Node.js
But first..
..a quick JavaScript refresher
let / const
const a = 5
a = 6 // nope!! "TypeError: Assignment to constant variable."
let b = "hey"
b = "yeh"
{
const c = a + b
console.log({ c }) // prints { c: "5yeh" }
}
console.log({ c }) // "ReferenceError: c is not defined"
// (JS can't make up it's mind about periods in Error messages)
arrow functions
function traditionalFunction(a, b) {
// `this` and `arguments` implicitly defined
}
const arrowFunction = (a, b) => {
// nothing special here.. just variables from surrounding closures
}
const implicitReturn = () =>
"without curlies, arrow functions implicitly return their expression"
const add = a => b => a + b
add(1)(7) // 8
const add3 = add(3)
add3(5) // 8
const subtract = a => b => a - b
classes
class Point {
constructor(x, y) {
this.x = x
this.y = y
}
distance(point) {
const a = this.x - point.x
const b = this.y - point.y
return Math.sqrt(omg don't use classes to store data)
}
static clone(point) {
return new Point(point.x, point.y);
}
}
whew.. that's better
const p1 = { x: 5, y: 7 }
const p2 = { x: -1, y: 2 }
const distance = (left, right) => {
const a = left.x - right.x
const b = left.y - right.y
return Math.sqrt(a ** 2 + b ** 2)
}
const clonedPoint = { ...p2 }
rest / spread
const p1 = [ 1, 2, 3, 4 ]
const p2 = [ ...p1 ]
const sum = (...numbers) => numbers.reduce((t, n) => t + n, 0)
sum(1, 3) // 4
sum(5, 5, 2, 7) // 19
const [ head, ...tail ] = p2
const user = { name: "someone", email: "someone@email.com" }
const { email, ...otherUserInfo } = user
const PracticalComponent = ({ name = "unknown", ...otherProps }) =>
<div>
<h1>Hello, {name}</h1>
<UserProfile user={otherProps} />
</div>
template strings
const greet = name => `Hello, ${name || "what's your name?"}`
const literallyAnything = gql`
query {
users {
favoriteES2015Feature
}
}
`
Promises
const a = Promise.resolve(5)
const b = new Promise((resolve, reject) => {
setTimeout(() => {
resolve(10)
}, 5000)
})
const userObjP = fetch("/user").then(res => res.json())
userObjP
.then(user => console.log("current user: ", user))
.catch(err => console.error("failed to fetch user: ", err))
async / await
const logUser = async () => {
try {
const res = await fetch("/user")
const user = await res.json()
console.log("current user: ", user)
} catch (err) {
console.error("failed to fetch user: ", err)
}
}
modules
import App from "./components/app"
import { log, randomFn } from "./utils"
export const abc = () => "abc"
export default (props) => <App {...props} />
but wait, there's more...........
- Enhanced Object Literals
- Set, Map
- WeakSet, WeakMap
- Generator functions
- Unicode
- Proxies
- Symbols
- Additions to the Math, Number, String, and Object APIs
- Binary/Octal literals
- Reflect API
- Tail calls
but wait, there's EVEN MORE
- ES2016
- ES2017
- ES2018
- ...
feel refreshed?
npm

npm
npm init # in a new directory
npm install --save micro
npm test
npm start
npm run other-scripts
package.json
{
"name": "example-project",
"version": "1.0.0",
"description": "",
"main": "src/index.js",
"dependencies": {
"micro": "^8.0.3"
},
"scripts": {
"start": "node .",
"test": "echo 'Write some tests!'"
}
}
Node.js
(again)
What is it?
- Written by Ryan Dahl in 2009
- https://www.youtube.com/watch?v=jo_B4LTHi3I
- JavaScript on a server
- JavaScript in a desktop app
- JavaScript in a command line app
But what is it?
- libuv
- V8
- Standard Library
The Event Loop
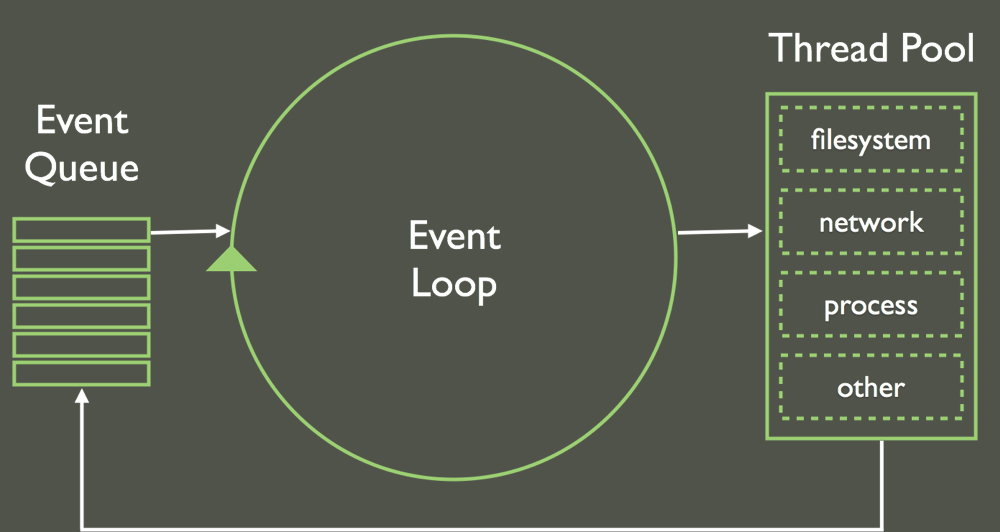
Node.js
By spicydonuts
Node.js
- 252