Why and How to do Server Side Rendering?
Shota Papiashvili
Nov 17, 2018
About Me
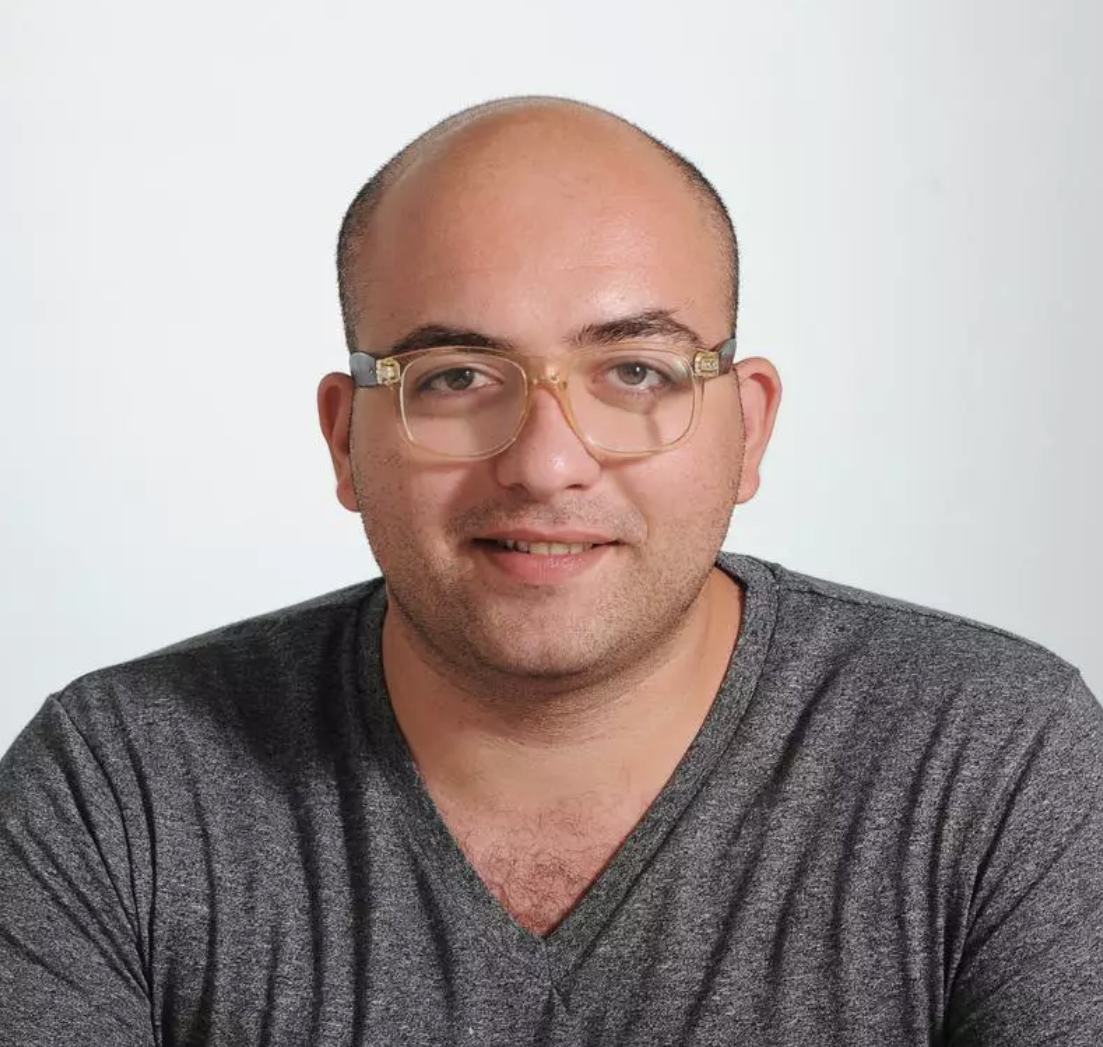
Tel Aviv, Israel 🇮🇱
Head of Web
and Architect @
Shota Papiashvili
About Me
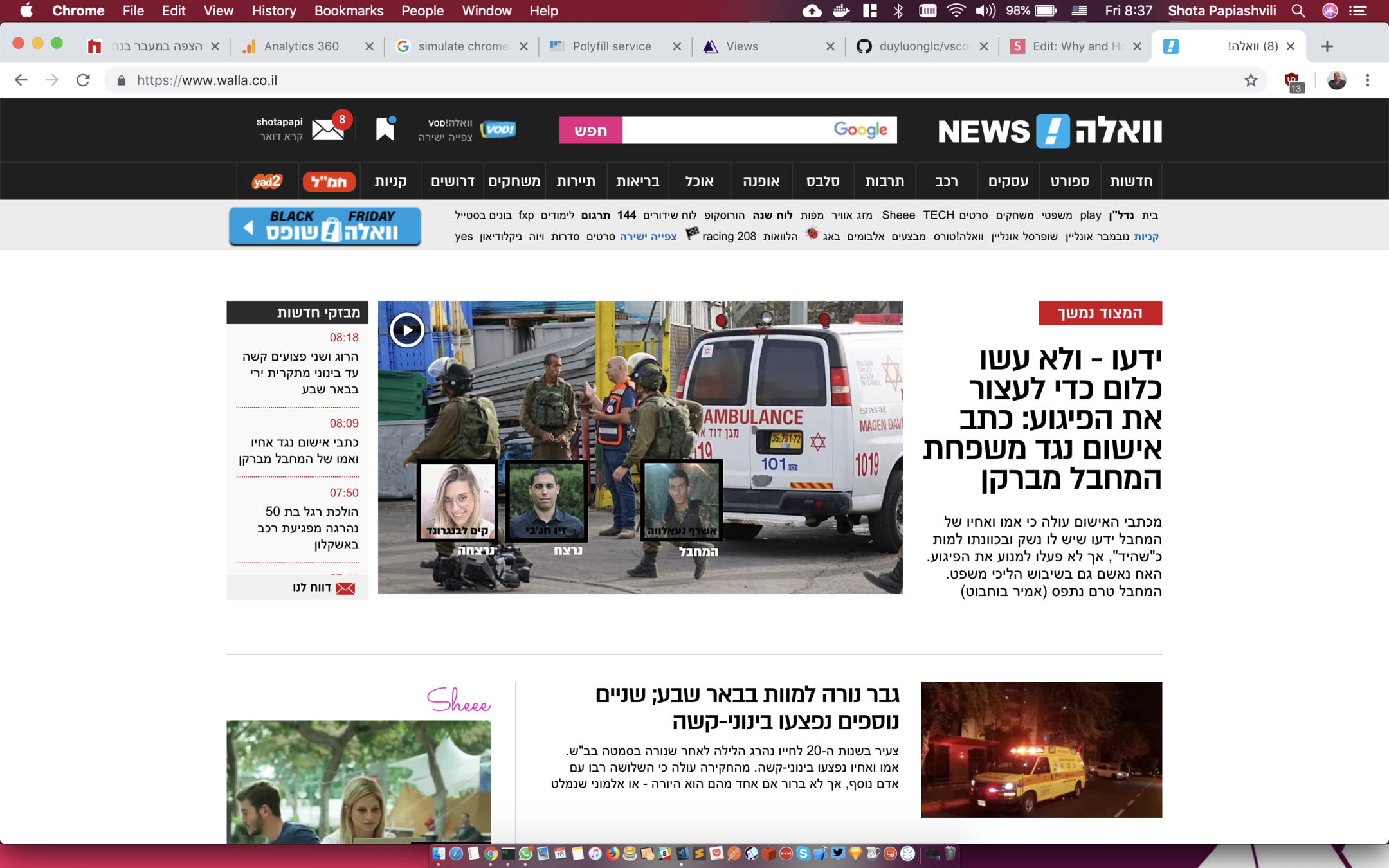
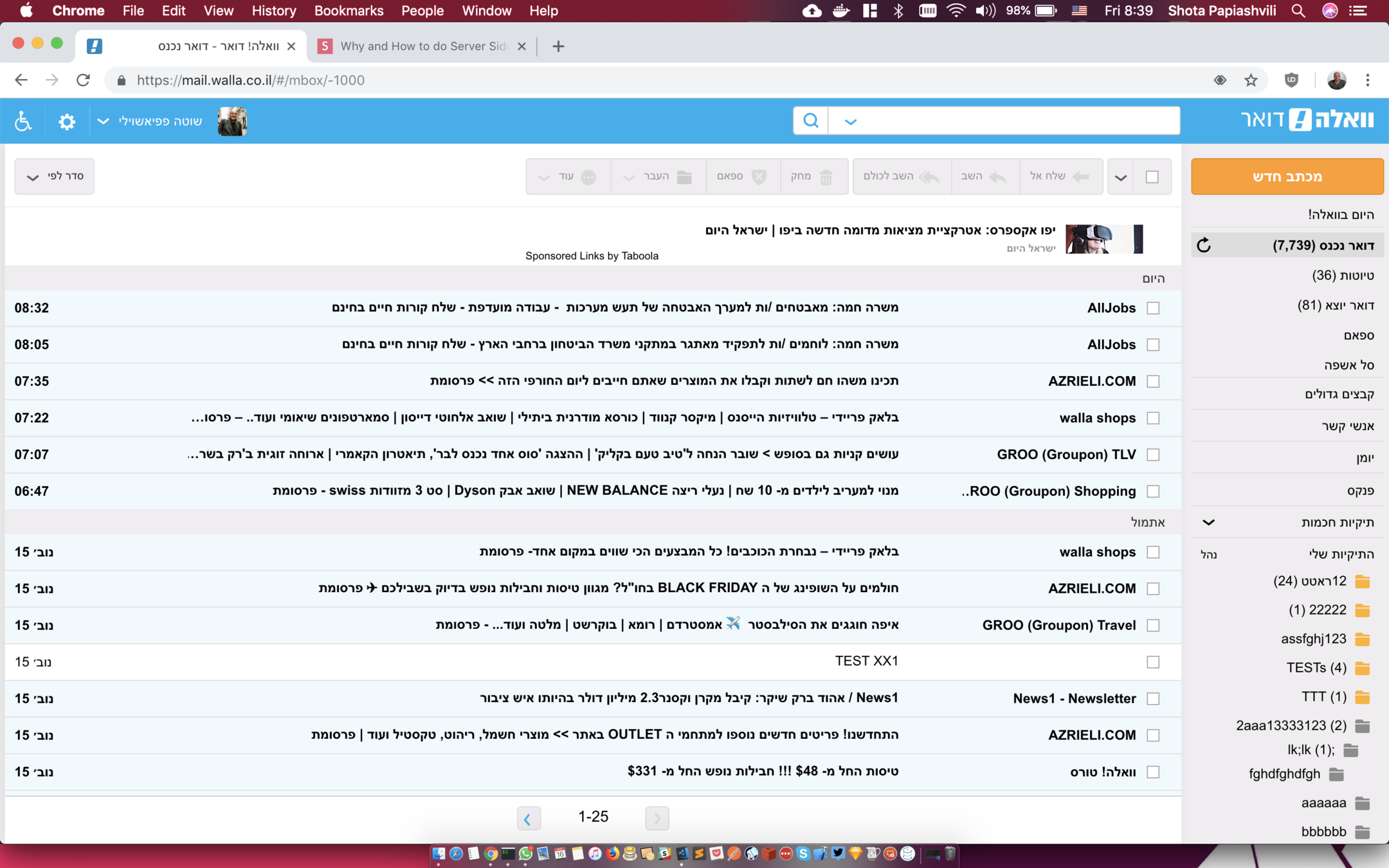
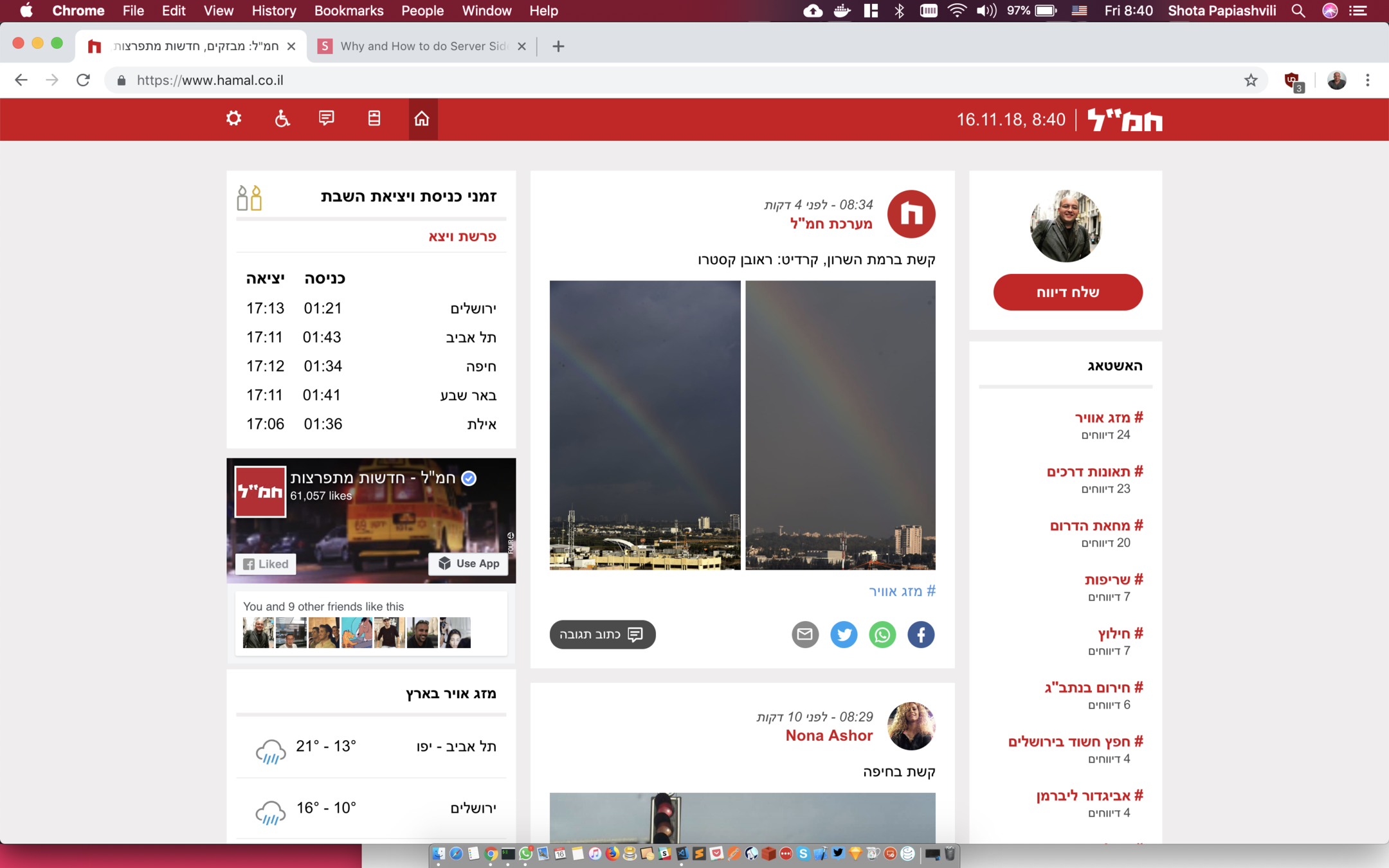
Agenda
- What is Server Side Rendering (SSR)
- SSR vs CSR
- Why use SSR?
- Rendering and GoogleBot
- SSR Disadvantages
- How to SSR - React example
- Dynamic Rendering (DR)
- Why DR?
- How to DR
What?
Server Side Rendering (SSR) refers to the process of taking a client-side JavaScript Framework website and rendering it to static HTML and CSS on the server
SSR vs CSR

CSR
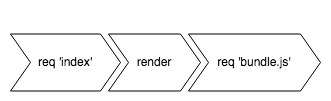
SSR
Why?
- SEO
- FMP - First Meaningful Paint
- TTI - Time to Interactive
Why? - SEO
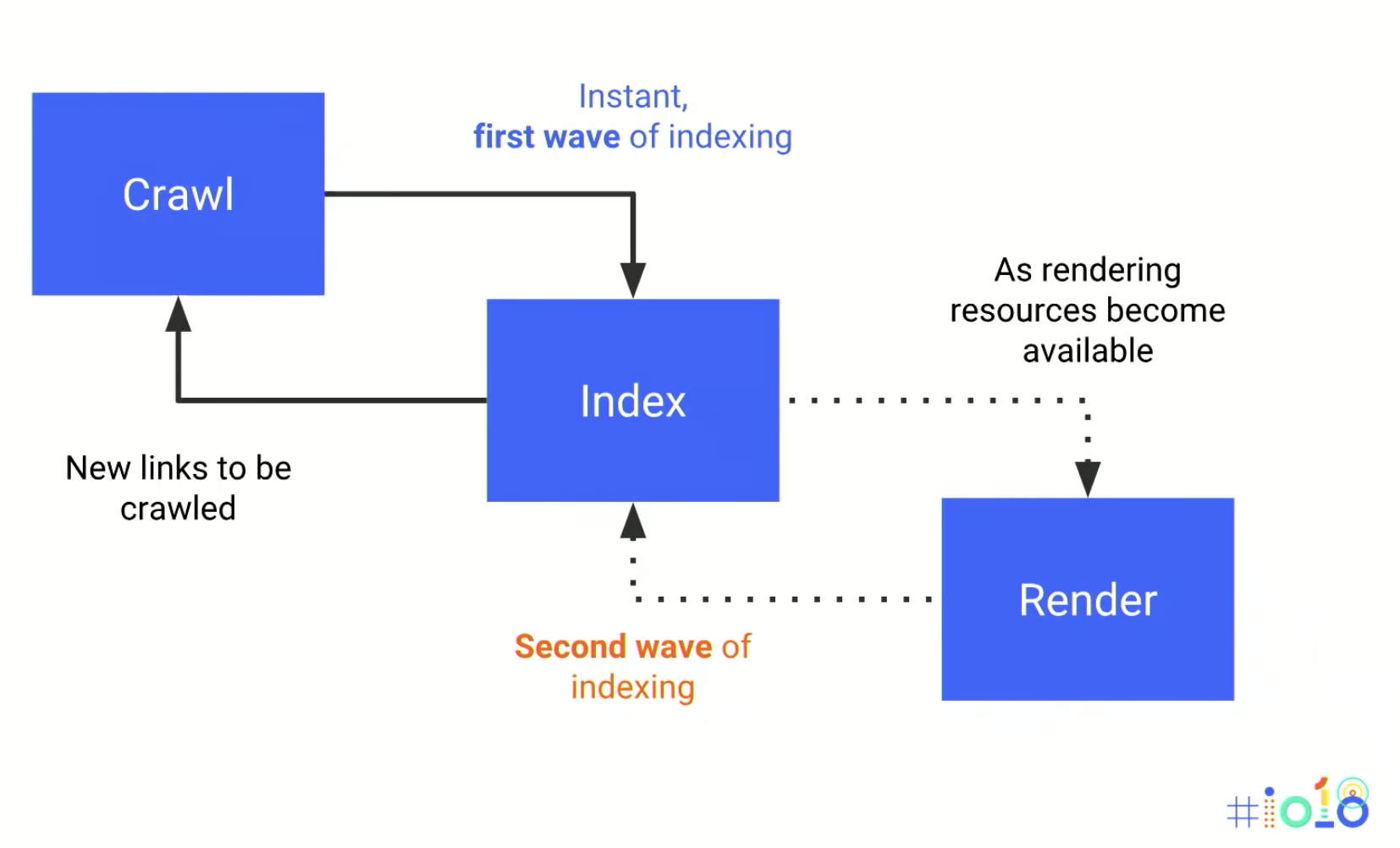
If your website uses CSR google will split the indexing process to 2 steps that can be days apart and some data will be lost acording to google (like canonical tags)
Why? - SEO


Chrome 41 was released 3 years ago, some of the fancy features of the JS frameworks wont work on this version
Why? - FMP
The First Meaningful Paint asks us to time how long it takes for the user to see primary content instead of just a nav bar.
Why? - TTI
Time to Interactive is defined as the point at which layout has stabilized, key webfonts are visible, and the main thread is available enough to handle user input
Why?
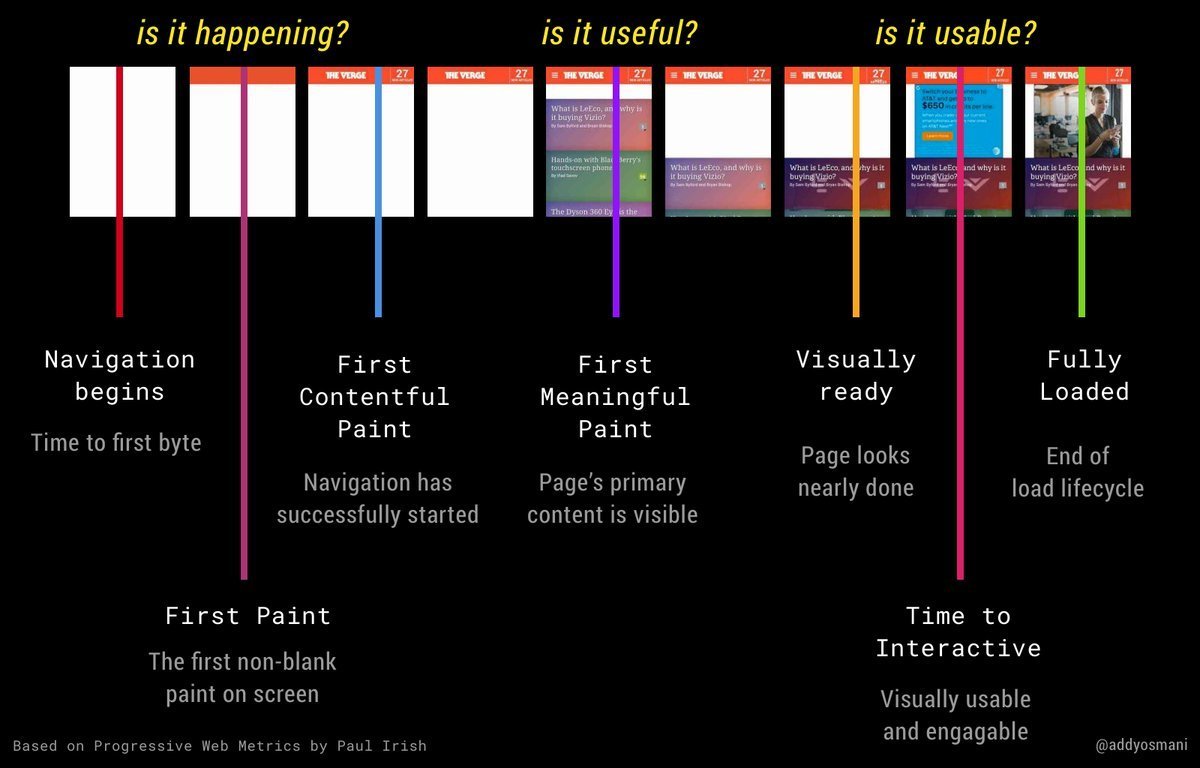
Disadvantages
- You need a real web server and can not use S3 hosting/Firebase hosting
- Cost - rendering costs a lot of compute power
- Its hard in scale
- No real official boilerplate
- Server side must be Node.js
Where?
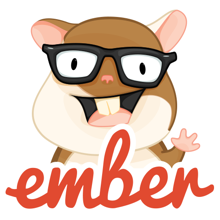
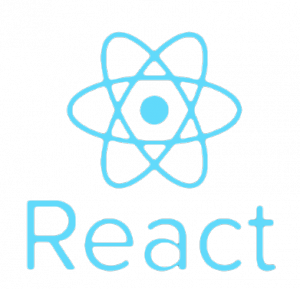
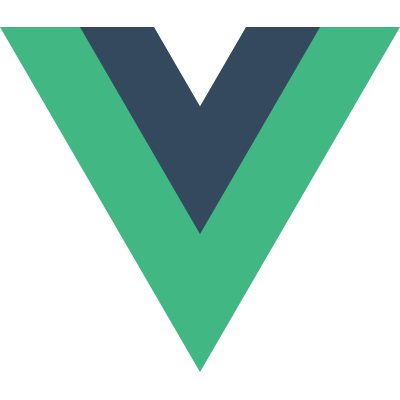
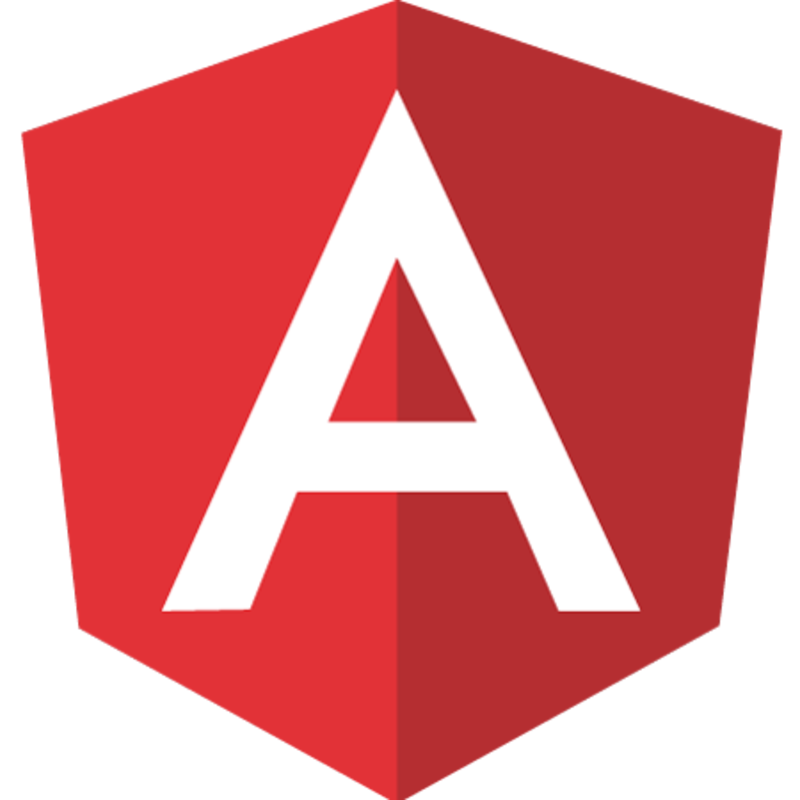
How?
// src/shared/App.js
import * as React from 'react';
const App = (props) => {
return (
<div>Hello World! 🌷</div>
);
};
export default App;
How?
import express from 'express'
import { renderToString } from 'react-dom/server'
import App from '../shared/App'
import React from 'react'
const app = express()
app.get("*", async (req, res) => {
const markup = renderToString(<App />)
res.send(`
<!DOCTYPE html>
<html>
<head>
<title>SSR</title>
</head>
<body>
<div id="app">${markup}</div>
<script src="/bundle.js"></script>
</body>
</html>
`)
})
app.listen(4200, () => console.log(`Server is listening on port: 4200`))
How?
// src/client/index.js
import React from 'react'
import { hydrate } from 'react-dom'
import App from '../shared/App'
hydrate(
<App />,
document.getElementById('app')
);
If you call ReactDOM.hydrate() on a node that already has this server-rendered markup, React will preserve it and only attach event handlers, allowing you to have a very performant first-load experience.
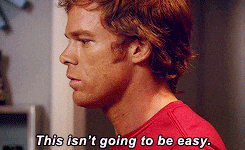
When we fetch data?
Who handles routing?
Dynamic Rendering
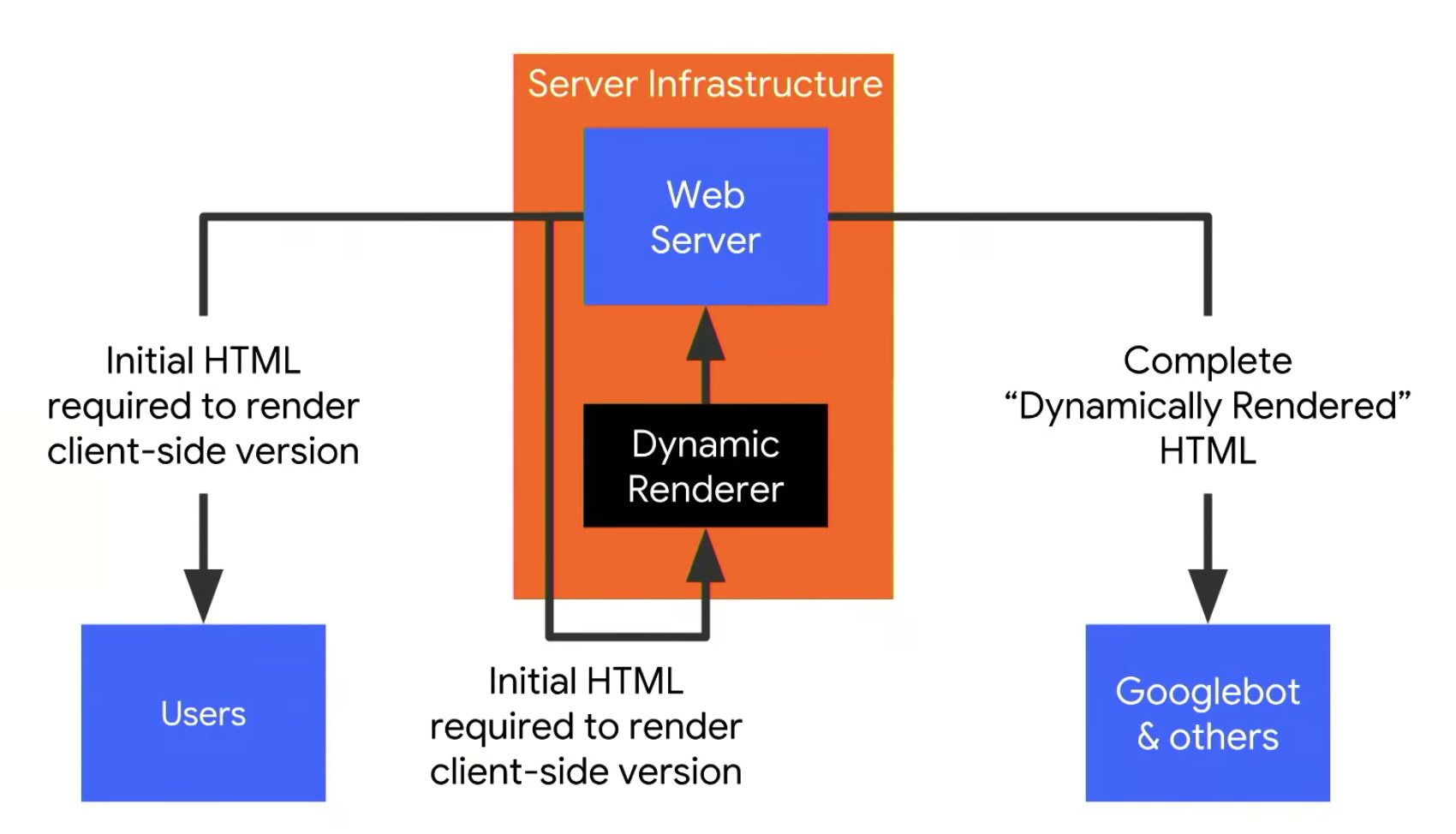
Recommended by Google @ IO18
How?
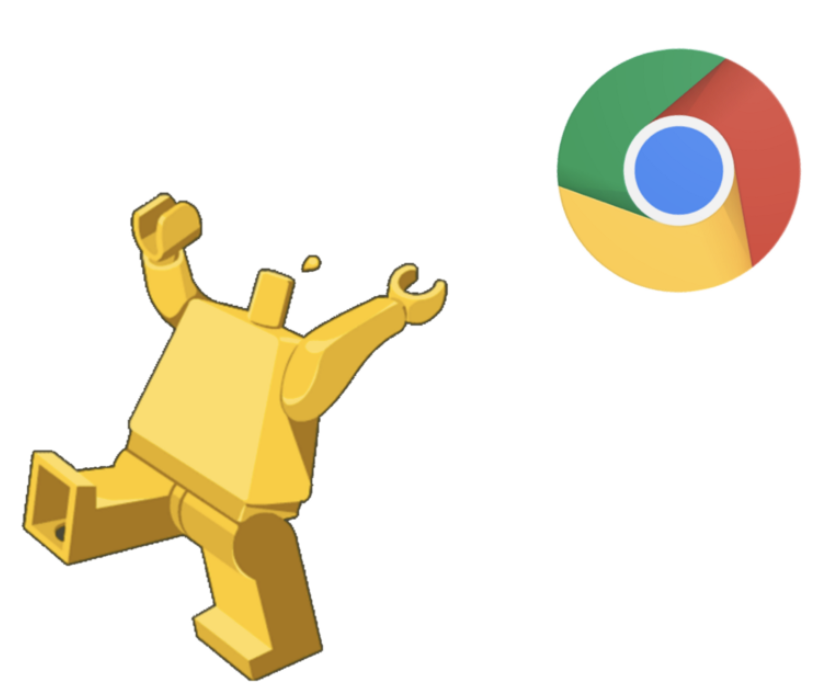
Headless Chrome is shipping in Chrome 59. It's a way to run the Chrome browser in a headless environment. Essentially, running Chrome without chrome! It brings all modern web platform features provided by Chromium and the Blink rendering engine to the command line."
How?
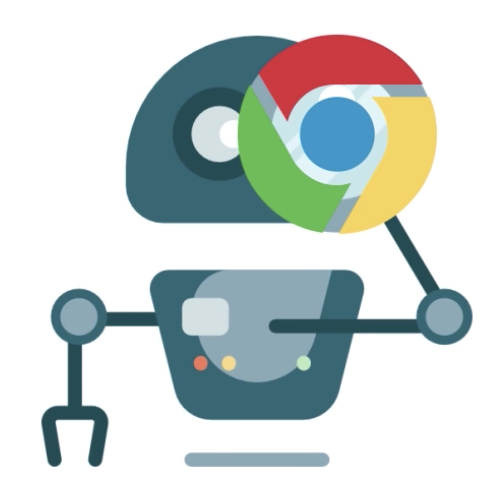
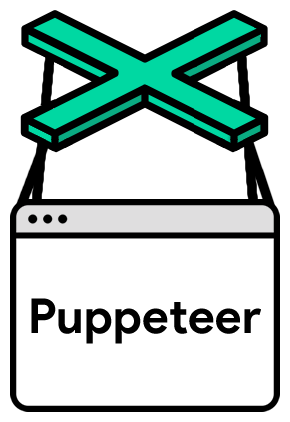
Rendertron
Why?
- EASY, EASY, EASY
- You can choose to apply it only for bots (google, facebook, ...)
- Regular JS code
- You can use browser only objects (window, document)
- Easy to migrate old apps
Where?
Everywhere!
This approach is totally agnostic to the client framework, you can change client FW without any effect on the DR
How?
const express = require('express');
const app = express();
const puppeteer = require('puppeteer');
app.get('*', async (req, res) => {
const browser = await puppeteer.launch({headless: true});
const page = await browser.newPage();
const local_url = 'http://localhost:8080' + req.originalUrl;
await page.goto(local_url, {
waitUntil: "networkidle0",
});
const html = await page.evaluate(() => {
return document.documentElement.innerHTML;
});
res.send(html);
});
app.listen(3000, () => console.log(`Server is listening on port: 3000`))
Thank You!
Shota Papiashvili
Nov 17, 2018
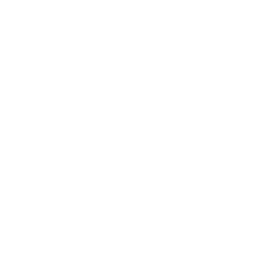
@shotapa shotap
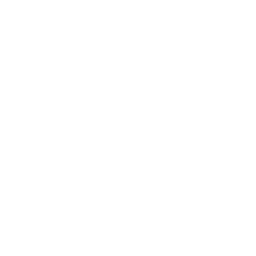
❤️🇧🇬
Why and How to do Server Side Rendering?
By Shota Papiashvili
Why and How to do Server Side Rendering?
We will talk about why to do server side rendering, what we can achieve with that and some best practices for the different frameworks and approaches
- 1,092