5 min Passport.js 🗺✨
By Stefan Baatz
Sounds kinda cool. But uh, what is it again?
- An OAuth authentication middleware for Node.js, and it works great with Express, too!
- Simple and compartmentalized, it strictly deals with authenticating users in your app
- Piggy-backs off the established authentication services of companies such as Facebook, Twitter, GitHub, etc...
- Easy to pull into your app, just run npm install passport or yarn add passport in ya repo!
How does it work bruh?
- Different websites employ different types of considerations to allow the client to successfully authenticate themselves
- These different authentication mechanisms are handled in Passport via a growing library of strategies, with 300+ already available
- Each strategy provides an abstracted roadmap for connecting to a specific third-party app's authentication service, e.g. Facebook
- You can also just handle authorization in your app via a Local Strategy that you specify, or even let the user choose how to sign in!
Roadmap after installing the package
- Require in Passport and Local Strategy
- const passport = require('passport')
- const LocalStrategy = require('passport-local').Strategy
- Include passport.initialize() and passport.session() as middleware in Express (e.g. in the app.js use stack)
- app.use(passport.initialize())
- app.use(passport.session())

Here's a sample of Local Strategy code
Here's a sample of Facebook Strategy code

So what happens when the user is authenticated?
- You will end up using the OAuth response from the 3rd party site to create a user (or sign in if they've created an account before)
- You can then use the response to build a session token, e.g. with cookies
- And voilà ... you've leaned on a major corporation for auth services and security responsibility, signed in and/or created the user, and built a session so that they are authorized on your app
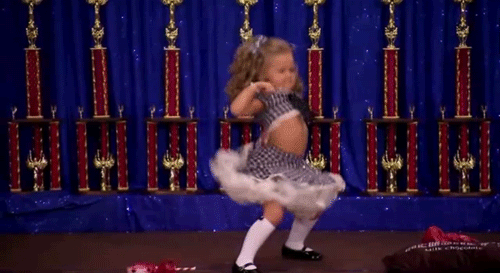
Bonus best-practice stuff ... serializing and deserializing user information
- Both these functions are middleware on passport
- With serializing you filter down their user object via custom parameters to just what you need to initiate routes and identify the user. This occurs when the user is authenticated on your app, and the data is saved in the user's session
- When the user makes a new request on your site, you can employ passport.deserializeUser to recover the full user object from your database, or even modify the user object via JavaScript logic if you need to
Why serialize and deserialize session data?
Obfuscate session information that the user simply doesn't need to see!
Simple.
Now go forth, use Passport, be blameless and lazy 🙌🏻
FIN.
deck
By sabaatz
deck
- 397