LINQ
(Language Integrated Query)
TIMELINE
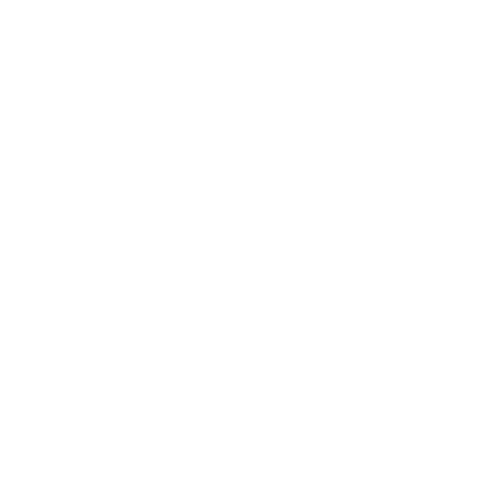
TIMELINE
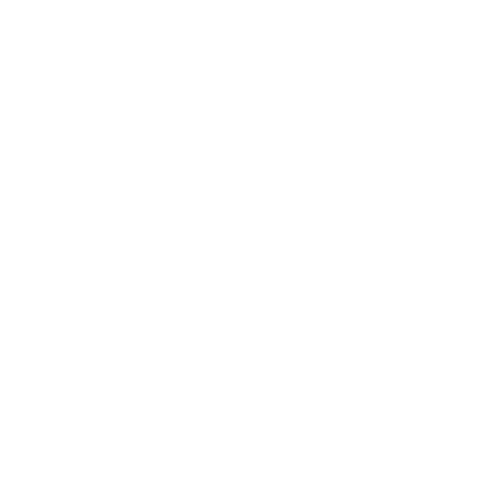
Contents
-
Introduction to LINQ
-
Purpose of LINQ
-
Advantages of LINQ
-
Disadvantages of LINQ
-
LINQ Syntax
- Query Syntax
- Method Syntax
LINQ ?
A set of technologies based on the integration of query capabilities directly into the C# language
WHY LINQ ?
LINQ is simpler, tidier, and higher-level than SQL.
When it comes to querying databases, In most cases, LINQ is more productive querying language than SQL.
LINQ Architecture
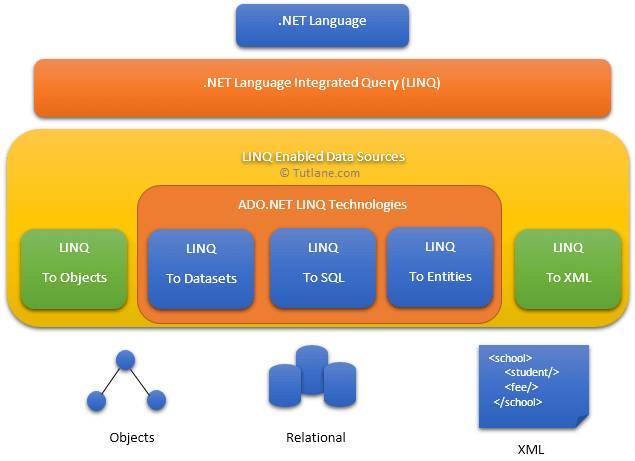
ADVANTAGES of LINQ
-
Full type checking at compile time
-
Has IntelliSense (can avoid silly errors)
-
Query can be reused
-
Can debug using .NET debugger
-
Suport filtering, sorting, ordering, grouping with much less effort.
DISADVANTAGES of LINQ
-
Writing complex queries is LINQ is a bit tedious as compared to SQL
-
The performance is degraded - if the query is not written properly
-
Need to build the project and deploy the DDL every time has a changes.
LINQ Execution

Syntax of LINQ
-
Query Syntax
-
Method Syntax
var numbers = Enumerable.Range(1, 100); //1,2,...,100
//query syntax:
var query = from n in numbers
where n % 3 == 0
select n * 2;
var numbers = Enumerable.Range(1, 100);
//method syntax
var method = numbers
.Where(n => n % 3 == 0)
.Select(n => n * 2);
LINQ Query Syntax
from object in datasource
where condition
select object
Initialization
Condition
Selection
// Select all EBs.
var ebs = (from e in _dataContext.EBs
select e).ToList();
LINQ Query Syntax
from object in datasource
where condition
select object
Initialization
Condition
Selection
// Select all approved form submissions.
var formSubmissions = (from s in _dataContext.FormSubmissions
where s.status == FormSubmission.STATUS_APPROVED
select s).ToList();
LINQ Query Syntax
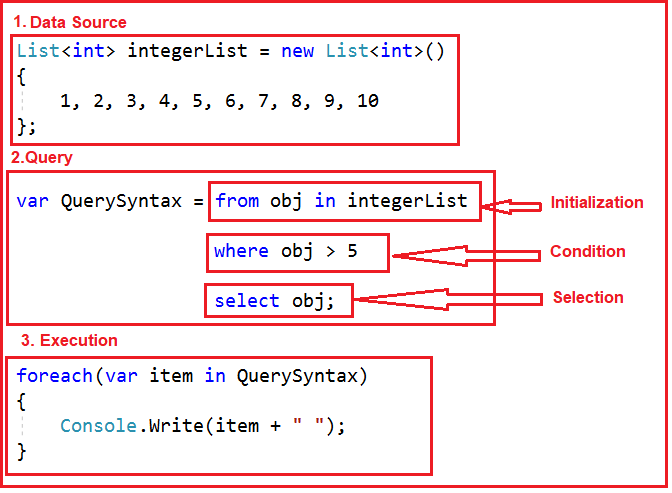
LINQ Query Syntax
// Load FormEB record.
var formEB = await (from r in _dataContext.FormEBs
where r.listing_job_id == listingJobId
&& r.fe_email == email
&& r.eb_id == ebId
select r).AsNoTracking().FirstAsync();
OPERATOR | DESCRIPTION |
---|---|
where | Returns values from the datasource based on a predicate function. |
AsNoTeacking() | returns a new query and the returned entities will not be cached by the context (DbContext or Object Context) |
FirstAsync() | Asynchronously returns the first element of the query that satisfies a specified condition. |
LINQ Method Syntax
DataSource
.ConditionMethod()
.SelectionMethod()
Initialization
Condition
Selection
// Get all photos for this EE.
var localEePhotos = localJobPhotos
.Where(x => x.uid == localEE.uid)
.ToList();
LINQ Method Syntax
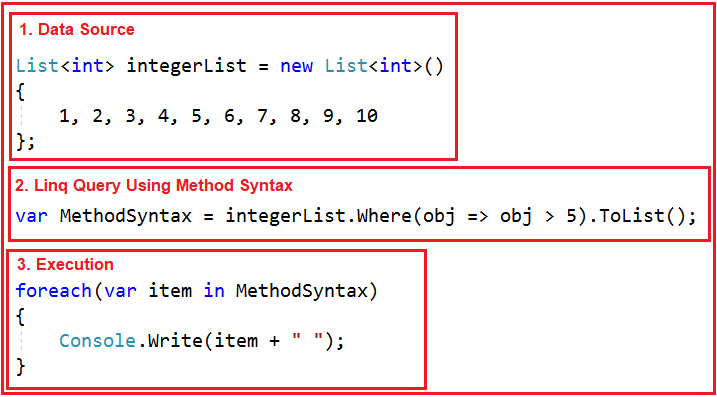
LINQ Method Syntax
// Get all photos for this EE.
var localEePhotos = localJobPhotos
.Where(x => x.uid == localEE.uid)
.ToList();
OPERATOR | DESCRIPTION |
---|---|
.ToList() | Takes the elements from the given source and it returns a new List. The input would be converted to type List. |
References
LINQ
By nur amirah
LINQ
- 298