An introduction to Elm
Elm
A delightful language for reliable webapps.
Generate JavaScript with great performance and no runtime exceptions.
A gentle introduction to types
Most languages have types
Elm is statically typed
The type spectrum
#include <stdio.h>
int main ()
{
int a = 2;
char b[] = "2";
printf("2 + 2 = %d", a + b);
}
add.c
$ gcc add.c -o add
#include <stdio.h>
int main ()
{
int a = 2;
char b[] = "2";
printf("2 + 2 = %d", a + b);
}
add.c
$ gcc add.c -o add
add.c: In function ‘main’:
add.c:8:22: warning: format ‘%d’ expects argument of type ‘int’, but
argument 2 has type ‘char *’ [-Wformat=]
printf("2 + 2 = %d", a + b);
#include <stdio.h>
int main ()
{
int a = 2;
char b[] = "2";
printf("2 + 2 = %d", a + b);
}
add.c
$ gcc add.c -o add
add.c: In function ‘main’:
add.c:8:22: warning: format ‘%d’ expects argument of type ‘int’, but
argument 2 has type ‘char *’ [-Wformat=]
printf("2 + 2 = %d", a + b);
$ ./add
2 + 2 = 1152074146
$ ./add
2 + 2 = 450267314
?????
public class Add {
public static void main(String[] args) {
int a = 2;
String b = "2";
System.out.printf("2 + 2 = %d", a + b);
}
}
Add.java
$ javac Add.java
public class Add {
public static void main(String[] args) {
int a = 2;
String b = "2";
System.out.printf("2 + 2 = %d", a + b);
}
}
Add.java
$ javac Add.java
$ java Add
2 + 2 = Exception in thread "main" java.util.IllegalFormatConversionException: d != java.lang.String
at java.util.Formatter$FormatSpecifier.failConversion(java.base@9-Ubuntu/Formatter.java:4275)
at java.util.Formatter$FormatSpecifier.printInteger(java.base@9-Ubuntu/Formatter.java:2790)
at java.util.Formatter$FormatSpecifier.print(java.base@9-Ubuntu/Formatter.java:2744)
at java.util.Formatter.format(java.base@9-Ubuntu/Formatter.java:2525)
at java.io.PrintStream.format(java.base@9-Ubuntu/PrintStream.java:974)
at java.io.PrintStream.printf(java.base@9-Ubuntu/PrintStream.java:870)
at Add.main(Add.java:6)
Runtime errors!
a = 2
b = "2"
main = putStrLn ("2 + 2 = " ++ show (a + b))
add.hs
$ ghc add.hs
[1 of 1] Compiling Main ( add.hs, add.o )
add.hs:1:5:
No instance for (Num [Char]) arising from the literal ‘2’
In the expression: 2
In an equation for ‘a’: a = 2
add.hs:4:40:
No instance for (Num [Char]) arising from a use of ‘+’
In the first argument of ‘show’, namely ‘(a + b)’
In the second argument of ‘(++)’, namely ‘show (a + b)’
In the first argument of ‘putStrLn’, namely
‘("2 + 2 = " ++ show (a + b))’
Compile time errors!
"Generate JavaScript with great performance and no runtime exceptions."
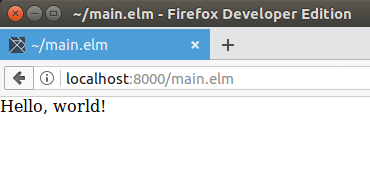
import Html exposing (text)
main =
text "Hello, world!"
import Html exposing (text)
import List exposing (..)
greetings =
[ "Hola, mundo!"
, "Hello, world!"
, "Bonjour, le monde!"
]
main =
text (head greetings)
import Html exposing (text)
import List exposing (..)
greetings =
[ "Hola, mundo!"
, "Hello, world!"
, "Bonjour, le monde!"
]
main =
text (head greetings)

import Html exposing (text)
import List exposing (..)
greetings =
[ "Hola, mundo!"
, "Hello, world!"
, "Bonjour, le monde!"
]
greeting =
case head greetings of
Just value -> value
Nothing -> "No greetings! :("
main =
text greeting

An introduction to Elm
By Joseph Wynn
An introduction to Elm
- 1,726