In Harmony with Javascript
ES6, Javascript, and You
http://slides.com/scurker/es6-javascript-and-you
scurker.com

@scurker


Jason Wilson

Text
Interaction Designer @ Daxko
ES6
Ha
R
Mo
Ny
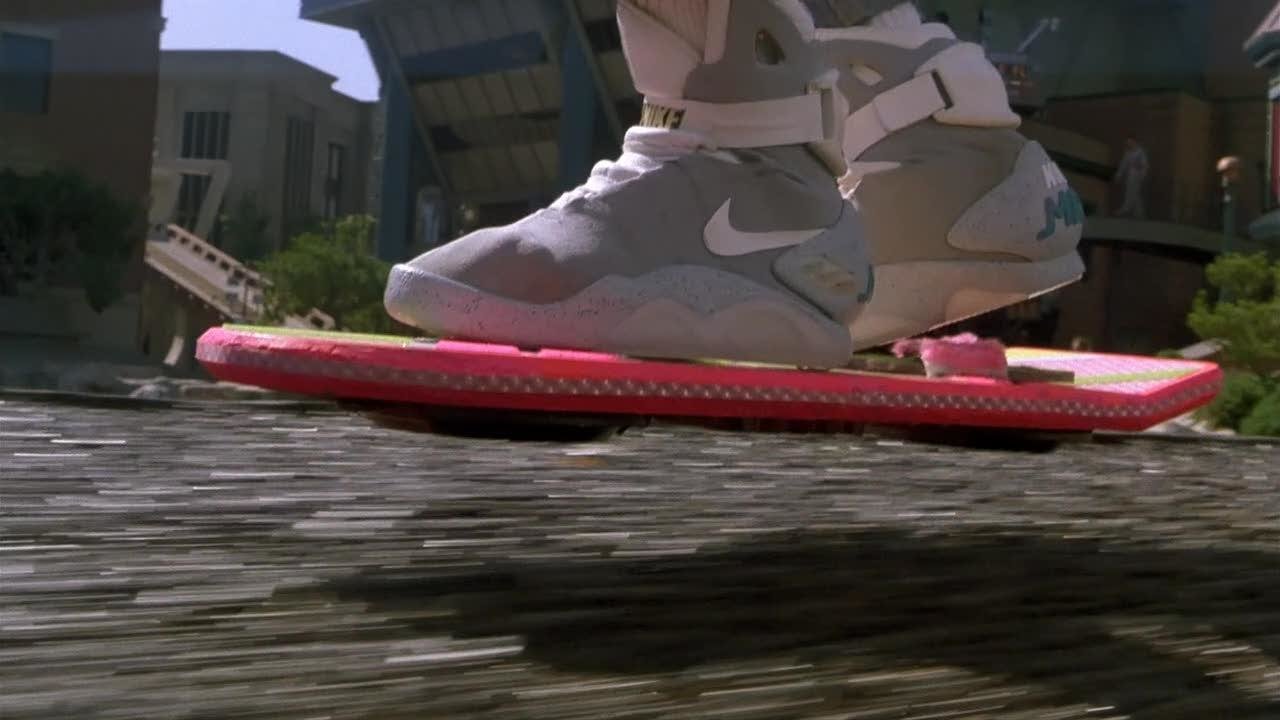
welcome to the future
(of javascript)

Arrow Functions
Arrow Functions
// Ye Old Javascript
var evenNumbers = [1,2,3,4,5,6].filter(function(num) {
return num % 2 === 0;
});
// ES6 Harmony
var evenNumbers = [1,2,3,4,5,6].filter((num) => num % 2 === 0);
console.log(evenNumbers); // => [2,4,6]
Arrow Function Scope
var favorites = {
name: "Jason",
movies: ["Inception", "The Avengers", "Groundhog Day"],
likes: function() {
this.movies.forEach(m => console.log(`${this.name} likes ${m}`));
}
}
favorites.likes();
// "Jason likes Inception"
// "Jason likes The Avengers"
// "Jason likes Groundhog Day"
Block Scoping
Block Scoping
var scoping = function() {
if(0 < 1) {
var a = 1;
let b = 2;
}
console.log(a);
console.log(b);
}
scoping();
// 1
// ReferenceError: b is not defined
Global Scope
var a = 1;
function() {
var b = 2;
if(true) {
let c =3;
}
}
Functional Scope
Block Scope
Const
Const
const numbers = [4, 8, 15, 16, 23, 42];
numbers = [0];
console.log(const);
// => [4, 8, 15, 16, 23, 42]
String Templates
String Templates
var suffix = 'tel';
console.log(`Ho${suffix}, Mo${suffix}, Whatcha gonna do today?`);
// Hotel, Motel, Whatcha gonna do today?
console.log(`1 + 2 = ${1 + 2}`);
// 1 + 2 = 3
Multi-line Strings
// Ye Old Javascript
var mystring = "this is \n a multiline string"
// ES6 Harmony
var mystring = `this is
a multiline string`;
console.log(mystring);
// this is
// a multiline string
Function Parameters
Default Parameters
function add(x, y=0) {
return x + y;
}
add(1);
// => 1
add(2, 3);
// => 5
Rest Parameter
function add(a, ...values) {
return a + values.reduce((x, y) => x + y);
}
add(1);
// => 1
add(1, 2, 3);
// => 6
add(1, 2, 3, 5);
// => 11
Spread Operator
// Array Literal
[...values, 1, 2, 3];
// Better apply
function foo(x, y, z) {}
var args = [1, 2, 3];
f.apply(null, args);
// ES6
f.apply(...args);
Promises

Welcome to...
CALLBACK HELL
.then()
is the new callback hell
A(+) Promise
var promise = new Promise(function(resolve, reject) {
// do something asynchronously
foo(function(ok) {
if(ok) {
resolve("It's good!");
} else {
reject("Nope!");
}
});
return promise;
});
.then()
Promise.then(function(result) {});
Promise.catch(function(err) {});
Chaining
function doSomethingAsync() {
return new Promise(function(resolve, reject) {
async(function() {
resolve("success!");
});
}
}
doSomethingAsync()
.then(function(result) {
// do something with result
})
.then(function(result) {
// do something else result
})
.then(function(result) {
// do another thing, don't think about it
})
.then(function(result) {
console.log('all done!');
});
Multiple Promises
function doSomethingAsync() {
return new Promise(function(resolve, reject) {
async(function() {
resolve("success!");
});
}
}
doSomethingAsync()
.then(function(result) {
return anotherPromise(result);
})
.then(function(result) {
// handle the result of the second promise
});
Enhanced Object Literals
(quite literally)
Shorthand Property Names
// Ye Old Javascript
var o = {
a: 'a',
b: '1',
c: {};
};
// ES6 Harmony
var a = 'a';
var b = '1';
var c = {};
var o = { a, b, c };
Shorthand Method Names
// Ye Old Javascript
var o = {
a: "foo",
toString: function() {
return this.a;
}
};
// ES6 Harmony
var o = {
a: "foo",
toString() {
return this.a;
}
};
Computed Property Names
var whatever = 'string';
var o = {
a: "foo",
['to' + whatever.toUpperCase()]: function() {
return this.a;
}
};
Some Things I Left Out
- Classes
- Generators
- Destructuring
- Enhanced Iterators
- Modules
- Maps and Sets
- Proxies
- More Math
- ...and more
Check out https://github.com/lukehoban/es6features
ES6 Harmony Today


(no, it's actually not IE)

Supports minimal ES6 features :(

Most ES6 Harmony Features are in the Preview Release
IE11 does not support most everything without shims
See https://status.modern.ie/?term=es6



Firefox 25+

node 0.11+ (with flags)
--harmony_generators
--harmony_modules
--harmony_proxies
--harmony_scoping
--harmony_collections
--harmony_iteration

Most ES6 features are available
--es_staging
Classes & Object Literals available with
Check out https://kangax.github.io/compat-table/es6/
For Full Compatibility Tables
Polyfills & Transpilers
Polyfills (Shims)
Fill in the gaps
- Corejs - https://github.com/zloirock/core-js
- es6-shim - https://github.com/paulmillr/es6-shim/
Transpilers
- Babel - https://babeljs.io/
- Traceur - https://github.com/google/traceur-compiler
Compiles ES6 into ES5 compatible code
Thanks!
scurker.com

@scurker


ES6, Javascript and You
By scurker
ES6, Javascript and You
- 2,679