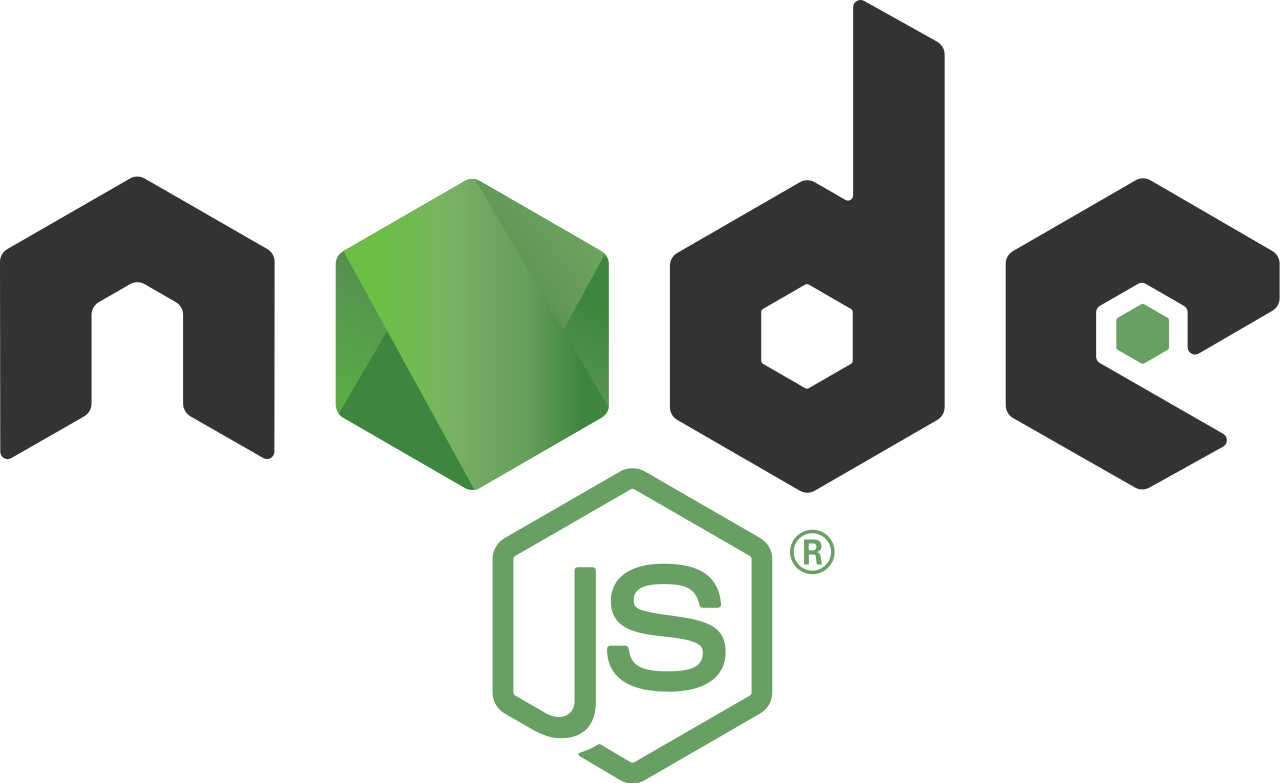
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine.
How to install
With installer
brew install node
How to use
// index.js
console.log('Hello world!')
$ node index.js
-> Hello world!
$ node --help
In order to get list of available options and basic usage execute:
What Node.js can do?
- Web servers
- Development tooling
- Desktop apps
- Command line tools
- etc
Host environment
A host environment provides platform-specific objects and functions additional to the language core. Web browsers give a means to control web pages. Node.JS provides server-side features, and so on.
The most common host environment for JavaScript is web browsers. Web browsers typically use the public API to create host objects responsible for reflecting the DOM into JavaScript.
For example:
- window
- document
- addEventListener
- removeEventListener
- history
- etc.
Node.js host environment
- global
- require
- module
- __filename
- __dirname
- process
- setTimeout
- setInterval
- setImmediate
- etc.
require(id)
// Importing a local module:
const myLocalModule = require('./path/myLocalModule');
// Importing a JSON file:
const jsonData = require('./path/filename.json');
// Importing a module from node_modules or Node.js built-in module:
const crypto = require('crypto');
Used to import modules, JSON, and local files. Modules can be imported from node_modules. Local modules and JSON files can be imported using a relative path (e.g. ./, ./foo, ./bar/baz, ../foo).
module.exports
// module B
const config = {
apiKey: '111-222-333-444'
}
module.exports = config
// module A
const config = require('./config')
console.log(config.apiKey) // 111-222-333-444
Built-in modules
Internal modules overview (fs, http)
The Node.js file system module allows you to work with the file system on your computer.
const fs = require('fs');
const path = require('path');
fs.readFile(__filename, 'utf-8', (err, file) => {});
const file = fs.readFileSync(__filename, 'utf-8');
fs.writeFile(path.join(__dirname, './1.txt'), 'Hello\nWorld\n!', (err) => {});
fs.writeFileSync(path.join(__dirname, './1.txt'), 'Hello\nWorld\n!');
fs.readdir(__dirname, (err, dir) => {});
fs.readdirSync(__dirname);
Node.js has a built-in module called HTTP, which allows Node.js to transfer data over the Hyper Text Transfer Protocol (HTTP).
const http = require('http');
const server = http.createServer((request, response) => {
response.end('Hello world!');
});
server.listen(8080);
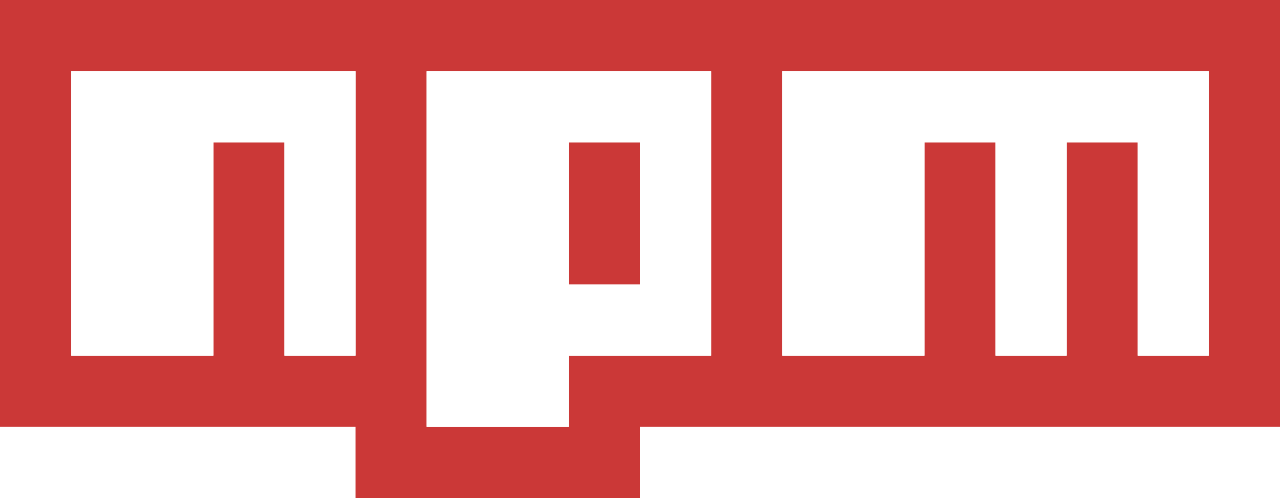
Npm - node package manager
Npm is installed alongside with Node.js
$ npm install moduleName
But before we install any module we should create a package.json file
$ npm init
This can be done manually or via
{
"name": "test",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Nodejs and npm basics
By Nikita Rudy
Nodejs and npm basics
- 600