JS Libraries and Frameworks
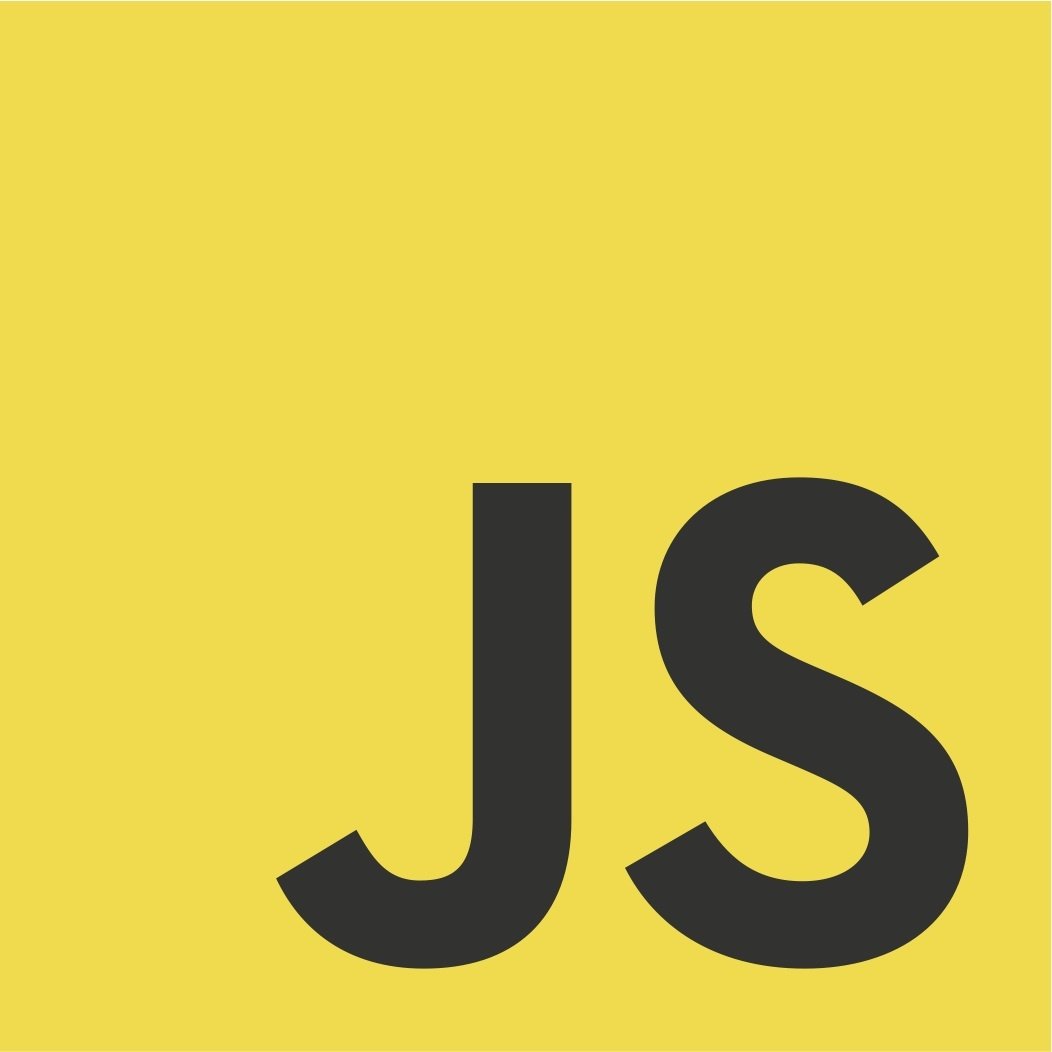
What's the difference between a library and a framework?
Library
- Collection of pre-written code
- Less rules
- Call the code whenever you want
- i.e. Jquery, React are JS libraries
Framework
- Defines the entire application design
- More rules
- The framework calls your code
- i.e. AngularJS, VueJS are frameworks
Some trending JS Libraries (2019)
- howler js flexible audio library
- sweet alert two universal popup library
- cleave js input format library
- html 2 canvas web screenshot library
- popmotion js animation library
- typed.js typing animation library
- scroll magic scroll interaction library
React vs Angular vs Vue
Which is the most popular?
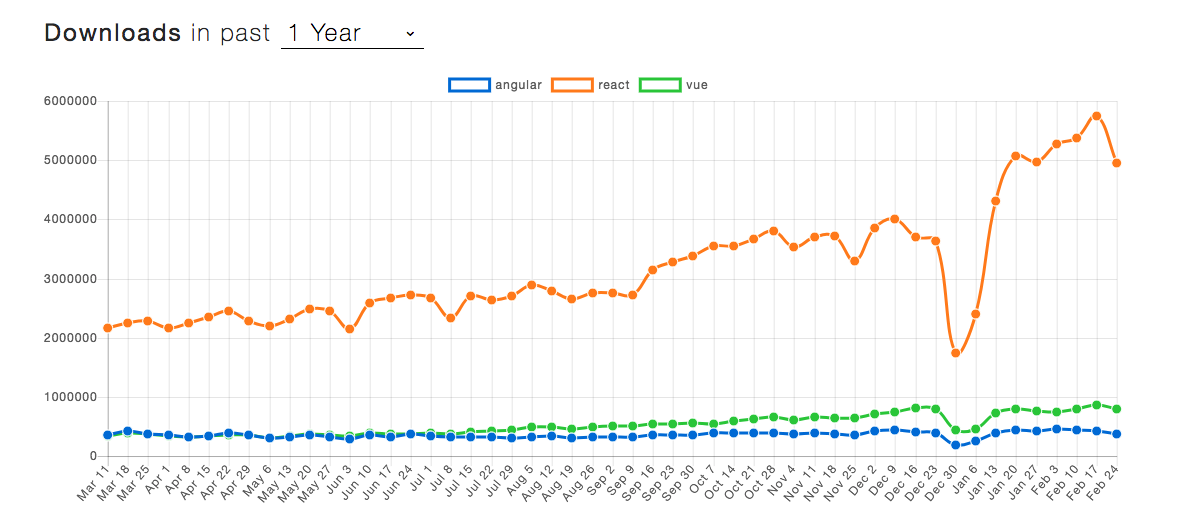
React
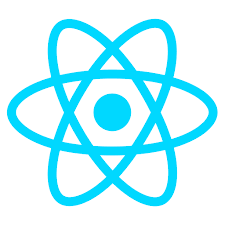
What is React?
-
React is a JS Library created by Facebook
-
It is a user interface (UI) library used to build UI components
-
Makes changes seamless
-
Strong demand in the job market
-
Good documentation and resources
-
Facebook support
React Resources
Hello World Example
ReactDOM.render(
React.createElement(
'h1',
null,
'Hello World'
),
document.getElementById('app')
);
//JSX syntax extension example
ReactDOM.render(
<h1>Hello World, this is written in JSX!</h1>,
document.getElementById('app')
);
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<link rel="stylesheet" href="css/styles.css">
<script crossorigin src="https://unpkg.com/react@16/umd/react.development.js"></script>
<script crossorigin src="https://unpkg.com/react-dom@16/umd/react-dom.development.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-core/5.8.2/browser.min.js"></script>
</head>
<body>
<div id="app"></div>
<script src="js/app.js" type='text/babel'></script>
</body>
</html>
AngularJS
What is AngularJS?
-
Is a framework for dynamic web apps
-
It lets you use HTML as your template language and extend the HTML syntax
-
Data binding allows you to eliminate much of the code you would otherwise have to write
-
Full featured framework
-
Google support
-
Controls the complete user experience, navigation
AngularJS Resources
Hello World Example
<!DOCTYPE html>
<html lang="en" ng-app="">
<!-- ng = angular working with app in angular -->
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Angular JS Intro</title>
<link rel="stylesheet" href="css/styles.css">
<script src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.7.7/angular.min.js"></script>
</head>
<body>
<input type="text" ng-model='name'>
<div>Hello {{ name }}</div>
<div ng-bind='name'></div>
<script src="js/app.js"></script>
</body>
</html>
VueJS
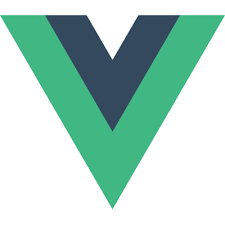
What is VueJS?
-
Vue is a JS framework that lets you extend HTML and HTML attributes
-
Good documentation
-
Rising in popularity
-
Easy to learn
VueJS Resources
Hello World Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Vue Intro</title>
<link rel="stylesheet" href="css/styles.css">
<script src="https://cdn.jsdelivr.net/npm/vue"></script>
</head>
<body>
<div id="main">
{{ msg }}
</div>
<script src="js/app.js"></script>
</body>
</html>
new Vue({
el: '#main',
data: {
msg: 'Hello World'
}
});
JS Libraries and Frameworks
By vic_lee
JS Libraries and Frameworks
- 469