Kotlin for Java Developers
Yuvraj Waghray
Who Am I?

https://github.com/yuvrajw

Java
- Static Typing
- Strong Ecosystem
- Community
- JVM
Java
public class Person {
private final String first;
private final String last;
public Person(String first, String last) {
this.first = first;
this.last = last;
}
public String getFirst() {
return first;
}
public String getLast() {
return last;
}
@Override
public String toString() {
return "Person( first=" + first + "last=" + last + ")";
}
@Override
public int hashCode() {
return Objects.hash(first, last);
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Person person = (Person) obj;
return Objects.equals(first, person.first)
&& Objects.equals(last, person.last);
}
public Person copy(Object obj) {
return null;
}
}
Kotlin
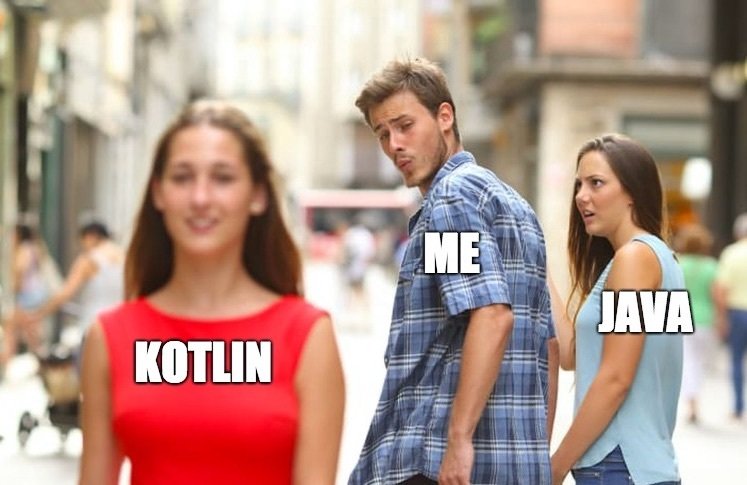
What is Kotlin?
JVM
Android
Browser
Native
Why Kotlin?
- Concise
- Versatile
- Brings goodies from other languages
- Interoperable
- Tooling
Key Features
- Null safe type system
- Immutable by design
- Type inference
- Smart/Dynamic casts
- String templating
- Multiline strings
- ....
- Automatic properties
- Data classes
- if / when / for expressions
- Functional Programming
- Extension methods
- No Checked Exceptions
- ....
Let's Dive In!
Drawbacks
- Smaller / Growing Community
- Fewer developers with expertise
- Lack of support in tools like HP Fortify
Resources
Questions?
Kotlin for JVM
By Yuvraj Waghray
Kotlin for JVM
Eonics Hacknight presentation
- 906