By Colten Rouska
v0.10.28
What?
Node.js is a platform built on Chrome's JavaScript runtime for easily building fast, scalable network applications. Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices.
Really??
Node is only 5 years old.
Ryan Dahl
Advantages
-
Commonality of language (javascript)
-
Can handle thousands of concurrent connections on 1 process
-
Models, validation, services can be shared (Client, Server)
- Huge community
Example Good Use Cases
JSON API's
Streaming
data
Single page apps
Unix tools/scripting
Real time applications*
* Fairly light realtime web applications
Disadvantages
-
Intensive CPU
-
http://Serve.me/content
-
Unclear code organization
- Asynchronous code
asynchronous code
async1(function(input, result1) {
async2(function(result2) {
async3(function(result3) {
async4(function(result4) {
async5(function(output) {
// do something with output
});
});
});
});
})
Packages!

-
N
ode
P
ackage
M
anager
~75,000 pkgs

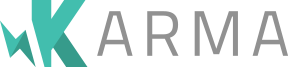
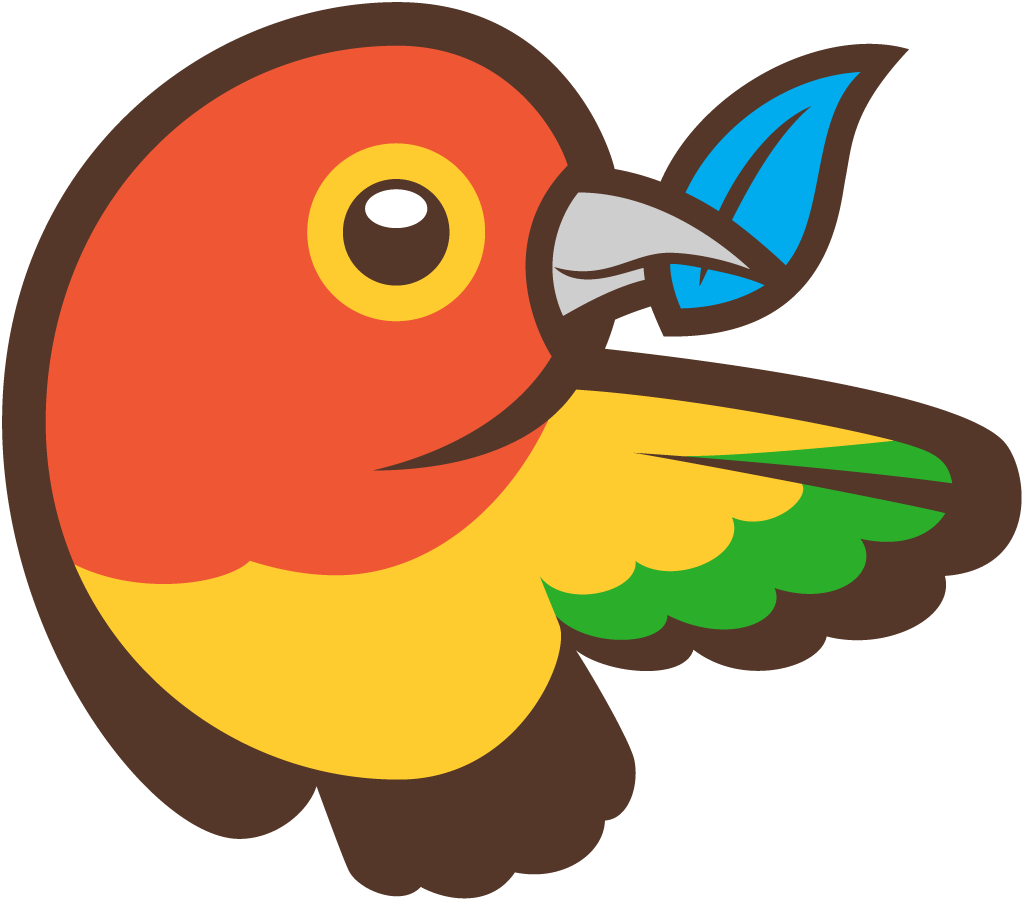
using npm
package.json
npm install <some-pkg> [--save-dev]
This will generate a node_modules/ directory with the specified packages inside.
npm install <some-package> [-g]
Install the package globally making it so it doesn't need to be in the project's package.json file. You can also use the package anywhere in your terminal.
Existing package.json?
npm install
package.json
Used for managing custom NPM package variables
{
"name": "SomeCoolPlugin", "version": "9.0.0.1", "description": "Something cool over 9000!", "main": "server.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "author": "My Good Friend", "license": "BSD-2-Clause" }
Used for managing
dependencies
{
"dependencies" : {...} "devDependencies": {...} }
Simple sample!
var http = require('http');
http.createServer(function(request, response) {
response.writeHead(200, {'content-Type' : 'text/plain'});
response.end("helloWorld");
}).listen(8080, '127.0.0.1');
console.log("Running Server on http://127.0.0.1:8080");
helloworld
TCP server
var net = require('net'),
readline = require('readline');
var server = net.createServer();
server.on('connection', function(socket){
console.log("Connected! " + socket.remoteAddress);
socket.write("Welcome " + socket.remoteAddress);
socket.on('data', function(message){
console.log('Received "' + message + '". Applying mystifications.');
message += "!";
socket.write(message);
});
});
server.listen(8081, '127.0.0.1');
TCP Client
var net = require('net'),
readline = require('readline');
var client = new net.Socket();
client.connect(8081, '127.0.0.1', function() {
console.log('Connected');
var rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
rl.on('line', function(cmd){
console.log("Sending: "+ cmd);
client.write(cmd);
});
});
client.on('data', function(data) {
console.log('Received: ' + data);
});
client.on('close', function() {
console.log('Connection closed');
});
Extending Functionality
To the chat client!
Who use it?
Groupon
Linkedin
Microsoft
Yahoo!
Walmart
PayPal
Resources
Node.js in Action - by Mike Cantelon
CONCURRENCY X REQUESTS
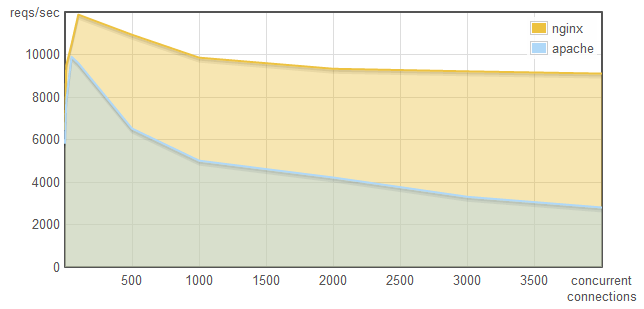
CONCURRENCY X MEMORY
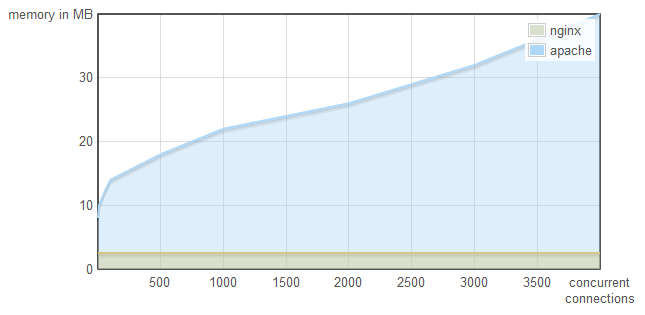
Node.js
By Colten Rouska (Rizowski)
Node.js
Towers Watson presentation on NodeJs
- 2,932