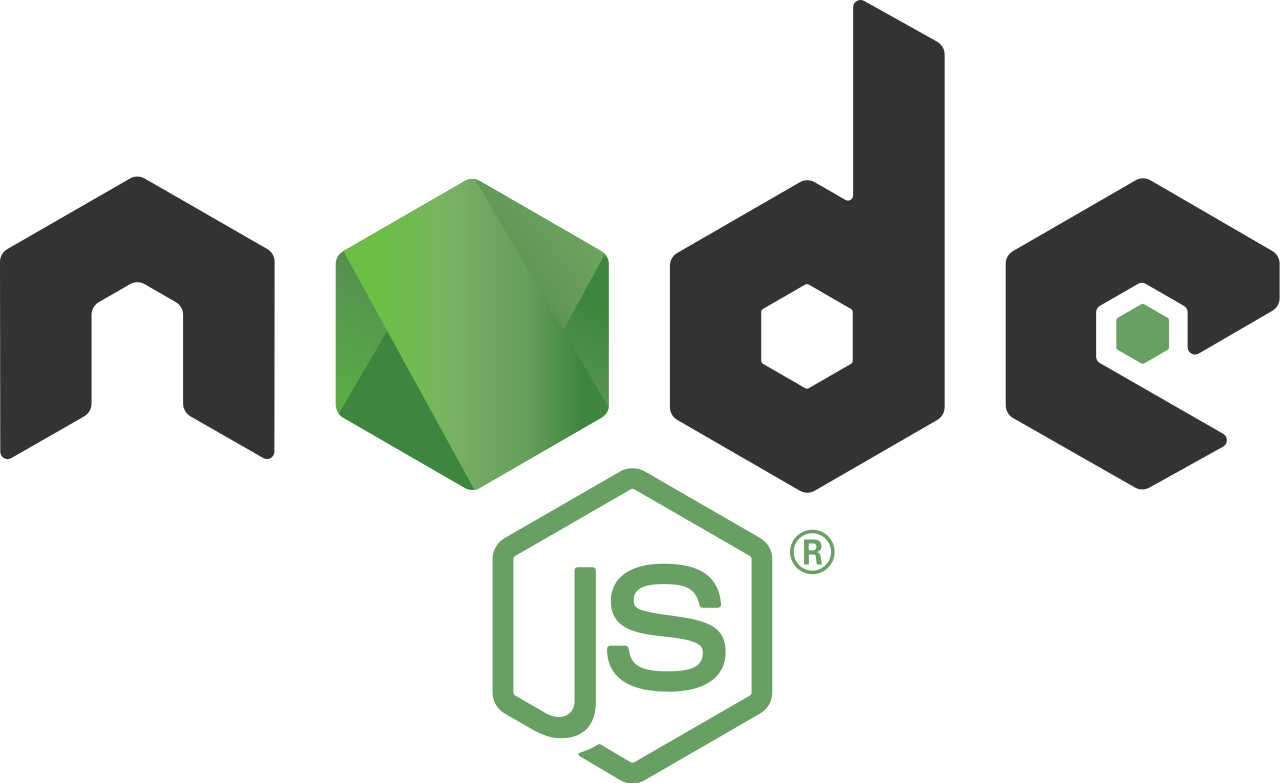
Networking in Node.js
The Net module provides a way of creating TCP or IPC servers and TCP or IPC clients.
TCP (Transmission Control Protocol) - is a standard that defines how to establish and maintain a network conversation via which application programs can exchange data.
IPC (Inter-process communication) is a standard that defines how to establish and maintain a process communication.
const net = require('net');
// server code
const server = net.createServer();
server.on('connection', (socket) => {
socket.on('data', (data) => {
console.log('message from client: ', data.toString());
});
socket.write('hello client!');
})
server.listen(8080, () => {
console.log(server.address());
})
// client code
const client = net.createConnection(8080, () => {
client.write('hello server!');
})
client.on('data', (data) => {
console.log('server responded with: ', data.toString());
})
Node.js has a built-in module called HTTP, which allows Node.js to transfer data over the Hyper Text Transfer Protocol (HTTP).
const http = require('http');
const stream = require('stream');
const server = http.createServer();
server.on('request', (request, response) => {
console.log(request instanceof stream.Readable);
console.log(response instanceof stream.Writable);
})
server.listen(8080);
Nodejs networking
By Nikita Rudy
Nodejs networking
- 202