State Management and Vuex
A look at state management on the web.
Agenda
- What is State Management?
- Vuex
- Fun Exercises
What is State?
The state is all of the information that is retain by your application, often with respect to previous events or interactions.
What is State Management?
State management makes the state of your application tangible in the form of a data structure that you can read from and write to. It makes your invisible state clearly visible for you to work with.
Why does it matter?
State can be a messy part of application development, especially when there are a lot of user interactions to manage.
Views
Data from stores are displayed in views.
When a view uses data from a store it must also subscribe to change events from that store.
Actions
Actions define the internal API of your application.
They capture the ways in which anything might interact with your application.
Vuex
- A state management pattern and library for Vue.js applications
- It serves as a centralised store for all the components in an application
- It can only be mutated in a predictable fashion
One-way data flow

What is a State Manage Pattern?
It is a self-contained app
- The state, the source of truth
- The view, a declarative mapping of the state
- The actions, the possible ways the state could change

What is State in your App?

Without Vuex

Changing State

Default Communication

Single Source of Truth

State is Reactive

Need Standardisation

Vuex Store

X-Men




Getters
Vuex in Motion

Lab
- Please click on fork for your own version
- Remember to save for the changes to take effect
mapState
export default {
computed: {
user() {
return this.$store.state.user;
}
}
}
import {mapState} from 'vuex';
export default {
computed: mapState({
user: state => state.user
})
}
mapState shortcuts
import {mapState} from 'vuex';
export default {
computed: mapState({
user: state => state.user
})
}
import {mapState} from 'vuex';
export default {
computed: mapState({ user: 'user' })
}
import {mapState} from 'vuex';
export default {
computed: mapState(['user'])
}
import {mapState} from 'vuex';
export default {
computed: {
localComputed() { return 'something'; },
...mapState(['user']),
}
}
Lab 1
- Create title in your store with 'My Hero Academia'
- By using mapState update the title from the store
- Update name property according to your name
Lab 2
- Move heroes list from data to state
Getters
export default new Vuex.Store({
...,
getters: {
doneTodos: state => {
return state.todos.filter(todo => todo.done);
},
doneTodosCount: (state, getters) => {
return getters.doneTodos.length;
},
}
});
Lab 3
- create a getter call currentHeroes which filters out 'Iron Man'
- create a getter call countHeroes which counts currentHeroes
- update OnCall.vue accordingly to consume getters
Lab 4
- create an ADD_HERO mutation which adds a new hero in the state
- map the mutation onto Hero.vue
- create the method addHero to execute mapped mutation with the input newHero
Lab 5
- create a REMOVE_HERO mutation which removes a hero in the state
- create an action removeHero to commit the mutation
- map the actions onto Hero.vue
- create the method removeHeroes on the component to execute the mapped action with the corresponding index
Lab 6
- create a REMOVE_ALL mutation which makes heroes in state as empty
- create an action removeAll to commit the mutation
- map the actions onto OnCall.vue
- create the method removeAllHeroes on the component to execute the mapped action and set msg to 'All heroes have been removed'
Resources
- Vuex
- Keystone
- Keystone Component
Questions?
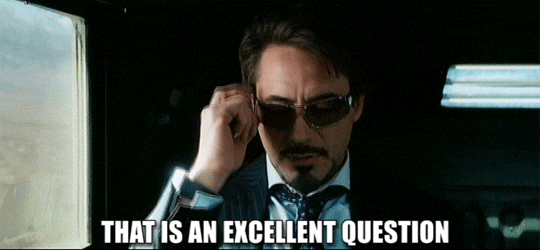
Latest State Management
By Thomas Truong
Latest State Management
State Management Slides
- 66