Before we start.
先來抽個下禮拜的
This 15 speech
Vue.js - Basic
2020 / 09 / 20
Lecturer:Yan
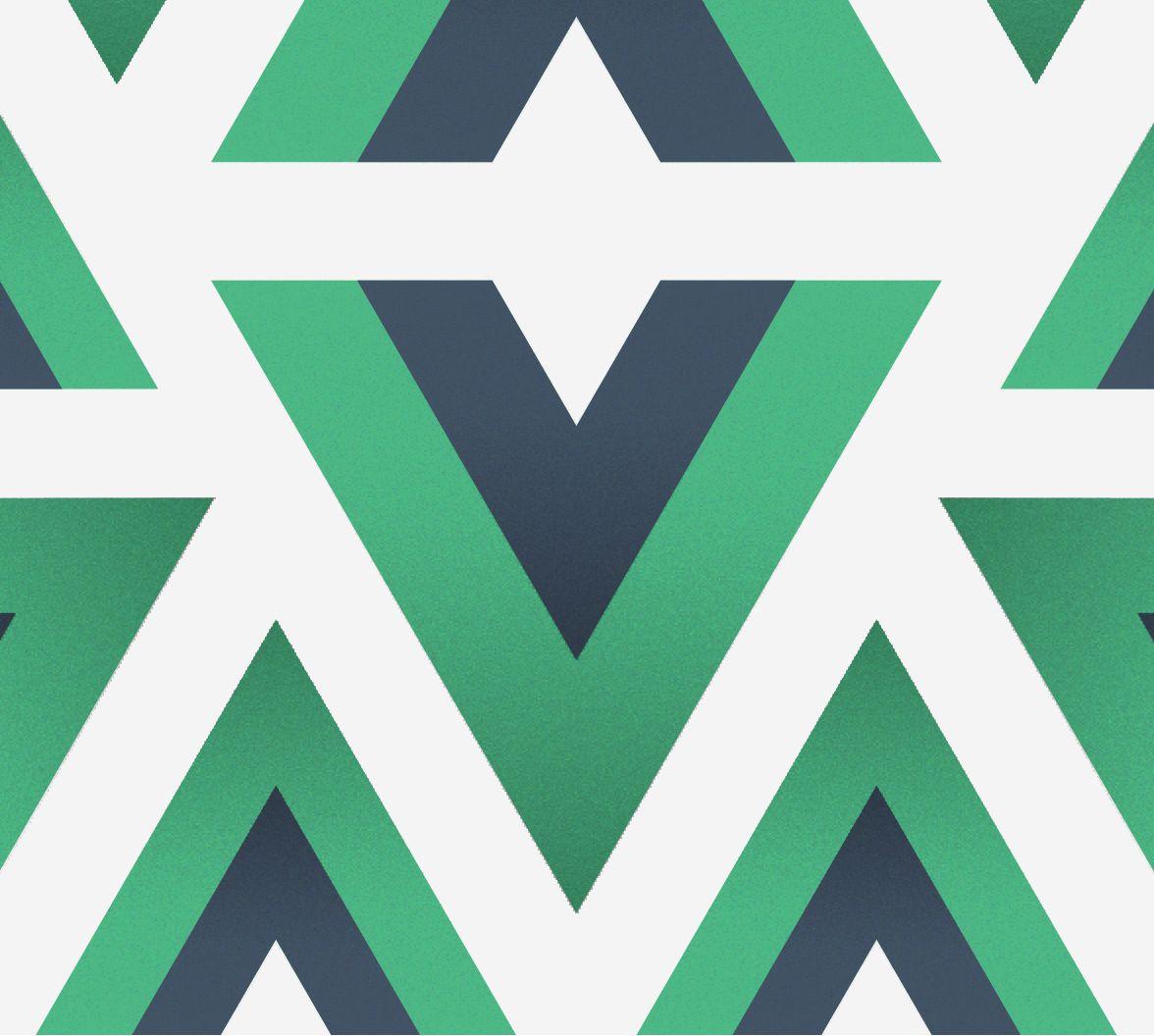
-
Introduction
-
Getting Started
-
Vue - CLI
-
Tools
-
Vue component
-
References
Outline

Introduction
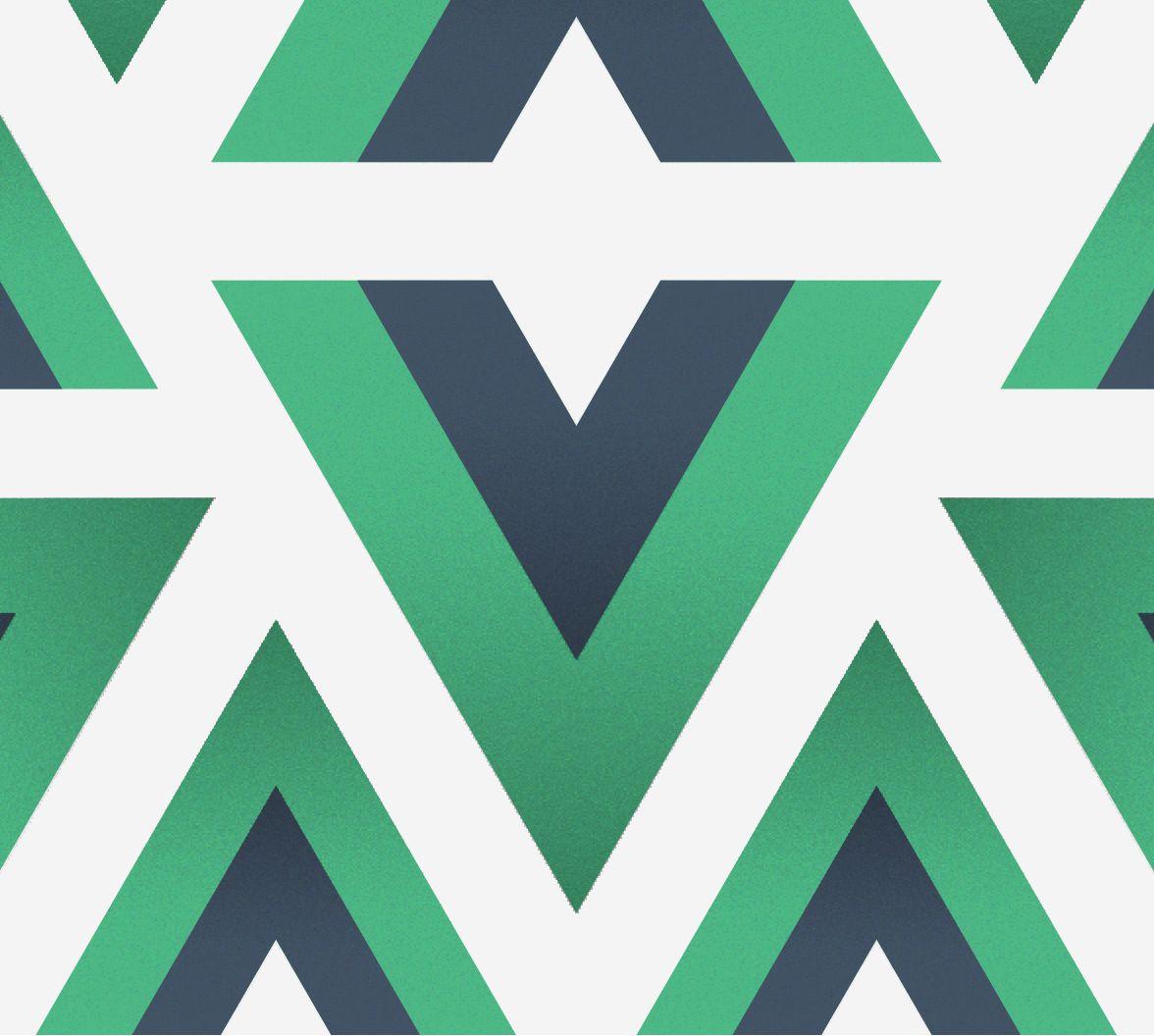
常見的網頁框架




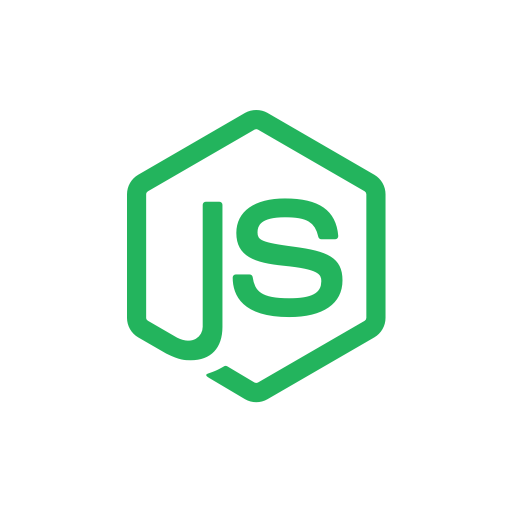

尤雨溪



The Progressive
JavaScript Framework


漸進式 JavaScript 框架
易用
靈活
高效
Vue 的特色

MVVM 架構
M
V
VM
Model
View
提供的版本

Getting Started
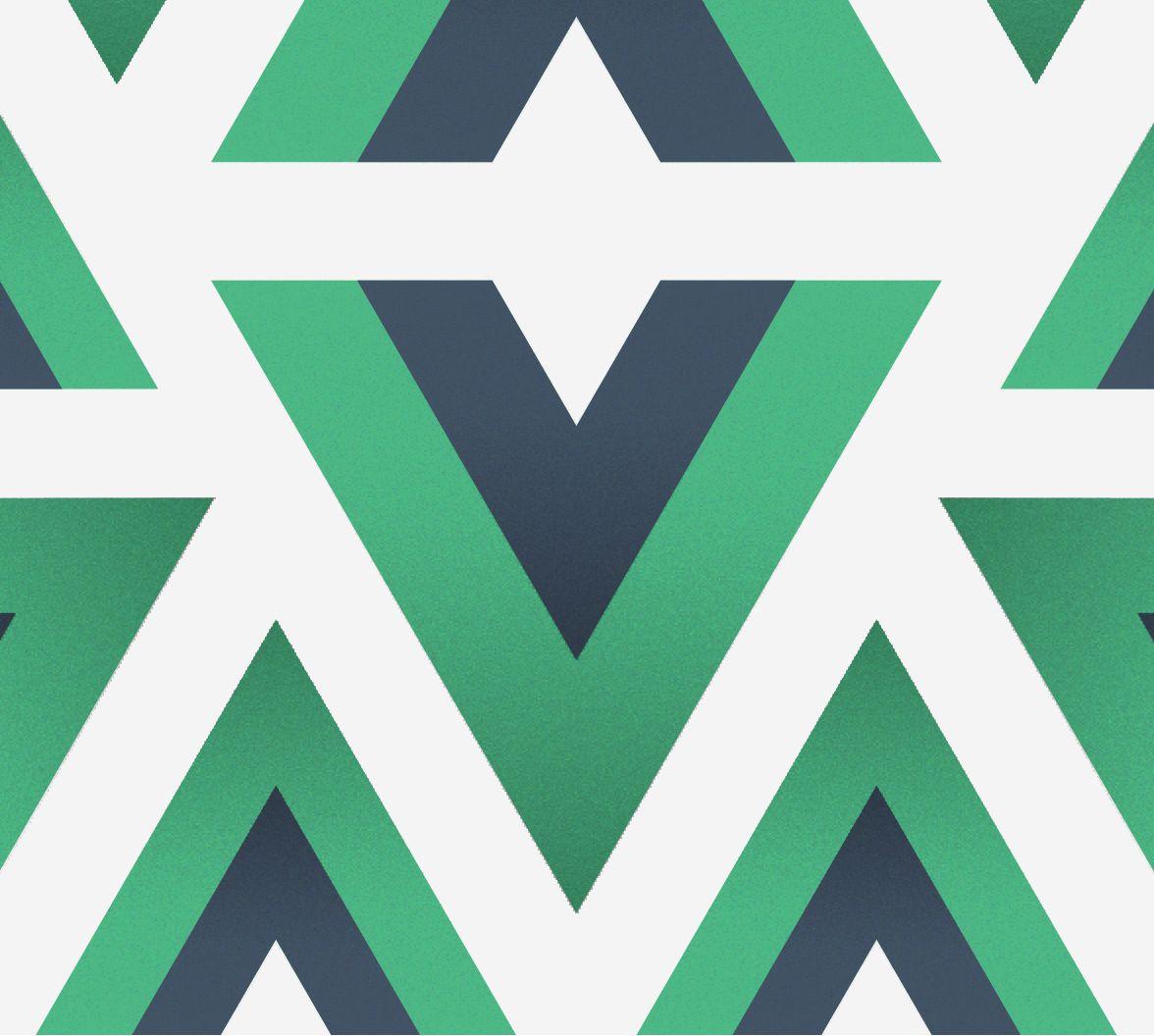
透過 CDN 使用 Vue
<!-開發環境版本,包含了有幫助的命令行警告->
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<!-生產環境版本,優化了尺寸和速度->
<script src="https://cdn.jsdelivr.net/npm/vue"></script>
Hello world !
Use Declarative Rendering
<div id="app">
{{ message }}
</div>
var app = new Vue({
el: '#app',
data: {
message: 'Hello Vue!'
}
})
Declarative Rendering
<!DOCTYPE html>
<html>
<head>
<title>My first Vue app</title>
</head>
<body>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<div id="app">
{{ message }}
</div>
<script>
var app = new Vue({
el: '#app',
data: {
message: 'Hello Vue!'
}
})
</script>
</body>
</html>
放進基本的 HTML 裡
app.message = "<your message>";
打開網頁修改 app.message 的值
Vue - CLI
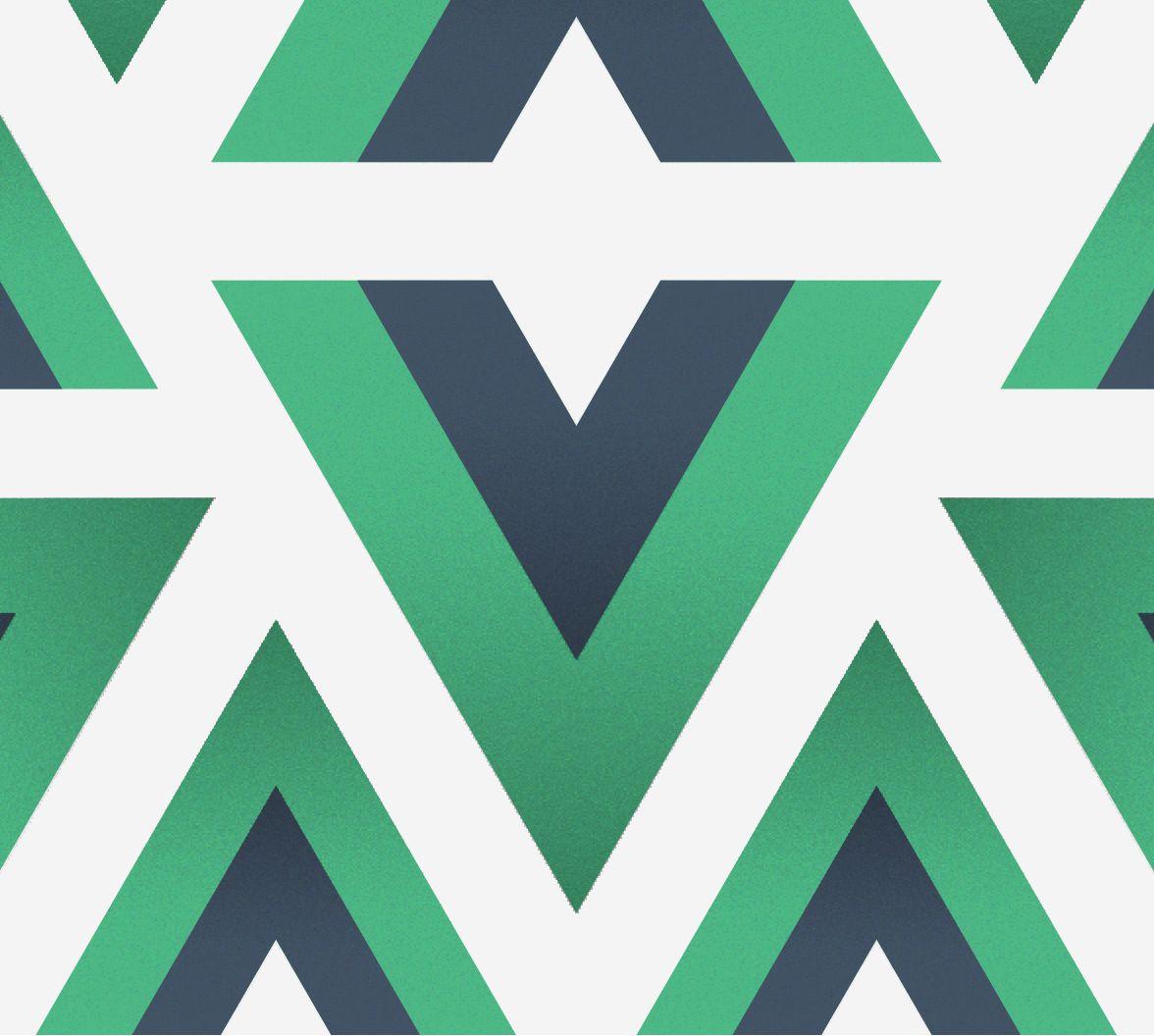
Vue-CLI
Vue-CLI 是 Vue 的命令行工具,可以快速開發大型單頁應用程式 (SPA)。
主要是提供開發者一個快速建置 Vue.js 架構的工具,
並基於 webpack,提供了許多相關的功能、套件安裝協助。

安裝 Node.js
確認一下裝好了
$ node -v
透過 npm 全局安裝 Vue - CLI
$ sudo npm install -g @vue/cli
for Windows
for Mac
$ npm install -g @vue/cli
確認一下裝好了
$ vue
Start a project.
建立專案
$ vue create <your-project-name>
初始設定
Vue CLI v4.5.6
? Please pick a preset:
Default ([Vue 2] babel, eslint)
Default (Vue 3 Preview) ([Vue 3] babel, eslint)
❯ Manually select features
- Default:會一步直接開始建構
- Manually:自己手動選擇需要的工具
初始設定 - 2
Vue CLI v4.5.6
? Please pick a preset: Manually select features
? Check the features needed for your project:
(Press <space> to select, <a> to toggle all,
<i> to invert selection)
❯◉ Choose Vue version
◉ Babel
◯ TypeScript
◯ Progressive Web App (PWA) Support
◯ Router
◯ Vuex
◯ CSS Pre-processors
◉ Linter / Formatter
◯ Unit Testing
◯ E2E Testing
Babel, Router, Vuex, Linter, Unit
初始設定 - 3
Vue CLI v4.5.6
? Please pick a preset: Manually select features
? Check the features needed for your project: Babel, Router, Vuex, Linter, Unit
? Use history mode for router? (Requires proper server setup for index fallback in production) (Y/n)
使用 Router 歷史記錄模式
初始設定 - 4
Vue CLI v4.5.6
? Please pick a preset: Manually select features
? Check the features needed for your project: Babel, Router, Vuex, Linter, Unit
? Use history mode for router? (Requires proper server setup for index fallback
in production) Yes
? Pick a linter / formatter config: (Use arrow keys)
❯ ESLint with error prevention only
ESLint + Airbnb config
ESLint + Standard config
ESLint + Prettier
選擇 ESLint + Prettier
初始設定 - 5
Vue CLI v4.5.6
? Please pick a preset: Manually select features
? Check the features needed for your project: Babel, Router, Vuex, Linter, Unit
? Use history mode for router? (Requires proper server setup for index fallback
in production) Yes
? Pick a linter / formatter config: Prettier
? Pick additional lint features: (Press <space> to select, <a> to toggle all, <i
> to invert selection)
❯◉ Lint on save
◯ Lint and fix on commit
選擇 Lint on save
初始設定 - 6
Vue CLI v4.5.6
? Please pick a preset: Manually select features
? Check the features needed for your project: Babel, Router, Vuex, Linter, Unit
? Use history mode for router? (Requires proper server setup for index fallback
in production) Yes
? Pick a linter / formatter config: Prettier
? Pick additional lint features: Lint on save
? Pick a unit testing solution:
Mocha + Chai
❯ Jest
選擇 Jest
初始設定 - 6
Vue CLI v4.5.6
? Please pick a preset: Manually select features
? Check the features needed for your project: Babel, Router, Vuex, Linter, Unit
? Use history mode for router? (Requires proper server setup for index fallback
in production) Yes
? Pick a linter / formatter config: Prettier
? Pick additional lint features: Lint on save
? Pick a unit testing solution: Jest
? Where do you prefer placing config for Babel, ESLint, etc.? (Use arrow keys)
❯ In dedicated config files
In package.json
選擇 In dedicated config files
初始設定 - 7
Vue CLI v4.5.6
? Please pick a preset: Manually select features
? Check the features needed for your project: Babel, Router, Vuex, Linter, Unit
? Use history mode for router? (Requires proper server setup for index fallback
in production) Yes
? Pick a linter / formatter config: Prettier
? Pick additional lint features: Lint on save
? Pick a unit testing solution: Jest
? Where do you prefer placing config for Babel, ESLint, etc.? In dedicated confi
g files
? Save this as a preset for future projects? (y/N)
是否將上述配置儲存到 preset 的 default
初始設定 - 8

DONE Compiled successfully in 18073ms
App running at:
- Local: http://localhost:8080/
- Network: http://192.168.0.11:8080/
Note that the development build is not optimized.
To create a production build, run npm run build.
運行專案項目
$ cd <your-project>
$ npm run serve
打開瀏覽器

我們開發只需要關注 src 的資料夾即可,其他的部分目前可以先放著沒關係。
├── README.md
├── babel.config.js
├── node_modules
│ ├──...
├── package-lock.json
├── package.json
├── public
│ ├── favicon.ico
│ └── index.html
└── src
├── App.vue
├── assets
│ └── logo.png
├── components
│ └── HelloWorld.vue
└── main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
new Vue({
render: h => h(App)
}).$mount('#app')
main.js
<template>
<div id="app">
...
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
...
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
...
}
</style>
App.vue
Tools
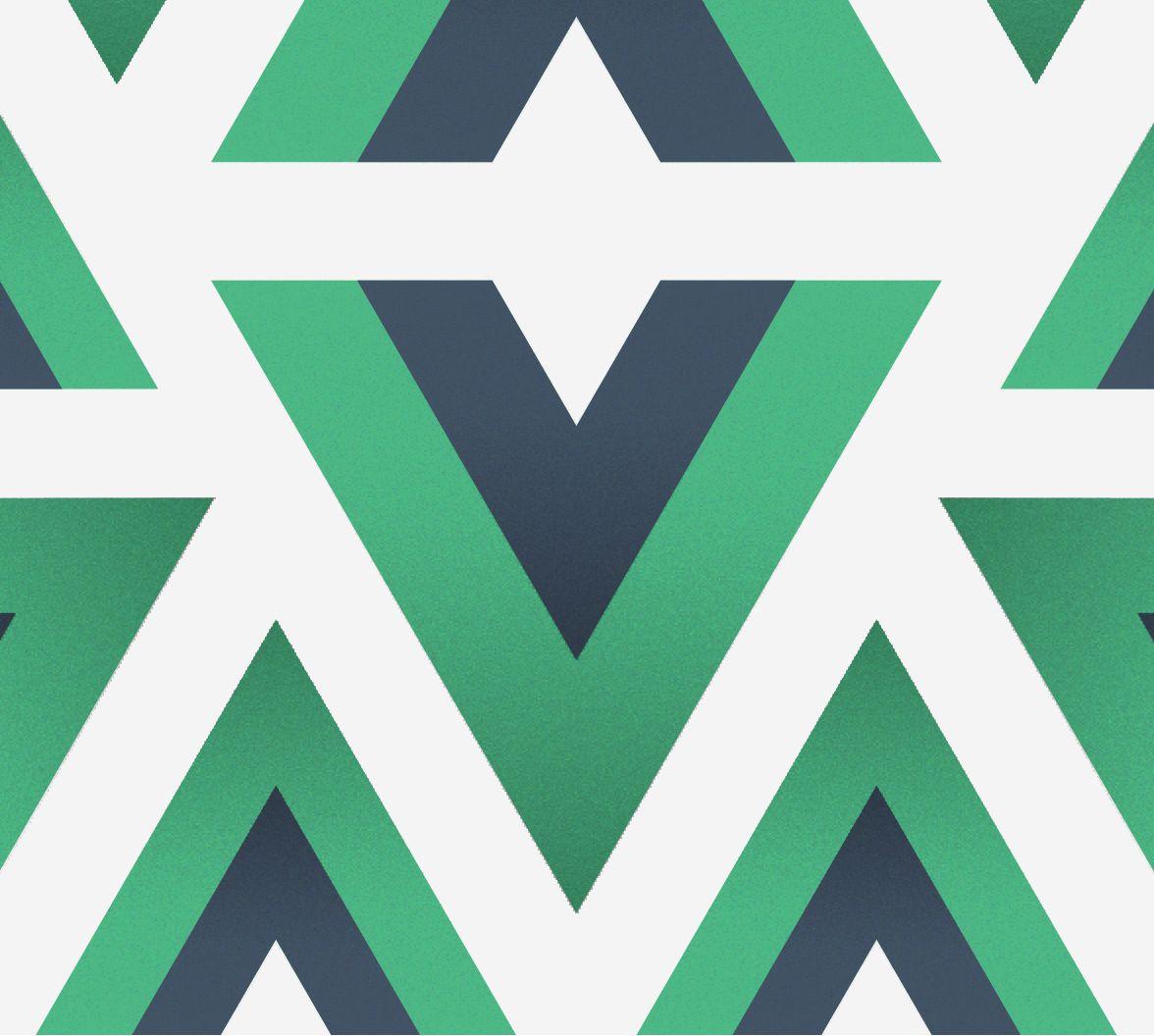
安裝 VS Code 延伸模組 - Vetur

Vue Devtools

Vue component
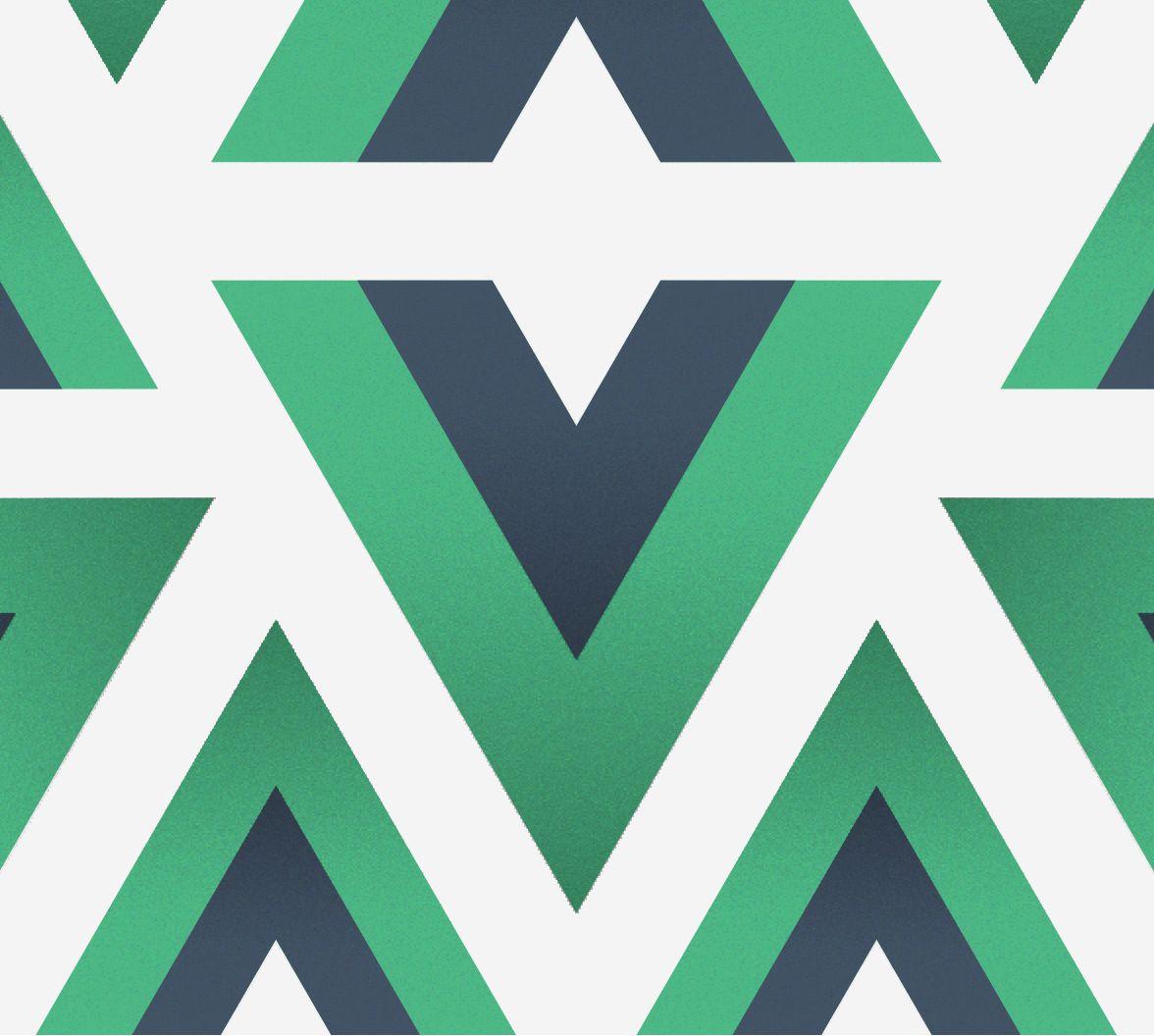
Components
每個 Vue 的應用程式都是從 Vue 建構式建立根實體開始,
再一個個元件(Components)搭建上去而來的,透過元件的方式能讓開發者將程式碼封裝而更好重用。
- Props
- Emit
而每個 Component 當中的 data 都會是互相獨立的,所以資料傳遞時,通常會仰賴下面兩種做法:

全域註冊 (Registration)
使用 Vue.component(tagName, options) 來註冊一個元件。注意,元件的註冊必須在 Vue Instance 初始化前完成。
Vue.component('my-component-name', {
// ... options ...
})

<div id="app">
<prompt-component></prompt-component>
</div>
全域註冊 (Registration) 範例
Vue.component('prompt-component', {
template: '<div><p>{{message}}</p><button @click="sayHi">Say Hi!</button></div>',
data: function () {
return {
message: 'Hello World!'
}
},
methods: {
sayHi: function() {
alert('Hi');
}
}
})
var vm = new Vue({
el: '#app',
});
全域註冊 (Registration) 範例 - 2
var Prompt = {
template: '<div><p>{{ message }}</p><button @click="sayHi">Say Hi!</button></div>',
data: function () {
return {
message: 'Hello World!'
}
},
methods: {
sayHi: function() {
alert('Hi');
}
}
};
var vm = new Vue({
el: '#app',
components: {
'prompt-component': Prompt
}
});
局部註冊 (Local Registration)
References
apa 格式加載中
- https://ithelp.ithome.com.tw/articles/10213639
- https://cythilya.github.io/2017/05/11/vue-component-intro/
Vue.js - Basic
By oneone
Vue.js - Basic
- 67