Python
講者:邱妍瑛
在開始之前
網址加上 /live 就會跟著我一起
想直接看就去掉 /live
然後 所有程式碼 請親自打過一遍
看到粉紅色就代表輪到你了
大綱
- Python 簡介
- 環境架設
- 基本資料型態
- 變數
- 基礎語法
- 串列
- 流程控制
- 迴圈
outline
Python 是什麼?
What is Python ?
程式?
那是什麼?
能吃嗎?
簡單來說就是一串可以交由電腦執行的指令



作曲家
樂譜
樂團
作曲
演奏
程式設計師
程式
電腦
撰寫
執行
程式語言?
那又是什麼?
這次我知道不能吃了

樂譜


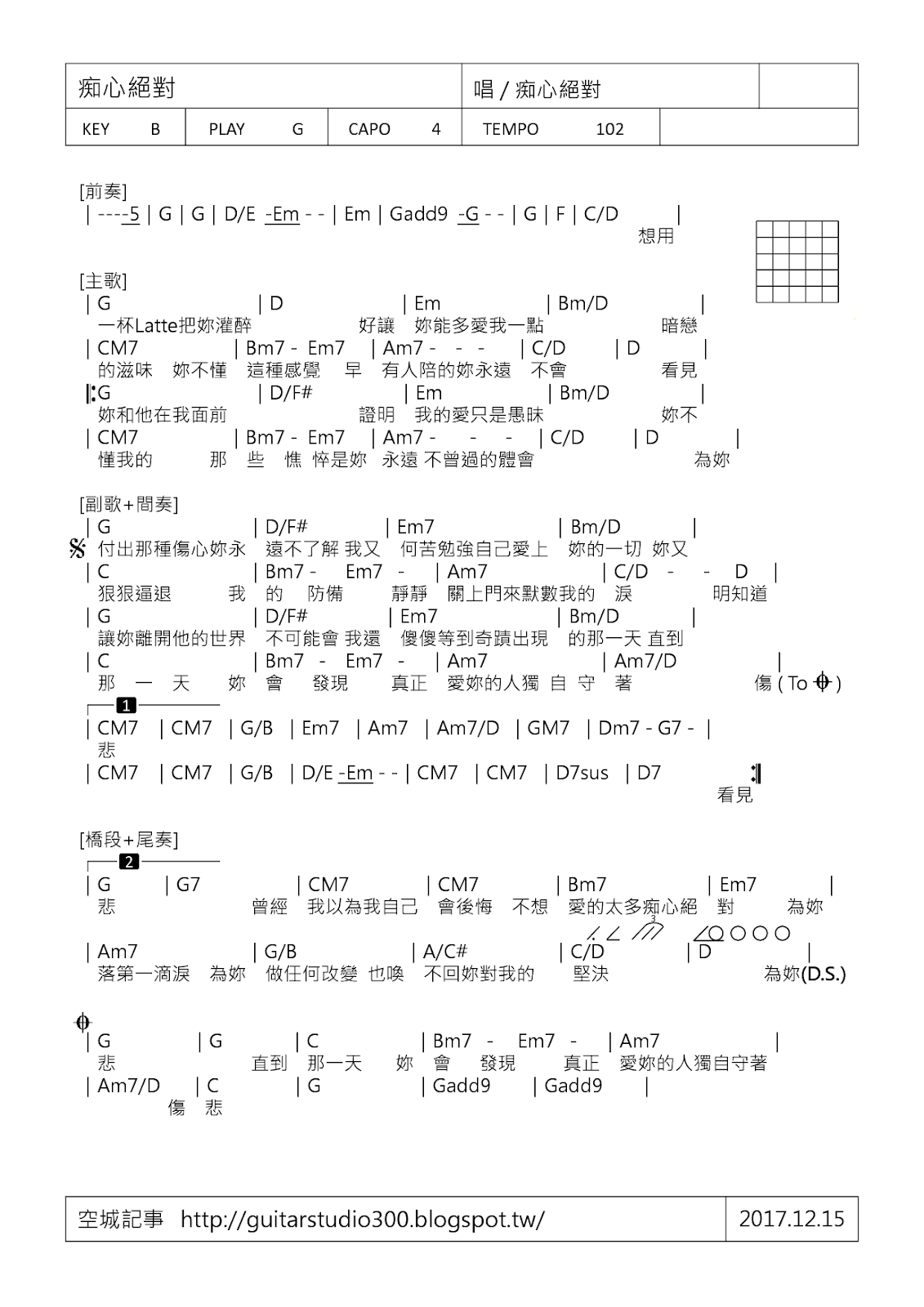
相同的,程式語言也有很多種

為什麼要學寫程式?
因為 有興趣
因為 喜歡寫完程式帶來的成就感
因為 喜歡解決問題
最重要的是
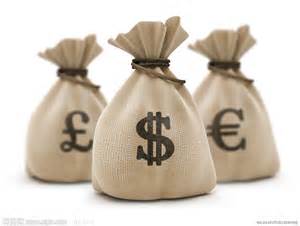
( =$ω$=)( =$ω$=)( =$ω$=)
選 Python 的原因
- 簡單易學、簡潔易讀
- 用途廣泛且實用
- 聊天機器人
- 物聯網
- 數據分析
- 網站開發
- 遊戲製作
- ...
- 活躍的社群
- 開發者能夠用更少的代碼表達想法

public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello! World!");
}
}
#include <iostream>
using namespace std;
int main() {
cout << "Hello! World!";
return 0;
}
print('Hello World')



環境架設
Environment
安裝 Python
▶ Step 1. 前往官網 ( https://www.python.org/ )
▶ Step 2. 按下 Downloads

安裝 Python

▶ Step 3. 按下黃色 Download
安裝 Python
▶ Step 4. 點擊安裝執行檔 python3.7.4.exe
▶ Step 5. 點擊後出現安裝視窗
▶ Step 6. 勾選Add python 3.7 to Path(加入環境變數)
▶ Step 7. 點擊安裝


▶ Step 1. 開啟 IDLE
▶ Step 2. 新增檔案 File ➔ New File

然後就可以開始打程式啦~
Python 起手式
print('Hello World')

存檔

按下 control + S
或按 File ➔ Save
存檔

找到 .py
或直接打 .py
執行
按下 F5
或是按下 Run ➔ Run Module
會出現像上圖這樣的畫面
如果出現的話,就表示你成功踏出第一步了!

變數
Variable
變數
在 Python 中,變數像是標籤一般,
將各種型態的資料貼上標籤,方便之後使用。

變數
資料
名稱

變數
另一筆資料
另一個名稱
使用規則
利用 = 將資料放進變數裡
= 代表 assign (指派),而不是數學上的等於
- 使用 A-Z, 0-9, 和 _ 底線命名
- Python 會辨別大小寫
- 不能使用 Python 的保留字
- 開頭不能為數字

變數實例
例如我們設這個變數叫做 pet,
內容則是一個字串 'Kitty'。
pet = 'Kitty'

變數實例


Pet
'Kitty'


使用者
Python
變數實例
pet = 'Kitty'
pet = '小黑'
既然是「變」數,代表我們可以改變它的內容:
一個標籤只能貼在一個內容上,所以 pet 就變成 '小黑' 了

小黑
試試看吧!
pet = 'Kitty'
pet = '小黑'
print(pet)
變數實例


Pet
'小黑'


使用者
Python
基本資料型態
Basic Data Type
資料型態 | 名稱 | 例子 |
---|---|---|
int | 整數 | 1, 2, -1, -2 |
float | 小數 | 0.1, 0.2, -0.1, -0.2 |
str | 字串 | 'abc', "abc" |
bool | 布林值 | True, False |
complex | 複數 | 1 + 2j, 3 + 4j |
str
要被單引號或雙引號包起來
以下這些都是 str 型態:
'abc', "abc"
'123', "123"
'0.1', "0.1"
'True', "True"
bool
第一個字母要大寫
只能表示對(True)或錯(False)
通常用於條件判斷

資料型態簡介
資料型態決定了
資料將佔用的記憶體空間大小、
以及程式該如何處理資料。電腦中,所有資料
int | 12 |
float | 16 |
str | 21 |
bool | 12 |
資料型態 | 佔用記憶體大小 |
---|
type(參數):查看各種型別,如:int, str…
print(type(123))
print(type(0.123))
print(type('123'))
資料型態太多分不清怎麼辦?
<class 'int'>
<class 'float'>
<class 'str'>
等差公式: an = a1 + (n-1)d
n 就像參數
什麼是函式呢?
試試看吧!
print(type(407))
print(type(0.407))
print(type('407'))
print(type(True))
print(type(1 + 2j))
<class 'int'>
<class 'float'>
<class 'str'>
<class 'bool'>
<class 'complex'>
'123'
強制型態轉換
123
int('123')
字串
整數
強制型態轉換
'123'
123
str(123)
字串
整數
強制型態轉換
試試看吧!
print(type('407'))
print(type(int('407')))
print(type(407))
print(type(str(407)))
<class 'str'>
<class 'int'>
<class 'int'>
<class 'str'>
基礎語法
Basic
註解
- 分為單行註解 跟 多行註解
- 幫助你看懂程式碼
#我是註解
'''
我是很多行註解
我是很多行註解
我是很多行註解
'''
輸入
使用 input() 進行輸入,讀進來的東西是一個 String
input() #直接輸入
input("請輸入姓名: ") #帶有提示的輸入
name = input("請輸入姓名: ") #存進變數
phone_number = int(input("請輸入電話:")) #強制轉成 int
輸入實例
將 「請輸入姓名:」
直接輸出到螢幕上
a = input('請輸入姓名: ')
使用者輸入的「Yan」
assign 到 a 這個變數中

請輸入姓名:
Yan
試試看吧!
name = input("請輸入姓名: ")
print(name)
請輸入姓名:Yan
Yan
輸出
使用 print() 進行輸出
a = 1
print("這個數字是" + a) #會出錯,因為a是int
print("這個數字是" + str(a)) #要把a轉型為str
- 加號: 每個變數資料型態都要是字串
a = 1
print('1' + str(a))
print(1+1)
若是數字的 1+1 結果會是 2,而非 11
輸出
使用 print() 進行輸出
- 逗號: 變數與變數中間會有空格,可用sep參數變更
a = 1
print("這個數字是", a)
#結果會是 「這個數字是 1」 而非 「這個數字是1」
print("這個數字是", a, sep=".")
#結果會是 這個數字是.a
輸出
a = 1
b = 2
print('show a and b: {}, {}'.format(a, b))
str.format(args1, args2, ...),
我們可以藉由這個功能來格式化輸出
show a and b: 1, 2
使用 print() 進行輸出
LAB-1
Input:姓名
Output:Hello加姓名,中間不能有空格
可使用剛剛講完的 逗號、format()
請輸入姓名:妍瑛
Hello妍瑛
LAB-1 解答
user_name = input()
print('Hello{}'.format(user_name))
user_name = input()
print('Hello', user_name, sep = '')
流程控制
flow control
if-else 簡介
if-else 和上面的例子對應
if,如果
elif,否則如果
else,不然
如果 分數大於90:
除了不會被當之外,證明你是學霸
否則如果 分數大於60:
你及格了,不會被當
不然:
你會被當
if-else 寫法
if 條件:
成立時執行的程式片段
elif 條件:
上面 if 不成立且條件成立時執行的程式片段
else :
以上都不成立時執行的程式片段
if-else
- 每個 else 都需要有 if 或 elif 在它之前
- elif 前面至少需要一個 if
- if、elif 後面都需要有判斷式,else 後則不能有
- 該行結束時要加冒號
- 執行區塊需要縮排
if 判斷式:
blabalbla...
blabalbla...
blabalbla...
else:
blabalbla...
blabalbla...
blabalbla...
if 的執行區塊
else 的執行區塊
縮排
- 用來劃分程式的執行區塊
- 可以用 Tab 或 空白鍵
- 同一區塊的縮排一定要一致
- 整份程式碼建議使用相同的標準當作縮排
while playing:
for event in pygame.event.get():
if event.type == pygame.QUIT:
playing = False
這就是縮排
算數運算子

比較/邏輯運算子


試試看吧!
temp = int(input('請輸入氣溫:'))
if temp >= 25:
if temp < 33:
print("穿短袖")
else:
print("太熱了不出門")
else:
print("穿長袖")
串列
list
串列簡介
- 由一連串資料所組成、有順序且可改變內容的序列。
- 以 [ ] 標示
- 裡面的資料以「 , 」隔開
- 串列中可以包含不同型別的元素。
- 若串列的元素相同但順序不同,表示不同串列。
[1, 'Yan', 2, 'Erica']
[2, 'Erica', 1, 'Yan']
name_list = ['Yan', 'Erica', 'oneone']
串列
建立串列有兩種方法
empty_list = []
another_empty_list = list()
將字串(str)轉換為串列
list1 = list('Y.a.n') #['Y','.','a','.','n']
list2 = 'Y.a.n'.split('.') #['Y','a','n']
"要被分割的字串" .split("以甚麼為分割點")
試試看吧!
old_list = ['a','b','c']
new_list = list('abc')
print(old_list)
print(new_list)
list1 = list('a.b.c')
list2 = 'a.b.c'.split('.')
print(list1)
print(list2)
取得 list 的元素
在 list 名稱後面使用中括號 [ ]
加上想取得元素的位置 ( index )
alphabets = ['a', 'b', 'c']
print(alphabets[1])
b
疑?第一個怎麼不是 a?
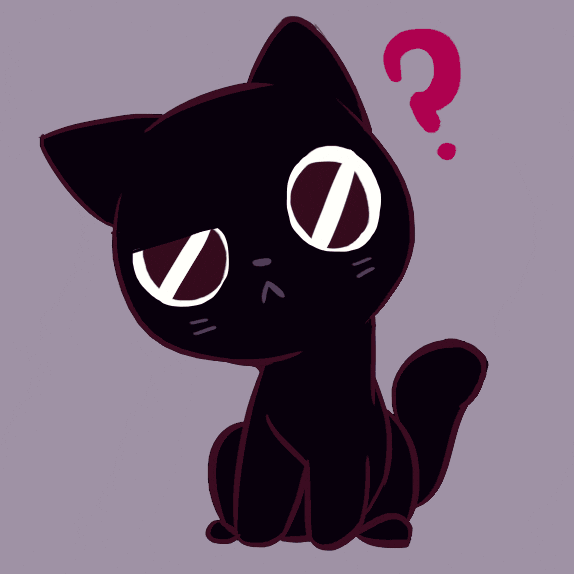
取得 list 的元素
alphabets = ['a', 'b', 'c']
print(alphabets[1])
0 | 1 | 2 |
---|
a | b | c |
---|
程式語言中的 index 是從 0 開始的
所以如果要取得第一個位置的元素,要輸入的位置值會是 0
想取得第二個元素則得輸入 1 ,以此類推
b
位置1
取得最後一個元素
alphabets = ['a', 'b', 'c']
print(alphabets[-1])
c
index 值取 -1
試試看吧!
my_list = ['a', 'b', 'c']
print(my_list[0])
print(my_list[1])
print(my_list[2])
print(my_list[-1])
a
b
c
c
以範圍取值
alphabets = ['a', 'b', 'c']
print(alphabets[0:2])
list名稱 [ 開始的index : 結束的index : 間隔 ]
取出來的元素只會包含開頭,不包含結束
後面的間隔預設為 1
a,b
0 | 1 | 2 |
---|
a | b | c |
---|
以範圍取值
alphabets = ['a', 'b', 'c']
print(alphabets[0:2])
print(alphabets[:2])
若省略開始的 index,預設是從 0 開始
所以上下兩種方式都會得到 a,b
兩者結果是相同的
若省略結束的 index,預設是取到最後一位
alphabets = ['a', 'b', 'c']
print(alphabets[0:])
以範圍取值
[0 : 2]
0 | 1 | 2 |
---|
a | b | c |
---|
總結:取前不取後
試試看吧!
letter = ['w','x','y','z']
print(letter[2])
print(letter[0:2])
print(letter[:2])
print(letter[0:])
y
['w', 'x']
['w', 'x']
['w', 'x', 'y', 'z']
以範圍取值-間隔
alphabets = ['a', 'b', 'c', 'd']
print(alphabets[0:3:2])
list名稱 [ 開始的index : 結束的index : 間隔 ]
a,c
0 | 1 | 2 | 3 |
---|
a | b | c | d |
---|
因為取前不取後
而且間隔是2
因為間隔是2
新增元素
alphabets = ['a', 'b', 'c']
alphabets.append('d')
list名稱 .append( 元素 )
['a', 'b', 'c', 'd']
['a', 'b', 'c']
append('d')
試試看吧!
letter = ['w','x','y']
letter.append('z')
print(letter)
print(letter[0:3:2])
['w', 'x', 'y', 'z']
['w', 'y']
迴圈
Loops
迴圈簡介
迴圈是用來重複執行某個動作。
Python 的迴圈有兩種
迴圈中還有三種 Control Condition (控制語句)
- break
- continue
- pass
- for
- while
迴圈用途
name_list = ['Erica', 'Amy', 'Chia', 'Sam', 'Bessy',..., 'Yan']
print(name_list[0])
print(name_list[1])
print(name_list[2])
print(name_list[3])
print(name_list[4])
...
print(name_list[50])

for i in name_list:
print(i)
for 迴圈
可放入 字串、串列
將裡面的元素一個一個拿出來
for variable in list:
your code...
縮排
冒號
「for」和「in」是 Python 的關鍵字,
兩者之間可以放置使用者自訂的變數
適用於 已知迴圈圈數
用法
- 串列 list
the_list = [1, 3, 5, 7, 9]
for i in the_list:
print(i)
1, 3, 5, 7, 9
- 字串
the_str = "Erica"
for letter in the_str:
print(letter)
E, r, i, c, a
for 迴圈 - range
使用 range物件
來控制執行次數
for variable in range():
your code...
縮排
冒號
變數
用法
-
range( start, stop, 間隔 )
- start:計數從此開始 (包含該值),預設從 0 開始
- stop:計數到此結束 (不包含該值)
- 間隔:每次改變量,預設為 1
for i in range(1, 5):
print(i)
for i in range(1, 5, 1):
print(i)
1, 2, 3, 4
range() 可以想成重複 (stop - start) 次
試試看吧!
ans = 0
for num in range(1,101):
ans = ans + num
print(ans)
從 1 加到 100
LAB-2
使用串列存放分數,
讓使用者輸入5個分數,
然後計算串列中元素的總和並輸出總分。
#input
請輸入成績 1 :
請輸入成績 2 :
請輸入成績 3 :
請輸入成績 4 :
請輸入成績 5 :
#output
總分:
偷吃步:可使用內建函式 sum() 來算總分
LAB-2 解答
grades = []
for i in range(1,6):
score = int(input("第{}個分數:".format(i)))
grades.append(score)
print("總分為",sum(grades))
While 迴圈
適用於 未知迴圈圈數
用於重複執行某個動作,
使用 條件式是否成立 來控制執行次數。
k = input()
while int(k) != 0:
k = input()
條件式
k = input()
while k != '0':
k = input()
While 迴圈

可以看你的程式碼是怎麼執行的:http://www.pythontutor.com/visualize.html#mode=edit
LAB-3
Input:學號及三個成績
Output:學號、三個成績跟平均成績
條件:要使用迴圈跟串列,格式如下
#input
請輸入學號:
請輸入成績 1 :
請輸入成績 2 :
請輸入成績 3 :
#output
學號:
成績 1 為:
成績 2 為:
成績 3 為:
平均:
小提示:一樣可使用內建函式 sum()
LAB-3 解答
number = input('請輸入學號:')
gra = []
for i in range(3):
gra.append(int(input('請輸入成績 {} :'.format(i+1))))
average_gra = sum(gra)/3
print('學號:{}'.format(number))
for i in range(3):
print('成績 {} 為:{}'.format(i+1, gra[i]))
print('平均:{}'.format(average_gra))
使用 format()
LAB-3 解答
number = input('請輸入學號:')
gra = []
for i in range(3):
gra.append(int(input('請輸入成績 {} :'.format(i+1))))
average_gra = sum(gra)/3
print('學號:',number)
for i in range(3):
print('成績',i+1,'為:',gra[i])
print('平均:',average_gra)
使用逗號

參考資料
Python 入門教學(土豆):
https://hackmd.io/GcikZaGORSqp4Xn-qHkhxg
Python(王柏凱):
https://slides.com/adkevin3307/python-3#/
Python 入門(姚韋辰):
以上是幫助我很多的筆記和 Slides 們,
也在此特別感謝 黃丰嘉 學姊提供了一系列的PPT,拯救了我
參考資料
Victor’s Python中文教程(Victor Lin):
http://ez2learn.com/index.html
Python 入門(ccClub):
https://medium.com/ccclub/tagged/python-%E5%85%A5%E9%96%80
Python 入門 (django girls):
https://djangogirlstaipei.herokuapp.com/tutorials/python/?os=windows
這些參考資料也是你學習 Python 的好地方,
這頁比較偏教程,有興趣可以看看~
最後的最後

Python
By oneone
Python
- 134