ES6/ES2015
Bringing JavaScript into this decade
May the Fourth be with you!!!

What is ES6/2016?
- ECMAScript 6 which was officially renamed ECMAScript 2015 (the next version is ES2016)
- The newest version of ECMAScript with new toys (yay!)
Ecma International is an industry association founded in 1961, dedicated to the standardization of information and communication systems.

What the literal F is ECMAScript?
ECMAScript is the language, JavaScript (and others) are dialects.
Sorta like how English is the language, but there are eleventybillion dialects (how many different ways can you say the plural you? Y'all, Youins, You's, You's Guys, etc.)
So, What's New, Pussycat?
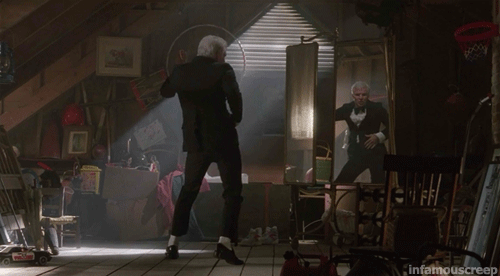
ES5 - (Old Busted)
ES6 - (New Hotness)
var cantina = "Mos Eisley";
Say goodbye to var, let const be your friends
const cantina = "Mos Eisley";
let karaoke;
karaoke = "Bill Murray";
- Use const for constants
- Use let for variables
- Both const and let are block scoped (var was function or global scoped)
- Prefer const unless you have a good reason to use let.

ES5 - (Old Busted)
ES6 - (New Hotness)
function blah() {
return "blah";
}
function defaultParams(name) {
if(name === null || name === '') {
name = 'Emperor Palpatine';
}
...
}
Dysfunction Junction - What's your function?
blah() {
return blah;
}
defaultParams(name='Emperor Palpatine') {
// no need to provide if statements
// if name is not provided, it uses
// the specified value
...
}
So, let's be real here: is this earth shattering? Does the elimination of the keyword prevent you from getting carpal tunnel? (Well, it might...)
(Note that the function keyword is still required for global functions, but 95% of the time this won't apply)

ES5 - (Old Busted)
ES6 - (New Hotness)
Fat Arrow syntax => Take that, Cupid!
blahs.forEach(function(blah) {
return "I can't even " + blah;
});
blahs.forEach(blah => {
return "I can't even " + blah;
});
blahs.forEach(blah =>
"I can't even " + blah;
)
Unlike function, fat arrows (no, this isn't arrow shaming)
share this with their surrounding code
(which means no more of that stupid .bind() bs.
Well, mostly...)
-> Skinny arrow | => Fat Arrow

ES5 - (Old Busted)
ES6 - (New Hotness)
ES2015 went from ashy... to classy
//There is no direct class in ES5
//Here's a pretty lame attempt
function Course() {
var self = this;
var _title = "Course Title";
get title = function() {
return _title;
}
set title = function(title) {
self._title = title;
}
}(ICourse);
class Course extends ICourse {
constructor(sweetness) {
super(sweetness);
this._title = "Course Title";
}
get title() {
return this._title;
}
set title(title) {
this._title = title;
}
}
ES2015 classes support inheritance, static methods and constructor functions. How's that look to you Java or C# peeps?
This is just syntactic sugar over prototypal inheritance, specifically to please the Java and C# peeps...
ES5 - (Old Busted)
ES6 - (New Hotness)
"this" + "string" + "concatenation" + "sucks";
var theAnswerToEverything = 42;
var stringificated = "Jackie Robinson: #"_
+ theAnswerToEverything;
//This would not work in ES5
var multiLineString = "This string is
broken onto multiple different
lines and would break ES5.";
//Go ahead, try it in ES5, see if it works.
function ynot(name, age, favoriteShow) {
return "Hello, " + name + ". Your age is "_
+ age + " years old. Your favorite show_
is " + favoriteShow + ". But what about_
Hamilton?";
}
const theAnswerToEverything = 42;
const stringificated = `Jackie Robinson: #_
{theAnswerToEverything}`;
//This works all day long in ES2015
const multiLineString = `This string is
broken onto multiple different lines
and works just fine in ES2015.`;
ynot(name, age, favoriteShow) => {
return `Hello, ${name}. Your age is ${age}
years old. Your favorite show is
${favoriteShow}. But what about
Hamilton?`;
}
String templating, the ES2015 way. Easier to read, supports multiple lines, doesn't require escaping of characters, etc.
Note the use of the backtick (`) to indicate a string template.

ES5 - (Old Busted)
ES6 - (New Hotness)
Object, why do you have to be so literal?
var color = "blue";
var lightSaber = {
color: color,
owner: 'Yoda',
slash: function() {
return "Wobble";
}
};
const color = 'blue';
const lightSaber = {
// shortcut for color: color
color,
// method shortcut
slash() {
return "Wow!"
}
// super calls
toString() {
return `Yoda ${super.toString()}`;
}
// dynamic property names
[ 'prop' + (() => 1138)() ]: 42 + 1138;
}
A few little niceties added for ES2015 -
shortcuts, dynamic property names, super calls, etc.
And now a word from our sponsor
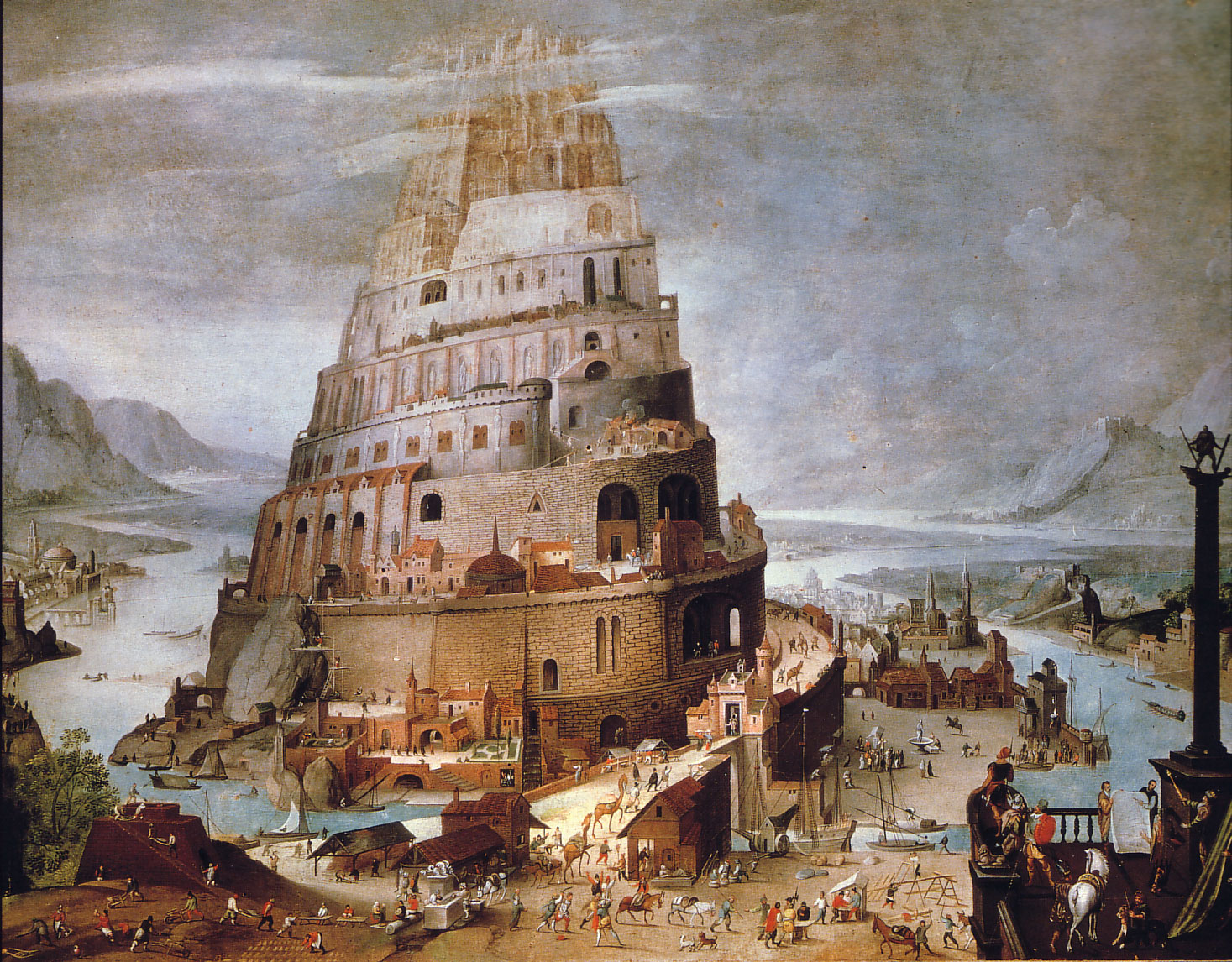
And now a word from our sponsor
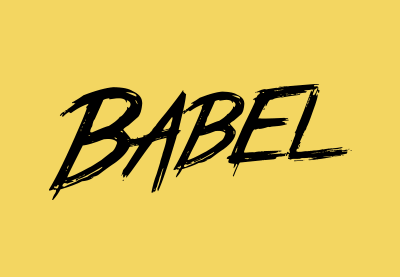
Babel FAQ
- What is it?
- Babel is a JavaScript library
- What does it do?
- It transpiles ES2015/16 to ES5 (for compatibility)
- It allows you to use ES2015/16 syntax and not worry about browser compatibility issues
- Does it translate Vogon poetry?
- Mercifully, no.

Just when I thought I was out, they pull me back in...
ES2015 Module Imports
import moduleName from 'moduleName';
import { pieces, ofFunctionality, fromModules } from 'moduleName';
//Real world example
import React, {Component, PropTypes} from 'react';
import * as Constants from './constants';
-
Modules aren't imported until needed, and are implicitly imported asynchronously (no code executes until modules (and dependencies) are successfully loaded)
-
Does require a module loader (like Webpack, SystemJS/JSPM, CommonJS, etc.)
Revolutions are not made for export - Nikita Khrushchev
Yeah, well modules are (in ES2015)
export function destroyTheSith() {
return "The force will you use";
}
export default class SithLord {
constructor() {
...
}
}
export const ITS_A_TRAP = 'Admiral Akbar';
-
Any primitive can be exported:
Functions
Consts
Classes
I don't know about the rest of you, but I'm betting on the spread... - Jason Clark
Rest/Spread (...) operator
const { sith, jedi, empire } = this.props;
const newCharacter = {
sith,
jedi,
empire,
{...restOfProps}
};
- Rest/Spread operator is a shortcut for arrays
- Rest - provide the remaining items of an array as an array (without having to define explicitly)
- Spread - apply each item of an array without having to use an explicity forEach (or other array functions)
function characters(luke, jarjar, ...others) {
// ... operator provides the remainder of
// params provided as an array
others.forEach((other) => {
...
});
}
const additions = [];
function addToList(item) {
additions.push(item);
}
addToList(...[han, leia, chewie]);
// (each of the array items is passed)
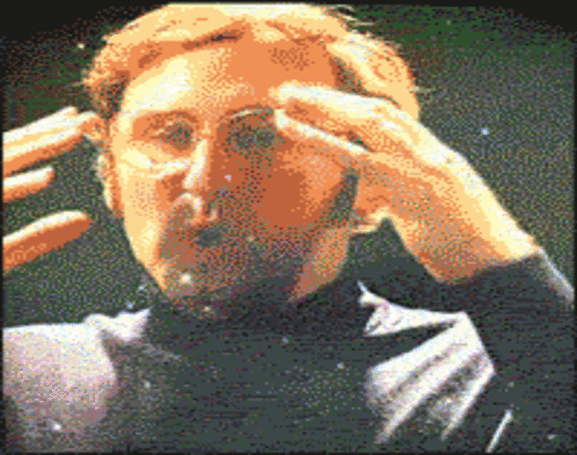
Other New Stuff
- Destructuring - An initial run at pattern matching for both objects and arrays (not nearly as powerful as pure functional pattern matching, but a step in that direction)
- Iterators - C# peeps, do you like IEnumerable? Java peeps, do you like Iterable? This is right up your alley.
- Symbols
- Binary and Octal Literals
- Generators
- Promises (still in infancy)
- await/async (ES2016)
Questions???
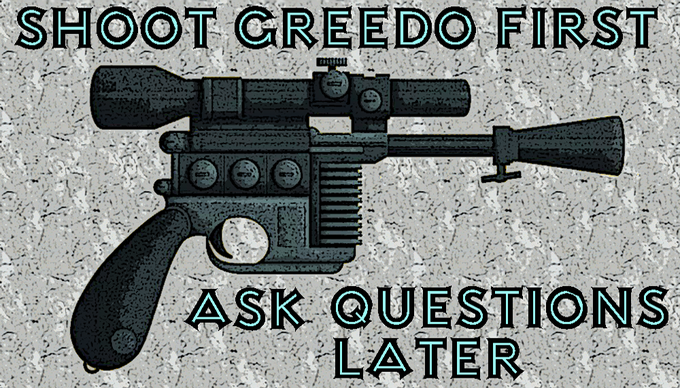
WISE Links
- WISE Sharepoint Site - http://bit.ly/wisesite
- WISE Survey - http://www.cengage.com/wisesurvey
These slides are available independently at https://slides.com/riceboyler/es2015
Thank you!!!
ES2016
By Jason Clark
ES2016
Bringing JavaScript into the Teens
- 834