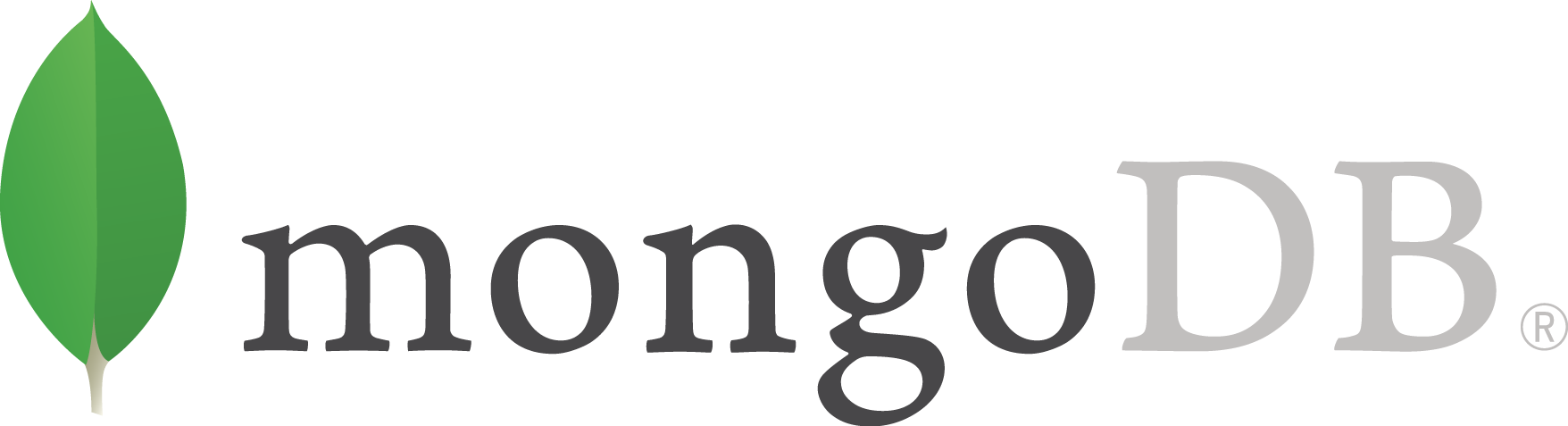
Fundamental Database Concepts
- Records (i.e. rows/documents)
- Tables/collections
- Databases
- Authentication and authorization concepts
- Joins and how they work in relational databases
- Transactions and a basic understanding related ideas such as commits and rollback.
Memory Management and Data Representation
- Physical memory
- Virtual memory
- Memory mapping
- Hexidecimal (base-16) representations of data
Basics of JavaScript Programming
- How to assign values to variables in JavaScript
- How to iterate (e.g., using for or while)
> var x = 0
> while ( x < 5 ) { x++; print(x) }
1
2
3
4
5
> for (i=0; i<=3; i++) { print(i) }
0
1
2
3
> x = { a : 4, b : 3, c : 2 }
{ "a" : 4, "b" : 3, "c" : 2 }
> for (key in x) { print(key + ': ' + x[key]) }
a: 4
b: 3
c: 2
Key Features (of MongoDB)
-
High Performance
-
Rich Query Language
-
High Availability
-
Horizontal Scalability
-
Support for Multiple Storage Engines
JSON (JavaScript Object Notation) is a lightweight data-interchange format.
It is easy for humans to read and write. It is easy for machines to parse and generate. It is based on a subset of the JavaScript Programming Language, Standard ECMA-262 3rd Edition - December 1999.
JSON is built on two structures:
- A collection of name/value pairs.
(In various languages, this is realized as an object, record, struct, dictionary, hash table, keyed list, or associative array.) - An ordered list of values.
(In most languages, this is realized as an array, vector, list, or sequence.)
These are universal data structures. Virtually all modern programming languages support them in one form or another. It makes sense that a data format that is interchangeable with programming languages also be based on these structures.





BSON [bee · sahn], short for Binary JSON, is a binary-encoded serialization of JSON-like documents.
Its is:
- Lightweight
- Traversable
-
Efficient
(to encoding/decoding)
Example
{"hello": "world"}
\x16\x00\x00\x00 // total document size
\x02 // 0x02 = type String
hello\x00 // field name
\x06\x00\x00\x00world\x00 // field value
\x00 // 0x00 = type EOO ('end of object')
MongoDB stores data records as BSON documents. BSON is a binary representation of JSON documents, though it contains more data types than JSON.
Vertical and Horizontal Scaling
CRUD
CRUD operations create, read, update, and delete documents.
db.collection.insertOne() db.collection.insertMany() db.collection.find() db.collection.updateOne() db.collection.updateMany() db.collection.replaceOne() db.collection.deleteOne() db.collection.deleteMany()
Create
Read
db.collection.find() - returns a Cursor
-
Operators for db.collection.find():
-
Array Operators:
Cursor Methods
- .hasNext(), next()
- .count()
- .sort(), .skip(), .limit()
Update
-
Operators for db.collection.find():
-
$set and $unset
-
$rename
-
-
Array Operators:
-
$push, $pushAll
-
$pull, $pullAll
-
$addToSet
-
Delete
MongoDB CRUD
By Ashesh Kumar
MongoDB CRUD
- 710