You (still!) don't know async ⚡️ await?
The C# / .NET edition, not the JavaScript one...
#WinDays19, Šibenik 2019-04-05
bit.ly/wd19-async-await
Vedran Mandić, MCSD, MCT, @vekzdran
OH DEAR, REALLY... STILL!? WHY! ...ME ALSO :')
Thank you sponsors!

Hi, I am Vedran. 👋
- I freelance, talk and lecture C# / .NET and JavaScript
- currently building a greenfield manufacturing ind. ERP
- on medium.com and dev.to by @vekzdran
- on github by @vmandic
- programming for the last 8 years
- bad at basics, suck at CSS, moderate in C#... jk 🙈

Agenda
- Must-know 🌟 terminology
- async / await FAQ 15+ examples
- demo code 👩💻
- Q&A (please after 1 && 2) ⏰
- Share and apply, please, plz...
10min + 30min (insane tempo)
(very boring) Important
Terminology Recap
Take deep breaths now please!
System.Threading.Thread
- CPU time to process work
- has priority, (synchronization) context
- OS thread mapped to managed .NET
- "types" e.g. main, UI, worker, ThreadPool t ...
- new Thread() is not from a ThreadPool & no SyncContext
- you can run in Multiple/Parallel
- synced by many constructs such as lock() { ... }, monitors...
System.Threading.ThreadPool
- a pool of process threads
- has a min and max limit, careful!!
- TP bg threads won't block the fg threads
- [ThreadStaticAttribute] values are not cleared on reuse
System.Threading.Tasks.Task
- since .NET v4.0, before async / await
- promise of work to be done (by a Thread)
- uses bg Threads from ThreadPool
- use LongRunning Tasks if you must run long op
- can be awaited (in an async scope)
- Task<T> to return a T .Result (else void .Wait())
- can be nested / attached
SynchronizationContext
- represents a "location" where a Thread is run / executed
- WinFormsSynchronizationContext, AspNetSC, default...
-
behaviour differs across implementations
-
WinForms forbids updating UI from other contexts!
- await captures the current context and resumes on it
- ASP.NET Core has no SynchronizationContext:
-> perf is better!
TaskScheduler
- Tasks scheduling & execution
- Abstraction over SyncContext
- TaskScheduler.Default
->ThreadPool global and local queues - abstract, build your own, limit #No of Threads

How is a task scheduled?
Deadlock
- one or more threads blocks / waits / hangs on a line of code indefinitely, the application is blocked and UNUSABLE
(thread) Blocking
-
blocked thread waits / hangs and can not be used for other work execution, process memory usage spikes
- applying valid async / await syntax releases the awaiting thread back to the ThreadPool
Sync
- Crack egg 1
- Fry egg 1
- Prepare plate 1
- Serve egg 1
- Crack egg 2
- Fry egg 2
- Prepare plate 2
- Serve egg 2
- Crack egg 3
- Fry egg 3
- Prepare plate 3
- Serve egg 3
VS Async VS
- Crack and mix 3 eggs
-
Let fry 3 eggs together
-
While frying prepare 3 plates
- Serve 3 eggs
Parallel
-
3 cooks crack their egg
- 3 cooks fry their egg
- 3 cooks get their plate
- 3 cooks serve their egg
That's it for the boring part...
demo bit.ly/wd19-async-await-demo
thanks you can leave now :)
If this was too much... :-)
https://michaelscodingspot.com/2019/01/17/c-deadlocks-in-depth-part-1/
https://michaelscodingspot.com/2019/01/24/c-deadlocks-in-depth-part-2/
Locking and Deadlocks explained by example with async / await
https://odetocode.com/blogs/scott/archive/2019/03/04/await-the-async-letdown.asp
thoughts by a senior C# developer and author on why working with async / await is hard and prone to errors
an excellent summary of multiple how-to and don’ts with async / await in dotnet core
https://dev.to/stuartblang/miscellaneous-c-async-tips-3o47
how to deal with lazy initialization in CTOR that has to become async (AsyncLazy example)
http://blog.i3arnon.com/2015/07/02/task-run-long-running/
TaskCreationOptions.LongRunning explained, one can read that an actual thread (not from the thread pool) is created
http://blog.stephencleary.com/2017/03/aspnetcore-synchronization-context.html - SyncContext in ASP.NET Core and dangers one can face
https://msdn.microsoft.com/en-us/magazine/dn802603.aspx
a detailed overview how async applies to the ASP.NET runtime, how thread-pool allocates threads and when does context switching happen
http://blog.stephencleary.com/2012/07/dont-block-on-async-code.html
the evergreen example of why not to block async calls with .Result or .Wait() in traditional environments which have a dedicated syncContext
https://msdn.microsoft.com/en-us/magazine/gg598924.aspx
why we need the synchronization context
https://markheath.net/post/async-antipatterns
a summary of all the examples and more, fresh 2019 article
https://www.youtube.com/watch?v=av5YNd4X3dY
correcting Common Mistakes When Using Async/Await in .NET - Brandon Minnick
https://www.youtube.com/watch?v=Al8LrBKpZEU
Back to Basics: Efficient Async and Await - Filip Ekberg
https://dev.to/hardkoded/a-fairy-tale-about-async-voids-events-and-error-handling-1afi
real world example of async void impact
https://www.youtube.com/watch?v=-cJjnVTJ5og
“Why your ASP.NET Core application won’t scale?”, has a lot of cool async trap examples at the end - D. Edwards and D. Fowler at NDC London 2019
https://www.youtube.com/watch?v=ghDS4_NFbcQ
“The promise of an async future awaits” - overview of async and await and it’s issues, Bill Wagner (.NET docs team) at NDC London 2019, explaining Sync, Async and Parallel with making an English breakfast example.
https://www.youtube.com/watch?v=XpgN7y_EXps
“Async & Await (You’re doing it wrong)”, good examples of bad usage: async void, async void lambda, async constructor and property, running async in sync (blocking examples)
https://chrisstclair.co.uk/demystifying-async-await
a quick and simple example of how bad application blocks the UI and how to quickly fix it.
https://medium.com/rubrikkgroup/understanding-async-avoiding-deadlocks-e41f8f2c6f5d
when and how can a deadlock occur with async / await, a table view breakdown depending in which execution context the task is run, excellent overview
https://olitee.com/2015/01/c-async-await-common-deadlock-scenario/
a deadlock scenario explained
https://devblogs.microsoft.com/pfxteam/asyncawait-faq/
probably the best and concrete FAQ on async / await there is on the internet
https://devblogs.microsoft.com/pfxteam/await-and-ui-and-deadlocks-oh-my/
the most simple and right explanation of deadlock common scenario when the UI thread is blocked due to .Result or Wait() and can not restore new state from the updated SynchronizationContext (i.e. windows message loop when working with Windows Forms and WPF).
The end! 📸 Questions?
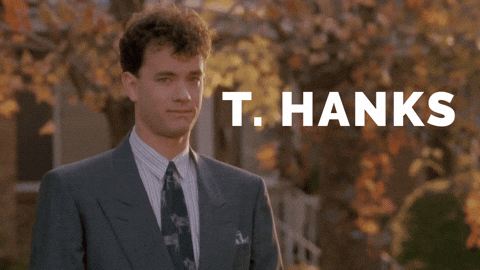
VEDRAN MANDIĆ
mandic.vedran@gmail.com
@vekzdran @vmandic
bit.ly/wd19-async-await-demo
bit.ly/wd19-async-await
You (still!) don't know async / await?
By Vedran Mandić
You (still!) don't know async / await?
Still feeling unsure when you write an async method in C# with .NET? Well, I got you covered, example by example where you might've failed. This presentation will cover basic terminology and the common async / await pitfalls which might cost you your job.
- 1,627