Float on Shodan Pentesting
Using Libraries with Python
Lecturer: Иo1lz Date: Apr. 5th, 2020
Outline
-
Why Shodan?
-
How to use Shodan?
-
Demo
Why Shodan?
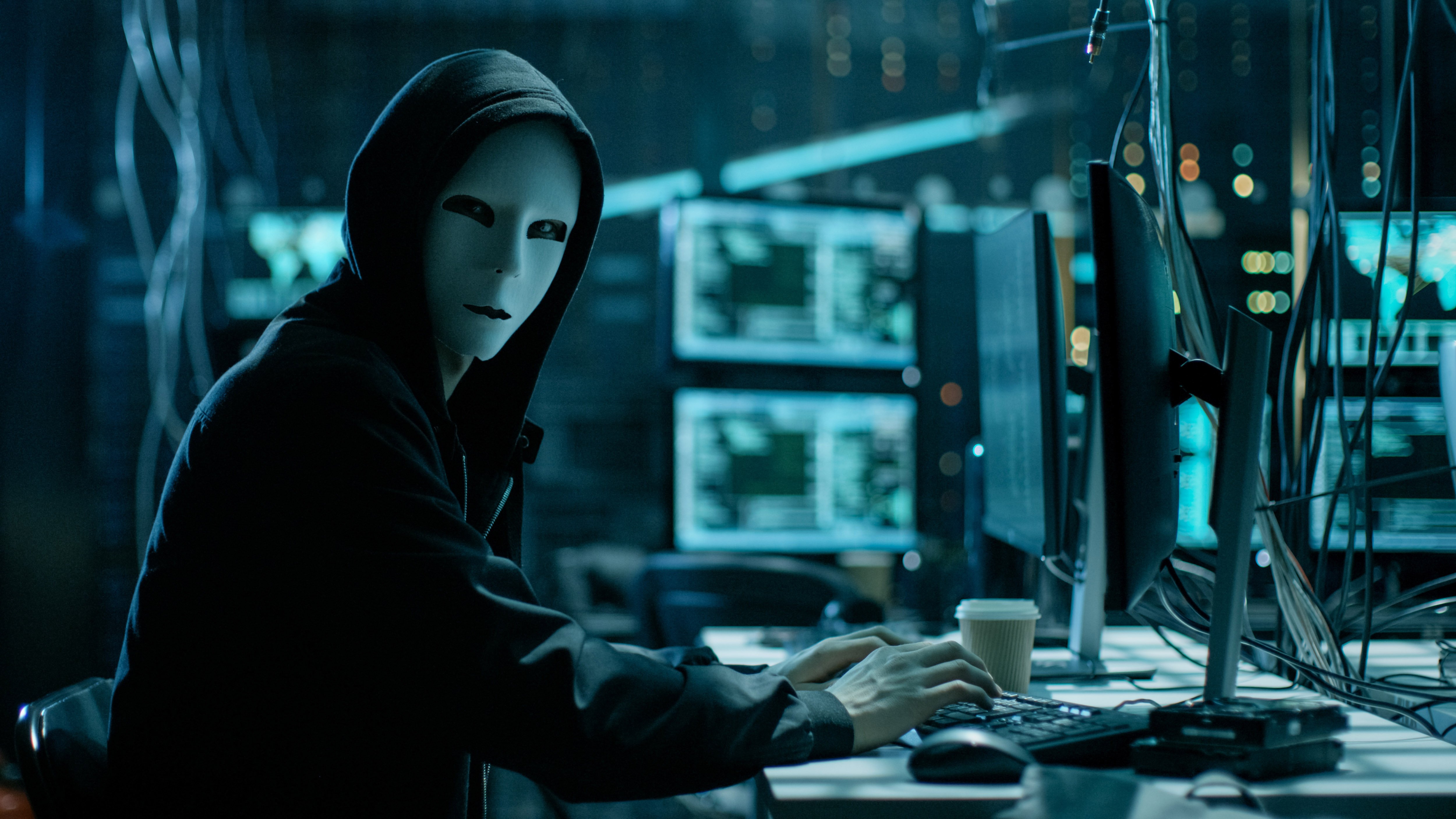
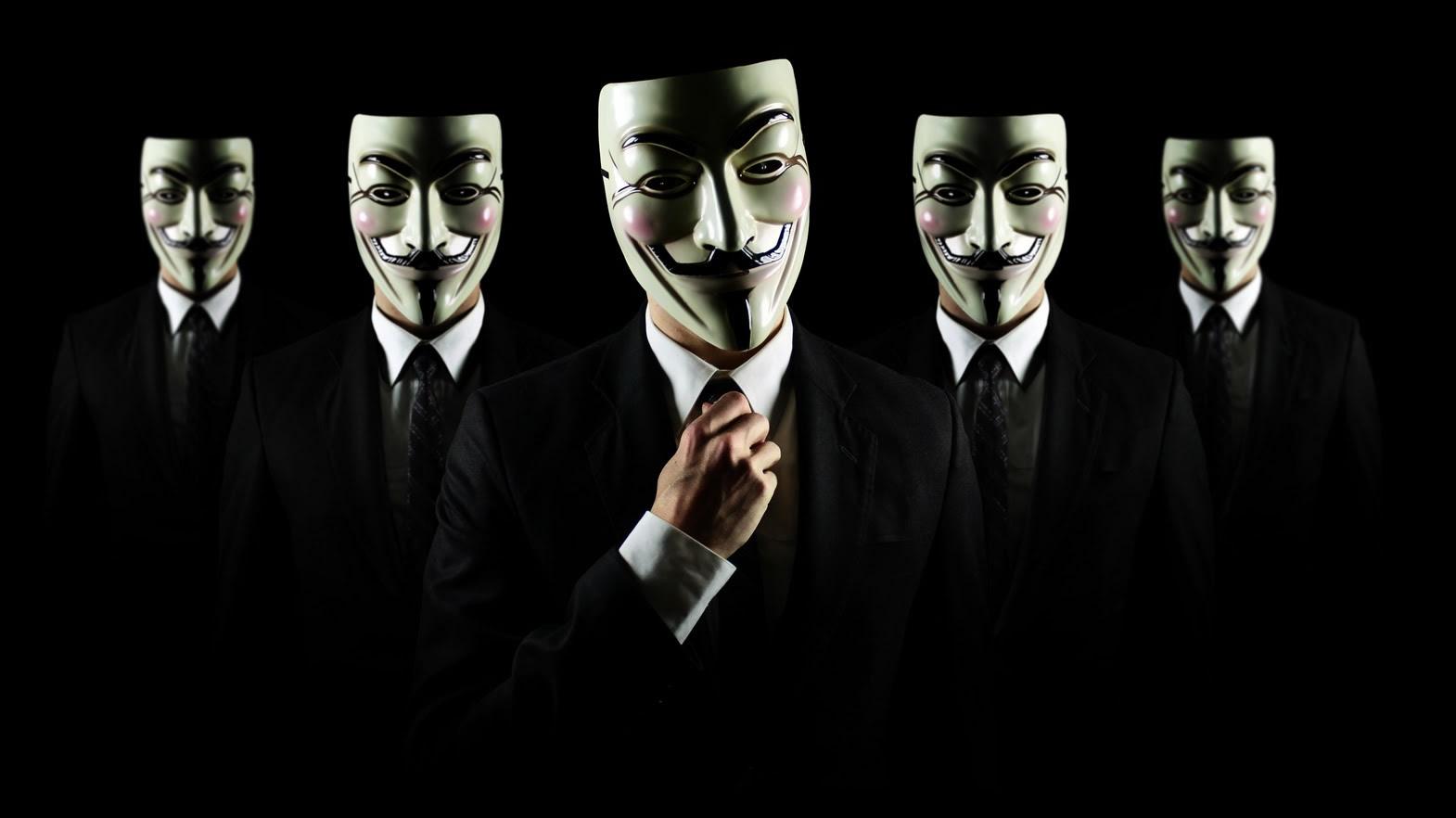

What is Shodan?
- A tool for searching devices connected to the internet.

- Common use:
- Network Security
- Market Research
- Cyber Risk
- Scanning IoT Devices
- Tracking Ransomware
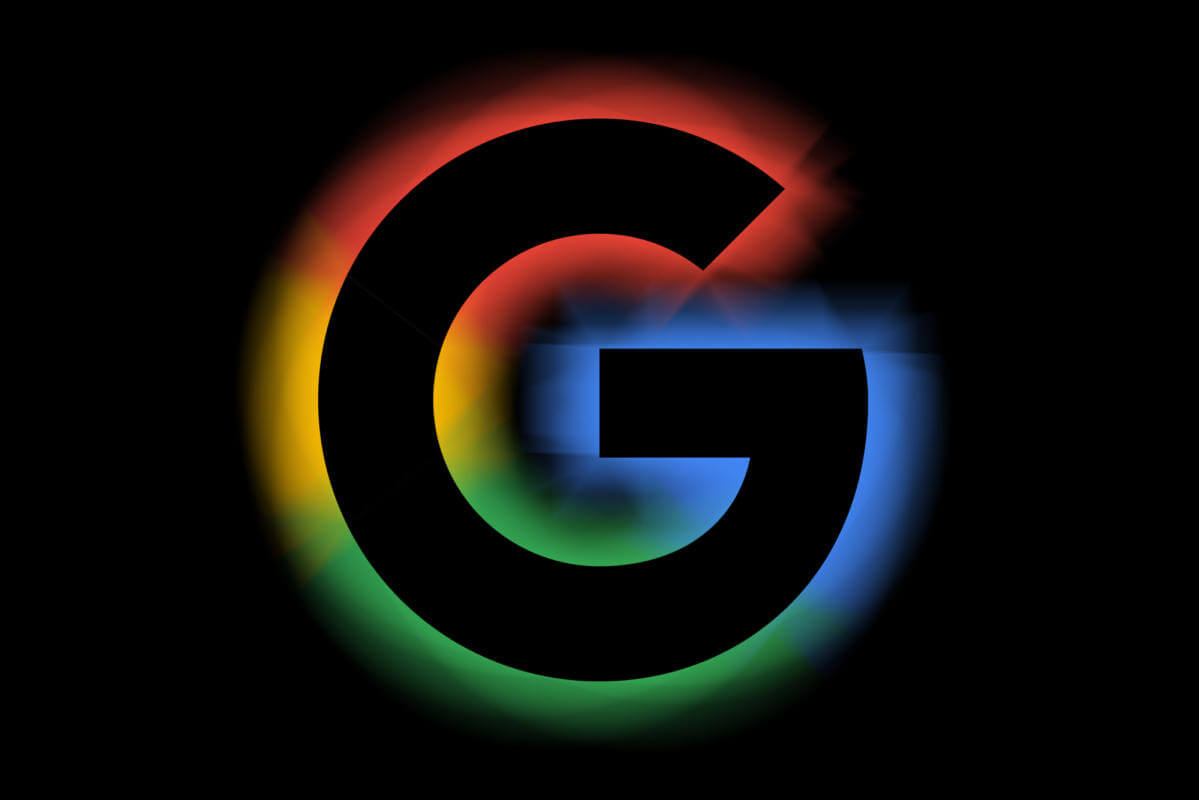
Dark Google for Hackers
How to use Shodan?
Shodan's Interfaces
- CLI Tool
- Website
- REST API
- Language Wrappers (libraries)
- Plugins
We always use Shodan in website or command line This time, I want to teach how to use the Shodan library when programming
Step 1. Installation
if os == windows:
$ pip install shodan
elif (os == macos) of (os == linux):
$ sudo pip3 install shodan
Step 2. Initialize
The API key should always be initialized:


import shodan
SHODAN_API_KEY = "(API KEY Here)"
api = shodan.Shodan(SHODAN_API_KEY)
Step 3. Basic Search
# Initialize the shodan API Key
try:
# Search Shodan
results = api.search('apache')
# Show results
print("Results found: {}".format(results['total']))
for result in results['matches']:
print("IP: {}".format(result['ip_str']))
print(result['data'])
print()
except shodan.APIError as e:
print("Error: {}".format(e))
Step 4. Available ports of a host
# Initialize the shodan API Key
try:
# Lookup the host
host = api.host('140.136.152.180')
# Print general info
print("""
IP: {}
Organization: {}
Operating System:{}
""".format(host['ip_str'], host.get('org', 'n/a'), host.get('os', 'n/a')))
# Print all banners
for item in host['data']:
print("""
Port: {}
Banner: {}
""".format(item['port'], item['data']))
except shodan.APIError as e:
print("Error: {}".format(e))
Step 5. Displaying stats
# Initialize the shodan API Key
# The list of properties we want summary information on
FACETS = [
('org', 3),
'domain',
'port',
'asn',
('country', 10),
]
FACET_TITLES = {
'org': 'Top 3 Organizations',
'domain': 'Top 5 Domains',
'port': 'Top 5 Ports',
'asn': 'Top 5 Autonomous Systems',
'country': 'Top 10 Countries',
}
try:
query = 'apache 2.4'
# Count results
result = api.count(query, facets = FACETS)
print("Shodan Summary Information")
print("Query: %s" % query)
print("Total Results: %s\n" % result["total"])
# Print the summary info from the facets
for facet in result['facets']:
print(FACET_TITLES[facet])
for term in result['facets'][facet]:
print("%s: %s" % (term['value'], term['count']))
print()
except shodan.APIError as e:
print("Error: {}".format(e))
Demo
-
Get a list of subdomains for a domain
-
FTP server with anonymous authentication enabled
-
VNC servers without authentication
$ shodan domain fju.edu.tw
$ shodan search '230 login successful port:21'
$ shodan search '"authentication disabled" port:5900,5901'
Thanks for listening.

References
- Alexandre ZANNI. (2020, April). Shodan Pentesting Guide [Web blog message], URL: https://community.turgensec.com/shodan-pentesting-guide/
- Shodan. (2019). Shodan Help Center [Web blog message], URL: https://help.shodan.io/
- Michael Schearer. (2018), SHODAN for Penetration Tester , URL: https://www.defcon.org/images/defcon-18/dc-18-presentations/Schearer/DEFCON-18-Schearer-SHODAN.pdf
Float on Shodan Pentesting
By Иo1lz
Float on Shodan Pentesting
This is the slide for SIRLA "This 15 Speech". Contents of this slide is VERY EASY for an experienced pentester or programmer. Don't expect too much...
- 174