Kotlin for Java Developers
Yuvraj Waghray
Who Am I?

https://github.com/yuvrajw

Java
- Static Typing
- Strong Ecosystem
- Community
- JVM
Java
import java.util.Objects;
public class Customer {
private String firstName;
private String lastName;
private String email;
private String company;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getCompany() {
if (company != null) {
return company;
}
return "";
}
public void setCompany(String company) {
this.company = company;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Customer customer = (Customer) o;
return Objects.equals(email, customer.email);
}
@Override
public int hashCode() {
return Objects.hash(email);
}
}
Kotlin
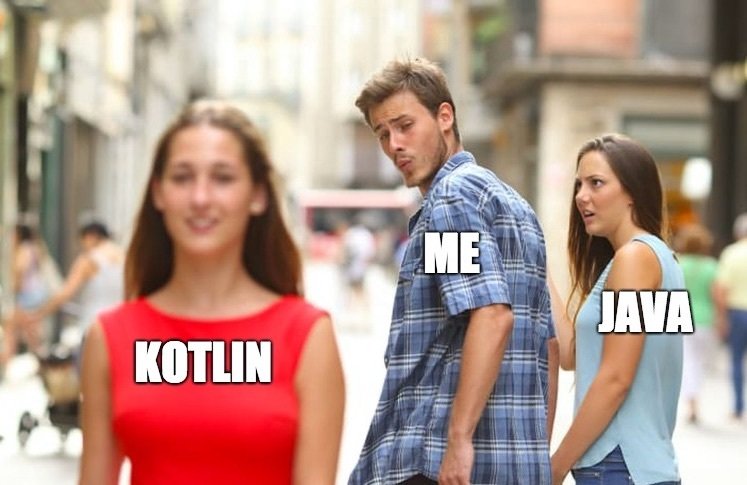
What is Kotlin?
JVM
Android
Browser
Native
Why Kotlin?
- Concise
- Versatile
- Brings goodies from other languages
- Interoperable
- Tooling
Key Features
- Null safe type system
- Immutable by design
- Type inference
- Smart/Dynamic casts
- String templating
- Multiline strings
- ....
- Automatic properties
- Data classes
- if / when / for expressions
- Functional Programming
- Extension methods
- No Checked Exceptions
- ....
Let's Dive In!
Drawbacks
- Smaller / Growing Community
- Fewer developers with expertise
- Lack of support in tools like HP Fortify
- JVM issues such as Type erasure at runtime
Resources
Questions?
Copy of deck
By Yuvraj Waghray
Copy of deck
- 677