Elegant state management
@rahulgaba16 → ReactFoo-VueDay Pune 2020
Using Context API + Hooks
Rahul Gaba


UX Engineer @ Google
Elegant state management
Using Context API + Hooks
@rahulgaba16 → ReactFoo-VueDay Pune 2020
Outline
-
Why state management?
-
Benefits of redux
-
Problems with redux
-
Context, Providers and Consumers
-
The useReducer Hook
-
Benefits of Context API + Hooks
-
When to use what?
Why state management?
- Sharing data
- Caching data
- Separation of concerns
Redux

Benefits of redux
- Separation of concerns.
- Share data between multiple components.
- Cache data between multiple routes.
- Time travel debugging.
- Structured way to maintain the code.
Problems with Redux
- What to store in redux? Shared data/Persistent data?
- Keeping something in store and then realising that it shouldn't have be there.
- Too much of a boilerplate.
- Too many things to read about.
Problems with Redux

Context, Providers and Consumers.
Context & Provider

Consumer


Provider
- Holds the module level state
- Contains methods to manipulate state.
- Defines API for the consumers (using value prop)
- ContextInstance.Provider
Context Instance
- Used by Regular React components, called consumers.
- Used to access the APIs defined by the Provider.
- React.createContext(<intialValue>)
Two most important hooks

Consumer with useContext



The useReducer Hook
Building a cart
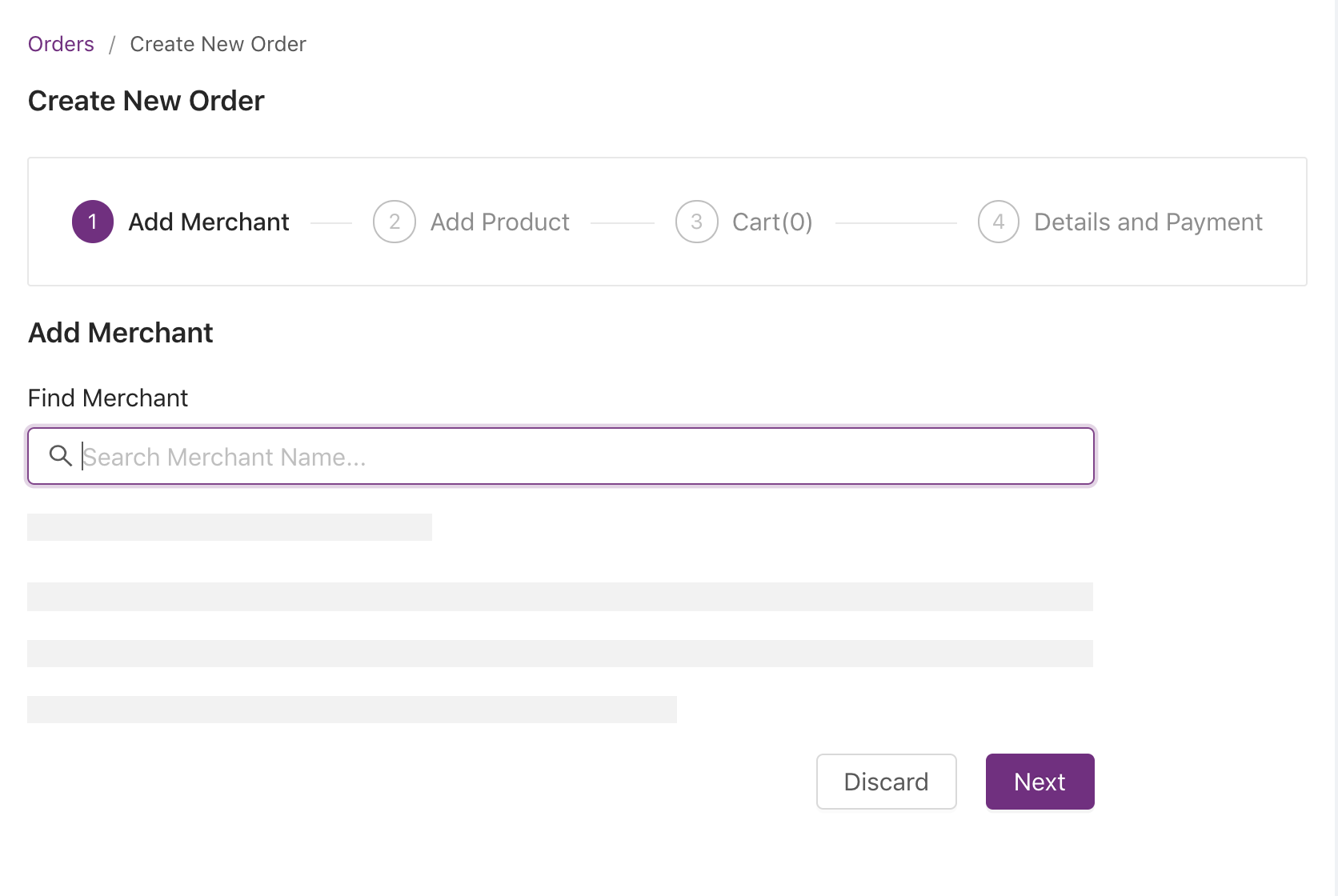



Provider with reducer


Where to use Providers?

Consumers?

5 Reasons to use redux
- Sharing data
- Caching data
- Separation of concerns
- Middlewares*
- Ease of debugging*
Advantages over Redux
Advantages over Redux
-
Have module level "stores" (Chunking)
-
Use reducer only if you feel it's necessary.
-
Very easy to move logic from a component to a Provider.
-
Incremental, composite way of doing things.
Redux
Providers + useReducer
Disadvantages
- No middleware support
- Limited SSR support
- Too many render cycles*
- No time travel debugging
When to use What?
Use Context API + Hooks when...
Use Redux when...
- You are already using it 😉
- You need middlewares
- You need SSR
- Possible use-cases:
Don't

Tips
- One file exports both context instance and provider.
- Create Module level context for sharing module-level state.
- `useContext` hook provides a very convenient and cleaner way to use multiple contexts in a component.
- Avoiding the bad parts of redux: Solve present problems instead of problems which might occur in future.
- Use latest dev tools to debug context.
References
- https://kentcdodds.com/blog/the-state-reducer-pattern-with-react-hooks
- https://twitter.com/threepointone/status/1056594421079261185
- https://www.youtube.com/watch?v=dpw9EHDh2bM
- https://twitter.com/sarah_edo/status/1186615191808536576?s=09
- https://stackoverflow.com/questions/45416237/axios-calls-in-actions-redux
- https://github.com/troch/reinspect
- https://dev.to/selbekk/persisting-your-react-state-in-9-lines-of-code-9go
- https://twitter.com/dan_abramov/status/955593635390808064

Thank you!
@rahulgaba16 → ReactFoo-VueDay Pune 2020
Q&A
Elegant state management
By Rahul Gaba
Elegant state management
Slides for the talk on Elegant state management (Using Context API + Hooks)
- 2,884