Telegram Bot Workshop


zaoldyeck @
Vincent Chiang @
江品陞 @

Slide @
Source Code @
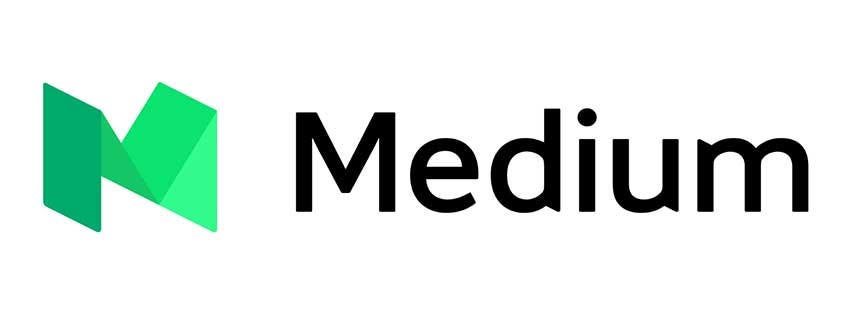
Vincent Chiang

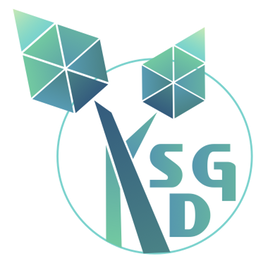

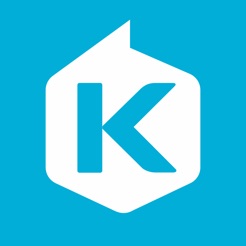
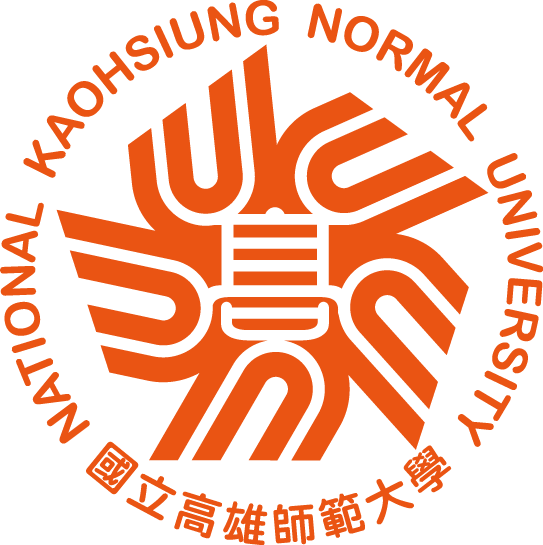
Goals
- Understanding the whole chatbot architecture
- Coding yourself(助教常見問題集)
- Build your own chatbot like https://t.me/innovation_chatbot
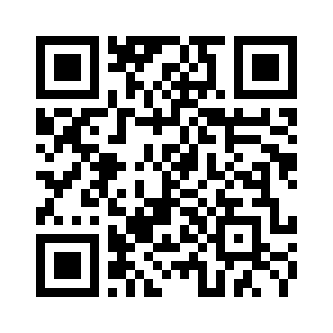
Why we need Chatbot?

The popularization of communication software
Millions of companies build relationships
with customers through communication App
Customers tired of install Apps
Chatbot is not for
- Be an assistant
- Replace customer service
- Chat
Chatbot is for
- Solve specific problems
- Get key words from users
- Improve efficiency
NLU



Multi Skills
3rd API
We will use
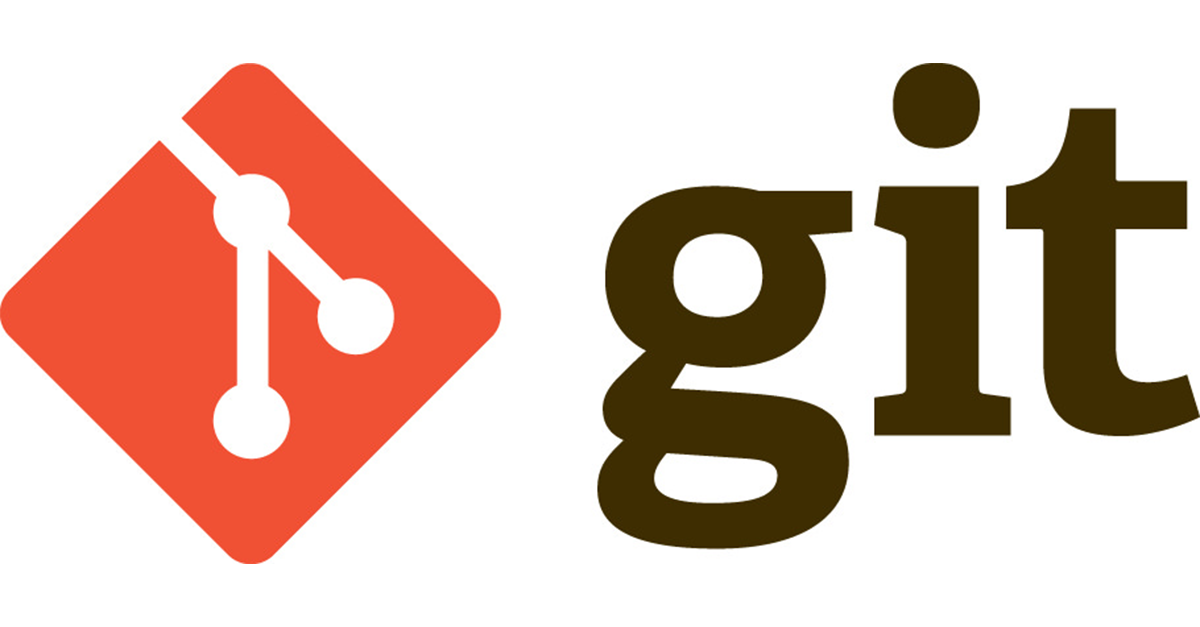
Scenario
Telegram
Webhook
NLU
Intent, Slots
Third-party APIs
Intent A
Intent B
Intent C
Intent D
Intent E
Fetch data by intent
Web Server
Send Message to User
Third-party APIs
Intent A
Intent B
Intent C
Intent D
Intent E
Response Data
Web Server
Generate Message
Telegram
BotFather(link)
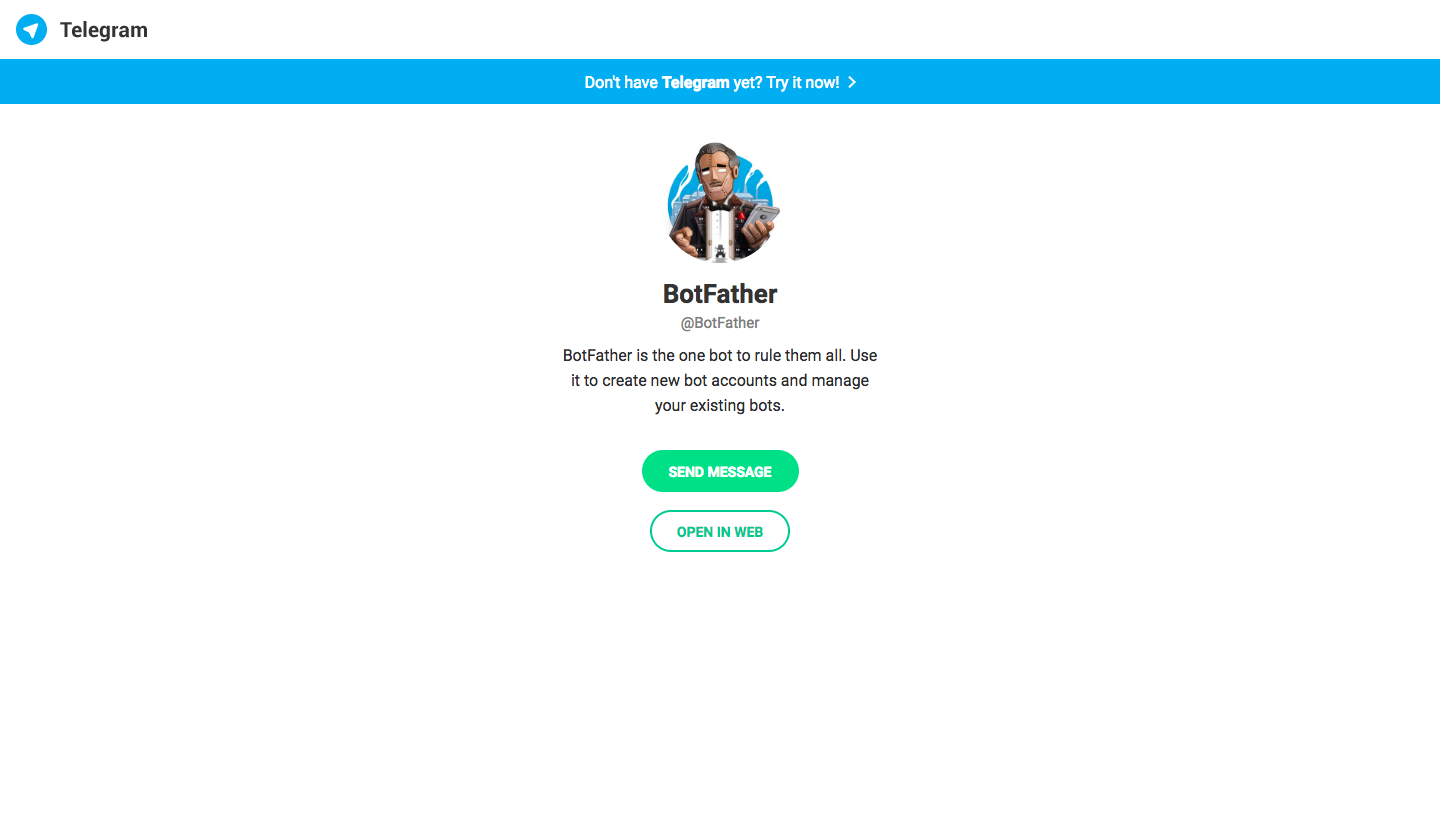
New bot

Your first echo chatbot
$ mkdir project_name # 建立專案目錄
$ cd project_name # 移動工作目錄至至專案目錄
$ pipenv install --three python-telegram-bot flask gunicorn requests # 安裝套件
$ vi config.ini # 新增&編輯 config.ini, 內容在下面連結
add config.ini
config.ini(See following slides)
[TELEGRAM]
ACCESS_TOKEN =
[OLAMI]
APP_KEY =
APP_SECRET =
[KKBOX]
ID =
SECRET =
# 請不要複製以下這一段
# 範例 ACCESS_TOKEN = 739453631:AAGeIaHIDQ1L48_q8uq7woWvLEw7zyFs0IA
Start coding
$ vi main.py # 新增 main.py 主程式, 內容在下面連結
Project Directory
├── config.ini
├── main.py
├── Pipfile.lock
└── Pipfile
add main.py
main.py
import configparser
import logging
import telegram
from flask import Flask, request
from telegram.ext import Dispatcher, MessageHandler, Filters
# Load data from config.ini file
config = configparser.ConfigParser()
config.read('config.ini')
# Enable logging
logging.basicConfig(format='%(asctime)s - %(name)s - %(levelname)s - %(message)s',
level=logging.INFO)
logger = logging.getLogger(__name__)
# Initial Flask app
app = Flask(__name__)
# Initial bot by Telegram access token
bot = telegram.Bot(token=(config['TELEGRAM']['ACCESS_TOKEN']))
@app.route('/hook', methods=['POST'])
def webhook_handler():
"""Set route /hook with POST method will trigger this method."""
if request.method == "POST":
update = telegram.Update.de_json(request.get_json(force=True), bot)
# Update dispatcher process that handler to process this message
dispatcher.process_update(update)
return 'ok'
def reply_handler(bot, update):
"""Reply message."""
text = update.message.text
update.message.reply_text(text)
# New a dispatcher for bot
dispatcher = Dispatcher(bot, None)
# Add a handler for handling message, there are many kinds of message. For this handler, it particular handle text
# message.
dispatcher.add_handler(MessageHandler(Filters.text, reply_handler))
if __name__ == "__main__":
# Running server
app.run(debug=True)
Router
Hook Function
Dispatcher
Message Handler
Program structure(web server)
Requests
Method: POST
Endpoint: /hook
Command Handler
Message Queue
Router
Hook Function
Dispatcher
Message Handler
Program structure(web server)
Requests
Command Handler
Message Queue
Method: POST
Endpoint: /hook
python-telegram-bot
Running server
$ pipenv run python3 main.py # 執行程式,之後請維持在 background 運作,程式有修改會熱更新

# 開新的 Terminal
$ ngrok http 5000 # 利用 ngrok 建立 public domain 至 localhost 5000 port 的 poxy, 之後維持在 background 運作

Telegram
Webhook
NLU
Intent, Slots
Third-party APIs
Intent A
Intent B
Intent C
Intent D
Intent E
Fetch data by intent
Web Server
Telegram
Webhook
ngrok server
proxy
ngrok client
Python HTTP server
listen port 5000
localhost
ngrok server
proxy
ngrok client
localhost
Generate Message
Send Message to User
Telegram
Setting webhook
# 在瀏覽器網址列中輸入
https://api.telegram.org/bot{$token}/setWebhook?url={$webhook_url}
# 舉例,記得 ngrok 的網址後面要加 '/hook'
https://api.telegram.org/bot739453631:AAGeIaHIDQ1L48_q8uq7woWvLEw7zyFs0IA/setWebhook?url=https://bcf4cd97.ngrok.io/hook
# 看到網頁出現以下訊息表示設定成功
{
ok: true,
result: true,
description: "Webhook was set"
}
Try it

What is NLU?
高雄今天天氣如何?
台積電的股價
播放周杰倫的告白氣球
Grammar:<locate>今天天氣如何?
Intent:weather
Slot:高雄
Grammar:<stock_name>的股價
Intent:stock
Slot:台積電
Grammar:播放<artist_name>的<track_name>
Intent:play_music
Slot:artist_name => 周杰倫,
track_name => 告白氣球
Webhook
Intent: weather
Slots: {locate: 高雄, date: today}
Third-party APIs
Weather API
Intent B
Intent C
Intent D
Intent E
Fetch Weather Data
高雄今天天氣如何?
NLU
Web Server
Telegram
Third-party APIs
Weather API
Intent B
Intent C
Intent D
Intent E
Response Data
高雄今天天氣晴,室溫 28 度
Web Server
Generate Message
Send Message to User
Telegram
Add NLU feature
- Rule-based
- Default IDS module skill
- Can add custom skill

Add NLU feature
# 開新的 Terminal
$ mkdir nlp
$ vi nlp/__init__.py
$ vi nlp/olami.py
$ vi main.py
Project Directory
├── nlp
| ├── __init__.py
| └── olami.py
├── config.ini
├── main.py
├── Pipfile
└── Pipfile.lock
add __init__.py
update main.py
add olami.py
__init__.py
from . import olami
olami.py(API doc)
import configparser
import json
import logging
import time
from hashlib import md5
import requests
config = configparser.ConfigParser()
config.read('config.ini')
logger = logging.getLogger(__name__)
class NliStatusError(Exception):
"""The NLI result status is not 'ok'"""
class Olami:
URL = 'https://tw.olami.ai/cloudservice/api'
def __init__(self, app_key=config['OLAMI']['APP_KEY'], app_secret=config['OLAMI']['APP_SECRET'], input_type=1):
self.app_key = app_key
self.app_secret = app_secret
self.input_type = input_type
def nli(self, text, cusid=None):
response = requests.post(self.URL, params=self._gen_parameters('nli', text, cusid))
response.raise_for_status()
response_json = response.json()
if response_json['status'] != 'ok':
raise NliStatusError("NLI responded status != 'ok': {}".format(response_json['status']))
else:
return response_json['data']['nli'][0]['desc_obj']['result']
def _gen_parameters(self, api, text, cusid):
timestamp_ms = (int(time.time() * 1000))
params = {'appkey': self.app_key,
'api': api,
'timestamp': timestamp_ms,
'sign': self._gen_sign(api, timestamp_ms),
'rq': self._gen_rq(text)}
if cusid is not None:
params.update(cusid=cusid)
return params
def _gen_sign(self, api, timestamp_ms):
data = self.app_secret + 'api=' + api + 'appkey=' + self.app_key + 'timestamp=' + \
str(timestamp_ms) + self.app_secret
return md5(data.encode('ascii')).hexdigest()
def _gen_rq(self, text):
obj = {'data_type': 'stt', 'data': {'input_type': self.input_type, 'text': text}}
return json.dumps(obj)
Update main.py
import configparser
import logging
import telegram
from flask import Flask, request
from telegram.ext import Dispatcher, MessageHandler, Filters
from nlp.olami import Olami
# Load data from config.ini file
config = configparser.ConfigParser()
config.read('config.ini')
# Enable logging
logging.basicConfig(format='%(asctime)s - %(name)s - %(levelname)s - %(message)s',
level=logging.INFO)
logger = logging.getLogger(__name__)
# Initial Flask app
app = Flask(__name__)
# Initial bot by Telegram access token
bot = telegram.Bot(token=(config['TELEGRAM']['ACCESS_TOKEN']))
@app.route('/hook', methods=['POST'])
def webhook_handler():
"""Set route /hook with POST method will trigger this method."""
if request.method == "POST":
update = telegram.Update.de_json(request.get_json(force=True), bot)
dispatcher.process_update(update)
return 'ok'
def reply_handler(bot, update):
"""Reply message."""
text = update.message.text
user_id = update.message.from_user.id
reply = Olami().nli(text, user_id)
update.message.reply_text(reply)
# New a dispatcher for bot
dispatcher = Dispatcher(bot, None)
# Add handlers for handling message, there are many kinds of message. For this handler, it particular handle text
# message.
dispatcher.add_handler(MessageHandler(Filters.text, reply_handler))
if __name__ == "__main__":
# Running server
app.run(debug=True)

Try it
Something strange,
why?

IDS modules(Doc)
// NLU API 回傳結構
{
"data": {
"nli": [
{
"desc_obj" : {
"result" : "",
"status" : int
},
"data_obj" : [
{
"xxx" : ""
}
],
"type" : "xxx"
}
]
},
"status": "ok"
}
// 今天星期幾?
{
"data": {
"nli": [
{
"desc_obj": {
"result": "今天是2017年9月13號星期三。",
"status": 0
},
"type": "date"
}
]
},
"status": "ok"
}
// 講個笑話
{
"data": {
"nli": [
{
"desc_obj": {
"result": "好的,那你聽好嘍。",
"name": "",
"type": "joke",
"status": 0
},
"data_obj": [
{
"content": "海邊,爸爸指著快要落下的太陽神氣的喊道:“下去,下去!”不一會兒,太陽果然下去了,孩童也看呆了,一面鼓掌,一面説:“爸爸,再來一次,再來一次!” "
}
],
"type": "joke"
}
]
},
"status": "ok"
}
Selection type(Doc)
// 今日新聞
{
"data": {
"nli": [
{
"desc_obj": {
"result":"主人,查看詳情,請說第幾條,更多新聞,請說下一頁。",
"type":"news",
"status":0
},
"data_obj": [
{
"image_url":"https://static.ettoday.net/images/2922/b2922335.jpg",
"ref_url":"http://feedproxy.google.com/~r/ettoday/star/~3/8MAnn0ZHPv8/1051340.htm",
"time":"2017-11-13",
"detail":"愷樂近日推出第二波主打《三人行》,特邀老闆羅志祥、好友鼓鼓跨刀演唱,這首歌最初構想來自羅志祥,他親自打電話向好友鼓鼓邀歌,不只譜曲還要寫詞,而且還特別要求要三人對唱。而兩人也分享追求女生的方法,沒想到",
"title":"愷樂尬鼓鼓、羅志祥合唱! 「2天寫曲」互飆Rap帥炸"
},
{
"image_url":"https://static.ettoday.net/images/2922/b2922289.jpg",
"ref_url":"http://feedproxy.google.com/~r/ettoday/star/~3/Gv3ZxlCBeAA/1051326.htm",
"time":"2017-11-13",
"detail":"小甜甜張可昀及王宇婕隨著《閨蜜的奇幻旅程》赴泰國錄影,製作單位幫她完成三年多來的心願。這次閨蜜的旅程中邀請甜甜的乾姊加入,製作單位騙她將出發去某一個比較遠的廟宇參拜,沒想到一下車眼前出現的是乾姊與龍波",
"title":"小甜甜懼怕跳水 王宇婕一把抱住:相信我會接住妳"
}...
],
"type":"selection"
}
]
},
"status": "ok"
}
Implement intent detection
import configparser
import json
import logging
import time
from hashlib import md5
import requests
config = configparser.ConfigParser()
config.read('config.ini')
logger = logging.getLogger(__name__)
class NliStatusError(Exception):
"""The NLI result status is not 'ok'"""
class Olami:
URL = 'https://tw.olami.ai/cloudservice/api'
def __init__(self, app_key=config['OLAMI']['APP_KEY'], app_secret=config['OLAMI']['APP_SECRET'], input_type=1):
self.app_key = app_key
self.app_secret = app_secret
self.input_type = input_type
def nli(self, text, cusid=None):
response = requests.post(self.URL, params=self._gen_parameters('nli', text, cusid))
response.raise_for_status()
response_json = response.json()
if response_json['status'] != 'ok':
raise NliStatusError("NLI responded status != 'ok': {}".format(response_json['status']))
else:
nli_obj = response_json['data']['nli'][0]
return self.intent_detection(nli_obj)
def _gen_parameters(self, api, text, cusid):
timestamp_ms = (int(time.time() * 1000))
params = {'appkey': self.app_key,
'api': api,
'timestamp': timestamp_ms,
'sign': self._gen_sign(api, timestamp_ms),
'rq': self._gen_rq(text)}
if cusid is not None:
params.update(cusid=cusid)
return params
def _gen_sign(self, api, timestamp_ms):
data = self.app_secret + 'api=' + api + 'appkey=' + self.app_key + 'timestamp=' + \
str(timestamp_ms) + self.app_secret
return md5(data.encode('ascii')).hexdigest()
def _gen_rq(self, text):
obj = {'data_type': 'stt', 'data': {'input_type': self.input_type, 'text': text}}
return json.dumps(obj)
def intent_detection(self, nli_obj):
def handle_selection_type(type):
if type == 'news':
return desc['result'] + '\n\n' + '\n'.join(
str(index + 1) + '. ' + el['title'] for index, el in enumerate(data))
elif type == 'poem':
return desc['result'] + '\n\n' + '\n'.join(
str(index + 1) + '. ' + el['poem_name'] + ',作者:' + el['author'] for index, el in enumerate(data))
elif type == 'cooking':
return # TODO
else:
return '對不起,你說的我還不懂,能換個說法嗎?'
type = nli_obj['type']
desc = nli_obj['desc_obj']
data = nli_obj.get('data_obj', [])
if type == 'kkbox':
id = data[0]['id']
return ('https://widget.kkbox.com/v1/?type=song&id=' + id) if len(data) > 0 else desc['result']
elif type == 'news':
return data[0]['detail']
elif type == 'baike':
return # TODO
elif type == 'joke':
return # TODO
elif type == 'cooking':
return data[0]['content']
elif type == 'selection':
return handle_selection_type(desc['type'])
elif type == 'ds':
return desc['result'] + '\n請用 /help 指令看看我能怎麼幫助您'
else:
return desc['result']
update olami.py

Well done!
Add music skill


KKBOX Open API(link)
What data it provide?(see KUBE)
KKBOX Open API
Get access token(doc)



Search API(doc)
Search API response
{
"playlists": {
"data": [
{
"id": "DYmnfdp1w4Kz4xVQcU",
"title": "古典音樂花房 歷年精選",
"description": "2018-10",
"url": "https://event.kkbox.com/content/playlist/DYmnfdp1w4Kz4xVQcU",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/57960105v17/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/57960105v17/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/57960105v17/cropresize/1000x1000.jpg"
}
],
"updated_at": "2018-10-01T03:08:57+00:00",
"owner": {
"id": "T-YMdq3gnf6AVbuqgt",
"url": "https://www.kkbox.com/tw/profile/T-YMdq3gnf6AVbuqgt",
"name": "KKBOX",
"description": "",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/noimg/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/noimg/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/noimg/cropresize/300x300.jpg"
}
]
}
},
{
"id": "4pFzHUR4FJSOMvK8Sy",
"title": "第60屆葛萊美古典音樂獎入圍",
"description": "",
"url": "https://event.kkbox.com/content/playlist/4pFzHUR4FJSOMvK8Sy",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/60456680v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/60456680v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/60456680v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-11-29T03:00:59+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "1Znzs_MOPcQzNd9EPb",
"title": "古典音樂廚房:下廚日記",
"description": "邊下廚邊聽音樂,讓你在廚房裡不寂寞\r\n伴隨悠揚的古典音樂,讓鍋盤中的食物隨著音符跳躍\r\n細細悠然,輕挑而入耳,享受美味的香氣和美妙的樂聲",
"url": "https://event.kkbox.com/content/playlist/1Znzs_MOPcQzNd9EPb",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/28991876v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/28991876v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/28991876v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-11-23T09:45:20+00:00",
"owner": {
"id": "D_YoomYcGauVGLHfua",
"url": "https://www.kkbox.com/tw/profile/D_YoomYcGauVGLHfua",
"name": "索尼大台柱",
"description": "這次我要播很多 華語 類別的歌曲,快來跟我一起聽音樂吧!",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/80343705v128/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/80343705v128/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/80343705v128/cropresize/300x300.jpg"
}
]
}
},
{
"id": "-nuSHQfVRZWo-BwfQi",
"title": "男生把妹必備的古典音樂",
"description": "男生把妹必備的古典音樂\r\n快存在手機吧!這是初級認識古典音樂的小入門,帥氣又不失浪漫,很好用。",
"url": "https://event.kkbox.com/content/playlist/-nuSHQfVRZWo-BwfQi",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/35325465v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/35325465v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/35325465v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2016-08-19T08:50:26+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "1Z14wWMuPcQzNak1AX",
"title": "2017留聲機古典音樂大獎",
"description": "",
"url": "https://event.kkbox.com/content/playlist/1Z14wWMuPcQzNak1AX",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/60020428v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/60020428v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/60020428v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-09-06T10:30:44+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "4sSRz7XoNYyiXqEIoA",
"title": "古典音樂的藍色海洋傳說",
"description": "Vivaldi 「La tempesta di mare」海上風暴、艾爾加 Elgar 「Sea Pictures, Op. 37」海景、R. Vaughan Williams 「A Sea Symphony 」海的交響曲、法國作曲家德布西Debussy的La Mer「海」…四個大海整座被我放入歌單!但也可聽到有關海洋的小作有現代古典到跨界,請進入奇幻不設限的古典音樂藍色海洋傳說。",
"url": "https://event.kkbox.com/content/playlist/4sSRz7XoNYyiXqEIoA",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/44673710v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/44673710v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/44673710v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2016-12-16T09:33:22+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "-q40NGVA8C9JyV0-Qd",
"title": "婚禮音樂 古典首選",
"description": "結婚的人可以參考,沒有打算結婚得更可以欣賞。當然這些曲目多少都有”婚禮”音樂的背景或故事,聽起來喜悦動人,想結婚了嗎?可以自己排一張屬於你的婚禮音樂喔!",
"url": "https://event.kkbox.com/content/playlist/-q40NGVA8C9JyV0-Qd",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/31039846v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/31039846v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/31039846v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2018-07-03T08:47:23+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "4o5zjH4hBrbXxia-gb",
"title": "古典音樂廚房:餐桌盛宴",
"description": "邊下廚邊聽音樂,讓你在廚房裡不寂寞\r\n隨著旋律擺動,隨處就是你的舞台\r\n透過音符的魔法,讓料理更佳美味\r\n最棒的聽覺與味覺饗宴,今晚,你選哪一道?",
"url": "https://event.kkbox.com/content/playlist/4o5zjH4hBrbXxia-gb",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/28530925v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/28530925v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/28530925v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-12-10T10:59:25+00:00",
"owner": {
"id": "D_YoomYcGauVGLHfua",
"url": "https://www.kkbox.com/tw/profile/D_YoomYcGauVGLHfua",
"name": "索尼大台柱",
"description": "這次我要播很多 華語 類別的歌曲,快來跟我一起聽音樂吧!",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/80343705v128/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/80343705v128/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/80343705v128/cropresize/300x300.jpg"
}
]
}
},
{
"id": "OllyeK1N5edzHdUvG_",
"title": "波西米亞與古典音樂",
"description": "波希米亞主義(Bohemianism)統指稱那些希望過非傳統生活風格的一群藝術家、作家與任何對傳統不抱持幻想的人的一種生活方式。但”波希米亞人”是指”捷克波希米亞”省的當地人。波希米亞人的第二個涵義卻是出現在19世紀的法國。這個詞反映了15世紀以來法國人對來自於波希米亞的吉普賽人的觀感。\r\n「波希米亞」是個地理名詞,「吉普賽」卻是種族名稱。\r\n「波希米亞」這個地名,範圍是在目前捷克西部地區的「波希米亞省」拉丁文書寫成「Boii」,早期因戰亂而衰弱,直到奧匈帝國結束,為了推動一個不具歧視聯想的民族代名詞,「捷克(Czech)」這個字在二十世紀才逐漸取代「波西米亞」。所以「波希米亞人」和「吉普賽人」定義並不盡相同。歌單中收錄居住在當時波西米亞省的古典作曲家作品如 史麥塔納、德弗札克、馬勒等。",
"url": "https://event.kkbox.com/content/playlist/OllyeK1N5edzHdUvG_",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/52010540v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/52010540v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/52010540v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-03-10T09:54:04+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "LZCDWuESYzN164sdpL",
"title": "取樣翻玩古典音樂的西洋流行音樂 (上)",
"description": "當古典音樂穿越時空被西洋流行音樂取樣翻玩,\r\n\r\n貝多芬遇見 Lukas Graham、Robin Thicke 和 Nas、\r\n\r\n巴哈碰到 Sweetbox、One Republic 和 Jem、\r\n\r\n佛瑞 和 Little Mix 有了交集、\r\n\r\n李斯特和 Beyoncé 產生共鳴、 \r\n\r\n那就是這份歌單了。",
"url": "https://event.kkbox.com/content/playlist/LZCDWuESYzN164sdpL",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/26561988v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/26561988v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/26561988v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2016-04-24T14:12:55+00:00",
"owner": {
"id": "ClhY2c4XdP1wYK4Qz6",
"url": "https://www.kkbox.com/tw/profile/ClhY2c4XdP1wYK4Qz6",
"name": "DJ Will (張譽鐘)",
"description": "塵世迷途中的小小音樂人,歷經快五年被古典樂矯正叛逆的童年,不只五年用力搖滾東西洋樂團駐唱的青春,接著享受追逐著每週西洋排行榜單上新專輯和爵士、電影音樂的美妙,曾入圍廣播金鐘獎,專訪葛萊美獎、金曲獎等國內外傑出音樂人,現任臺北電臺節目製作主持人。",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/6458752v5/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/6458752v5/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/6458752v5/cropresize/300x300.jpg"
}
]
}
},
{
"id": "PXa2t3wuT1DRMOnHmP",
"title": "古典音樂中孤獨又燦爛的鬼怪",
"description": "我們常聽到這樣的作品被應用在電影電視配樂中,而這些知名的古典作曲家當時創作的背景,也是有關孤獨又燦爛的鬼怪故事呢。\r\n\r\n1.聖桑/骷髏之舞\r\n2.帕格尼尼/魔鬼的笑聲\r\n3.莫札特/魔笛夜后/我心燃燒著地獄般的仇恨\r\n4.舒伯特/死神與少女\r\n5.歌劇魅影/暗夜樂章\r\n6.朱塞佩·塔替尼/魔鬼的顫音\r\n7.舒伯特/魔王\r\n8.穆索爾斯基/荒山之夜\r\n9.古諾/浮士德\r\n10.拉威爾/夜裡的加斯巴「水之精靈」(Ondine)\r\n11.「絞刑架」(Le Gibet)\r\n12.「小魔鬼」(Scarbo)\r\n13.莫札特/唐.喬凡尼 \r\n14.貝多芬/鬼魂鋼琴三重奏\r\n15.白遼士/女巫沙柏特的夜夢",
"url": "https://event.kkbox.com/content/playlist/PXa2t3wuT1DRMOnHmP",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/44720524v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/44720524v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/44720524v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2016-12-23T06:26:10+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "PZUO_gIuMWFJrCcAoZ",
"title": "取樣翻玩古典音樂的西洋流行音樂 (下)",
"description": "當古典音樂穿越時空被西洋流行音樂取樣翻玩,\r\n\r\n帕海貝爾 優雅的(卡農)遇見痞痞的 Coolio、\r\n\r\n薩悌 悲傷的(吉諾佩第) 被 Janet Jackson 翻轉情緒、\r\n\r\n奧夫 壯闊的(布蘭詩歌)搭上東岸饒舌大腕 Nas 和 P.Daddy、\r\n\r\n普羅高菲夫 蕩氣迴腸的(羅密歐與茱麗葉) 遇見歌聲一樣有戲的 Sia、\r\n\r\n羅西尼 聽見一樣有才的 MIKA、\r\n\r\n天才神童莫札特 被古靈精怪的 Clean Bandit撞到、\r\n\r\n浪漫的德布西 第一眼看見才氣過人的 Janelle Monáe 和 Alicia Keys、\r\n\r\n這就是那個他們相遇的時刻。",
"url": "https://event.kkbox.com/content/playlist/PZUO_gIuMWFJrCcAoZ",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/26554084v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/26554084v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/26554084v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2016-04-20T01:31:47+00:00",
"owner": {
"id": "ClhY2c4XdP1wYK4Qz6",
"url": "https://www.kkbox.com/tw/profile/ClhY2c4XdP1wYK4Qz6",
"name": "DJ Will (張譽鐘)",
"description": "塵世迷途中的小小音樂人,歷經快五年被古典樂矯正叛逆的童年,不只五年用力搖滾東西洋樂團駐唱的青春,接著享受追逐著每週西洋排行榜單上新專輯和爵士、電影音樂的美妙,曾入圍廣播金鐘獎,專訪葛萊美獎、金曲獎等國內外傑出音樂人,現任臺北電臺節目製作主持人。",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/6458752v5/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/6458752v5/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/6458752v5/cropresize/300x300.jpg"
}
]
}
},
{
"id": "9X7BWQMSUFThPIbD2I",
"title": "古典音樂花房 -古典樂愛好者的花園",
"description": "滿滿的古典芬芳小品,放空吧!隨著音符一起進入夢幻花園......",
"url": "https://event.kkbox.com/content/playlist/9X7BWQMSUFThPIbD2I",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/61625021v4/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/61625021v4/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/61625021v4/cropresize/1000x1000.jpg"
}
],
"updated_at": "2018-05-07T15:32:33+00:00",
"owner": {
"id": "Sqq8A0t62GqA2ZME-u",
"url": "https://www.kkbox.com/tw/profile/Sqq8A0t62GqA2ZME-u",
"name": "音樂家宇宙日誌 Musiker",
"description": "情境音樂頻道\r\n每週一至五,上午九點至下午五點\r\n在 KKBOX 一起聽和你 Say Hi \r\n我們有 IG :https://www.instagram.com/musiker.loveyouandmusic/",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/2023280v10/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/2023280v10/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/2023280v10/cropresize/300x300.jpg"
}
]
}
},
{
"id": "GseRRO7TqlVekkF6Vp",
"title": "第59屆葛萊美獎[古典音樂]入圍",
"description": "",
"url": "https://event.kkbox.com/content/playlist/GseRRO7TqlVekkF6Vp",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/44646119v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/44646119v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/44646119v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2016-12-07T06:33:36+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "HaNeqR68_aVO2ROVun",
"title": "古典音樂家的浪漫",
"description": "",
"url": "https://event.kkbox.com/content/playlist/HaNeqR68_aVO2ROVun",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/61060620v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/61060620v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/61060620v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2018-02-08T08:48:08+00:00",
"owner": {
"id": "5-0rkVNyTkJjX3k0Iq",
"url": "https://www.kkbox.com/tw/profile/5-0rkVNyTkJjX3k0Iq",
"name": "貴族唱片",
"description": "無論哪種音樂類型,都有吸引人的旋律或歌詞並能replay一整天,一起和我們聽聽吧!\rIG:https://www.instagram.com/noblemusictw/\rFB:https://www.facebook.com/noblemusictw/",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/300x300.jpg"
}
]
}
},
{
"id": "8rBfNAOT0-n8SQwYtg",
"title": "古典音樂裡的狗狗跟鳥鳥",
"description": "古典音樂中,有許多跟動物有關的作品,但是這份歌單中,我特別挑選以狗狗跟鳥鳥為主的作品!而大部份的曲目並不是大家所熟知的,希望能跟大家分享,原來古典音樂中,還有這些別出心裁的好音樂:\n\n管弦樂版:蓋希文的“遛狗去” ,雷史碧基用新古典語法寫的五個樂章“鳥”作品,佛漢威廉士充滿英國民謠風的“黃蜂序曲”。\n\n鋼琴版:舒曼的“預言鳥”,葛利格抒情小品中的“蝴蝶”跟“小鳥”,薩提寫的三首獻給狗的前奏曲,最後兩首則是印象派大師德布西的“金魚”,跟拉威爾“悲傷的鳥”。",
"url": "https://event.kkbox.com/content/playlist/8rBfNAOT0-n8SQwYtg",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/60205626v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/60205626v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/60205626v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-11-20T07:05:35+00:00",
"owner": {
"id": "-nXHjDkXkOJZCBrXBX",
"url": "https://www.kkbox.com/tw/profile/-nXHjDkXkOJZCBrXBX",
"name": "施孟玟",
"description": "中部老招牌資深古典音樂老師,也是一位熱愛爵士樂到無法自拔的愛樂大嬸,近年來認真推廣爵士樂,藉由古典、爵士音樂講座,把音樂介紹給普羅大眾,自稱是中年婦女界的最佳楷模!",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/12514123v2/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/12514123v2/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/12514123v2/cropresize/300x300.jpg"
}
]
}
},
{
"id": "Kpu5x3HPwNsRjop8Ve",
"title": "Naxos三十週年慕尼黑古典音樂會",
"description": "",
"url": "https://event.kkbox.com/content/playlist/Kpu5x3HPwNsRjop8Ve",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/56972102v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/56972102v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/56972102v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-05-17T09:40:51+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "PXaserweT1DRNbJ8ho",
"title": "樂。古典:春日音樂萬花筒",
"description": "",
"url": "https://event.kkbox.com/content/playlist/PXaserweT1DRNbJ8ho",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/24563085v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/24563085v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/24563085v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2016-03-10T10:05:58+00:00",
"owner": {
"id": "KttvV5OuuGL7-EGabK",
"url": "https://www.kkbox.com/tw/profile/KttvV5OuuGL7-EGabK",
"name": "全球大熱門",
"description": "英文不通沒關係,有時候音樂就是聽一種氛圍、聽一種感動、聽一種…天不怕地不怕的精神啦!為各位介紹包羅萬象的西洋音樂,內行的讓你聽門道,外行的一起湊熱鬧!一起聽搖滾、嘻哈、舞曲、抒情、跨界…一起享受音樂的喜怒哀樂。",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/31438308v101/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/31438308v101/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/31438308v101/cropresize/300x300.jpg"
}
]
}
},
{
"id": "8q4oJr3Aq1RxdNGYL0",
"title": "薄酒萊古典音樂饗宴",
"description": "一起舉杯!",
"url": "https://event.kkbox.com/content/playlist/8q4oJr3Aq1RxdNGYL0",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/17987776v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/17987776v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/17987776v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2015-11-18T09:17:12+00:00",
"owner": {
"id": "8ljyz63jwBeA224nhC",
"url": "https://www.kkbox.com/tw/profile/8ljyz63jwBeA224nhC",
"name": "証聲音樂圖書館 ECHO MUSIC",
"description": "Mon.-Fri. 10:00-18:30 \r\n我們音樂圖書館專門發行你尚未聽過的私藏好聲音。\r\n歡迎跟著証聲一起聽每天為你準備的專屬好音樂~~~",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/41751068v82/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/41751068v82/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/41751068v82/cropresize/300x300.jpg"
}
]
}
},
{
"id": "0tPyuK2wJBduIOzVEF",
"title": "跟著音樂達人林伯杰輕鬆成為古典通",
"description": "文/音樂達人 林伯杰\r\n\r\n台灣知名音樂人,以幽默風趣的文筆與風采帶領人們進入古典樂的世界。現任金革唱片音樂總監、樂樂文化編輯總監、MUZIK古典樂刊專欄主筆以及各公私立機關藝文講座講師。\r\n\r\n○從跨界遇見古典樂○\r\n即使你不曾刻意聆聽古典音樂,但它們就像匪諜般無時無刻出現在你生活周遭,在電影電視裡時時入耳,就連倒垃圾也會被魔音穿腦。於是,有人藉由村上春樹小說按圖索驥,有人透過莎拉布萊曼愛上「公主徹夜未眠」。我個人推薦幾年前的日劇《交響情人夢》,是測試你會不會愛上古典樂的絕佳試金石。(聽說中國開始籌拍《交響情人夢》,可以不要嗎?)\r\n\r\n有道是「昨日之流行,乃今日之經典」,想想小約翰史特勞斯的圓舞曲,在一百多年前可是維也納最Hito的熱門舞曲,如今卻成為我們認定的古典音樂。可以設想,一兩百年後,我們這個世代的音樂還有什麼會流傳下去?或許,我認為電影配樂、音樂劇、爵士樂與探戈等,將會成為我們子孫所謂的「古典音樂」。而這些樂種,自然也很適合當做邁向古典音樂的入門磚。",
"url": "https://event.kkbox.com/content/playlist/0tPyuK2wJBduIOzVEF",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/60055000v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/60055000v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/60055000v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-09-15T07:39:30+00:00",
"owner": {
"id": "WmaEJtorCmWhDR_oJo",
"url": "https://www.kkbox.com/tw/profile/WmaEJtorCmWhDR_oJo",
"name": "JINGO MUSIC 金革音樂",
"description": "金革音樂,陪伴你的任何時刻。https://www.facebook.com/Jingorecord/",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/129238267v11/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/129238267v11/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/129238267v11/cropresize/300x300.jpg"
}
]
}
},
{
"id": "WqnKFY3snmTecvZsLi",
"title": "致親愛的莎翁400年經典。藝術古典感音樂",
"description": "莎士比亞創造了很多名言 詩句和戲劇\r\n在逝世400年之時\r\n緬懷起這位大文豪曾經帶給世界的美好\r\n\r\n也讓自己的心靈 用藝術的音樂沉澱\r\n找回文學的氣息和氣質\r\n用音樂來感受藝術古典的美好\r\n\r\n也用音樂致敬這位藝術楷模!!!",
"url": "https://event.kkbox.com/content/playlist/WqnKFY3snmTecvZsLi",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/26553538v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/26553538v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/26553538v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2016-04-20T01:11:32+00:00",
"owner": {
"id": "9_GX0ig26XV_73JEkG",
"url": "https://www.kkbox.com/tw/profile/9_GX0ig26XV_73JEkG",
"name": "風潮音樂 Wind Music LiFe",
"description": "風潮音樂空間分享音樂予你我的心靈和生活中產生共鳴,讓音樂如同朋友一般陪伴著,ENJOY美好的音樂時光吧!",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/128693558v1/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/128693558v1/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/128693558v1/cropresize/300x300.jpg"
}
]
}
},
{
"id": "5XPKnkV9CAvDECYVCM",
"title": "宮崎駿動畫經典音樂",
"description": "",
"url": "https://event.kkbox.com/content/playlist/5XPKnkV9CAvDECYVCM",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/19347168v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/19347168v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/19347168v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2016-01-04T02:50:48+00:00",
"owner": {
"id": "5-0rkVNyTkJjX3k0Iq",
"url": "https://www.kkbox.com/tw/profile/5-0rkVNyTkJjX3k0Iq",
"name": "貴族唱片",
"description": "無論哪種音樂類型,都有吸引人的旋律或歌詞並能replay一整天,一起和我們聽聽吧!\rIG:https://www.instagram.com/noblemusictw/\rFB:https://www.facebook.com/noblemusictw/",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/300x300.jpg"
}
]
}
},
{
"id": "GtUJGLP-D--02NwK03",
"title": "蔣三省經典音樂會3/1",
"description": "",
"url": "https://event.kkbox.com/content/playlist/GtUJGLP-D--02NwK03",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/51975315v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/51975315v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/51975315v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-03-01T13:27:28+00:00",
"owner": {
"id": "-liv_F_fa_TNkISRM5",
"url": "https://www.kkbox.com/tw/profile/-liv_F_fa_TNkISRM5",
"name": "蔣三省 JMS",
"description": "蔣三省\n華語流行音樂界知名製作人。他在音樂創作與音樂教育雙重領域,歷經四十多年持續擁有一席之地。曾入圍並多次擔任金曲獎評審委員,擔任過飛碟、點將、華納、歌林、BMG、寶麗金等唱片公司製作人,製作張清芳、林慧萍、蔡幸娟、姜育恆等歌手暢銷專輯,創作無數金曲。",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/338070577369v2/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/338070577369v2/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/338070577369v2/cropresize/300x300.jpg"
}
]
}
},
{
"id": "8riFCwOj0-n8TI8u5j",
"title": "嚴選經典音樂劇名曲",
"description": "嚴選「伊莉莎白」、「西貢小姐」、「紅花俠」、「羅密歐與茱麗葉」、「莫札特」等知名音樂劇劇中名曲,你最喜歡哪部作品呢?",
"url": "https://event.kkbox.com/content/playlist/8riFCwOj0-n8TI8u5j",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/6687159v2/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/6687159v2/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/6687159v2/cropresize/1000x1000.jpg"
}
],
"updated_at": "2015-06-17T14:01:51+00:00",
"owner": {
"id": "5XIttVqe0foATcIFMp",
"url": "https://www.kkbox.com/tw/profile/5XIttVqe0foATcIFMp",
"name": "KKBOX 西洋小編",
"description": "",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/94563062v1/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/94563062v1/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/94563062v1/cropresize/300x300.jpg"
}
]
}
},
{
"id": "CsR5efkotRtI6Ds2B6",
"title": "來自台灣的古典精品 Taiwan (更新)",
"description": "這是來自台灣的古典音樂及演奏家",
"url": "https://event.kkbox.com/content/playlist/CsR5efkotRtI6Ds2B6",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/28801640v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/28801640v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/28801640v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-02-06T06:30:15+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "5ZunaoQ0Mpn-zqReaz",
"title": "聽見古典吉他的優雅內蘊",
"description": "歌單中有許多大腕級的古典吉他高手,曲目精選在古典音樂類別。",
"url": "https://event.kkbox.com/content/playlist/5ZunaoQ0Mpn-zqReaz",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/39827536v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/39827536v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/39827536v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2016-10-14T07:08:55+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "8k3NnX0gMgMI2wUeKY",
"title": "陽光下的慵懶,聆聽古典樂的美好",
"description": "午後陽光灑落,在硬石上慵懶倚靠,聆聽古典音樂,享受這寧靜的美好...",
"url": "https://event.kkbox.com/content/playlist/8k3NnX0gMgMI2wUeKY",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/54492528v2/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/54492528v2/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/54492528v2/cropresize/1000x1000.jpg"
}
],
"updated_at": "2018-08-16T06:12:38+00:00",
"owner": {
"id": "5-0rkVNyTkJjX3k0Iq",
"url": "https://www.kkbox.com/tw/profile/5-0rkVNyTkJjX3k0Iq",
"name": "貴族唱片",
"description": "無論哪種音樂類型,都有吸引人的旋律或歌詞並能replay一整天,一起和我們聽聽吧!\rIG:https://www.instagram.com/noblemusictw/\rFB:https://www.facebook.com/noblemusictw/",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/300x300.jpg"
}
]
}
},
{
"id": "GmuxiL3hoIXuIByVPt",
"title": "古典配上陽光和美食",
"description": "沒有人聲的干擾,古典音樂最適合當野餐時間的最佳配角!",
"url": "https://event.kkbox.com/content/playlist/GmuxiL3hoIXuIByVPt",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/11353360v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/11353360v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/11353360v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2015-12-12T17:49:19+00:00",
"owner": {
"id": "L-SE6qZKdZM0zpUHtO",
"url": "https://www.kkbox.com/tw/profile/L-SE6qZKdZM0zpUHtO",
"name": "KKBOX 古典小編",
"description": "",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/96156911v1/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/96156911v1/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/96156911v1/cropresize/300x300.jpg"
}
]
}
},
{
"id": "P-POMJSCJkzqpyyH0i",
"title": "綿密甜點的古典樂章",
"description": "Dolce 拿來形容樂章 是甜還是蜜 其中綿密的感覺緩緩的速度進行\n# Dolce義大利文 甜點 甜蜜 可用於古典音樂術語",
"url": "https://event.kkbox.com/content/playlist/P-POMJSCJkzqpyyH0i",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/59902323v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/59902323v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/59902323v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-11-23T07:52:54+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "8rJYlNOT0-n8Qd0rOc",
"title": "聖誕快樂2018古典大福袋 (12/31更新)",
"description": "每年聖誕節古典音樂,都會出很多應景的專輯。也順便打著鈴聲告知要過年了,這次從十二月開始,我將不斷更新福袋的內容,充滿驚喜!也希望給你帶來滿滿的福氣。",
"url": "https://event.kkbox.com/content/playlist/8rJYlNOT0-n8Qd0rOc",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/60543002v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/60543002v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/60543002v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-12-31T01:23:09+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "1_8XT_nD-qZsEyrKXO",
"title": "古典音樂花房 - Classical Music for Sleep (古典音樂花房:舒眠曲集)",
"description": "",
"url": "https://event.kkbox.com/content/playlist/1_8XT_nD-qZsEyrKXO",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/56993079v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/56993079v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/56993079v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-05-22T13:43:13+00:00",
"owner": {
"id": "1ZawjEisTOnUAenzj2",
"url": "https://www.kkbox.com/tw/profile/1ZawjEisTOnUAenzj2",
"name": "Sammi",
"description": "",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/123919088v2/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/123919088v2/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/123919088v2/cropresize/300x300.jpg"
}
]
}
},
{
"id": "_akVP6ewhzgngrItl5",
"title": "親親寶貝,愛上古典樂",
"description": "專門為學齡前小朋友、同時也是獻給爸爸媽媽與孩子創造共同聆聽時光的古典音樂選集。",
"url": "https://event.kkbox.com/content/playlist/_akVP6ewhzgngrItl5",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/52040085v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/52040085v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/52040085v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-03-20T07:16:04+00:00",
"owner": {
"id": "D_YoomYcGauVGLHfua",
"url": "https://www.kkbox.com/tw/profile/D_YoomYcGauVGLHfua",
"name": "索尼大台柱",
"description": "這次我要播很多 華語 類別的歌曲,快來跟我一起聽音樂吧!",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/80343705v128/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/80343705v128/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/80343705v128/cropresize/300x300.jpg"
}
]
}
},
{
"id": "KpECC5H_wNsRj0ptsZ",
"title": "就是要打破你對古典樂催眠的印象",
"description": "誰說古典樂只能在音樂廳昏昏欲睡?就是要用古典樂曲振奮你的心!",
"url": "https://event.kkbox.com/content/playlist/KpECC5H_wNsRj0ptsZ",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/11268077v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/11268077v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/11268077v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2015-08-11T03:09:58+00:00",
"owner": {
"id": "L-SE6qZKdZM0zpUHtO",
"url": "https://www.kkbox.com/tw/profile/L-SE6qZKdZM0zpUHtO",
"name": "KKBOX 古典小編",
"description": "",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/96156911v1/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/96156911v1/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/96156911v1/cropresize/300x300.jpg"
}
]
}
},
{
"id": "XYFmQuZVwbbDLCKy9I",
"title": "文藝復興古典正好眠",
"description": "文藝復興時期的古典音樂 簡單 不複雜 沒有過多的起伏衝突 淡淡的 動機也單純 聽聽養心 休息 睡眠 都有不錯的聯想。",
"url": "https://event.kkbox.com/content/playlist/XYFmQuZVwbbDLCKy9I",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/60020413v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/60020413v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/60020413v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-09-08T09:59:44+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "OoLxjJuOUsmp7dspED",
"title": "古典音樂花房 - Classical Music for Relax (古典音樂花房:忘憂曲集)",
"description": "",
"url": "https://event.kkbox.com/content/playlist/OoLxjJuOUsmp7dspED",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/61100017v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/61100017v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/61100017v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2018-02-16T06:41:43+00:00",
"owner": {
"id": "LYSJ4f2VZxyBifxrUp",
"url": "https://www.kkbox.com/tw/profile/LYSJ4f2VZxyBifxrUp",
"name": "Popu Hsiao",
"description": "",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/43052306v1/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/43052306v1/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/43052306v1/cropresize/300x300.jpg"
}
]
}
},
{
"id": "X-Rb7M-owzDxkaV6Z2",
"title": "韓劇配樂中大量的古典",
"description": "密會一劇中大量的使用古典鋼琴的曲目相當的高雅不可擋再融入其它韓劇配樂裡以古典的編法之作不失為接觸古典或了解古典音樂迷人之處。古典也是有劇情的。",
"url": "https://event.kkbox.com/content/playlist/X-Rb7M-owzDxkaV6Z2",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/28991689v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/28991689v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/28991689v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2016-06-03T10:16:12+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "OtIUvheLPHGat18Q6g",
"title": "古典與華語流行的融合",
"description": "你聽的出來哪一首華語歌曲有用到古典音樂嗎?",
"url": "https://event.kkbox.com/content/playlist/OtIUvheLPHGat18Q6g",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/31043179v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/31043179v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/31043179v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2018-08-16T06:16:16+00:00",
"owner": {
"id": "5-0rkVNyTkJjX3k0Iq",
"url": "https://www.kkbox.com/tw/profile/5-0rkVNyTkJjX3k0Iq",
"name": "貴族唱片",
"description": "無論哪種音樂類型,都有吸引人的旋律或歌詞並能replay一整天,一起和我們聽聽吧!\rIG:https://www.instagram.com/noblemusictw/\rFB:https://www.facebook.com/noblemusictw/",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/300x300.jpg"
}
]
}
},
{
"id": "CqO-gQnPpwXnZKOXu1",
"title": "春風沉醉電影中的古典夜晚",
"description": "提到電影中的古典音樂,多是有古典專輯挑出曲目 \r\n其實電影影片是有自己的節奏不太可能為了哪個古典樂曲而重新配剪,這歌單的特殊之處是我從真正電影原聲帶裡的古典音樂一首首找出來(因有的未必會收錄),可以發現,很多都是配樂家自己所彈過或重新編合,加入電影主題的旋律或另請單位重新演奏,很難得的一張古典與電影配樂中真實關係的歌單。引用了”春風沉醉的夜晚”電影,”那些花兒”也收錄其中。",
"url": "https://event.kkbox.com/content/playlist/CqO-gQnPpwXnZKOXu1",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/54522375v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/54522375v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/54522375v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-07-13T07:10:21+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "Omnjy9TZy7BGeiZNGX",
"title": "那些成就我的古典樂、歌手及樂團",
"description": "林暐哲:「我最初是從古典音樂開始,也受到古典樂非常深的影響,在擔任製作人時,也都有從古典樂的概念取經。」\r\n\r\n\r\nFrom 風和日麗唱片和「音樂啟蒙 – 他們都是這樣聽音樂的」系列講座",
"url": "https://event.kkbox.com/content/playlist/Omnjy9TZy7BGeiZNGX",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/52086430v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/52086430v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/52086430v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-04-07T10:25:48+00:00",
"owner": {
"id": "CmKH4D8szn2YjmGeDM",
"url": "https://www.kkbox.com/tw/profile/CmKH4D8szn2YjmGeDM",
"name": "風和日麗唱片行",
"description": "從風和日麗出發, 我們將前往美好的音樂國度, 天氣未必時時晴朗, 旅途卻勢必精彩萬分。 如果每次演出,每張專輯,都是一趟旅程…\r\n\r\n",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/125933966v5/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/125933966v5/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/125933966v5/cropresize/300x300.jpg"
}
]
}
},
{
"id": "9aWUHKX8IfG-KgHiw_",
"title": "畢業祭,催淚系古典鋼琴:畢典必備歌單",
"description": "又是一年梔子花開,又到一年畢業季來。此時此刻,適合聽哪些古典音樂?畢業典禮必備的20首古典回憶曲目",
"url": "https://event.kkbox.com/content/playlist/9aWUHKX8IfG-KgHiw_",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/28801203v3/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/28801203v3/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/28801203v3/cropresize/1000x1000.jpg"
}
],
"updated_at": "2018-06-07T01:28:53+00:00",
"owner": {
"id": "5-0rkVNyTkJjX3k0Iq",
"url": "https://www.kkbox.com/tw/profile/5-0rkVNyTkJjX3k0Iq",
"name": "貴族唱片",
"description": "無論哪種音樂類型,都有吸引人的旋律或歌詞並能replay一整天,一起和我們聽聽吧!\rIG:https://www.instagram.com/noblemusictw/\rFB:https://www.facebook.com/noblemusictw/",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/5323612v36/cropresize/300x300.jpg"
}
]
}
},
{
"id": "1ZeZ2PMePcQzOgpTy4",
"title": "古典音樂/活動用",
"description": "就是這樣",
"url": "https://event.kkbox.com/content/playlist/1ZeZ2PMePcQzOgpTy4",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/706402v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/706402v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/706402v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2015-08-05T18:51:55+00:00",
"owner": {
"id": "Kqoip9dSldEiKs1-Y1",
"url": "https://www.kkbox.com/tw/profile/Kqoip9dSldEiKs1-Y1",
"name": "CrushZion",
"description": "",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/noimg/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/noimg/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/noimg/cropresize/300x300.jpg"
}
]
}
},
{
"id": "Sqh6dlE2219sKB4MxU",
"title": "Skyline 天際線爵士樂團",
"description": "爵士、古典、世界音樂的完美融合 橫跨全球五大洲華麗旅程",
"url": "https://event.kkbox.com/content/playlist/Sqh6dlE2219sKB4MxU",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/62282414v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/62282414v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/62282414v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2018-08-08T02:09:44+00:00",
"owner": {
"id": "4sr2MkSmWI-OwNsrJc",
"url": "https://www.kkbox.com/tw/profile/4sr2MkSmWI-OwNsrJc",
"name": "布萊德船長",
"description": "跟著音樂一起酷,讓我們航向品牌的航道吧!",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/338065226046v1/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/338065226046v1/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/338065226046v1/cropresize/300x300.jpg"
}
]
}
},
{
"id": "0l5CB0tWFlT-7mjk3z",
"title": "優雅古典音樂花房 ",
"description": "我的春夏生活風味",
"url": "https://event.kkbox.com/content/playlist/0l5CB0tWFlT-7mjk3z",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/54472249v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/54472249v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/54472249v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-04-16T08:14:41+00:00",
"owner": {
"id": "GlJGyAguISe0ySqCdI",
"url": "https://www.kkbox.com/tw/profile/GlJGyAguISe0ySqCdI",
"name": "莎莎S",
"description": "說說唱唱",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/26983081v15/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/26983081v15/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/26983081v15/cropresize/300x300.jpg"
}
]
}
},
{
"id": "-tGIMEKBSIS_w5yf1C",
"title": "寶寶睡眠音樂冠軍排行優質選集",
"description": "嚴選寶寶睡眠音樂排行榜上的曲目,從真實的古典樂器演奏,天然養成寶寶對音色美學的先入認知。",
"url": "https://event.kkbox.com/content/playlist/-tGIMEKBSIS_w5yf1C",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/59828491v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/59828491v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/59828491v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-08-29T04:05:22+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "CqXzSynPpwXnZPg4Ry",
"title": "古典發熱包:溫暖過冬",
"description": "寒冷的冬天就以溫暖的音樂包覆身心!【古典發熱包:溫暖過冬】橫越古典、爵士、跨界音樂、電影原聲帶,輕盈的歌聲與豐富交織的琴聲,時刻為生活加溫。",
"url": "https://event.kkbox.com/content/playlist/CqXzSynPpwXnZPg4Ry",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/60632675v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/60632675v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/60632675v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-12-15T06:13:09+00:00",
"owner": {
"id": "D_YoomYcGauVGLHfua",
"url": "https://www.kkbox.com/tw/profile/D_YoomYcGauVGLHfua",
"name": "索尼大台柱",
"description": "這次我要播很多 華語 類別的歌曲,快來跟我一起聽音樂吧!",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/80343705v128/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/80343705v128/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/80343705v128/cropresize/300x300.jpg"
}
]
}
},
{
"id": "4l0ikP5chw1HtGomyJ",
"title": "古典音樂花房, Chopin - Classical Music for Dinner (古典音樂花房:燭光晚餐曲)",
"description": "",
"url": "https://event.kkbox.com/content/playlist/4l0ikP5chw1HtGomyJ",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/59564865v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/59564865v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/59564865v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-06-27T15:55:22+00:00",
"owner": {
"id": "_YZFwln5ByXQoE7ZOh",
"url": "https://www.kkbox.com/tw/profile/_YZFwln5ByXQoE7ZOh",
"name": "神祕嘉賓",
"description": "",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/noimg/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/noimg/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/noimg/cropresize/300x300.jpg"
}
]
}
},
{
"id": "L-HFdYf3ZKwmxqwphZ",
"title": "Hola!濃烈的西班牙古典",
"description": "西班牙因地理位置及民族的特性,其音樂都有著迷人熱情的結構:流暢的旋律、晃蕩的拍子,古典音樂也是。亦可追朔到中世紀宗教性樸實的單純。",
"url": "https://event.kkbox.com/content/playlist/L-HFdYf3ZKwmxqwphZ",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/57004821v2/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/57004821v2/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/57004821v2/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-05-26T08:04:28+00:00",
"owner": {
"id": "4nZOCDKORLgbsSWpDb",
"url": "https://www.kkbox.com/tw/profile/4nZOCDKORLgbsSWpDb",
"name": "祖崇",
"description": "歌單可以是短文、長詩或小說。享閱其中,生活的好陪伴。\r\n\r\n#想聽甚麼可以告訴我",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/142687519v4/cropresize/300x300.jpg"
}
]
}
},
{
"id": "-kJH3a4ANZlr5eVvBA",
"title": "古典音樂合輯1",
"description": "很棒的背景音樂",
"url": "https://event.kkbox.com/content/playlist/-kJH3a4ANZlr5eVvBA",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/62688897v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/62688897v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/62688897v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2018-10-02T01:03:37+00:00",
"owner": {
"id": "1_mAWj4Y2MLMVjlAXV",
"url": "https://www.kkbox.com/tw/profile/1_mAWj4Y2MLMVjlAXV",
"name": "曉綾",
"description": "",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/11834721v1/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/11834721v1/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/11834721v1/cropresize/300x300.jpg"
}
]
}
},
{
"id": "8qgtrX3gq1RxfOrNEc",
"title": "古典音樂",
"description": "",
"url": "https://event.kkbox.com/content/playlist/8qgtrX3gq1RxfOrNEc",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/61743264v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/61743264v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/61743264v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2018-05-26T04:04:21+00:00",
"owner": {
"id": "CpHGiaYOsUlYTCP3gf",
"url": "https://www.kkbox.com/tw/profile/CpHGiaYOsUlYTCP3gf",
"name": "神祕嘉賓",
"description": "",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/noimg/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/noimg/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/noimg/cropresize/300x300.jpg"
}
]
}
},
{
"id": "LX0FPtX2qyA6RCfA23",
"title": "好詩意,不是失意",
"description": "古典與電音的碰撞\r\n古典與R&B的碰撞\r\n古典與現代音樂的碰撞\r\n多元融合的音樂層次",
"url": "https://event.kkbox.com/content/playlist/LX0FPtX2qyA6RCfA23",
"images": [
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/playlist/global/60069910v1/cropresize/300x300.jpg"
},
{
"height": 600,
"width": 600,
"url": "https://i.kfs.io/playlist/global/60069910v1/cropresize/600x600.jpg"
},
{
"height": 1000,
"width": 1000,
"url": "https://i.kfs.io/playlist/global/60069910v1/cropresize/1000x1000.jpg"
}
],
"updated_at": "2017-09-22T02:29:01+00:00",
"owner": {
"id": "KmTYDfo5gXOrl7mB21",
"url": "https://www.kkbox.com/tw/profile/KmTYDfo5gXOrl7mB21",
"name": "華夏金曲我最牛",
"description": "這次我要播很多 華語 類別的歌曲,快來跟我一起聽音樂吧!",
"images": [
{
"height": 75,
"width": 75,
"url": "https://i.kfs.io/muser/global/5864398v2/cropresize/75x75.jpg"
},
{
"height": 180,
"width": 180,
"url": "https://i.kfs.io/muser/global/5864398v2/cropresize/180x180.jpg"
},
{
"height": 300,
"width": 300,
"url": "https://i.kfs.io/muser/global/5864398v2/cropresize/300x300.jpg"
}
]
}
}
],
"paging": {
"offset": 0,
"limit": 50,
"previous": null,
"next": "https://api.kkbox.com/v1.1/search?limit=50&q=%E5%8F%A4%E5%85%B8%E9%9F%B3%E6%A8%82&territory=TW&type=playlist&offset=50"
},
"summary": {
"total": 358
}
},
"paging": {
"offset": 0,
"limit": 50,
"previous": null,
"next": "https://api.kkbox.com/v1.1/search?limit=50&q=%E5%8F%A4%E5%85%B8%E9%9F%B3%E6%A8%82&territory=TW&type=playlist&offset=50"
},
"summary": {
"total": 358
}
}
KKBOX HTML Widgets(link)
https://widget.kkbox.com/v1/?id={id}&type={type}
# type - {song, album, playlist}


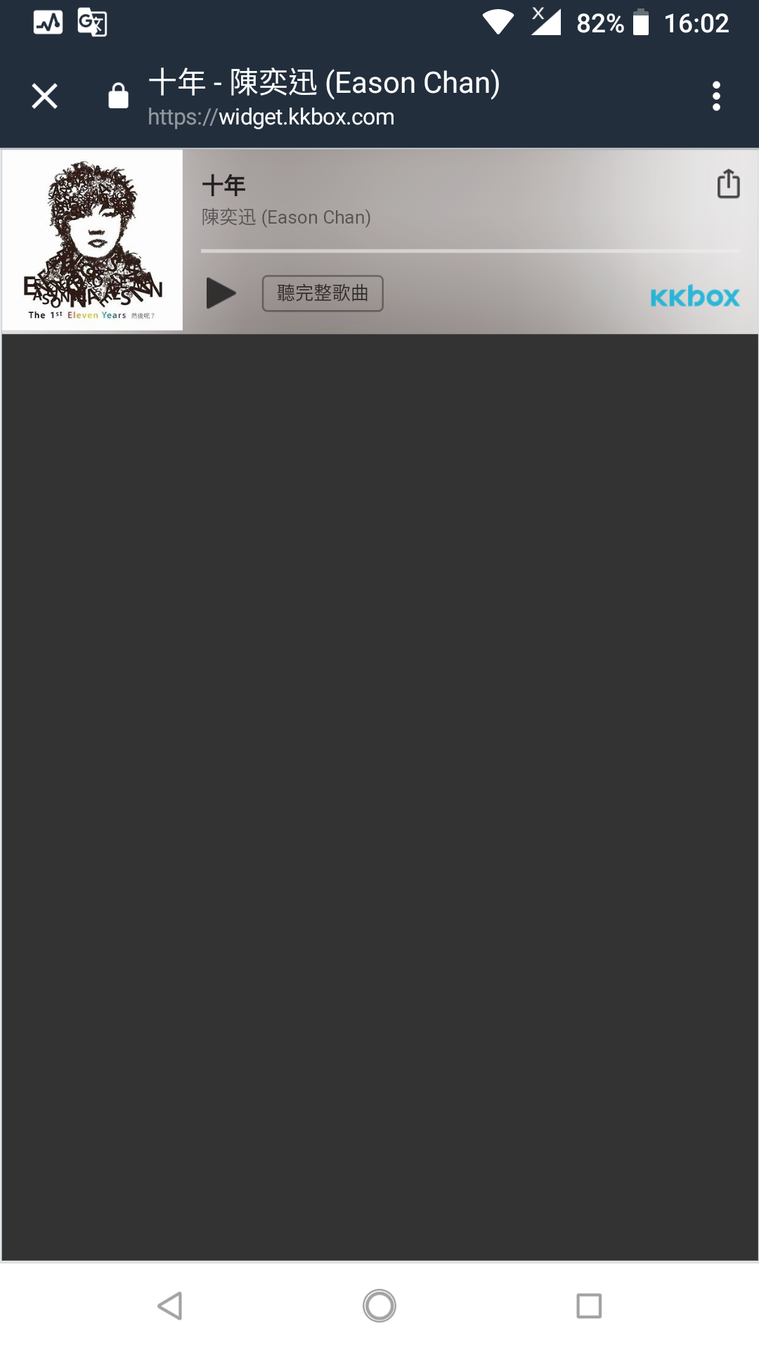
Webhook
Intent: music_play_playlist
Slots: {keyword: 古典音樂}
Third-party APIs
Weather API
KKBOX Open API
Intent C
Intent D
Intent E
Fetch Music Data
播放古典音樂類型的歌
NLU
Web Server
Telegram
Third-party APIs
Weather API
KKBOX Open API
Intent C
Intent D
Intent E
Response Data
Web Server
Generate Message
Send Message to User
Telegram
- 播放<track_name><{@=music_play_track}>
- 播放<artist_name>的歌<{@=music_play_artist}>
- 播放<album_name>專輯的歌<{@=music_play_album}>
- 播放<keyword>類型的歌<{@=music_play_playlist}>
track_name, artist_name, album_name, keyword
Intent
- music_play_track
- music_play_artist
- music_play_album
- music_play_playlist
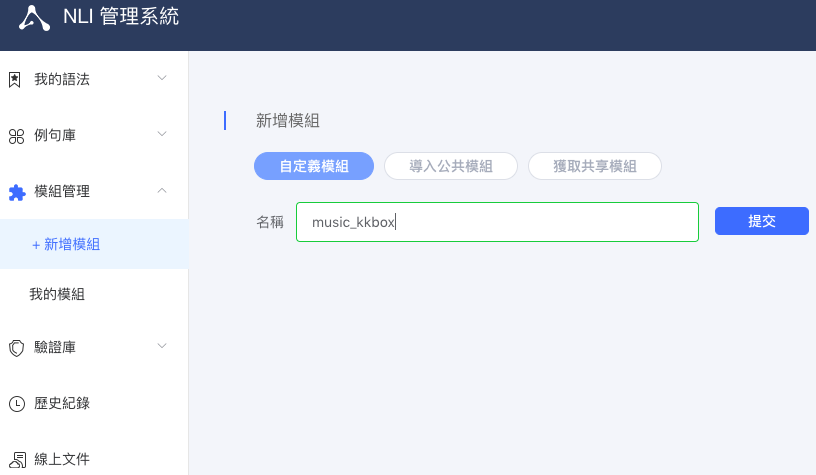



https://tw.olami.ai/open/nliclient/#/slot (Remember deploy it)



Figure out NLI API response
{
"nli": [
{
"desc_obj": {
"status": 0
},
"semantic": [
{
"app": "music_kkbox",
"input": "播放古典音樂類型的歌",
"slots": [
{
"name": "keyword",
"value": "古典音樂"
}
],
"modifier": [
"music_play_playlist"
],
"customer": "59e031f7e4b0a8057efdce99"
}
],
"type": "music_kkbox"
}
]
}

Integrate third-party API
$ mkdir api
$ vi api/__init__.py
$ vi api/kkbox.py
$ vi nlp/olami.py
Project Directory
├── api
| ├── __init__.py
| └── kkbox.py
├── nlp
| ├── __init__.py
| └── olami.py
├── config.ini
├── main.py
├── Pipfile
└── Pipfile.lock
add kkbox.py
update olami.py
add __init__.py
__init__.py
from . import kkbox
kkbox.py
import configparser
import logging
import requests
config = configparser.ConfigParser()
config.read('config.ini')
logger = logging.getLogger(__name__)
class KKBOX:
AUTH_URL = 'https://account.kkbox.com/oauth2/token'
API_BASE_URL = 'https://api.kkbox.com/v1.1/'
def __init__(self, id=config['KKBOX']['ID'], secret=config['KKBOX']['SECRET']):
self.id = id
self.secret = secret
self.token = self._get_token()
def _get_token(self):
response = requests.post(self.AUTH_URL, data={'grant_type': 'client_credentials'}, auth=(self.id, self.secret))
response.raise_for_status()
return response.json()['access_token']
def search(self, type, q, territory='TW'):
response = requests.get(self.API_BASE_URL + 'search', params={'type': type, 'q': q, 'territory': territory},
headers={'Authorization': 'Bearer ' + self.token})
response.raise_for_status()
response_json = response.json()
if type == 'artist':
return response_json['artists']['data'][0]['url']
else:
id = response_json[type + 's']['data'][0]['id']
return 'https://widget.kkbox.com/v1/?id=' + id \
+ '&type=' + ('song' if type == 'track' else type)
Update olami.py
import configparser
import json
import logging
import time
from hashlib import md5
from api.kkbox import KKBOX
import requests
config = configparser.ConfigParser()
config.read('config.ini')
logger = logging.getLogger(__name__)
class NliStatusError(Exception):
"""The NLI result status is not 'ok'"""
class Olami:
URL = 'https://tw.olami.ai/cloudservice/api'
def __init__(self, app_key=config['OLAMI']['APP_KEY'], app_secret=config['OLAMI']['APP_SECRET'], input_type=1):
self.app_key = app_key
self.app_secret = app_secret
self.input_type = input_type
def nli(self, text, cusid=None):
response = requests.post(self.URL, params=self._gen_parameters('nli', text, cusid))
response.raise_for_status()
response_json = response.json()
if response_json['status'] != 'ok':
raise NliStatusError("NLI responded status != 'ok': {}".format(response_json['status']))
else:
nli_obj = response_json['data']['nli'][0]
return self.intent_detection(nli_obj)
def _gen_parameters(self, api, text, cusid):
timestamp_ms = (int(time.time() * 1000))
params = {'appkey': self.app_key,
'api': api,
'timestamp': timestamp_ms,
'sign': self._gen_sign(api, timestamp_ms),
'rq': self._gen_rq(text)}
if cusid is not None:
params.update(cusid=cusid)
return params
def _gen_sign(self, api, timestamp_ms):
data = self.app_secret + 'api=' + api + 'appkey=' + self.app_key + 'timestamp=' + \
str(timestamp_ms) + self.app_secret
return md5(data.encode('ascii')).hexdigest()
def _gen_rq(self, text):
obj = {'data_type': 'stt', 'data': {'input_type': self.input_type, 'text': text}}
return json.dumps(obj)
def intent_detection(self, nli_obj):
def handle_selection_type(type):
if type == 'news':
return desc['result'] + '\n\n' + '\n'.join(
str(index + 1) + '. ' + el['title'] for index, el in enumerate(data))
elif type == 'poem':
return desc['result'] + '\n\n' + '\n'.join(
str(index + 1) + '. ' + el['poem_name'] + ',作者:' + el['author'] for index, el in enumerate(data))
elif type == 'cooking':
return desc['result'] + '\n\n' + '\n'.join(
str(index + 1) + '. ' + el['name'] for index, el in enumerate(data))
else:
return '對不起,你說的我還不懂,能換個說法嗎?'
def handle_music_kkbox_type(semantic):
music_type = semantic['modifier'][0].split('_')[2]
slots = semantic['slots']
kkbox = KKBOX()
def get_slot_value_by_key(key):
return next(filter(lambda el: el['name'] == key, slots))['value']
key = 'keyword' if music_type == 'playlist' else (music_type + '_name')
return kkbox.search(music_type, get_slot_value_by_key(key))
type = nli_obj['type']
desc = nli_obj['desc_obj']
data = nli_obj.get('data_obj', [])
if type == 'kkbox':
id = data[0]['id']
return ('https://widget.kkbox.com/v1/?type=song&id=' + id) if len(data) > 0 else desc['result']
elif type == 'baike':
return data[0]['description']
elif type == 'joke':
return data[0]['content']
elif type == 'news':
return data[0]['detail']
elif type == 'cooking':
return data[0]['content']
elif type == 'selection':
return handle_selection_type(desc['type'])
elif type == 'ds':
return desc['result'] + '\n請用 /help 指令看看我能怎麼幫助您'
elif type == 'music_kkbox':
return handle_music_kkbox_type(nli_obj['semantic'][0])
else:
return desc['result']
Figure out NLI API response
{
"nli": [
{
"desc_obj": {
"status": 0
},
"semantic": [
{
"app": "music_kkbox",
"input": "播放古典音樂類型的歌",
"slots": [
{
"name": "keyword",
"value": "古典音樂"
}
],
"modifier": [
"music_play_playlist"
],
"customer": "59e031f7e4b0a8057efdce99"
}
],
"type": "music_kkbox"
}
]
}

- 播放<track_name><{@=music_play_track}>
- 播放<artist_name>的歌<{@=music_play_artist}>
- 播放<album_name>專輯的歌<{@=music_play_album}>
- 播放<keyword>類型的歌<{@=music_play_playlist}>
track_name, artist_name, album_name, keyword
Intent
- music_play_track
- music_play_artist
- music_play_album
- music_play_playlist
Try it


User-friendly design
/start



Keyboard
/help

Error handling
Update main.py(link)
import configparser
import logging
import telegram
from flask import Flask, request
from telegram import ReplyKeyboardMarkup
from telegram.ext import Dispatcher, CommandHandler, MessageHandler, Filters
from nlp.olami import Olami
# Load data from config.ini file
config = configparser.ConfigParser()
config.read('config.ini')
# Enable logging
logging.basicConfig(format='%(asctime)s - %(name)s - %(levelname)s - %(message)s',
level=logging.INFO)
logger = logging.getLogger(__name__)
# Initial Flask app
app = Flask(__name__)
# Initial bot by Telegram access token
bot = telegram.Bot(token=(config['TELEGRAM']['ACCESS_TOKEN']))
welcome_message = '親愛的主人,您可以問我\n' \
'天氣,例如:「高雄天氣如何」\n' \
'百科,例如:「川普是誰」\n' \
'新聞,例如:「今日新聞」\n' \
'音樂,例如:「我想聽周杰倫的等你下課」\n' \
'日曆,例如:「現在時間」\n' \
'詩詞,例如:「我想聽水調歌頭這首詩」\n' \
'笑話,例如:「講個笑話」\n' \
'故事,例如:「說個故事」\n' \
'股票,例如:「台積電的股價」\n' \
'食譜,例如:「蛋炒飯怎麼做」\n' \
'聊天,例如:「你好嗎」'
reply_keyboard_markup = ReplyKeyboardMarkup([['播放陳奕迅的歌'],
['播放Cmon in~專輯的歌'],
['播放誰來剪月光'],
['播放金曲獎類型的歌']])
@app.route('/hook', methods=['POST'])
def webhook_handler():
"""Set route /hook with POST method will trigger this method."""
if request.method == "POST":
update = telegram.Update.de_json(request.get_json(force=True), bot)
dispatcher.process_update(update)
return 'ok'
def start_handler(bot, update):
"""Send a message when the command /start is issued."""
update.message.reply_text(welcome_message, reply_markup=reply_keyboard_markup)
def help_handler(bot, update):
"""Send a message when the command /help is issued."""
update.message.reply_text(welcome_message, reply_markup=reply_keyboard_markup)
def reply_handler(bot, update):
"""Reply message."""
text = update.message.text
user_id = update.message.from_user.id
reply = Olami().nli(text, user_id)
update.message.reply_text(reply)
def error_handler(bot, update, error):
"""Log Errors caused by Updates."""
logger.error('Update "%s" caused error "%s"', update, error)
update.message.reply_text('對不起唷~ 我需要多一點時間來處理 Q_Q')
# New a dispatcher for bot
dispatcher = Dispatcher(bot, None)
# Add handlers for handling message, there are many kinds of message. For this handler, it particular handle text
# message.
dispatcher.add_handler(MessageHandler(Filters.text, reply_handler))
dispatcher.add_handler(CommandHandler('start', start_handler))
dispatcher.add_handler(CommandHandler('help', help_handler))
dispatcher.add_error_handler(error_handler)
if __name__ == "__main__":
# Running server
app.run(debug=True)
Deployment

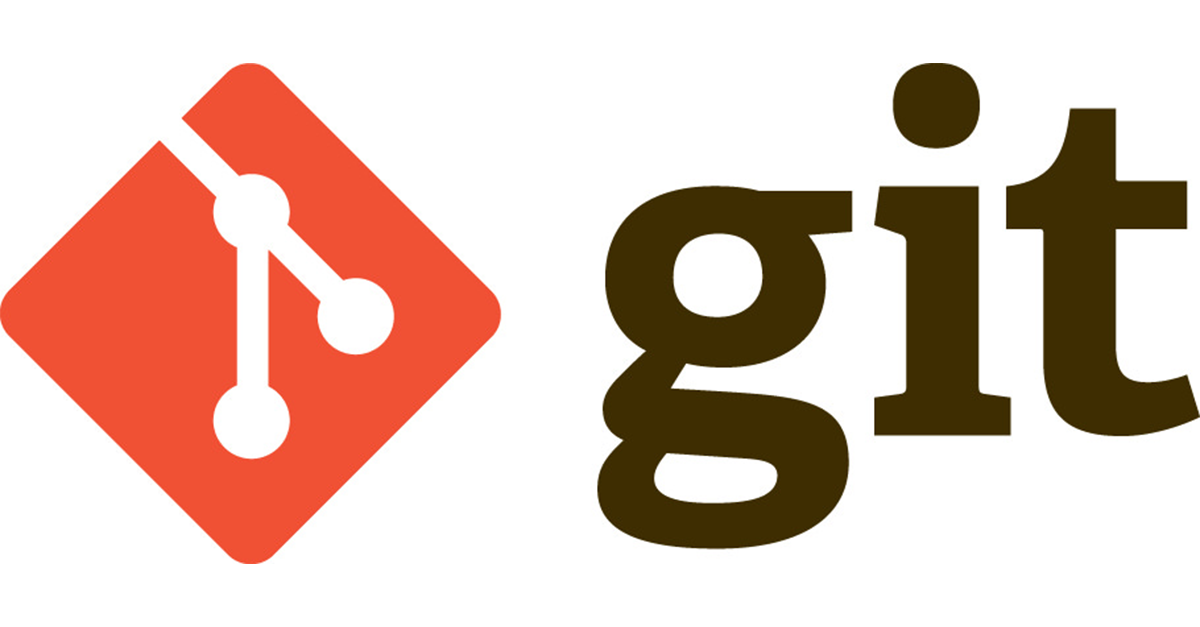
Deploy to Heroku(link)
add Procfile
web: gunicorn main:app --log-file -
$ vi Procfile
$ vi .gitignore
Project Directory
├── api
| ├── __init__.py
| └── kkbox.py
├── nlp
| ├── __init__.py
| └── olami.py
├── config.ini
├── main.py
├── Pipfile
├── Pipfile.lock
└── Procfile
.gitignore
### Python template
# Byte-compiled / optimized / DLL files
__pycache__/
*.py[cod]
*$py.class
# C extensions
*.so
# Distribution / packaging
.Python
build/
develop-eggs/
dist/
downloads/
eggs/
.eggs/
lib/
lib64/
parts/
sdist/
var/
wheels/
*.egg-info/
.installed.cfg
*.egg
MANIFEST
# PyInstaller
# Usually these files are written by a python script from a template
# before PyInstaller builds the exe, so as to inject date/other infos into it.
*.manifest
*.spec
# Installer logs
pip-log.txt
pip-delete-this-directory.txt
# Unit test / coverage reports
htmlcov/
.tox/
.coverage
.coverage.*
.cache
nosetests.xml
coverage.xml
*.cover
.hypothesis/
.pytest_cache/
# Translations
*.mo
*.pot
# Django stuff:
*.log
local_settings.py
db.sqlite3
# Flask stuff:
instance/
.webassets-cache
# Scrapy stuff:
.scrapy
# Sphinx documentation
docs/_build/
# PyBuilder
target/
# Jupyter Notebook
.ipynb_checkpoints
# pyenv
.python-version
# celery beat schedule file
celerybeat-schedule
# SageMath parsed files
*.sage.py
# Environments
.env
.venv
env/
venv/
ENV/
env.bak/
venv.bak/
# Spyder project settings
.spyderproject
.spyproject
# Rope project settings
.ropeproject
# mkdocs documentation
/site
# mypy
.mypy_cache/
### JetBrains template
# Covers JetBrains IDEs: IntelliJ, RubyMine, PhpStorm, AppCode, PyCharm, CLion, Android Studio and WebStorm
# Reference: https://intellij-support.jetbrains.com/hc/en-us/articles/206544839
# User-specific stuff
.idea/**/workspace.xml
.idea/**/tasks.xml
.idea/**/dictionaries
.idea/**/shelf
# Sensitive or high-churn files
.idea/**/dataSources/
.idea/**/dataSources.ids
.idea/**/dataSources.local.xml
.idea/**/sqlDataSources.xml
.idea/**/dynamic.xml
.idea/**/uiDesigner.xml
# Gradle
.idea/**/gradle.xml
.idea/**/libraries
# CMake
cmake-build-debug/
cmake-build-release/
# Mongo Explorer plugin
.idea/**/mongoSettings.xml
# File-based project format
*.iws
# IntelliJ
out/
# mpeltonen/sbt-idea plugin
.idea_modules/
# JIRA plugin
atlassian-ide-plugin.xml
# Cursive Clojure plugin
.idea/replstate.xml
# Crashlytics plugin (for Android Studio and IntelliJ)
com_crashlytics_export_strings.xml
crashlytics.properties
crashlytics-build.properties
fabric.properties
# Editor-based Rest Client
.idea/httpRequests
### macOS template
# General
.DS_Store
.AppleDouble
.LSOverride
# Icon must end with two \r
Icon
# Thumbnails
._*
# Files that might appear in the root of a volume
.DocumentRevisions-V100
.fseventsd
.Spotlight-V100
.TemporaryItems
.Trashes
.VolumeIcon.icns
.com.apple.timemachine.donotpresent
# Directories potentially created on remote AFP share
.AppleDB
.AppleDesktop
Network Trash Folder
Temporary Items
.apdisk
### VirtualEnv template
# Virtualenv
# http://iamzed.com/2009/05/07/a-primer-on-virtualenv/
.Python
[Bb]in
[Ii]nclude
[Ll]ib
[Ll]ib64
[Ll]ocal
[Ss]cripts
pyvenv.cfg
.venv
pip-selfcheck.json
.idea
Deploy to Heroku
$ git init # 初始化版本控制
$ git checkout -b production # 創建並切換至 production 分支
$ git add . # 將目錄下所有檔案加入版本控制
# 如果你第一次使用 Git,需要用以下兩行指令設定 yourname 及 email
$ git config --global user.name "yourname"
$ git config --global user.email "yourname@example.com"
$ git commit -m "Deploying to Heroku" # 建立快照
$ heroku login # Heroku 認證登入
$ heroku git:remote -a {your_heroku_app_name} # 加入 Heroku remote repository
$ git push heroku production:master # 將本地的 production 分支內容 push 至 Heroku master 分支
Setting production webhook
# 在瀏覽器網址列中輸入
https://api.telegram.org/bot{$token}/setWebhook?url={$webhook_url}
# 舉例,記得 heroku 的網址 '/' 後面要加 'hook'
https://api.telegram.org/bot739453631:AAGeIaHIDQ1L48_q8uq7woWvLEw7zyFs0IA/setWebhook?url=https://kkbox-telegram-bot.herokuapp.com/hook
# 看到網頁出現以下訊息表示設定成功
{
ok: true,
result: true,
description: "Webhook was set"
}





All works are done!




Innovation Chatbot
救救最危險的一席!
-
FB 公開貼文
-
寫下簡短課後心得
-
附上這份簡報連結 https://goo.gl/sMi2By
-
辛苦了一整天,向朋友展示一下自己的成果吧!附上 Chatbot 連結,例如 https://t.me/innovation_chatbot
-
最後填寫回饋問券 https://goo.gl/forms/GQkbWiW5dwMph1K62
- 貼文即送手機扣環;兩週後(12/15),根據該貼文按讚*1+留言*1+被公開分享次數*3 計分,前 3 名送行動電源!
Free communication
Telegram Bot Workshop
By zaoldyeck
Telegram Bot Workshop
- 4,397