Angular.js
In depth
- Template - HTML with additional markup,
- Directives - extend HTML with custom attributes and elements,
- Model - the data shown to the user in the view and with which the user interacts,
- Scope - context where the model is stored so that controllers, directives and expressions can access it,
- Expressions - access variables and functions from the scope,
- Filter - formats the value of an expression for display to the user,
- View - what the user sees (the DOM),
- Data Binding - sync data between the model and the view,
- Controller - the business logic behind views
Core concepts
Advanced topics
- Compiler - parses the template and instantiates directives and expressions
- Dependency Injection - Creates and wires objects and functions
- Injector - dependency injection container
- Module - a container for the different parts of an app including controllers, services, filters, directives which configures the Injector
- Service - reusable business logic independent of views
Data binding
Two way
- From Template to View - $compile
- View - Model - constant watch on changes and updates
One time
Single merge Model and Template into View


In details

More complex example

How is done
Digest-Apply loop

Controllers
Do
- setup and init $scope,
- add methods on the $scope
Do not
- manipulate DOM,
- filter output,
- share code,
- manage lifecycle of other components
Services
Review
- lazy instantiated - JS style,
- singletons - also JS style
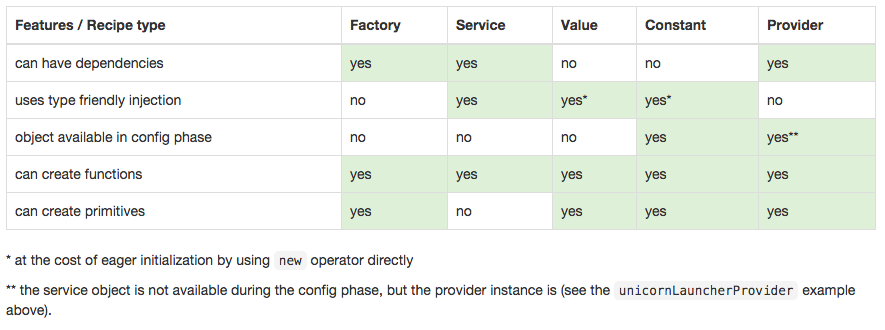
Scope
Concepts
- $watch - provides observer,
- $apply - propagate changes,
- sharing options:
- no scope - use parent,
- child - inherit from parent,
- isolated.
- $rootscope - main scope tree,
- adds context to expressions - {{ }}

Manual watches
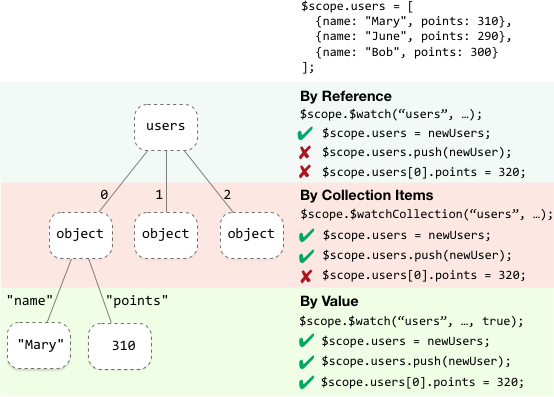
DI container
Basics
- $provide - recipe how to create injectable,
- $injector - creates instances, any function is called via method $injector.invoke
- during config phase only providers can be injected,
- controllers, filters and directives are special

DI in action
config
- Is being executed during provider registration and configuration phase.
- Only provider and const can be injected into config
run
- Is being executed after injector is created and used,
- Only service, value, const
Angular bootstrap process
Templates
What can be used
- Directives - reusable DOM components,
- expressions - {{}} evaluates functions, has access to scope
- Filters - data formatter,
- Forms - validation and data input
Filters
Basics
- can be used in controllers, services and directives,
- can be created via $filterProvider,
- by design should be stateless,
- can be chained,
- can take optional arguments
Directives
What are they, actually?
- have access to DOM element,
- can have own scope,
- can have dependencies,
- assembled by $compile,
- attaches behavior to elements,
- can transform children
Build-in
- ng-app - creates and inits angular.js app,
- ng-model - attach model to the input field,
- ng-click - runs function on user click,
- ng-if - removes DOM element from html,
- ng-repeat - foreach over DOM element
Custom directives
- restrict - defines way how it could be used in DOM (attribute, element, ...),
- template, templateUrl - defines HTML fragment used in directive body (single root),
-
scope
- false (default) - use parent,
- true - create new child,
-
{} - create new isolated scope,
- @ - one way binding of a string,
- = - two way biding between scopes,
- & - expresion evaluation on parent
Three happy friends
- compile - function to perform DOM element operations on each instance of our directive,
- link - function run as soon directive is linked to DOM, used to register callbacks on individual scope,
- controller - function used to interact between directives without touching DOM elements
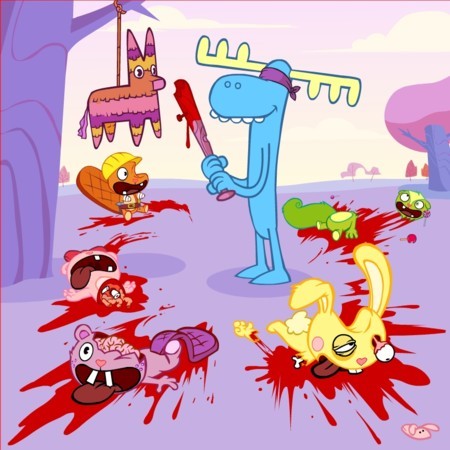
Transclusion
Nesting DOM elements in directives template.
- use transclude: true in directive function,
- on element in template use ng-transclude
Directives composition
Property require in directive
- foo - require "foo" directive on same element, pass it to linking function,
- ?foo - pass "foo" controler function to our linking function,
- ^foo - require "foo" on the one of the parent elements and pass to linking function,
- ?^foo - pass "foo" controller function from parent to our linking function.
Backend integration
Promises
- represents the eventual result of an operation,
- use them to specify what to do, when operation will succeed or fails,
- resolves single time
In practice
var promise = $http.get("/api/my/name");
promise.success(function(name) {
console.log("Your name is: " + name);
});
promise.error(function(response, status) {
console.log("The request failed with response " + response + " and status code " + status);
});
////////////////////////////////////////////////////////////////////////////////////////////////
// Same thing, diffrent way //
////////////////////////////////////////////////////////////////////////////////////////////////
$http.get("/api/my/name").then(
/* success */
function(response) {
console.log("Your name is: " + response.data);
},
/* failure */
function(error) {
console.log("The request failed: " + error);
});
$http
- returns $q promise,
- can utilize JSONP,
- general purpose AJAX (XHR) calls,
- can manually specified method, data, status or headers
- can utilize JSONP
$resource
- returns $q promise,
- can utilize JSONP,
- uses $http,
- single endpoint,
- resource as JS object
- wrapper for RESTful API scenarios (GET, POST, DELETE)
Comparison
////////////////// $http ////////////////////////
var users; // A place to store our data
// Setup the request.
var myHttpPromise = $http.get('users');
// Call the request.
myHttpPromise.then(function(response) {
// Handle the response.
users = response.data;
});
////////////////// $resource //////////////////
var MyResource = $resource('/users/:userId', { userId:'@userId' });
var getUser = function (userId) {
return MyResource.get({ userId: userId }).$promise;
}
FIN
Q&A
angular-in-depth
By Rafał Warzycha
angular-in-depth
- 588