
console.log(diffInDays); // Outputs the difference in days
Handling Timezones
const date = new Date();
console.log(date.toUTCString()); // Outputs date in UTC timezone
console.log(date.toLocaleString()); // Outputs date and time in local timezone
Understanding Regular Expressions in JavaScript
What are Regular Expressions?
- A regular expression (regex) is a sequence of characters that forms a search pattern.
- Used for string matching and manipulation.
- Commonly used for validation, searching, and replacing text.
Basic Syntax of Regular Expressions
Literal Characters:
- Matches the exact characters.
const regex = /hello/;
console.log(regex.test("hello world")); // true
Metacharacters:
- Characters with special meanings, like
.
,*
,+
,?
,^
,$
, etc.
Special Characters in Regular Expressions
Dot (.
):
- Matches any single character except newline.
const regex = /h.llo/;
console.log(regex.test("hello")); // true
console.log(regex.test("hallo")); // true
Special Characters in Regular Expressions
Asterisk (*
):
- Matches zero or more of the preceding character.
const regex = /ho*/;
console.log(regex.test("hoop")); // true
console.log(regex.test("h")); // true
Character Classes and Sets
Character Classes:
-
\d
matches any digit (0-9). -
\w
matches any word character (alphanumeric plus underscore). -
\s
matches any whitespace character.
const regex = /\d/;
console.log(regex.test("There are 3 apples")); // true
Character Classes and Sets
Character Sets:
- Defined using square brackets
[]
. -
[a-z]
matches any lowercase letter. -
[A-Z]
matches any uppercase letter.
const regex = /[a-z]/;
console.log(regex.test("Hello")); // true
Quantifiers in Regular Expressions
-
*
- Matches 0 or more times. -
+
- Matches 1 or more times. -
?
- Matches 0 or 1 time. -
{n}
- Matches exactly n times. -
{n,}
- Matches at least n times. -
{n,m}
- Matches between n and m times.
const regex = /\d{2,4}/;
console.log(regex.test("123")); // true
console.log(regex.test("1")); // false
Anchors in Regular Expressions
-
Start (
^
):- Matches the start of a string.
-
End (
$
):- Matches the end of a string.
const regex = /^hello/;
console.log(regex.test("hello world")); // true
console.log(regex.test("world hello")); // false
Anchors in Regular Expressions
- Matches a word boundary (the position between a word and a non-word character).
const regex = /\bworld\b/;
console.log(regex.test("hello world!")); // true
console.log(regex.test("helloworld")); // false
Groups and Ranges
Grouping:
- Parentheses
()
are used to group expressions.
const regex = /(abc)+/;
console.log(regex.test("abcabc")); // true
Regular Expression Flags
Flags modify the behavior of a regex search:
-
i
: Case-insensitive search. -
g
: Global search. -
m
: Multi-line search.
const regex = /hello/i;
console.log(regex.test("Hello")); // true
Useful Regular Expression Methods
test()
:
- Tests for a match in a string. Returns
true
orfalse
.
const regex = /\d+/;
console.log(regex.test("123")); // true
Useful Regular Expression Methods
match()
:
- Returns an array of matches or
null
.
const regex = /\d+/g;
console.log("There are 123 and 456".match(regex)); // ["123", "456"]
Useful Regular Expression Methods
replace()
:
- Replaces matched substrings in a string.
const regex = /apples/g;
console.log("I like apples".replace(regex, "oranges")); // "I like oranges"
Examples of Regular Expressions in Action
const regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
console.log(regex.test("example@example.com")); // true
const regex = /^\d{4}-\d{4}$/; // salvadoren format
console.log(regex.test("123-456-7890")); // true
Objects
An object is a collection of properties, each with a name (or key) and a value
Creating Objects
Object Literals:
- Syntax:
{ key1: value1, key2: value2, ... }
const car = {
make: "Ford",
model: "Mustang",
year: 1969
};
Creating Objects
Constructor Functions:
- Define objects using functions and the
new
keyword.
function Car(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
const myCar = new Car("Ford", "Mustang", 1969);
Object Initializers (Object Literals)
{ property1: value1, property2: value2, ... }
- Allows for easy object creation.
- Properties can be strings, numbers, or variables.
- Can use computed properties with square brackets.
const person = {
name: "John",
age: 30,
job: "Engineer"
};
Constructor Functions
- Create a template function for an object type.
- Use
this
keyword to define properties. - Useful for creating multiple objects of the same type.
- Allows for the encapsulation of object behavior.
function Person(name, age) {
this.name = name;
this.age = age;
}
const person1 = new Person("Alice", 25);
Using Object.create()
- Creates a new object with the specified prototype object and properties.
- Flexibility in object creation.
- Allows for inheritance without needing a constructor function.
-
Syntax:
Object.create(proto,[propertiesObject])
const animal = {
type: "Invertebrates",
displayType() {
console.log(this.type);
}
};
const fish = Object.create(animal);
fish.type = "Fishes";
fish.displayType(); // Logs: "Fishes"
Accessing Object Properties
-
Dot Notation:
- Example:
objectName.propertyName
- Usage: Direct and straightforward for most properties.
- Example:
-
Bracket Notation:
- Example:
objectName["propertyName"]
- Usage: Needed for dynamic properties or properties with special characters.
- Example:
let person = {
name: "Néstor",
lastname: "Aldana",
};
console.log(person.name);
console.log(person.age); // Undefined
person.age = 23; // defined later
person.name = "Nexxtor" // Change value
console.log(person.age);
delete person.age; // Delete a property
console.log(person.age);
console.log("age" in person); // Age exists in person?
console.log("name" in person); // Name exists in person?
Enumerating Properties
-
for...in
loop for enumerable properties. -
Object.keys()
returns an array of property names. -
Object.getOwnPropertyNames()
returns all property names, enumerable or not.
const obj = { a: 1, b: 2 };
for (const key in obj) {
console.log(key, obj[key]);
}
Properties
-
Adding Properties:
- Direct assignment:
objectName.newProperty = value;
- Dynamic assignment with bracket notation:
objectName["newProperty"] = value;
- Direct assignment:
-
Deleting Properties:
delete objectName.propertyName;
const myObj = { name: "Alice" };
myObj.age = 25; // Add a property
delete myObj.name; // Delete a property
Inheritance and Prototypes
- Inheritance: Objects can inherit properties from other objects (prototypes).
- Prototype Chain: Mechanism by which objects inherit properties and methods from their prototype.
function Animal(type) {
this.type = type;
}
Animal.prototype.speak = function() {
console.log(`The ${this.type} speaks.`);
};
const cat = new Animal("cat");
cat.speak(); // Outputs: "The cat speaks."
Methods in Objects
Functions that are properties of objects.
Prototype Methods:
- Define shared methods for all instances.
- Example
const car = {
make: "Toyota",
drive() {
console.log("Driving...");
}
};
Car.prototype.start = function() {
console.log("Car started");
};
Using this
in Objects
this
Keyword:
- Refers to the object from which a method was called.
- Allows methods to access and modify object properties.
const person = {
name: "Bob",
greet() {
console.log(`Hello, my name is ${this.name}`);
}
};
person.greet(); // Outputs: "Hello, my name is Bob"
function normalize() {
console.log(this.coords.map(n => n / this.length));
}
normalize.call({coords: [0, 2, 3], length: 5});
Arrow functions and this
let empty = {};
console.log(empty.toString);
console.log(empty.toString());
console.log(Object.getPrototypeOf({}) == Object.prototype);
console.log(Object.getPrototypeOf(Object.prototype));
Mutabilidad
let object1 = {value: 10};
let object2 = object1;
let object3 = {value: 10};
console.log(object1 == object2); // true
console.log(object1 == object3); // false
object1.value = 15;
console.log(object2.value); // 15
console.log(object3.value); // 10
JavaScript’s == operator, it compares objects by identity
Destructuring
const [a, b] = array;
const [a, , b] = array;
const [a = aDefault, b] = array;
const [a, b, ...rest] = array;
const [a, , b, ...rest] = array;
const [a, b, ...{ pop, push }] = array;
const [a, b, ...[c, d]] = array;
const { a, b } = obj;
const { a: a1, b: b1 } = obj;
const { a: a1 = aDefault, b = bDefault } = obj;
const { a, b, ...rest } = obj;
const { a: a1, b: b1, ...rest } = obj;
const { [key]: a } = obj;
let a, b, a1, b1, c, d, rest, pop, push;
[a, b] = array;
[a, , b] = array;
[a = aDefault, b] = array;
[a, b, ...rest] = array;
[a, , b, ...rest] = array;
[a, b, ...{ pop, push }] = array;
[a, b, ...[c, d]] = array;
({ a, b } = obj); // parentheses are required
({ a: a1, b: b1 } = obj);
({ a: a1 = aDefault, b = bDefault } = obj);
({ a, b, ...rest } = obj);
({ a: a1, b: b1, ...rest } = obj);
function phi([n00, n01, n10, n11]) {
return (n11 * n00 - n10 * n01) /
Math.sqrt((n10 + n11) * (n00 + n01) *
(n01 + n11) * (n00 + n10));
}
let {name} = {name: "Faraji", age: 23};
console.log(name);
Class
- constructor is a special method for creating and initializing objects created with the class.
- Methods are defined inside the class body.
class MyClass {
constructor(prop1, prop2) {
this.prop1 = prop1;
this.prop2 = prop2;
}
method1() {
console.log('This is method 1');
}
method2() {
console.log('This is method 2');
}
}
Creating an Instance
- Use the new keyword to create an instance of a class.
- The constructor is called when a new instance is created, initializing the object's properties.
const instance = new MyClass('value1', 'value2');
console.log(instance.prop1); // Output: value1
instance.method1(); // Output: This is method 1
Class Inheritance
- extends keyword is used to create a child class.
- Child class inherits all methods from the parent class.
- Methods can be overridden in the child class.
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
class Dog extends Animal {
speak() {
console.log(`${this.name} barks.`);
}
}
const dog = new Dog('Rex');
dog.speak(); // Output: Rex barks.
Static Methods
- Static methods are called on the class itself, not on instances.
- Use the static keyword to define static methods.
class MathUtility {
static add(a, b) {
return a + b;
}
}
console.log(MathUtility.add(5, 10)); // Output: 15
Private Fields and Methods (ES2022)
- Private fields and methods are prefixed with #.
- They cannot be accessed from outside the class.
class Rectangle {
#height = 0;
#width = 0;
constructor(height, width) {
this.#height = height;
this.#width = width;
}
#calculateArea() {
return this.#height * this.#width;
}
getArea() {
return this.#calculateArea();
}
}
const rect = new Rectangle(10, 5);
console.log(rect.getArea()); // Output: 50
// console.log(rect.#height); // Error: Private field '#height' must be declared in an enclosing class
Getters and Setters
- Getters and setters allow you to define how to access and mutate properties.
- Use get and set keywords.
Getters and Setters
class Person {
constructor(name) {
this._name = name;
}
get name() {
return this._name;
}
set name(newName) {
this._name = newName;
}
}
const person = new Person('Alice');
console.log(person.name); // Output: Alice
person.name = 'Bob';
console.log(person.name); // Output: Bob
JSON
- JSON stands for JavaScript Object Notation.
- It is a lightweight data interchange format.
- Easy for humans to read and write.
- Easy for machines to parse and generate.
- Commonly used for transmitting data in web applications.
Notes: JSON is language-independent but uses conventions familiar to programmers of the C family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
JSON Syntax Rules
- keys must be strings enclosed in double quotes.
- Values can be: Strings, Numbers. Objects, Arrays, Booleans (true or false), null.
Notes: JSON syntax is derived from JavaScript object notation syntax but is text-only, making it language-independent and ideal for data exchange.
{
"key": "value"
}
JSON Data Types
- String: "Hello, World!"
- Number: 42, 3.14
- Object:
["red", "green", "blue"]
{
"firstName": "John",
"lastName": "Doe"
}
- Array
- Boolean: true, false
- Null: null
A Simple JSON Example
{
"name": "Jane Doe",
"age": 30,
"isStudent": false,
"courses": ["Mathematics", "Computer Science"],
"address": {
"street": "123 Main St",
"city": "Anytown",
"zip": "12345"
}
}
JSON vs. XML
- JSON:
- More readable and compact.
- Easier to parse with JavaScript.
- Data format only (no display).
- XML:
- More verbose.
- Can include metadata and namespaces.
- Can be used for both data and document markup.
JSON is typically preferred over XML for data interchange due to its simplicity and ease of use with JavaScript, but XML remains in use for complex documents and when strict validation is needed.
Parsing and Stringifying JSON in JavaScript
const jsonString = '{"name": "John", "age": 25}';
const jsonObject = JSON.parse(jsonString);
console.log(jsonObject.name); // Output: John
const jsObject = { name: "Jane", age: 30 };
const jsonStr = JSON.stringify(jsObject);
console.log(jsonStr); // Output: {"name":"Jane","age":30}
Common Use Cases for JSON
-
Web APIs: Used to exchange data between client and server.
-
Configuration Files: Storing settings and configurations.
-
Data Storage: Storing data in NoSQL databases like MongoDB.
-
Data Interchange: Sharing data between different systems or applications.
Best Practices with JSON
- Ensure JSON is well-formed and valid.
- Use meaningful key names and consistent naming conventions.
- Avoid deeply nested structures for readability and performance.
- Validate JSON input to prevent security vulnerabilities like injection attacks.
Symbols
What are Symbols in JavaScript?
- Symbol is a primitive data type introduced in ES6 (ECMAScript 2015).
- Represents a unique and immutable identifier.
- Primarily used as keys for object properties to ensure uniqueness.
Notes: Unlike other primitive data types, each symbol is unique, even if two symbols have the same description.
Creating Symbols
Symbols are created using the Symbol() function.
The description is optional and used for debugging purposes; it does not affect the symbol's uniqueness.
let mySymbol = Symbol();
let anotherSymbol = Symbol('description');
Properties of Symbols
- Unique: No two symbols are the same, even with identical descriptions.
- Immutable: Once created, a symbol's value cannot be changed.
- Hidden: Symbols are not enumerable in for...in loops.
Symbols provide a way to add unique property keys to objects without risking property name collisions.
Using Symbols as Object Keys
Symbols can be used as unique keys in objects.
Using symbols as object keys helps prevent accidental property overwrites or conflicts with other keys.
const sym1 = Symbol('key1');
const obj = {};
obj[sym1] = 'value1';
console.log(obj[sym1]); // Output: 'value1'
Global Symbol Registry
- Symbol.for(): Checks the global symbol registry for a symbol with the given key. If it doesn't exist, it creates a new symbol.
- Symbol.keyFor(): Retrieves the key associated with a symbol in the global registry.
const globalSym = Symbol.for('globalKey');
console.log(Symbol.keyFor(globalSym)); // Output: 'globalKey'
Well-known Symbols
- JavaScript has several built-in symbols known as well-known symbols.
- They are used to change or customize object behaviors.
- Examples:
- Symbol.iterator: Defines default iterator for objects.
- Symbol.toStringTag: Customizes the output of Object.prototype.toString.
- Symbol.hasInstance: Customizes behavior of instanceof.
- Well-known symbols are often used to implement or extend JavaScript's built-in behavior.
Practical Use Cases for Symbols
- Private Properties: Use symbols to create properties that are not accessible through normal property enumeration.
- Unique Keys: Prevents accidental property collisions in objects.
- Custom Iterators: Use Symbol.iterator to create custom iteration logic.
Symbols offer a way to define unique keys and behaviors that help avoid naming collisions and enable advanced programming patterns.
Limitations of Symbols
- Not Enumerable: Symbols are not visible in for...in or Object.keys().
- Not Serializable: Symbols are ignored in JSON.stringify().
- Limited Browser Support: While modern browsers support symbols, older browsers may require polyfills
These limitations are by design, enhancing security and ensuring that symbols provide unique, non-colliding property keys.
Exercise
Create an object that registers a list (Array) of a person's life events in a single day, and a boolean property to check if that person has had an accident during that day
let day = {
events : ["cook", "sleep", "running"],
accident: false
};
Exercise
Create an array to have a journal of person's life event
let journal = [
{
events: ["work", "touched tree", "pizza",
"running", "television"],
accident: false
},
{
events: ["work", "ice cream", "cauliflower",
"lasagna", "touched tree", "brushed teeth"],
accident: false
},
{
events: ["weekend", "cycling", "break",
"peanuts","beer"],
accident: true
}
]
Exercise
Create a function to add a daily entry to a journal
let journal = [];
function addEntry(events, accident) {
journal.push({events, accident});
/******************************************
* Is {events, accident} shorthand to *
* {events: events, accident: accident} *
*****************************************/
}
addEntry(["weekend", "cycling", "break", "peanuts", "beer"]
, true);
addEntry(["weekend", "cycling", "break"], false);
Higher-order functions
Functions that operate on other functions
function repeat(n, action) {
for (let i = 0; i < n; i++) {
action(i);
}
}
let departments = [];
repeat(5, i => departments.push(`Department ${i}`));
console.log({departments}); // Only for get a name of the var
Abstracting
function lessThan(n) {
return m => m < n;
}
let lessThan10 = lessThan(10);
console.log(lessThan10(4));
function unless(test, then) {
if (!test) then();
}
unless( 2 > 3, n => console.log("ok"))
Create new functions
["A", "B"].forEach(i => console.log(i));
Array Higher-Order Functions
Exercise
Create a Higher-Order function that filters an array
function filter(array, test) {
let passed = [];
for (let element of array) {
if (test(element)) {
passed.push(element);
}
}
return passed;
}
filter([1,3,5,6,7,10], e => e % 2 == 0);
Array Higher-Order Functions
console.log([1,3,5,6,7,10].filter(e => e % 2 == 0));
Array Higher-Order Functions
Exercise
Create a Higher-Order function that transforms an array
function map(array, transform) {
let mapped = [];
for (let element of array) {
mapped.push(transform(element));
}
return mapped;
}
let cars = [{ brand: "Toyota", year: "1990"},
{ brand: "Dogge", year: "2020"},
{ brand: "Pollito", year: "2018"},];
map(cars, e=> e.brand);
Array Higher-Order Functions
let cars = [{ brand: "Toyota", year: "1990"},
{ brand: "Dogge", year: "2020"},
{ brand: "Pollito", year: "2018"},];
cars.map(e => e.brand);
Array Higher-Order Functions
Exercise
Create a Higher-Order function that reduces an array to one value
function reduce(array, combine, start) {
let current = start;
for (let element of array) {
current = combine(current, element);
}
return current;
}
console.log(reduce([1, 2, 3, 4], (a, b) => a + b, 0));
Array Higher-Order Functions
JavaScript - Lenguaje de Programación
By Néstor Aldana
JavaScript - Lenguaje de Programación
Que es JavaScript, Tipos de datos, operaciones básicas, funciones, estructura de un programa, datos estructurados
- 638