Vue.js London Conference
Alex Puia
Thomas Truong
Agenda
- Animations in Vue
- Scripting in Style
- Performance
- Composition API
- Lightning talks
- Resources
- Questions
Animations in Vue

Adam Jahr
Why animate?
-
Directing focus
- Guide users how to use your app
- Grab focus of our user's attention
-
Inspiring action
- Remove distraction
- Primal human instinct
Transitions
- Vue's transition component
- Entering Transition vs Leaving Transition
- Page Transition
- Group Transition
Transition Component

Vue's Transition Component

Scripting in Style

Maya Shavin
The FEAR of CSS
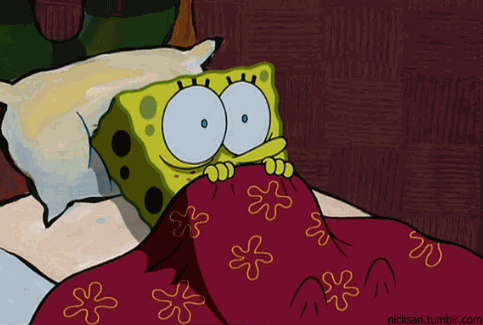
Scaling
- Global namespace
- Implicit dependencies
- Code Maintenance/DEAD code
- Minification
- Sharing Constants
- Non-deterministic order of source
Not the Bruce we deserved,
but the Bruce we need



/* darknight.css */
.bruce {
color: 'black';
}
/* smash.css */
.bruce {
color: 'green';
}
/* yippeekiyay.css */
.bruce {
color: 'red';
}
<div class="bruce">
I am Bruce
</div>
Bruce
Bruce
Bruce
!important 🤮
React Inline Style
const pStyle = {
fontSize: '15px',
textAlign: 'center'
};
const Box = () => (
<p style={pStyle}>Get started with inline style</p>
);
- Readability
- Scalability
- Animations
- Media Queries
CSS Modules
.title {
background-color: red;
}
- NOT full extendable CSS in global scope
- NAMING can still have collisions
- NO easy way for theming
- NOT really CSS-in-JS as it's in a separate file
import style from "./styles.css"
element.innerHTML = `<div class="${styles.title}"> Examples </div>`;
<div class="_styles__title_2805"> Example </div>
Styled Components
const Button = styled.button`
background: ${props => props.primary ? "white", 'black'};
`;
render(<div>
<Button primary>Primary</Buttons>
<Button>Normal</Button>
</div>)
- Creates a new class for every dynamic prop variable
-
Browser still needs to:
- Download
- Load
- Read
- Parse the CSS
- Â
- Inject into stylesheet
- HTML can paint
- No cache
Conclusion
- Use the right tool for the right job
-
CSS Modules
- ​Component
-
CSS/SCSS
- Global
IDENTIFYING AND SOLVING PERFORMANCE ISSUES IN VUE APPLICATIONS
Filip Rakowsky


What influences loading time performance

Sample Vue app modules

Sample Vue app modules

Solutions
- Code Splitting
- Lazy Loading
- Dynamic Import

Rule #1 - Split your code per route


Rule #2 - Load off-screen components lazily

Rule #3 - Load non-critical libraries lazily

Rule #4 - Choose your libraries carefully and try to find smaller equivalents if possible

Rule #5 - Prefetch lazily loaded resources

More performance wins
- Lazy load images
- Use functional components for lists
- Optimise initial state when using ServerSide Rendering
- Cache static assets in a Service Worker
Composition API
Greg Pollack
Thorsten LĂĽnborg
Jason Yu



Limitions of Vue 2
- Readability as components grow
- Code reuse patterns have drawbacks
- Limited TypeScript Support
Readability
-
Logical concerns/features are organised by component options:​
- ​Components
- Props
- Data
- Methods
- Computed Properties
- Lifecycle Methods

Readability

Setup
-
Executes before:
- ​Components
- Props
- Data
- Methods
- Computed Properties
- Lifecycle Methods
- Doesn't have access to this

Composition Functions

Mixins

- Organise by feature
- Conflict prone
- Unclear relationships
- Not easily reusable
Mixin Factories
- Easily Reusable
- Clearer Relationships
- Weak Namespacing
- Implicit property additions
- No instant access at runtime

Scoped Slots



Composition Functions Vue 3.0



Lightning Talks
How to get your product owners to write your functional tests


Focus Management

Tab Index
<h1 id="pageTitle" tabindex="-1">Tasks</h1>
Focus Directive
export default {
...,
directives: {
focus: {
inserted: function(el) {
el.focus();
}
}
}
};
-
A directive definition object can provide hook functions:
- bind
- inserted
- update
- componentUpdated
- unbind
<h2 id="newTask" tabindex="-1" v-focus>
Create New Task
</h2>
Awesome JS is Awesome

Renderless Event Component

Event Listening
<event-listener event="keyup" @fired="toggleVideo"/>
export default {
components: {
EventListener
},
methods: {
toggleVideo (e) {
if (e.code === 'Space') {
const video = this.$refs.video
video.paused ? video.play() : video.pause()
}
}
}
}
$listeners
<event-listener @keydown.space="toggleVideo"/>
export default {
components: {
EventListener
},
methods: {
toggleVideo (e) {
const video = this.$refs.video
video.paused ? video.play() : video.pause()
}
}
}
Resources
Other talks
"A new router to guide your apps"
Eduardo San Martin Morote

"D3 with Vue.js"
Ramona Biscoveanu

Slides + code
- "Animations in Vue" – Adam Jahr
- "Scripting in Style"Â - Maya Shavin
- "Performance" - Filip Rakowsky
Slides + code
Slides + code
Vue.js London Conference
By Thomas Truong
Vue.js London Conference
- 54