var object = new Object();
var object = Object.create(null);
var object = {};
function Person(name){
var object = {};
object.name=name;
object.age=21;
return object;
}
var object = new Person("Sudheer");
What is the prototype chain?
Prototype chaining is used to build new types of objects based on existing ones. It is similar to inheritance in a class-based language. The prototype on object instance is available through Object.getPrototypeOf(object) or proto property whereas prototype on constructors function is available through object.prototype.
What is the purpose of an array slice method?
The slice() method returns the selected elements in an array as a new array object. It selects the elements starting at the given start argument and ends at the given optional end argument without including the last element. If you omit the second argument then it selects till the end. Some of the examples of this method are
let arrayIntegers = [1, 2, 3, 4, 5]; let arrayIntegers1 = arrayIntegers.slice(2,2); // returns [1,2] let arrayIntegers2 = arrayIntegers.slice(2,3); // returns [3] let arrayIntegers3 = arrayIntegers.slice(4); //returns [4]
What is the purpose of the array splice method?
-
The splice() method is used either adds/removes items to/from an array, and then returns the removed item. The first argument specifies the array position for insertion or deletion whereas the option second argument indicates the number of elements to be deleted. Each additional argument is added to the array. Some of the examples of this method are,
Difference between slice & splice
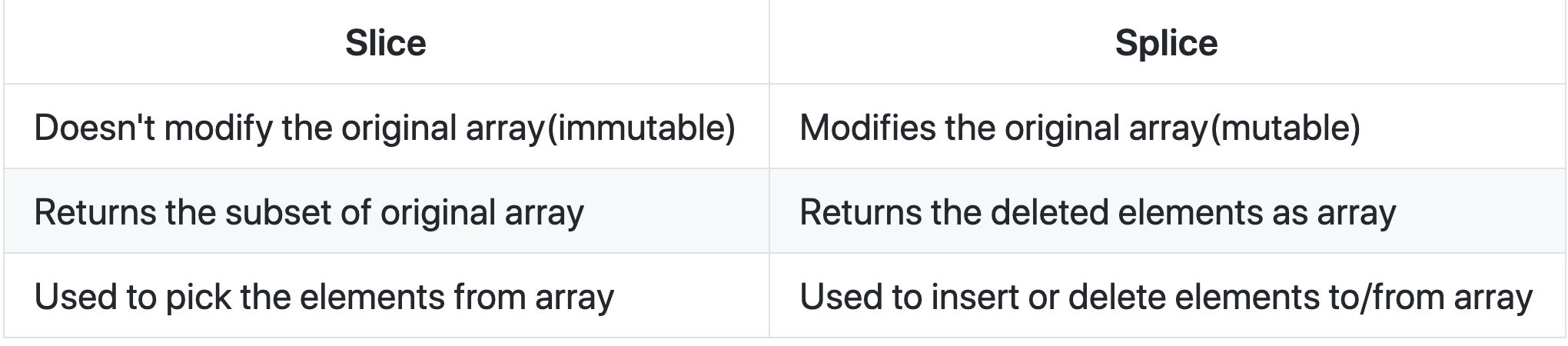
First class functions
In Javascript, functions are first class objects. First-class functions means when functions in that language are treated like any other variable. For example, in such a language, a function can be passed as an argument to other functions, can be returned by another function and can be assigned as a value to a variable. For example, in the below example, handler functions assigned to a listener
const handler = () => console.log ('This is a click handler function'); document.addEventListener ('click', handler);
Higher Order Function
Higher-order function is a function that accepts other function as an argument or returns a function as a return value.
const increment = x => x+1;
const higherOrder = higherOrder(increment);
console.log(higherOrder(1));
currying function ?
Currying is the process of taking a function with multiple arguments and turning it into a sequence of functions each with only a single argument. Currying is named after a mathematician Haskell Curry. By applying currying, a n-ary function turns it into a unary function. Let's take an example of n-ary function and how it turns into a currying function
const multiArgFunction = (a, b, c) => a + b + c; const curryUnaryFunction = a => b => c => a + b + c; curryUnaryFunction (1); // returns a function: b => c => 1 + b + c curryUnaryFunction (1) (2); // returns a function: c => 2 + c curryUnaryFunction (1) (2) (3); // returns the number 6
Difference between Var and let
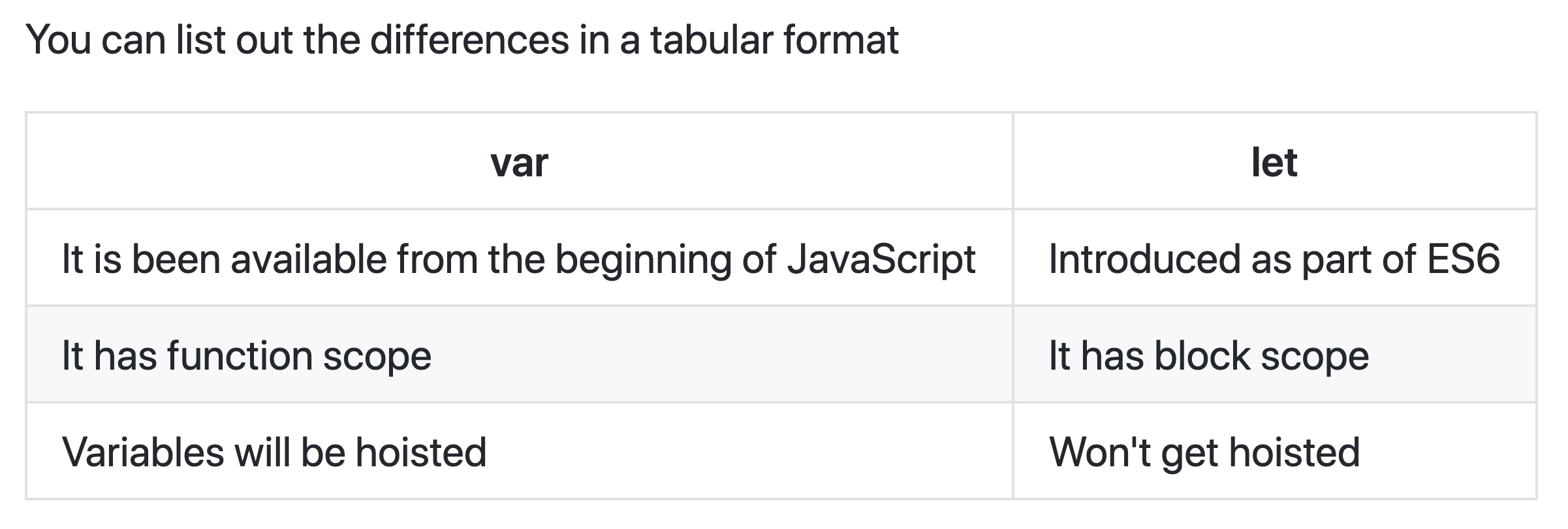
Done
JS INT-06
By Tarun Sharma
JS INT-06
React
- 354