Polymorphism
- Principle of OOP
- What is Polymorphism?
- Code improvement using polymorphism
- Polymorphism in SOLID principle
- Polymorphism in Design Pattern
Principles of OOP
Encapsulation
Abstraction
Inheritance
Polymorphism
Recap
What is Inheritance?
child class can reuse attribute and method in parent class

Without Inheritance

Inheritance
What is Polymorphism?
- the ability to take different forms
- use same method but do differently
many
forms



Animal = [
]
,
,
function makeSound(animal: String){
if(animal === "dog"){
console.log("bark bark bark")
}else if(animal === "cat"){
console.log("meow meow")
}else if(animal === "cow"){
console.log("moo")
}
}
function makeSound(animal: String){
switch(animal) {
case "dog":
console.log("bark bark bark");
break;
case "cat":
console.log("meow meow");
break;
case "cow":
console.log("moo");
break;
default:
break;
}
}
BEFORE
function makeSound(animal: Animal){
animal.makeSound();
}
AFTER
class Animal {
private name: String;
constructor(name){
this.name = name;
}
makeSound() {
console.log("blablabla")
}
}
class Dog extends Animal {
makeSound() {
console.log("bark bark bark")
}
}
class Cat extends Animal {
makeSound() {
console.log("meow meow")
}
}
class Cow extends Animal {
makeSound() {
console.log("moo")
}
}
const animals = [new Dog("a"), new Cat("b"), new Cow("c")];
animals.map(animal => animal.makeSound());
// bark bark bark
// meow meow
// moo

Polymorphism
https://slides.com/max_57070/programmers-quadrant#/3/2
interface Occupation {
doJob(): void;
}
class Cow {
private name: string
constructor(name: string){
this.name = name;
}
public makeSound(): void {
console.log("moo")
}
}
class SportCow extends Cow implements Occupation {
public doJob(): void {
console.log("provide bull riding")
}
}
class MilkCow extends Cow implements Occupation {
public doJob(): void {
console.log("provide milk")
}
}
class MeatCow extends Cow implements Occupation {
public doJob(): void {
console.log("provide meat")
}
}
const cowOccupations = [new SportCow("a"), new MilkCow("b"), new MeatCow("c")];
cowOccupations.map(occupation => occupation.doJob());

IMPROVE CURRENT CODE USING
POLYMORPHISM
Which SOLID principle used polymorphism?
S - Single Responsible Principle
O - Open Closed Principle
L - Liskov Substitution Principle
I - Interface Segregation Principle
D - Dependency Inversion Principle
Example of design pattern used polymorphism
Strategy Pattern
Factory Pattern


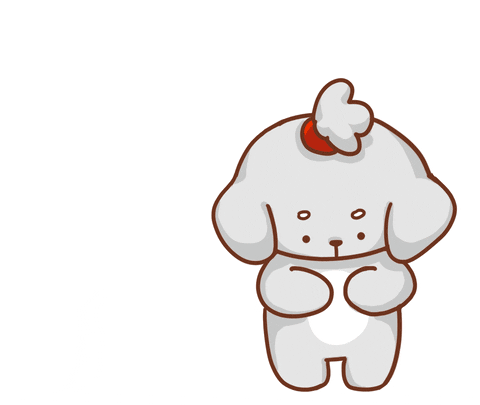
polymorphism
By shirlin1028
polymorphism
- 148