Python Basics
Present by NIghTcAt
OUTLINE
- Python Installation
- Introduction to Python
- Basic Construction
- LAB
Python Installation
- Download python
- Check "Add Python 3.7 to PATH"
- Click "Install Now"
- Download "get-pip.py"
- While finish installation of python 3.7, open the command line
- change the directory to where the file is, then key in the command "python get-pip.py"
Introduction to

- High-level programming language
- Design Philosophy:
- code readability
- significant whitespace
- simple and clear
- large and comprehensive standard library
- open source
Basic Construction
First of all,
how to input and output testcases?
Please try the code below:
print("Hello, World!")
name = input("Please input your name: ")
print("Hello, " + name)
name = input("Please input your name: ")
id = input("Please input your student ID: ")
print("Welcome, ID " + id + ", Mr./Ms. " + name)
Operator
- + => add
- - => subtract
- * => multiplication
- / => divide (float)
- // => divide (integer)
- ** => power
- % => remainder
Variable Name
- Must start with a letter or an underscore
- The remainder of variable name may consist a number, a letter, or an underscore
- Case sensitive
- Avoid the remainder word
import keyword
keyword.kwlist
* Python3 import unicode
Type Conversion
- Integer => int()
- float => float()
- string => str()
- boolean => boolean()
Condition
if [condition]:
[statement]
elif [condition]:
[statement]
else:
[statement]
Loop - for
Loop - while
for [variable] in [array]:
[statement]
#------------------------
for [variable] in range([3 parameters]):
[statement]
# parameters of range:
# 1. starting value
# 2. ending value
# 3. increment
while [condition]:
[statement]
LAB
LAB - 01
| Default Input
LAB - 02
| String Input
print(input() * 2)
this_is_the_string = "I am"
print(this_is_the_string + " nobody.")
print(this_is_the_string * 3)
LAB - 03
| Datatype Concersion
type_string = input("Please enter your favorite number: ")
print(type_string * 2)
type_integer = int(input("Please enter your favorite number again: "))
print(type_integer * 2)
type_float = float(input("Please enter your favorite number again and again: "))
print(type_float * 2)
LAB - 04
| Game Design ~ 勇者の始まる
1. 自我介紹:姓名、年齡、性別
2. 能力:血量、攻擊力、防禦力、幸運值、擊中值
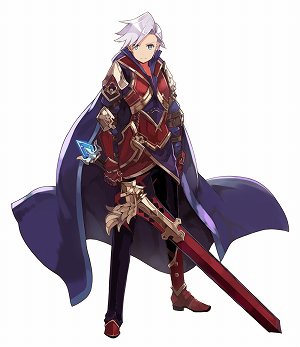
hero_name = input("君の名は? ")
hero_age = input("あなたの年齢は? ")
hero_gender = input("あなたは男ですか?女ですか? ")
print("ようこそこの世界え、勇者 " + hero_name + "。")
print("これからはあなたの最初の状態:")
print("名前: " + hero_name)
print("年齢: " + hero_age)
print("性別: " + hero_gender)
hero_HP = int(input("HP: "))
hero_attackValue = int(input("攻撃力: "))
hero_defenseValue = int(input("防御力: "))
hero_luckValue = int(input("ラック: "))
hero_hitValue = float(input("ストライク値: "))
print("\n")
LAB - 05
| Game Design ~ バケモノのデータ
1. 魔物資料:血量、閃躲值、攻擊力、防禦力

print("これからはモンスターの情報")
monster_HP = int(input("HP: "))
monster_attackValue = int(input("攻撃力: "))
monster_defenseValue = int(input("防御力: "))
monster_dodgeValue = float(input("ダッジ値: "))
print("\n")
LAB - 06
| Game Design ~ 戦いの瞬間(1)
1. 計算勇者成功攻擊怪獸的機率
hint:
1. 機率 = (勇者擊中值 - 魔物閃避值) / 1000 * 100(%)
hero_hitRate = (hero_hitValue - monster_dodgeValue) / 1000 * 100
LAB - 06
| Game Design ~ 戦いの瞬間(2)
2. 使用亂數作為怪獸成功閃躲的機率,並輸出是否攻擊成功
hint:
1. 引入random函式庫
2. random.randint(1, 100)
import random
hero_hitRate = (hero_hitValue - monster_dodgeValue) /1000 * 100
monster_dodgeRate = random.randint(1, 100)
if hero_hitRate > monster_dodgeRate:
print("HIT!")
else:
print("MISS~")
LAB - 06
| Game Design ~ 戦いの瞬間(3)
3.使用亂數取怪獸的幸運值,倘若小於勇者的幸運值則輸出暴擊(5倍攻擊)
hint:
1. random.randint(1, 1000)
import random
hero_criticalAttackerValue = hero_attackValue * 5
hero_hitRate = (hero_hitValue - monster_dodgeValue) /1000 * 100
monster_dodgeRate = random.randint(1, 100)
monster_luckValue = random.randint(1, 1000)
if hero_hitRate > monster_dodgeRate and hero_luckValue > monster_luckValue:
print("CRITICAL~~!")
elif hero_hitRate > monster_dodgeRate:
print("HIT!")
else:
print("MISS~")
LAB - 07
| Game Design ~ 世界のために、勇者を、戦おう!
1. 利用迴圈及判斷式,如果擊中怪獸則減少怪獸HP,若沒有擊中則減少勇者的HP,直到其中一方HP歸零倒地
hint:
1. while(魔物或勇者血量未歸零) => 進入迴圈
2. 若擊中,魔物血量 - (勇者攻擊力 - 魔物防禦力);
若未擊中,勇者血量 - (魔物攻擊力 - 勇者防禦力)
while hero_HP > 0 or monster_HP > 0:
if(Critical Hit):
monster_HP -= (hero_criticalAttackValue - monster_defenseValue)
elif(Hit):
monster_HP -= (hero_attackValue - monster_defenseValue)
else:
hero_HP -= (monster_attackValue - hero_defenseValue)
import random
# 勇者の情報
hero_name = input("君の名は? ")
hero_age = input("あなたの年齢は? ")
hero_gender = input("あなたは男ですか?女ですか? ")
print("ようこそこの世界え、勇者 " + hero_name + "。")
print("これからはあなたの最初の状態:")
print("名前: " + hero_name)
print("年齢: " + hero_age)
print("性別: " + hero_gender)
hero_HP = int(input("HP: "))
hero_attackValue = int(input("攻撃力: "))
hero_defenseValue = int(input("防御力: "))
hero_luckValue = int(input("ラック: "))
hero_hitValue = float(input("ストライク値: "))
print("\n")
#---------------------------------------------------
# モンスターの情報
print("これからはモンスターの情報")
monster_HP = int(input("HP: "))
monster_attackValue = int(input("攻撃力: "))
monster_defenseValue = int(input("防御力: "))
monster_dodgeValue = float(input("ダッジ値: "))
print("\n")
#---------------------------------------------------
# 戦う設定
hero_criticalAttackValue = hero_attackValue * 5
hero_hitRate = (hero_hitValue - monster_dodgeValue) /1000 * 100
hitCombo = 0
cnt = 0
while hero_HP > 0 and monster_HP > 0:
monster_dodgeRate = random.randint(1, 100)
monster_luckValue = random.randint(1, 1000)
if hero_hitRate > monster_dodgeRate and hero_luckValue > monster_luckValue:
hitCombo += 1
print("Combo " + str(hitCombo))
print("CRITICAL~~!\n")
if hero_criticalAttackValue >= monster_defenseValue:
monster_HP -= (hero_criticalAttackValue - monster_defenseValue)
elif hero_hitRate > monster_dodgeRate:
hitCombo += 1
print("Combo " + str(hitCombo))
print("HIT!")
if hero_attackValue >= monster_defenseValue:
monster_HP -= (hero_attackValue - monster_defenseValue)
else:
hitCombo = 0
print("MISS~\n")
if monster_attackValue > hero_defenseValue:
hero_HP -= (monster_attackValue - hero_defenseValue)
if hero_HP <= 0:
print("これは")
print("この世界の終わり")
print("全ての人間は死んだ")
print("残るのは、ただ勇者の体、雨に濡れる")
elif monster_HP <= 0:
print("歓呼しようよ!")
print("モンスターはもう倒すてきました!")
print("これは新しいの世界!")
print("これは新しいの時代!")
Python Basics
By Иo1lz
Python Basics
- 141