{Objects}
[Arrays]
Function(s){}
slides: slides.com/telegraphprep/telegraphPrepWeek1
exercises: https://github.com/telegraphPrep/week1

JS Data Types
Review

Context
Your programs rely on data.
Step 1 on your JavaScript journey is understanding the various data types and how to use them.
Once you've done this, you can move onto learning about program structure and control flow.
Then, as you write more complicated code, you'll want to take the time to learn about programming best practices like debugging and pseudocode.
Why is this important?
primitive data types
// Primitive Data Types var myString = 'hello world!'; var myInteger = 1; var myBoolean = true; var noValue = null; var notDefined = undefined; // values are immutable // (can't be changed, only overwritten)

primitives are immutable
// You can't mutate the value var myString = 'Can\'t change me'; myString[0]; //"C" myString[0] = 'Z'; myString; //"Can't change me" // You can only reassign the variable myString = 'Can overwrite me'; myString; //"Can overwrite me"
You can't change their value. To change a string, you have to create a totally new one and reassign your variable.

Mutable data types
var myObject = { key: 'value' }; var myArray = [1, 2, 3]; var myFunction = function() { return 'let\'s code!'; }; // values are mutable // (can be changed)
Note: Arrays and Functions are specialized Objects...
We'll talk more about this later in the course

Mutable data types
// You can change the value var myArray = ['apple', 'banana', 'orange']; myArray[0]; //"apple" myArray[0] = 'pear'; myArray; //['pear', 'banana', 'orange'] // You can also overwrite it with a new value myArray = ['carrot', 'lettuce', 'cucumber']; myArray; //['carrot', 'lettuce', 'cucumber']
Note: There's a difference between mutating and overwriting.

in this class
we'll cover the mutable data types
OBJECTS
ARRAYS
FUNCTIONS
Yes, functions are treated like data...
join us in Part 2 of this class to understand this fully.

Objects
{}

Context
- fundamental JS data type
- used to store information about a given entity
- ex: 'user' object stores information about a person
Why are Objects important?

OVERVIEW
- Property Access/Assignment
- Dot Notation
- Bracket Notation
- Iteration
- Nesting objects
KEY TAKEAWAY:
use DOT NOTATION only for STRINGS
use BRACKET NOTATION with VARIABLES and NUMBERS

Why use objects?

Store information about an entity
Creating Objects
var awesomeStudent = {}; // use dot notation to add // name // location // twitter/facebook handle

Terminology
var student = {};
student.name = "Julia";

var student = { "name" : "Julia" }
|| ||
property name property value
aka key
Assignment w/ Dots
var student = {};
student.name = "Julia";

note: property names (aka keys) are 'stringified'
var student = { "name" : "Julia" }
access w/ Dots
var student = {};
student.name = "Julia";
student.name //??

var student = { "name" : "Julia" }
Access w/ Dots
var student = {"name" : "Julia"}
||
awesomeStudent
var student = {};
student.name = "Julia";
var awesomeStudent = student.name;

Overwriting
student = {"name" : "Jonathan"}
student = {"name" : "Johnny"}
var student = {};
student.name = "Jonathan";
student.name = "Johnny";

Access before Assignment
var student = {"name" : "Julia"}
var student = {};
student.name = "Julia";
student.name; //"Julia"
student.location; //??

var student = {};
student.name = "Julia";
student.name; //"Julia"
student.location; //undefined
Dot notation Constraints

You can only use dot notation if your property is an alphanumeric string that starts with a letter
bracket notation

With bracket notation there are less constraints...
The most important use cases are if the property name is a variable or a number
bracket notation

It's easy to transform from dot to bracket notation
var student = {};
// Assignment
// with dot notation
student.name = "Julia";
// with bracket notation
student['name'] = "Julia";
// Access
// with dot notation
student.name; //"Julia"
// with bracket notation
student['name']; //"Julia"
var student = {"name" : "Julia"}
The in-memory representation is exactly the same for both
dot === shorthand

Think of dot notation as being shorthand for bracket notation that can only be used when the property name is a string that starts with a letter... it's easier and faster to type
You can imagine that under the hood, the JavaScript engine will take the string that's after the dot and insert it between some brackets.
Dot => bracket
var student = {};
student. name = "Jose";

var student = {};
student 'name' = "Jose";
var student = {};
student['name'] = "Jose";
var student = {"name" : "Jose"}
Numers
var MI6Agents = {};
MI6Agents.007 = "James Bond";

var MI6Agents = {"007" : "James Bond"}

var MI6Agents = {};
MI6Agents.007 = "James Bond";
MI6Agents['007'] = "James Bond";
Variables
var student = {};
student["name"] = "Jose";
var key = "name";
student[key]; //??

Expressions
var student = {};
student["name"] = "Jose";
var func = function(){
return "name";
};
student[func()]; //??

var student = {};
student["name"] = "Jose";
var key = "name";
student['key']; //??
var student = {};
student["name"] = "Jose";
var key = "name";
student['key']; //undefined
student.key; //??
var student = {};
student["name"] = "Jose";
var key = "name";
student['key']; //undefined
student.key; //undefined
var student = {};
student["name"] = "Jose";
var key = "name";
student['key']; //undefined
student.key; //undefined
student[key]; //??
var student = {};
student["name"] = "Jose";
var key = "name";
student['key']; //undefined
student.key; //undefined
student[key]; //"Jose"
Do's && Don'ts

Keys w/Special Chars
student = { "name" : "Jose", "^&*" : "testing 123" }
var student = {};
student["name"] = "Jose";
student["^&*"] = "testing 123";
var test = student["^&*"];
student.@#! //SYNTAX ERROR

Bracket vs. Dots

THE RULES
DOTS
strings
Brackets
"strings
"quotes required
"weird characters
other primitives
variables
numbers
quotations
weird characters
expressions
expressions

KEY TAKEAWAY

Dot notation can only be used when the property name is a string that starts with a letter.
Bracket notation must be used when dealing with everything else! Such as variables or numeric property names.
Exercise Time
- What is the format for exercises?
- Pair programming?
- Can I use references?
- devdocs.io
- stack overflow
- Who are the TAs?
- When should I ask for help?
- How will they help?

Exercise Time
https://github.com/TelegraphPrep/week1

Object Keys: primitives
obj = { "string" : "foobar", "0" : "meow", "3" : 3, "true" : "bark",
"null" : "hello", "undefined" : "goodbye" }
var obj = {};
obj["string"] = "foobar";
obj[0] = "meow";
obj[true] = "bark";
obj[null] = "hello";
obj[undefined] = "goodbye";

automatically get stringified!
Object values: anything
obj = { "string" : "string", "number" : 5, "boolean" : true, "null" : null, "undefined" : undefined, "nestedObj" : { "foo": "bar" }, "array": [1,2,3], "method": function() {...} }
var obj = {};
obj.string = "string";
obj.number = 5;
obj.boolean = true;
obj.null = null;
obj.undefined = undefined;
obj.nestedObj = { foo: 'bar' };
obj.array = [1,2,3];
obj.method = function() { ... }

Storing Other Objects
var box = {};
box["material"] = "cardboard";
box["size"] = {
"height": 2,
"width": 80
};
box.area = function(){
return box.size.height
* box.size.width;
};
box = { "material" : "cardboard", "size" : { "height": 2, "width": 80 }, "area" : fn() {...}
}

Object Literal
var box = {
"area" : function() {...},
"material" : "cardboard",
"size" : {
"height" : 2,
"width" : 80
}
};
var box = {
area : function() {...},
material : "cardboard",
size : {
height : 2,
width : 80
}
};

Key takeaways

- Property names are stringified
- Property values can be any JavaScript type
- when a value is an object, this is called "nesting"
- Only use dot notation when property name is an alphanumeric string
- Bracket notation must be used when doing property access in a for loop
What is an object?
What is the difference between dot and bracket notation?
When can you only use brackets and not dot?
When can you only use dot notation and not bracket?
How do you add a property with a key that contains special characters?
How do you add a property with a key that is stored in a variable?
How do you add a property whose key and value are stored in different variables?
How do you access an object that is inside another object?
How do you create an object that is nested inside another object?










Exercise time
Arrays
[]

Context
- fundamental JS data type
- used to store collection of values
- ex: 'users' array stores list of 'user' objects
Why are Arrays important?
Overview
- Arrays vs Objects
- Access && Assignment
- Native Properties

Arrays vs Objects
- Objects store information for a given entity (ex: person)
- Arrays work best for storing lists of values (ex: student names)
- Arrays store elements in a numbered index
- Arrays have built-in properties and methods to make it easier to work with lists of values
- Like objects, arrays can store any type of JavaScript value

Array creation

var students = ['Julia', 'Johnny', 'Jose'];
Arrays are good for storing lists of values
Welcome: Zero Indexing

The two hardest problems in programming are:
1. Cache invalidation
2. Naming things
3. Off-by-one errors.
numeric indexes

var students = ['Julia', 'Johnny', 'Jose'];
var students = [ 'Julia' , 'Johnny' , 'Jose' ];
index #: 0 1 2
Array access

var students = ['Julia', 'Johnny', 'Jose'];
students[0]; //'Julia'
students[4]; //undefined
var students = [ 'Julia' , 'Johnny' , 'Jose' ];
index #: 0 1 2
var students = ['Julia', 'Johnny', 'Jose'];
students[0]; //'Julia'
students[4]; //???
var students = ['Julia', 'Johnny', 'Jose'];
students[0]; //???
students[4]; //???
We use BRACKETS!!
overwriting

var students = ['Julia', 'Johnny', 'Jose'];
students[1]; //'Johnny'
students[1] = 'Jonathan';
students[1]; //??
var students = [ 'Julia' , 'Johnny' , 'Jose' ];
index #: 0 1 2
var students = ['Julia', 'Johnny', 'Jose'];
students[1]; //'Johnny'
students[1] = 'Jonathan'
var students = ['Julia', 'Johnny', 'Jose'];
students[1]; //'Johnny'
var students = ['Julia', 'Johnny', 'Jose'];
students[1]; //'Johnny'
students[1] = 'Jonathan';
students[1]; //'Jonathan'
Array assignment

var students = [ 'Julia' , 'Johnny' , 'Jose' , 'Jackie' ];
index #: 0 1 2 3
var students = ['Julia', 'Johnny', 'Jose'];
// Add Jackie to the end of the array
students[???] = 'Jackie';
var students = ['Julia', 'Johnny', 'Jose'];
// Add Jackie to the end of the array
students[ 3 ] = 'Jackie';
Bracket notation

Note that we have been using bracket notation (with index number) for:
- element access
- assigning new values to index positions
Just like for objects, you can't use dot notation for numbers... so you must use bracket notation.
There is a lot of overlap for rules with arrays and objects. We'll go deeper into this later.
native length property

var students = [ 'Julia' , 'Johnny' , 'Jose' ];
index #: 0 1 2
var students = ['Julia', 'Johnny', 'Jose'];
students.length; //??
var students = ['Julia', 'Johnny', 'Jose'];
students.length; //3
native push method

var students = [ 'Julia' , 'Johnny' , 'Jose' , 'Jackie'];
index #: 0 1 2 3
var students = ['Julia', 'Johnny', 'Jose'];
students.length; //3
students.push('Jackie');
var students = ['Julia', 'Johnny', 'Jose'];
students.length; //3
students.push('Jackie');
students.length; //??
var students = ['Julia', 'Johnny', 'Jose'];
students.length; //3
students.push('Jackie');
students.length; //4
native pop method

var students = [ 'Julia' , 'Johnny' ];
index #: 0 1
length: 1 2
var students = ['Julia', 'Johnny', 'Jose'];
students.length; //3
students.pop(); //'Jose'
var students = ['Julia', 'Johnny', 'Jose'];
students.length; //3
students.pop(); //'Jose'
students.length; //??
var students = ['Julia', 'Johnny', 'Jose'];
students.length; //3
students.pop(); //'Jose'
students.length; //2
What's the relationship between the length and the highest occupied index?
Arrays have order

var sortedStudents = [ 'Johnny' , 'Jose' , 'Julia' ];
index #: 0 1 2
var students = ['Julia', 'Johnny', 'Jose'];
var sortedStudents = students.sort();
The numeric indexes mean that the elements in the collection are ordered
They are often used to hold sorted information:
Access using variables

var students = [ 'Julia' , 'Johnny' , 'Jose' ];
index #: 0 1 2
var students = ['Julia', 'Johnny', 'Jose'];
// What is the last element in the array?
// Assume you don't know the element of the last item
// Do not remove the element
// (i.e. you can't use pop)
var students = ['Julia', 'Johnny', 'Jose'];
// What is the last element in the array?
// Assume you don't know the element of the last item
// Do not remove the element
// (i.e. you can't use pop)
// Last occupied index
var lastIndex = students.length-1;
var students = ['Julia', 'Johnny', 'Jose'];
// What is the last element in the array?
// Assume you don't know the element of the last item
// Do not remove the element
// (i.e. you can't use pop)
// Last occupied index
var lastIndex = students.length-1;
students[lastIndex]; //'Jose'
var students = ['Julia', 'Johnny', 'Jose'];
// What is the last element in the array?
// Assume you don't know the element of the last item
// Do not remove the element
// (i.e. you can't use pop)
// Last occupied index
var lastIndex = students.length-1;
students[lastIndex]; //'Jose'
// You'll also see this:
students[students.length-1]; //'Jose'
Array Element TYPES

var stuff = ['foobar', 4, true];
Values can be any JavaScript Type
var stuff = ['foobar', 4, true];
var moreStuff = [null, undefined];
var stuff = ['foobar', 4, true];
var moreStuff = [null, undefined];
var coordinates = [ [0,0], [0,1], [1,0], [1,1] ];
var stuff = ['foobar', 4, true];
var moreStuff = [null, undefined];
var coordinates = [ [0,0], [0,1], [1,0], [1,1] ];
var students = [ { name: 'Julia' }, { name: 'Jose' } ];
Array Review

Find the largest number in [ 3, 7, 4, 9, 1]
var set = [ 3, 7, 4, 9, 1];
// what native method can we use to help us out?
var set = [ 3, 7, 4, 9, 1];
// what native method can we use to help us out?
var sortedSet = set.sort();
var set = [ 3, 7, 4, 9, 1];
// what native method can we use to help us out?
var sortedSet = set.sort();
// what native property can we use to find the maximum?
var set = [ 3, 7, 4, 9, 1];
// what native method can we use to help us out?
var sortedSet = set.sort();
// what native property can we use to find the maximum?
var max = sortedSet[sortedSet.length-1];
sortedSet: [ 1, 3, 4, 7, 9 ]
Key takeaways

-
Arrays are used for storing collections of values
- keys are numbers in an index, making arrays useful for storing ordered lists of values
- have built in native properties (length) and methods (push and pop)
- property access and assignment works the same as for objects
- since keys are numbers use bracket notation
What are some different methods of adding values to an array?
What are some special properties about arrays that are different from objects?
What are some ways to access values in your array?
When would you use an array over an object?





Exercise Time
https://github.com/TelegraphPrep/week1
Array Exercises
More Pair Programming!!

Control flow
For Loops & Conditionals (if/else)

Context
Execution of code depends on a choice being made as to which path should be followed.
What is Control Flow?
Code rarely runs like this: 1st line, 2nd line, 3rd line, ... last line
Some lines are run more than once (loops)
Some lines are skipped entirely (conditional statements)
Context
useful for when you need to do something for every element in a collection (object or array)
Why are loops important?
Why are if statements important?
useful for when you only want to do something if a certain condition is true
ex: add 10 to every number in an array
ex: if age > 21, add to guest list
control flow
There are a few programming constructs that impact the order in which our code is run.

Generally code runs from top to bottom, but when the JavaScript interpreter encounters the following, it gets a bit more complicated...
We'll cover:
- for loops
- "for-i" loop (arrays)
- "for-in" loop (objects)
- if statements
- functions
... and more interesting!! We able to do more powerful things.
iteration (loops)
Objects store information about an entity.
Arrays store collections of values.

What for?
Loops allow us to take a look at each of the properties inside of our storage objects.
Within the loop we can do the normal property access and assignment stuff.
Types of For Loops
Arrays and Objects each get their own special for loop.
Occasionally you can get results that look somewhat correct when you use the wrong loop- DON'T EVER DO THIS!!!
They each have their own loop for a reason.
//ARRAYS
for( var i = 0; i < arr.length; i++) {
//do things...
}
//OBJECTS
for( var key in obj) {
//do things...
}

Array For loops
Arrays are numerically indexed.
This means you can access the values stored in an array by accessing a numbered position in that array.
var arr = ['pirate','dragon','unicorn'];
for( var i = 0; i < arr.length; i++) {
//do things...
}
var arr = [ 'pirate' , 'dragon' , 'unicorn' ];
index #: 0 1 2
Array For loops
The code in between the curly braces is called the body of the for loop.
var arr = ['pirate','dragon','unicorn'];
for( var i = 0; i < arr.length; i++) {
//this is the body of the loop
//the code in here gets executed once
//for each iteration
}

Array For loops
Let's break down the signature of the for loop- the part in between the parens.

var arr = ['pirate','dragon','unicorn'];
for( var i = 0; i < arr.length; i++) {
//this is the body of the loop
//the code in here gets executed once
//for each iteration
}
Array For loops
INITIALIZATION
i is just a number.
The index that we want to start at
(often 0)

var arr = ['pirate','dragon','unicorn'];
for( var i = 0; i < arr.length; i++) {
//this is the body of the loop
//the code in here gets executed once
//for each iteration
}
Array For loops
CONDITION
As long as this is true, we continue with the loop.
Once it is no longer true, the loop ends.

var arr = ['pirate','dragon','unicorn'];
for( var i = 0; i < arr.length; i++) {
//this is the body of the loop
//the code in here gets executed once
//for each iteration
}
Array For loops
FINAL EXPRESSION
This is the amount by which 'i' changes each time the body of the loop is run.
Often we simply increment by 1

var arr = ['pirate','dragon','unicorn'];
for( var i = 0; i < arr.length; i++ ) {
//this is the body of the loop
//the code in here gets executed once
//for each iteration
}
var arr = ['pirate','dragon','unicorn'];
for( var i = 0; i < arr.length; i=i+1) {
//this is the body of the loop
//the code in here gets executed once
//for each iteration
}
Array For loops
All together: we instantiate a new variable i and set it equal to 0, while i is less than the length of the array, we execute the code in the loop body, and then increment i by 1.
for( var i = 0; i < arr.length; i++) {
//body
}

Array For loops
Each iteration of the for loop just executes the code inside the loop body, no matter what that code is.
var arr = ['pirate','unicorn','dragon'];
for( var i = 0; i < arr.length; i++) {
console.log('hi from inside the for loop!');
}
//'hi from inside the for loop!'
//'hi from inside the for loop!'
//'hi from inside the for loop!'

Array For loops
i is just a number, starting at 0 and increasing up to one less than the length of the array.
Remember: arrays are 0 indexed, so the length = max index+1
var arr = ['pirate','unicorn','dragon'];
for( var i = 0; i < arr.length; i++) {
console.log('i: ' + i + ', arr.length: ' + arr.length);
}
//'i: 0, arr.length: 3'
//'i: 1, arr.length: 3'
//'i: 2, arr.length: 3'

Array For loops
How do we use the number stored in the variable i to access the values in the array?
var arr = ['pirate','unicorn','dragon'];
for( var i = 0; i < arr.length; i++) {
var element = arr[i];
console.log(element);
}
//'pirate'
//'unicorn'
//'dragon'

Array For loops
How do we use the number stored in the variable i to access the values in the array?
var arr = ['pirate','unicorn','dragon'];
for( var i = 0; i < arr.length; i++) {
console.log('the valued stored at
the ' + i + 'th position of the array
is: ' + arr[i]
);
}
//'the value stored at the 0th position of the array is pirate'
//'the value stored at the 1th position of the array is unicorn'
//'the value stored at the 2th position of the array is dragon'

Yay for arrays!!

Object For loops
Objects are key indexed.
This means you can access the values stored in an object by accessing a key in that object.
var obj = {
occupation: 'pirate',
steed: 'dragon',
bestFriend: 'unicorn'
};
for( var key in obj ) {
//do things...
}

Object For loops
The syntax of object for loops isn't like anything else you'll see in JavaScript.
You just have to memorize it.
for( var key in obj ) {
//this is the loop body
}

Object For loops


var obj = {
occupation: 'pirate',
steed: 'dragon',
bestFriend: 'unicorn'
};
for( var key in obj ) {
//body
}
Let's break down the pieces.
Specify the object to loop through
Object For loops
Create a new variable called key.
For each loop iteration, this variable will hold the value of a property name.

var obj = {
occupation: 'pirate',
steed: 'dragon',
bestFriend: 'unicorn'
};
for( var key in obj ) {
//key will be assigned all of the keys in the object
// that is: 'occupation', 'steed', 'bestFriend'
}
Object For loops
This part is magic. You don't need to understand how it works. Just accept the fact that it does work (trust me, it does).
If you doubt it, test it out in your console!

var obj = {
occupation: 'pirate',
steed: 'dragon',
bestFriend: 'unicorn'
};
for( var key in obj ) {
//key will be assigned all of the keys in the object
// that is: 'occupation', 'steed', 'bestFriend'
}
Object For loops
for(var key in obj) {
//body
}
All together now: we declare a new variable called key, and tell it which object we're iterating through, then it runs the body of the loop once for each iteration.

Object For loops
var obj = {occupation: 'pirate',
steed: 'dragon', bestFriend: 'unicorn'};
for(var key in obj) {
console.log('hi from inside obj loop!');
}
//'hi from inside obj loop!'
//'hi from inside obj loop!'
//'hi from inside obj loop!'
The body of the for loop is executed once for each of the keys in the object we're iterating through.

Object For loops
var obj = {occupation: 'pirate',
steed: 'dragon', bestFriend: 'unicorn'};
for( var key in obj ) {
console.log(key);
}
//'occupation'
//'steed'
//'bestFriend'
So what is key on each iteration?

Object For loops
var obj = {occupation: 'pirate',
steed: 'dragon', bestFriend: 'unicorn'};
for( var key in obj ) {
console.log(key);
}
//'bestFriend'
//'steed'
//'occupation'
Note: we can't assume that the keys in objects are in any certain order.

Object For loops
var obj = {occupation: 'pirate',
steed: 'dragon', bestFriend: 'unicorn'};
for( var propertyName in obj ) {
console.log(propertyName);
}
//'bestFriend'
//'steed'
//'occupation'
Note: we can use any name we want for the variable that will get set equal to all the keys.

Object For loops
var unitedStatesObj = {rhodeIsland: 'tiny',
alaska: 'untamed', ohio: 'electorally relevant',
california: 'heck yes!'};
for( var state in unitedStateObj ) {
console.log(state);
}
//'rhodeIsland'
//'alaska'
//'ohio'
//'california'
You can use this to be clear about what each property name is.
Generally though, just stick with var key in obj. That's the default and is easy to understand until things get super complex.

Object For loops
var obj = {occupation: 'pirate',
steed: 'dragon', bestFriend: 'unicorn'};
for( var key in obj ) {
var value = obj[key];
console.log(value);
}
//'pirate'
//'dragon'
//'unicorn'
So how do we use these property names to access the values in an object?

Object For loops
var obj = {occupation: 'pirate',
steed: 'dragon', bestFriend: 'unicorn'};
for( var key in obj ) {
console.log('the value stored at ' + key
+ ' is: ' + obj[key]);
}
//'the value stored at occupation is: pirate'
//'the value stored at steed is: dragon'
//'the value stored at bestFriend is: unicorn'
So how do we use these property names to access the values in an object?

Yay for Object loops!!

key takeaways
LOOPS
- Use loops when you need to access all of the properties in a collection
- For arrays always use the for-with-semicolons loop
- for (var i=startIndex; i<arr.length; i=i+increment) { ... }
- Order is guaranteed because you are explicitly specifying the indices to check
- For objects always use the for-in loop
- for (var key in obj) { ... }
- Order is not guaranteed

time to practice!!
Exercises are available at:
https://github.com/TelegraphPrep/06-2016-control-flow

If statements
if('something that will evaluate to true or false') {
//body of the if statement
//this code is only executed
//if the condition is true
}
Also called conditional statements

If statements
var alwaysFalse = false;
if(alwaysFalse) {
//this code is never executed
}
Also called conditional statements

If statements
var alwaysFalse = false;
if(alwaysFalse) {
//this code is never executed
} else {
//this code is executed if the condition is false
}
if can be followed by else. This ensures that some block of code is always executed.

If statements
var alwaysFalse = false;
if(alwaysFalse) {
console.log('code was executed');
} else {
console.log('code was executed');
}
//'code was executed'
//clearly this is not a useful program
//but it shows that no matter what,
//one of the two blocks of code is executed
//because the thing inside the if parens
//will be evaluated to be either true or false
//and the if block will be executed if true
//and the else block will be executed if false
if can be followed by else. This ensures that some block of code is always executed.

If statements
var temperature = 79;
if(temperature > 80) {
console.log('too hot inside,
party in the streets!');
} else {
console.log('good biking temperature');
}
//'good biking temperature'
if can be followed by else. This ensures that some block of code is always executed.

If statements
var temperature = 79;
if(temperature > 80) {
console.log('too hot inside,
party in the streets!');
} else if(temperature < 32) {
console.log('stay indoors you fools!');
} else {
console.log('good biking temperature');
}
//'good biking temperature'
if can also be followed by else if. This lets you chain together multiple conditions.

If statements
//Things that evaluate to true or false:
'preston' === 'albrey'
8 > 5
6*2 === 12
var arr = [6,7,8,9,10];
3 < arr.length
arr[2] === 8
The thing inside the parentheses next to if must evaluate to true or false.

If statements
//always use the triple equals sign.
//double equals does some freaking weird stuff.
//please don't make me go there
//keep it easy and just use threequals
//always.
Threequals!!

If statements
//Other things that evaluate to true or false:
'hi there' //true
var arr = undefined;
arr //false
arr = [1,2,3];
arr //true
0 //false
-1 //true
"" //false
When evaluating a boolean expression (true or false), JavaScript converts everything to be a true or false value.
These are called 'truthy' and 'falsy'.

If statements
var alwaysTrue = true;
var alwaysFalse = false;
alwaysTrue && alwaysFalse //false
alwaysTrue || alwaysFalse //true
// && means both sides must be true
// || means just one side must be true
We can also chain boolean expressions together using && (and) as well as || (or).

If statements
var alwaysTrue = true;
var alwaysFalse = false;
!alwaysTrue //false
!alwaysFalse //true
//we can apply the negation operator
//directly to a truthy/falsy value
8 !== 5 //true
'preston' !== 'preston' //false
We can also negate an operator. We can use !== to mean "is not equal to".

key takeaways
IF STATEMENT
- Use if statements to run code only if a conditional statement is true
- Use optional else if statement to check multiple conditions one after another
- Use else block to execute code if none of the conditionals evaluate to true

key takeaways
CONDITIONALS
- conditional statements always evaluate to true or false
- values that are not true/false get converted based on their truthiness/falsiness
- if you need to do an equality comparison, always use '===' / '!==' rather than '==' / '!='
- chain boolean expressions using && and/or ||
- logical NOT operator (!) converts the following expression to a boolean and then returns the opposite boolean value (ex: !null returns true)
- tip: use !! to see if something is truthy or falsey (ex: !!null returns false)

time to practice!!
Exercises are available at:
https://github.com/telegraphPrep/week1
under day3LoopsAndIf.js

Functions
function(s){}


context
DRY!! (don't repeat yourself)
- encapsulate repeated code into a function
- less typing, fewer places for bugs
Readability
- use expressive names for functions
- code is more organized
Control flow
- function can be invoked wherever you want and however many times you want
Why are Functions important?
Overview
- Anatomy
- Definition vs Invocation
- Named Functions vs Anonymous
- Parameters vs Arguments
- Return Values and Side Effects


What is a function?
A set of instructions that we create.
A block of code that we write that gets executed at some other point.
We use them to control the flow of our program.

Anatomy
var add = function (a, b) {
return a + b;
};
add(1, 2);
declaration / definition
invocation / call time
< Note 'function' keyword
< Note invocation operator ()

Anatomy
var add = function (a, b) {
return a + b;
};
add(1, 2);
body

Anatomy
var add = function (a, b) {
return a + b;
};
add(1, 2);
arguments
parameters

Definition
var nameImprover = function (name, adj) {
};

Body
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};

anonymous function
function(item) {
return item * 3;
}
An anonymous function has no name after the 'function' keyword
Often used as arguments to higher order functions
(we'll go into more detail in Part 2)

Named vs Anonymous
//Anonymous function saved into a variable
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
//named function
function nameImprover(name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
}
//both work!

Invocation/Call-time
Declaring a function is like creating a recipe: you decide what the steps are, but nothing actually gets made.
Invoking a function is like baking that recipe: now that you know what the steps are, you get to actually do them!
The cool part about this is that you can pass in different ingredients to that recipe each time! And each one is run totally independently of all other times that recipe has been created.

Invocation/Call-time
//Definition/Declaration:
var breadMaker = function(ingredient) {
return 'fresh baked ' + ingredient + 'bread';
}
//Invocation:
breadMaker('banana nut');
breadMaker('guacamole');
//Putting () next to a function name means
//that you are invoking it right then.

Arguments/Parameters
//When we define a function, what that function
// takes in (it's signature) are called parameters
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
//When we invoke a function, what we pass in to
//that particular invocation are called arguments
nameImprover('preston','purple');

Arguments/Parameters
var add = function(a, b) {
return a + b;
}
add(1, 2, 3);
Note: the number of parameters and arguments don't have to match
var add = function(a, b, c) {
return a + b;
}
add(1, 2);
c = undefined
if you're curious about how to access arguments with no corresponding parameter look up the "arguments object"
parameters with no corresponding argument are undefined

key takeaways
Why are functions useful?
- code reuse - DRY!!
- allow us to control when code gets executed
- more organized and readable
Definition vs Invocation
- defining the function is just like writing out the steps
- the code doesn't get run until the function gets invoked/called
Parameters vs Arguments (function input)
- parameters are variable names specified in the definition
- arguments are values specified at invocation
Practice TIME!!
https://github.com/telegraphPrep/week1
under day4Functions.js


Return/Side Effects
//Returned value:
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
//Side Effects:
var instructorName = 'preston';
var sideEffectImprover = function(adj) {
instructorName = 'Col ' + instructorName +
' Mc' + adj + ' pants';
};

Return/Side Effects
//Returned value:
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
var returnResults = nameImprover('preston','purple');
returnResults; //'Col preston Mcpurple pants'
//Side Effects:
var instructorName = 'preston';
var sideEffectImprover = function(adj) {
instructorName = 'Col ' + instructorName +
' Mc' + adj + ' pants';
};
var sideEffectResults = sideEffectImprover('purple');
sideEffectResults; //undefined
instructorName; //'Col preston Mcpurple pants'

Why Use Side effects?
var logResults = console.log('side effects are useful');
logResults; //undefined
var addUserToDatabase = function(username, userObj) {
if(database[username] === undefined) {
database[username] = userObj;
}
};
//note that we are not returning anything here.
//we don't want to return the entire database
//and we already have the userObj since we had it
//to pass into addUserToDatabase.
//The function is clearly still useful, even though
//it doesn't return anything.

Return ends function
var totallyHarmless = function() {
return 'world peace';
launchAllNuclearMissiles();
};
totallyHarmless(); //returns 'world peace'
//does not invoke launchAllNuclearMissiles
//because that comes after the return statement
//and return statements immediately stop the
//function and end it.

tunnel- return
Since a function creates it's own local scope, the global* scope can't see anything that's going on inside that function body.
The return value is the one way for the outside world to 'tunnel into' the function and see something that's going on.
We can use side effects to save the work that a function that has done for us into a variable that's accessible in the global scope.
Or, we can return a value, directly communicating the results to whatever we have in the global scope that's listening for the results of the function.

tunnel- return
var testArr = [1,2,3,4];
var globalSum = 0;
var sumFunc = function(arr) {
for (var i = 0; i < arr.length; i++) {
globalSum += arr[i];
}
return 'string from sumFunc';
};
var returnVal = sumFunc(testArr);
console.log(globalSum); //10
console.log(returnVal); //'string from sumFunc'

Quick Review
var add = function(a, b){
return a + b;
};
add(3, 4); // ??

Quick Review
var add = function(a, b){
return a + b;
};
add(3, 4); // 7

key takeaways
Returned Values (function output)
- whatever appears after the "return" keyword gets evaluated then provided as output when the function gets invoked
- return exits out of the function, code that comes after doesn't get run
- default return value is undefined
Side Effects
- when something outside of the function scope gets affected. Examples:
- a variable in an outer scope gets changed
- browser actions - console.log, alert, prompt
Practice TIME!!
https://github.com/telegraphPrep/week1
under day4Functions.js

they're all objects
objects {},
arrays [],
function(s){}

The Rules Don't Change!

object recap
{
"name": "Julia",
"location": "Oakland, CA",
"twitter": "@julia"
}
// Object literal
var student = { name: 'Julia'};
// Dot notation
student.location = 'Oakland, CA';
// Bracket notation
student['twitter'] = '@julia';

Objects store information (properties) about a given entity
in memory representation:
Array recap
{
"0": "Julia",
"1": "Johnny",
"length": "2"
}
// Array literal
var students = [ 'Julia' ];
// Bracket notation for access/assignment
students[0]; //'Julia'
students[1] = 'Johnny';
// Dot notation for native properties
students.length; //2

Arrays store collections of values
in memory representation:
Why can't we use dot notation for these?
Array are objects!
{
"0": "Julia",
"1": "Johnny",
"length": "2"
}

Arrays are special objects
- numeric indexes
- native length property
- native methods (push, pop)
in memory representation:

Functions are Objects too!
var treasureChest = function(){
return "you can't get nothin' from me!";
};
treasureChest.treasureMap = 'twirl three times';
console.log(treasureChest.treasureMap);
//'twirl three times'

They're all objects
Anything we can do with an object, we can do with an array and function.
Examples:
Add a property to it (not super useful)
Assign a variable to point to it
Overwrite a variable with it
Pass it in as an argument to a function
Return it as a value from a function

Nesting
var box = {"innerBox" : {}}
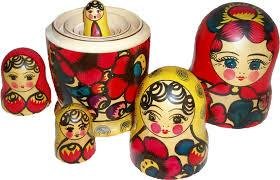
var box = {};
box.innerBox = {};
// or...
box['innerBox'] = {};

Nesting
var box = { "innerBox" : { "innerInnerBox" : {} } }
var box = {};
box.innerBox = {};
box['innerBox'].innerInnerBox = {};
box.innerBox //??

Nesting
var box = {};
box.innerBox = {};
box.innerBox.innerInnerBox = {};
// or...
box['innerBox'].innerInnerBox = {};
// or...
box['innerBox']['innerInnerBox'] = {};
var box = { "innerBox" : { "innerInnerBox" : {} } }
var box = {};
box.innerBox = {};
box.innerBox.innerInnerBox = {};
var box = {};
box.innerBox = {};
box.innerBox.innerInnerBox = {};
// or...
box['innerBox'].innerInnerBox = {};
var box = {};
box.innerBox = {};
box.innerBox.innerInnerBox = {};
// or...
box['innerBox'].innerInnerBox = {};
// or...
box['innerBox']['innerInnerBox'] = {};
// or...
var box = {
innerBox : {
innerInnerBox : {}
}
};

Nesting
var box = { "innerBox" : { "innerInnerBox" : { "full" : true } } }
var box = {};
box.innerBox = {};
box.innerBox['innerInnerBox'] = {};
var myInnerBox = box['innerBox'];
myInnerBox; //??
Jon's Copy of Week1: Objects, Arrays, Loops, andFunctions
By telegraphprep
Jon's Copy of Week1: Objects, Arrays, Loops, andFunctions
A deep introduction to JS fundamentals
- 963