Best Practices #1
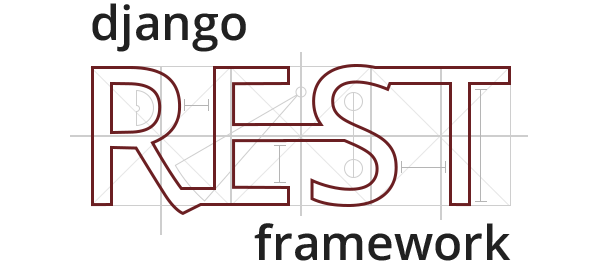
Andres Duque Hincapie
Software Developer
@saduqz
Documentation
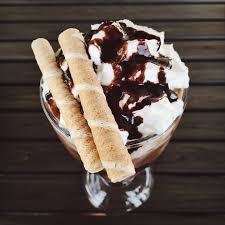
Write the Documentation
What would you choose?
Why is important?
- You need document the project for other people
- You always write code for other people
- You will not understand your code in 3 months
You'll want to learn and follow the standar Python best practices
reStructuredtext (RST)
Is the common markup language used
Docs Examples
- https://docs.djangoproject.com/en/1.11/
- https://docs.python.org/3/
- https://docs.python.org/3/
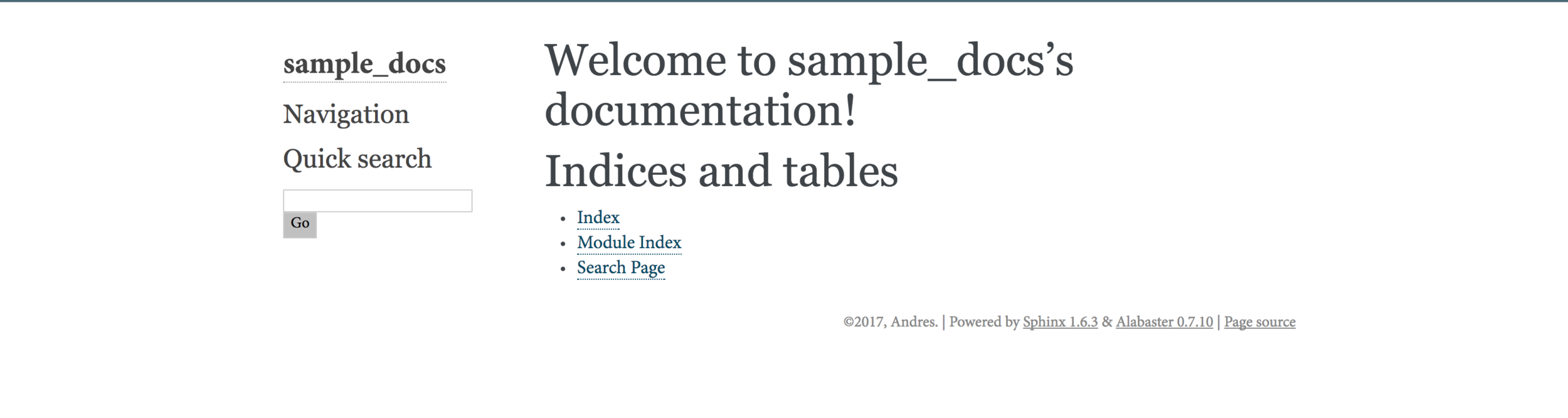
What Docs should django projects contain?
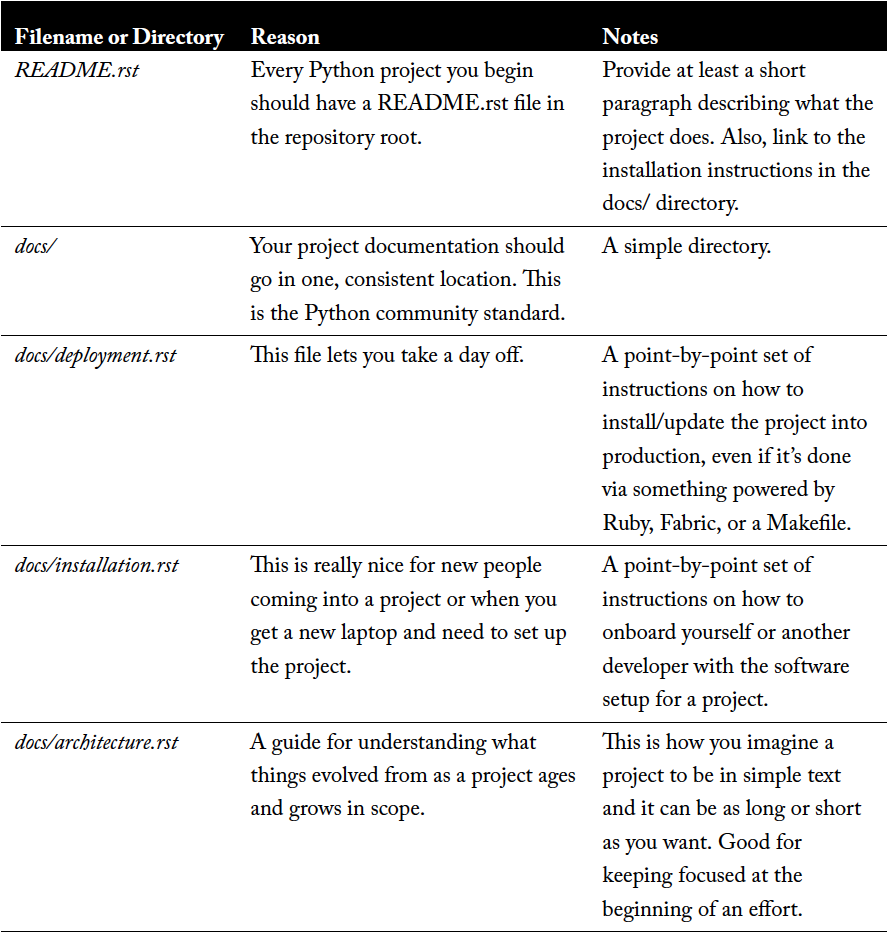
Docstrings
def kos_root():
"""Return the pathname of the KOS root directory."""
global _kos_root
if _kos_root: return _kos_root
...
def complex(real=0.0, imag=0.0):
"""Form a complex number.
Keyword arguments:
real -- the real part (default 0.0)
imag -- the imaginary part (default 0.0)
"""
if imag == 0.0 and real == 0.0:
return complex_zero
...
More Approachs
def complex(real=0.0, imag=0.0):
"""
Form a complex number.
:param real: the real part (default 0.0)
:param imag: the imaginary part (default 0.0)
:return: some calculate value
"""
if imag == 0.0 and real == 0.0:
return complex_zero
...
def complex(real=0.0, imag=0.0):
"""
Form a complex number.
:param real: Float, the real part (default 0.0). Ie, 1.4
:param imag: Float, the imaginary part (default 0.0). Ie, 1.8
:return: Float, some calculate value. Ie, 3.2
"""
if imag == 0.0 and real == 0.0:
return complex_zero
...
References
- https://www.python.org/dev/peps/pep-0257/
- https://www.twoscoopspress.com/products/two-scoops-of-django-1-11
Testing
Testing Save
Jobs, Money and Lives
A single miles vs kilometers mistake cost a company hundred of thousands of dollars in a matter of hours
When is critical ?
Your application ...
- Handle medical information
- Provides life-critical resources to people in need
- Works with other people's money or will do it
How to structure test?
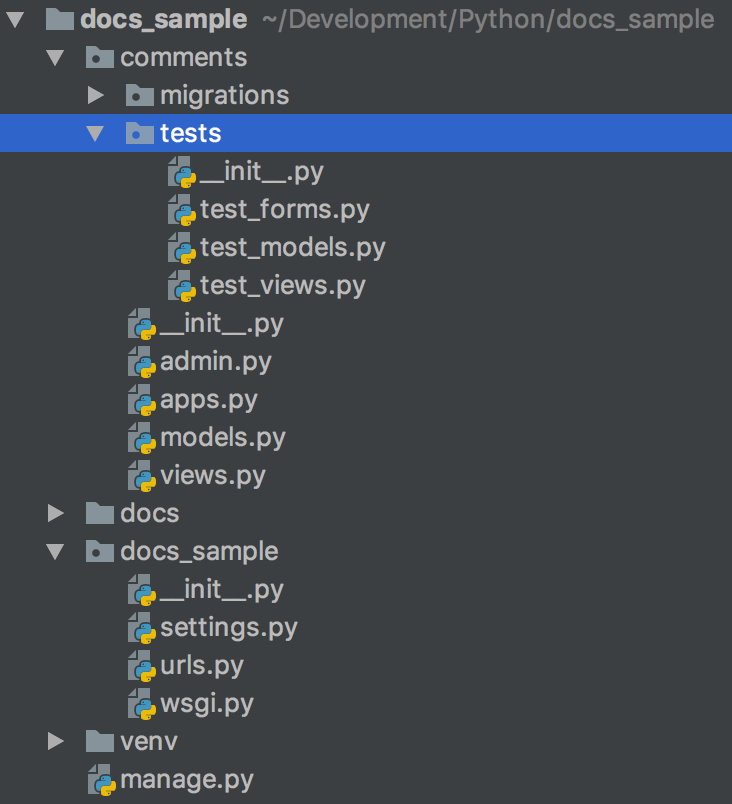
If you have another files, just create corresponding test files
TIP: Prefix test modules with test_
How to write Unit Tests
Each Test Method Tests One Thing
A single unit test should never assert the behavior of multiple views, models, forms
Unit test should assert the behavior of a single view, form, model or method
How does one run a test for a view, when views often require the use of models, forms, methods and functions?
The trick is be absolutely minimalist
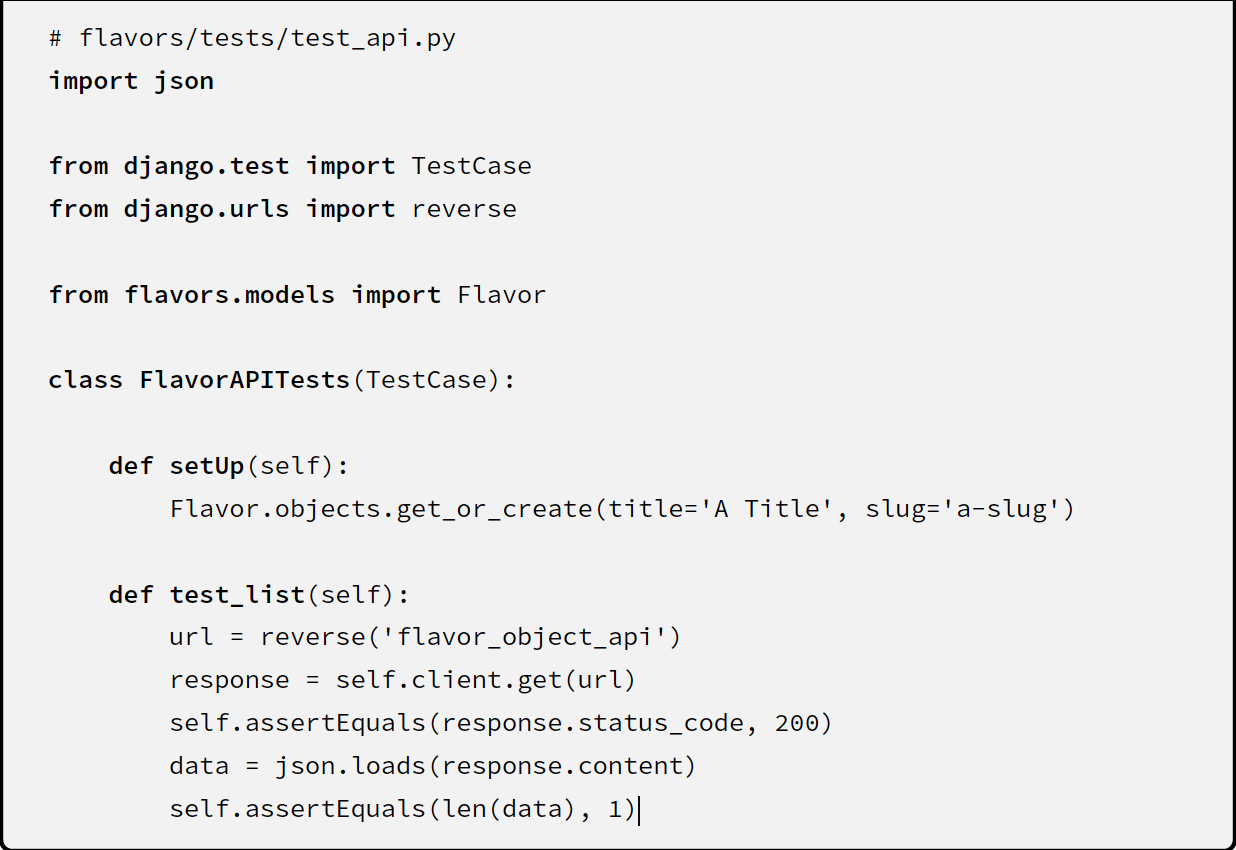
setUp(): Minimum possible number of records needed to run the test
Much larger example
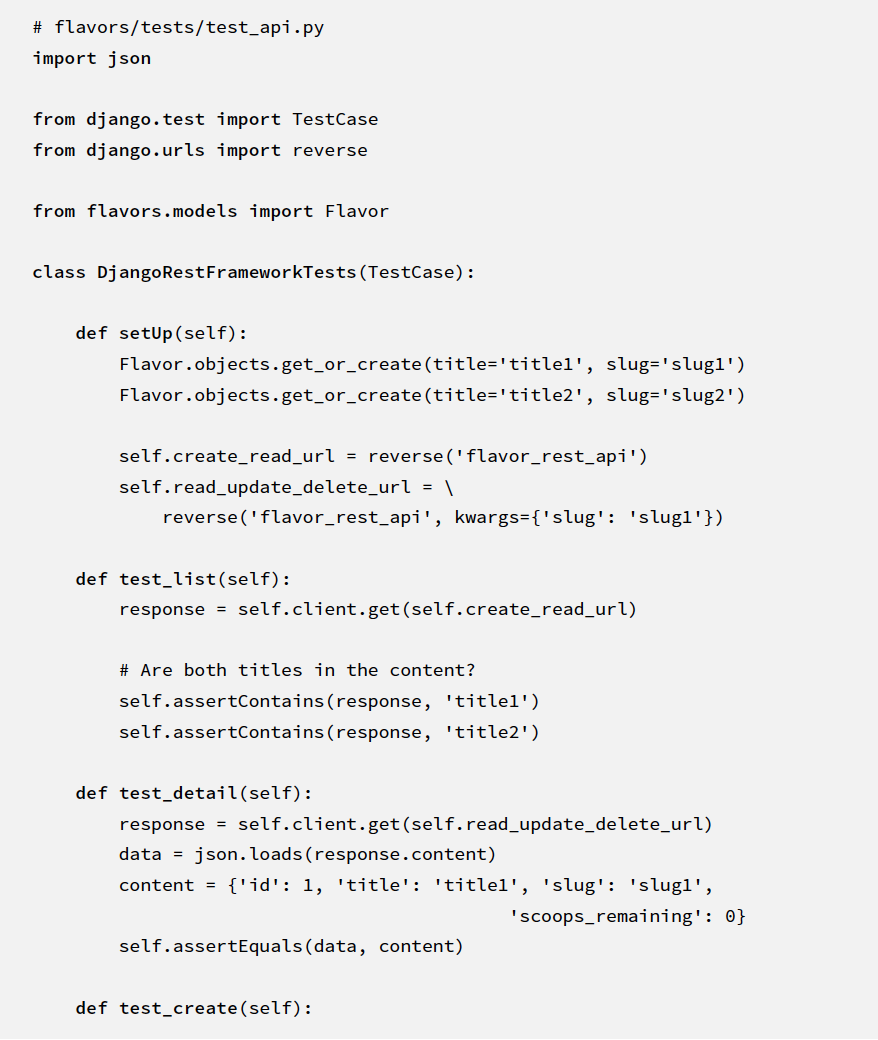
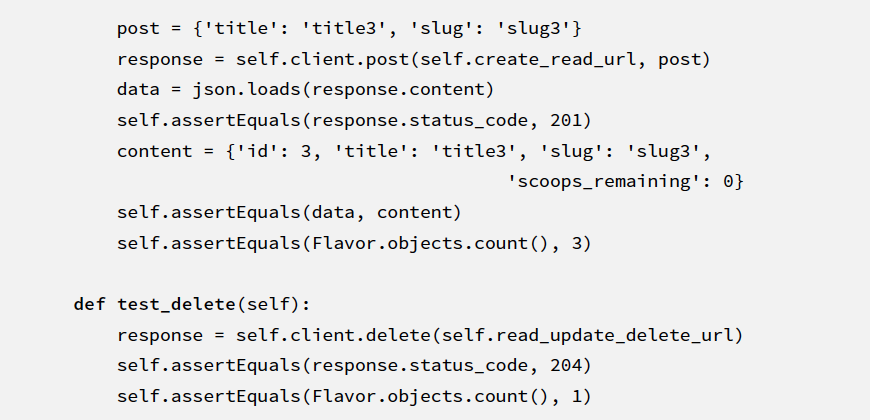
Tools to generate Test Data
Factory Boy
generates model test data
Faker
Generates test data, but localized names, addresses, text, etc
Mock
This allows you to replace parts of your system with mock objects
(Examples)
Things that should be tested
Text
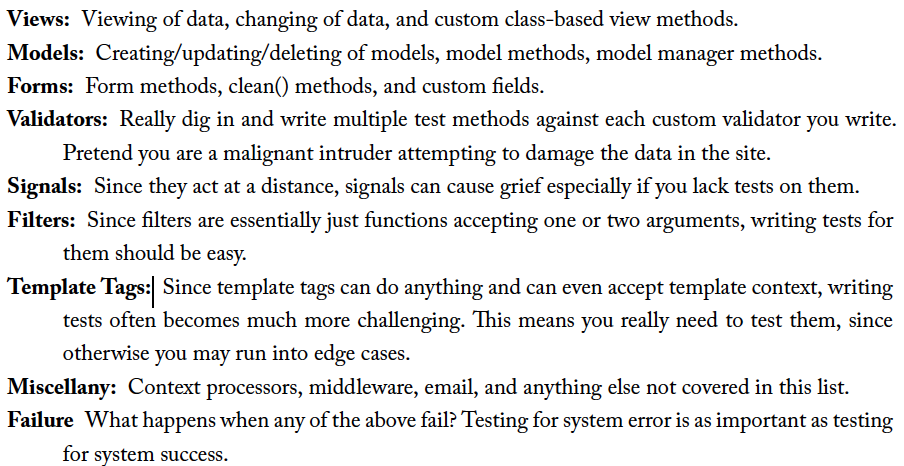
Use Fancier Assertion Methods
Comparing two list/tuples is a very common use case
Python and Django's unittest documentation includes good links to the very useful assertion types
What about integrations tests?
Integration testing is when individual software modules are combined and tested as a group. This is best done after unit test are complete.
Continuous Integration
For projects of any size, you can setting up a continuous integration (CI) server to run the project's test suite whenever code is commited and pushed to the project repo.
Lack of stability in a project can mean the loss of clients, contracts, and even employment.
References
- https://www.twoscoopspress.com/products/two-scoops-of-django-1-11
API Versioning
A small approach
v1
v2
v3
domain.com/api/v1/xxx
api.domain.com/v1/xxx
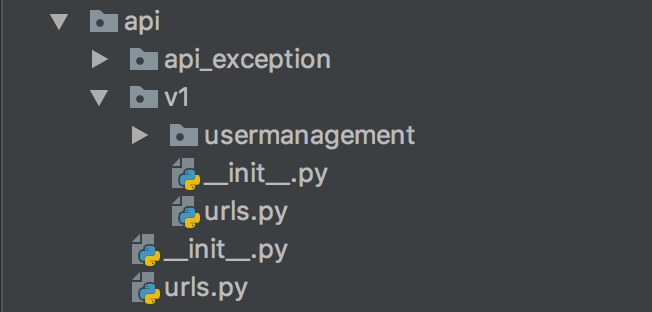
urlpatterns = [
...
url(r'^api/', include('api.urls', namespace='api')),
...
]
urlpatterns = [
...
url(r'^v1/', include('api.v1.urls', namespace='v1')),
url(r'^v2/', include('api.v2.urls', namespace='v2')),
...
]
urlpatterns = [
...
url(r'^user/$', views.create_user, name='create_user'),
...
]
# /api/v1/user
# api:v1:create_user
Thanks !
Django Rest Framework Best practices
By saduqz
Django Rest Framework Best practices
- 3,684