Algorithms #1

Starting your journey
How we will work

Please don't be shy
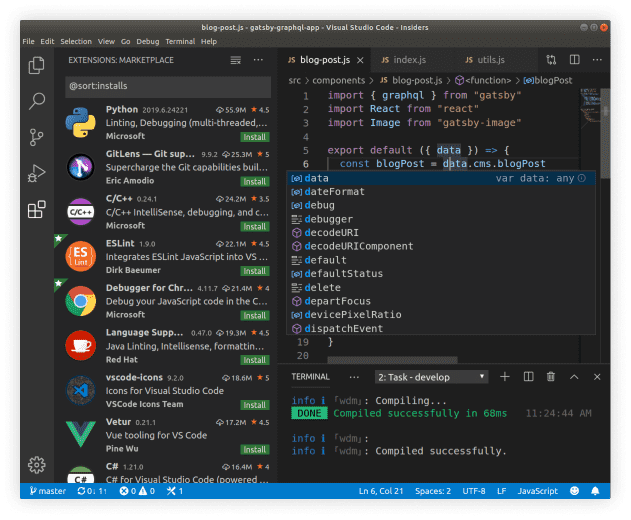
Setup
vscode
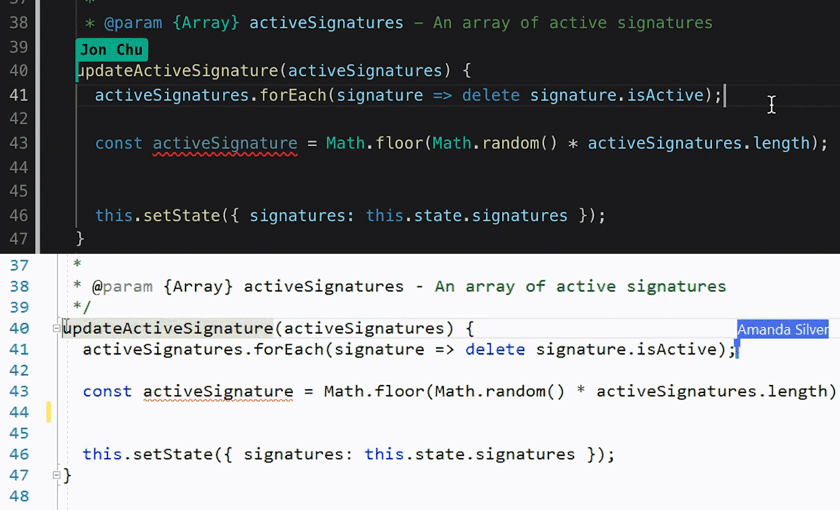
live-share plugin
Please download this two things
Do it now
Is It REALLY Necessary to learn algorithms?
An algorithm is any well-defined computational procedure that takes some value, or set of values, as input and produces some value, or set of values, as output. An algorithm is thus a sequence of computational steps that transform the input into the output.
Let us first find out what an algorithm is...

Let's check uses of algorithms
1. Google maps & Dijkstra algorithm and weighteg graph
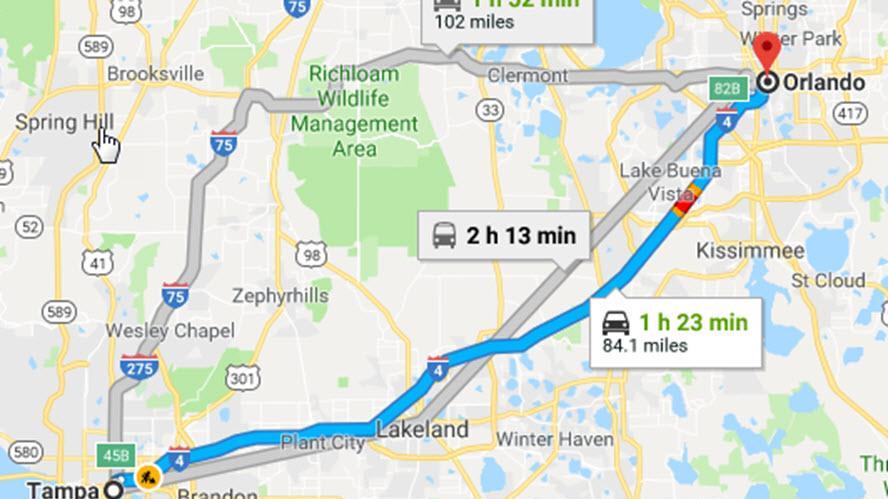
2. Companies optimizing expenses via DP methods
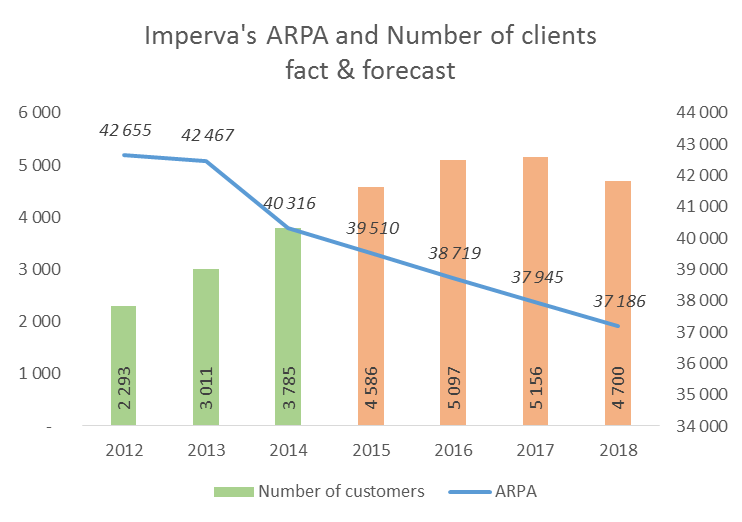
3. Recommendation systems and social networks use graphs

4. OS works with process priority using Heap & Priority Queue

5. DOM internal Tree structure organisation

6. How hashing & HashMap works. Which gives great boost in different in-memory DB stores.

7. Stack & Queue. Which are used everywhere (For ex. browser history)
8. Different ways of searching and sorting data in the collections

So should a programmer learn and know ?
Depends on the goals
Forms,
CRMs
REST services
etc.
NO
YES

New products & platforms,
science, on-line games
devices, startups
It is important to know which algorithm you should select and when to use it
It's essential to understand algorithm development so you can program more efficiently, write better programs and kick ass on interviews!

Course plan
Time & Space complexity
Approaches to solve problems
Searching & Sorting
LinkedList
Trees
Graphs
Dijkstra
Dynamic Programming
Gready algorithms
Finish
You are free to propose a topic/algorithm and we will add it to the course if others are interested also
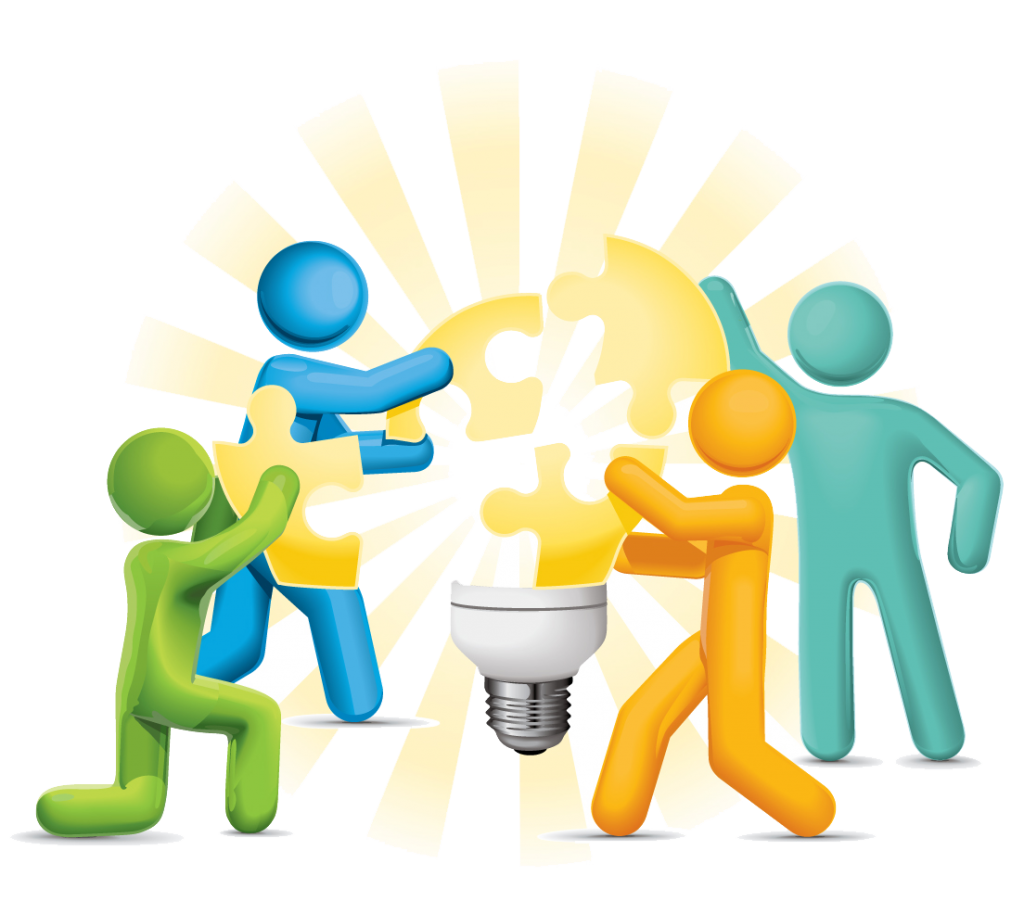
What is a good algorithm ?

GOOD ALGORITHM = Low time complexity & Low space complexity & Good readability & Correct results
Complexity ?
Big Ω , Big θ, and Big O
Asymptotic notations are super versatile and super cool. They measures how an algorithm scales in relation to its input size
Big O Notation is a convenient way to express the worst-case scenario for an algorithm


Big Omega represents the fastest possible running time

One function is Big θ of another if and only if that function is both Big O and Big Omega of it



Simplifying big O


In our case case, time complexity of addUpTp function is O(5n + 2) => O(n)
We need to know a GENERAL TREND, so we drop the constants and smaller powers
O(n + 5) = O(n)
O(2n) = O(n)
O(100) = O(1)
O(n² + 6n + 7) = O(n²)
O(6n^1/3 + n^1/2 + 7) = O(n^1/2)
Other examples:
Space complexity
Space complexity is a measure of the amount of working storage an algorithm needs. That means how much memory, in the worst case, is needed at any point in the algorithm.

This function requires 3 units of space for the parameters and 1 for the local variable, and this never changes, so this is O(1).
function sum(x, y, z) {
let r = x + y + z;
return r;
}
Space complexity
Big O of JS objects
let obj = {}; // O(1)
obj.test = 1 // O(1)
delete obj.test // O(1)
someVar = obj.test // O(1)
Object.keys(obj).find(key => key === “test”) // O(kn),
// where n = number of keys, k = max chars in key
Object.keys // O(n)
Object.values // O(n)
Object.entries // O(n)
Big O of JS arrays
let array = [“one”, “two”, “three”];
array[1] // accessing, O(1)
array.push(“four”) // appending, O(1)
array.shift(“zero”) // prepenging, O(n)
array.pop() // popping last element, O(1)
array.unshift() // retrieving first element, O(n)
array.concat // O(n)
array.slice // O(n)
array.splice // O(n)
array.forEach // O(n)
array.sort // O(n*log(n))
Timing JS code
performance.now()
let t0 = performance.now();
doSomething();
let t1 = performance.now();
console.log("Call to doSomething took " + (t1 - t0) + " milliseconds.");
Unlike other timing data available to JavaScript (for example Date.now), the timestamps returned by performance.now() are not limited to one-millisecond resolution. Instead, they represent times as floating-point numbers with up to microsecond precision.
console.time(label)
console.time("answer time");
alert("Нажмите для продолжения");
console.timeLog("answer time");
alert("Делаем кучу другой работы...");
console.timeEnd("answer time");
Notice that the timer's name is displayed when the timer value is logged using timeLog() and again when it's stopped. In addition, the call to timeEnd() has the additional information, "timer ended" to make it obvious that the timer is no longer tracking time
Reverse String ☆
Write a function that reverses a string. The input string is given as an array of characters char[].
Do not allocate extra space for another array, you must do this by modifying the input array in-place with O(1) extra memory.
/*
* Input: ["h","e","l","l","o"]
* Output: ["o","l","l","e","h"]
*/
function reverseString(s) {
};
Culculate SUM of digits☆
Write a function to calculate sum of all digits in a number
/*
* Input: 123
* Output: 6
*/
function calc(n) {
};
Find missing number task ☆
Given an unsorted array of 999 unique numbers from 1 to 1000. Find the missing number!
Homework
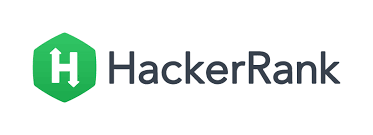
OR
Start solvig easy problems!
Unique Number of Occurrences ☆
Given an array of integers arr, write a function that returns true if and only if the number of occurrences of each value in the array is unique.
/* Input: arr = [1,2,2,1,1,3]
Output: true
Explanation: The value 1 has 3 occurrences, 2 has 2 and 3 has 1.
No two values have the same number of occurrences.
*/
/*
Input: arr = [1,2]
Output: false
*/
var uniqueOccurrences = function(arr) {
};
Thank You!

Links
https://www.youtube.com/watch?v=GhFmRmCK2Ck - Time Complexity and Big O Notation of Algorithms
https://www.youtube.com/watch?v=FJcG-6g4wA4 - Learning Algorithms: Is It REALLY Necessary?
GAYLE LAAKMANN MCDOWELL Cracking the Coding Interview 6th Edition: Big O page 38
http://getyouralgorithm.blogspot.com/2015/10/big-o-notation-simplify-define.html
Join telegram group!

Algorithms #1
By Vladimir Vyshko
Algorithms #1
Introduction to algorithms course
- 613